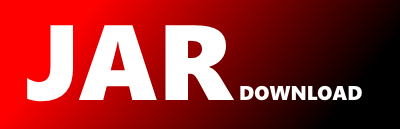
org.enhydra.xml.xmlc.codegen.JavaCompile Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: JavaCompile.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.codegen;
import java.io.PrintWriter;
import java.util.ArrayList;
import org.enhydra.xml.io.ErrorReporter;
import org.enhydra.xml.xmlc.XMLCException;
import org.enhydra.xml.xmlc.misc.ProcessRunner;
//FIXME: This could be integrated with JavaClass.
/**
* Run a Java compiler as a child process.
*/
public class JavaCompile extends ProcessRunner {
/**
* Default options.
*/
private static final int DEFAULT_OPTIONS = PASS_STDOUT|PASS_STDERR;
/**
* Default java compiler command.
*/
private static final String DEFAULT_JAVAC = "javac";
/**
* Error handler to use.
*/
private ErrorReporter fErrorReporter;
/**
* Java program.
*/
private String fJavac;
/**
* Class output root directory.
*/
private String fClassOutputRoot;
/**
* General argument command to execute.
*/
private ArrayList fArgs = new ArrayList();
/**
* Source files.
*/
private ArrayList fSrcs = new ArrayList();
/**
* Construct a javac runner, specifying a java compiler.
*/
public JavaCompile(ErrorReporter errorReporter,
String javac) {
super(DEFAULT_OPTIONS);
fErrorReporter = errorReporter;
if (javac != null) {
fJavac = javac;
} else {
fJavac = DEFAULT_JAVAC;
}
}
/**
* Set the class output root.
*/
public void setClassOutputRoot(String root) {
fClassOutputRoot = root;
}
/**
* Add an argument.
*/
public void addArg(String arg) {
fArgs.add(arg);
}
/**
* Add an argument and associate value.
*/
public void addArg(String arg,
String value) {
fArgs.add(arg);
fArgs.add(value);
}
/**
* Add an array of arguments.
*/
public void addArgs(String[] args) {
for (int i = 0; i < args.length; i++) {
fArgs.add(args[i]);
}
}
/**
* Add a source file to compile.
*/
public void addSrc(String srcFile) {
fSrcs.add(srcFile);
}
/**
* Run the javac process. Stdout/stderr are written to the standard
* descriptors.
*
* @param metaData Document metadata.
* @param errorReporter Write errors to this object.
* @param verboseOut Write verbose message to this file if not null.
*/
public void compile(PrintWriter verboseOut) throws XMLCException {
ArrayList cmd = new ArrayList();
cmd.add(fJavac);
if (fClassOutputRoot != null) {
cmd.add("-d");
cmd.add(fClassOutputRoot);
}
cmd.addAll(fArgs);
cmd.addAll(fSrcs);
// Run process
run((String[])cmd.toArray(new String[cmd.size()]),
fErrorReporter, verboseOut,
"compile of generated java code failed");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy