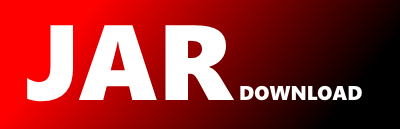
org.enhydra.xml.xmlc.codegen.JavaLang Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: JavaLang.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.codegen;
import java.io.File;
import java.util.StringTokenizer;
/**
* Collection of methods and constants dealing with the Java language.
*/
public final class JavaLang {
/**
* Indentation amount, as a string of blanks.
*/
public static final String INDENT_STR = " ";
/**
* Line seperator to use on this platform
*/
public static final char lineSeparator;
/*
* Class initializer.
*/
static {
String lineSepStr = System.getProperty("line.separator");
lineSeparator = ((lineSepStr != null) && (lineSepStr.length() > 0))
? lineSepStr.charAt(0) : '\n';
}
/**
* Prevent instanciation.
*/
private JavaLang() {
}
/*
* Parse a class or package name.
*/
public static String[] parseClassName(String name) {
StringTokenizer tokens = new StringTokenizer(name, ".");
String[] names = new String[tokens.countTokens()];
for (int i = 0; i < names.length; i++) {
names[i] = tokens.nextToken();
}
return names;
}
/*
* Get the class name without the package.
* @param obj Any object or class object.
*/
public static String simpleClassName(Object obj) {
if (obj instanceof Class) {
return simpleClassName(((Class)obj).getName());
} else {
return simpleClassName(obj.getClass().getName());
}
}
/*
* Get the class name without the package.
*/
public static String simpleClassName(String className) {
int lastDot = className.lastIndexOf('.');
if (lastDot < 0) {
return className;
} else {
return className.substring(lastDot+1);
}
}
/*
* Get the package name of a class, or null if the default
* package is used.
*/
public static String getPackageName(String className) {
int lastDot = className.lastIndexOf('.');
if (lastDot < 0) {
return "";
} else {
return className.substring(0, lastDot);
}
}
/**
* Convert a package name to a relative platform file name.
*/
public static String packageNameToFileName(String packageName) {
return packageName.replace('.', File.separatorChar);
}
/**
* Convert a package name to a relative Unix file name.
*/
public static String packageNameToUnixFileName(String packageName) {
return packageName.replace('.', '/');
}
/**
* Convert a unicode character to its \\uXXXX representation
* and append to a buffer.
*/
private static void toUnicodeEncoding(char ch, StringBuffer buf) {
String numStr = Integer.toHexString(ch);
buf.append("\\u");
for (int cnt = 4-numStr.length(); cnt > 0; cnt--) {
buf.append('0');
}
buf.append(numStr);
}
/**
* Generate a Java string constant, quoting `\' and `"' and converting
* newlines.
*
* @param text Text to quote.
* @return The quoted string.
*/
public static String createStringConst(String text) {
if (text == null) {
return "null";
}
StringBuffer str = new StringBuffer();
int len = text.length ();
str.append ("\"");
for (int idx = 0; idx < len; idx++) {
char textChar = text.charAt (idx);
switch (textChar) {
case '\\':
case '"':
str.append ('\\');
str.append (textChar);
break;
case '\t':
str.append ("\\t");
break;
case '\n':
str.append ("\\n");
break;
case '\r':
str.append ("\\r");
break;
default:
if ((textChar > 127) || (Character.isISOControl(textChar))) {
toUnicodeEncoding(textChar, str);
} else {
str.append (textChar);
}
break;
}
}
str.append ("\"");
return str.toString();
}
/*
* Determine if a string is a legal Java identifier
*/
public static boolean legalJavaIdentifier(String str) {
if (str.length() == 0) {
return false;
}
if (!Character.isJavaIdentifierStart(str.charAt(0))) {
return false;
}
for (int j = 1; j < str.length(); j++) {
if (!Character.isJavaIdentifierPart(str.charAt(j))) {
return false;
}
}
return true;
}
/**
* Construct a white-space string of a given length to use
* for indentation.
*/
public static String makeIndent(int len) {
StringBuffer prefix = new StringBuffer();
for (int idx = 0; idx < len; idx++) {
prefix.append(' ');
}
return prefix.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy