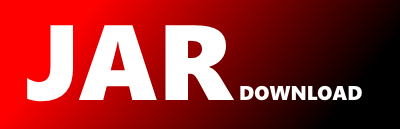
org.enhydra.xml.xmlc.codegen.JavaMethod Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: JavaMethod.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.codegen;
//FIXME: string arrays could be string buffers, since printer handles newlines.
/**
* Object that is used to assemble the Java source for a method.
*/
public final class JavaMethod {
/** The method name, or null for class constructor */
private String fName;
/** The return type */
private String fReturnType;
/** Modifiers of the method */
protected int fModifiers;
/** Parameters */
private JavaParameter[] fParameters;
/** Documentation */
private String[] fDoc;
/** Code body */
private JavaCode fCode = new JavaCode();
/**
* Adjust modifiers based on other modifiers.
*/
private static int adjustModifiers(String returnType,
int modifiers) {
// Only public methods are include in interfaces.
if ((modifiers & (JavaModifiers.PROTECTED|JavaModifiers.PRIVATE)) != 0) {
modifiers |= JavaModifiers.OMIT_INTERFACE;
}
// Only static methods are omitted from interfaces.
if ((modifiers & JavaModifiers.STATIC) != 0) {
modifiers |= JavaModifiers.OMIT_INTERFACE;
}
// Constructors and static initializers are omitted from the interface
if (returnType == null) {
modifiers |= JavaModifiers.OMIT_INTERFACE;
}
return modifiers;
}
/**
* Constructor.
*
* @param returnType The type the method returns. Specify null for a
* constructor.
* @param name The name of the method. If name is null, then this
* creates a static initializer.
* @param parameters A array describing the parameters; null or empty if
* no parameters.
* @param modifiers The method modifier bit set.
* @param doc The documentation to include. The parameter documentation
* will be appended to this, so should not be repeated.
* Each row of the array is a line of documentation.
*/
//FIXME: reorder arguments to be like java method def order.
public JavaMethod(String name,
String returnType,
int modifiers,
JavaParameter[] parameters,
String[] doc) {
fName = name;
fReturnType = returnType;
fModifiers = adjustModifiers(returnType, modifiers);
if (parameters != null) {
fParameters = (JavaParameter[])parameters.clone();
}
fDoc = (String[])doc.clone();
}
/**
* Get the code object associate with the method body.
*/
public JavaCode getCode() {
return fCode;
}
/**
* Get the name.
*/
public String getName() {
return fName;
}
/**
* Get the return type.
*/
public String getReturnType() {
return fReturnType;
}
/**
* Get the modifiers.
*/
public int getModifiers() {
return fModifiers;
}
/**
* Print the method signature. This is public, since it used in
* generating method listings as well as code.
*
* @param out Write to this stream.
* @param beginBody If true, start a method body, otherwise end with
* a semicolon.
*/
public void printMethodSignature(IndentWriter out,
boolean beginBody) {
int numParams = (fParameters == null) ? 0 : fParameters.length;
// Assemble upto `(' so length can be determined for indentation
StringBuffer start = new StringBuffer(JavaModifiers.toDecl(fModifiers));
if (fReturnType != null) {
start.append(fReturnType);
start.append(' ');
}
start.append(fName);
start.append('(');
out.print(start);
// Construct indentation string for subsequent parameters if needed.
String indent = (numParams == 0) ? "" : JavaLang.makeIndent(start.length());
// Output parameters
for (int idx = 0; idx < numParams; idx++) {
if (idx > 0) {
out.print(indent);
}
fParameters[idx].printDefinition(out);
if (idx < (numParams - 1)) {
out.println(',');
}
}
if (beginBody) {
out.println(") {");
} else {
out.println(");");
}
}
/**
* Print the method.
*/
public void print(IndentWriter out,
boolean printBody) {
if (fDoc != null) {
out.println("/**");
for (int idx = 0; idx < fDoc.length; idx++) {
out.print(" * ");
out.println(fDoc[idx]);
}
out.println(" */");
}
if (fName != null) {
printMethodSignature(out, printBody);
} else {
out.println("static {");
}
if (printBody) {
out.enter();
fCode.print(out);
out.leave();
out.println("}");
}
out.println();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy