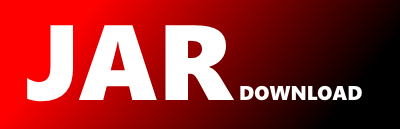
org.enhydra.xml.xmlc.codegen.JavaModifiers Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: JavaModifiers.java,v 1.1.1.1 2003/03/10 16:36:19 taweili Exp $
*/
package org.enhydra.xml.xmlc.codegen;
/**
* Definitions of Java modifiers for methods, fields and classes.
*/
public class JavaModifiers {
/**
* Public access modifier flag.
*/
public static int PUBLIC = 0x01;
/**
* Protected access modifier flag.
*/
public static int PROTECTED = 0x02;
/**
* Private access modifier flag.
*/
public static int PRIVATE = 0x04;
/**
* Class scope modifier flag.
*/
public static int STATIC = 0x08;
/**
* Unmodifiable scope modifier flag.
*/
public static int FINAL = 0x10;
/**
* Abstract modifier flag.
*/
public static int ABSTRACT = 0x20;
/**
* Don't include field or method in implementation if generating
* an interface and an implementation.
*/
public static int OMIT_IMPLEMENTATION = 0x40;
/**
* Don't include field or method in interface if generating
* an interface and an implementation.
*/
public static int OMIT_INTERFACE = 0x80;
/**
* Common modifiers for public constants. Shorthand for
* PUBLIC | STATIC | FINAL.
*/
public static int PUBLIC_CONST = PUBLIC | STATIC | FINAL;
/**
* Common modifiers for private constants. Shorthand for
* PRIVATE | STATIC | FINAL.
*/
public static int PRIVATE_CONST = PRIVATE | STATIC | FINAL;
/**
* Prevent instantiation.
*/
private JavaModifiers() {
}
/**
* Convert a modifier set to a string prefix for a declaration.
* The result includes a trailing blank if modifier is included.
*/
public static String toDecl(int modifiers) {
if (modifiers == 0) {
// Optimize for no modifiers.
return "";
}
StringBuffer str = new StringBuffer();
if ((modifiers & PUBLIC) != 0) {
str.append("public");
str.append(' ');
}
if ((modifiers & PROTECTED) != 0) {
str.append("protected");
str.append(' ');
}
if ((modifiers & PRIVATE) != 0) {
str.append("private");
str.append(' ');
}
if ((modifiers & STATIC) != 0) {
str.append("static");
str.append(' ');
}
if ((modifiers & FINAL) != 0) {
str.append("final");
str.append(' ');
}
if ((modifiers & ABSTRACT) != 0) {
str.append("abstract");
str.append(' ');
}
return str.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy