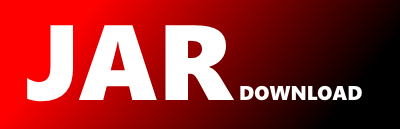
org.enhydra.xml.xmlc.commands.ErrorHandling Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: ErrorHandling.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.commands;
import java.io.FileNotFoundException;
import java.io.IOException;
import org.enhydra.error.ChainedThrowable;
import org.xml.sax.SAXException;
/**
* Error handling methods for use in commands.
*/
public class ErrorHandling {
/*
* Disallow instantiation.
*/
private ErrorHandling() {
}
/*
* Print out a stack trace with special handling of SAX exceptions.
*/
private static void printStackTrace(Throwable except) {
except.printStackTrace();
// If this is a chained exception, then walk the stack
// looking for a non-chained exception.
while ((except != null) && (except instanceof ChainedThrowable)) {
except = ((ChainedThrowable)except).getCause();
}
if ((except != null) && (except instanceof SAXException)) {
Exception except2 = ((SAXException)except).getException();
if (except2 != null) {
printStackTrace(except2);
}
}
}
/*
* Handle an exception, printing the approriate error
* message and exit the program
*
* @param except Exception or error to print.
* @param verbose If true, print all details of the error.
*/
public static void handleException(Throwable except,
boolean verbose) {
if (except instanceof ChainedThrowable) {
System.err.println("Error: " + except.getMessage());
if (verbose) {
printStackTrace(except);
}
// If this is result of a java.lang exception, print stack.
Throwable cause = ((ChainedThrowable)except).getCause();
if ((!verbose) && (cause != null)
&& cause.getClass().getName().startsWith("java.lang.")) {
printStackTrace(cause);
}
} else if ((except instanceof FileNotFoundException)
|| (except instanceof IOException)) {
System.err.println("Error: " + except.toString());
if (verbose) {
printStackTrace(except);
}
} else {
System.err.println("Error: " + except.toString());
printStackTrace(except);
}
System.exit(1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy