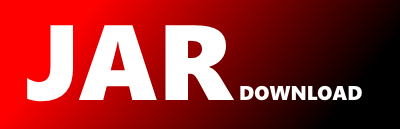
org.enhydra.xml.xmlc.commands.options.Option Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: Option.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.commands.options;
import org.enhydra.xml.io.ErrorReporter;
import org.enhydra.xml.xmlc.XMLCException;
/**
* Definition of an option. The general or specific options are classes
* derived from this class. A general class is parameterizes at instantiation
* and is able to create parse multiple options. A specific class only parses
* one option. The Option classes should be immutable; a generic client data
* object is pass to the parse methods to recieve the configuration.
*/
public abstract class Option {
/**
* The option name, including the leading `-'.
*/
protected final String name;
/**
* The number of arguments for the option.
*/
protected final int numArgs;
/**
* Are multiple instances of the option allowed?
*/
protected final boolean multipleAllowed;
/**
* Help message for the option.
*/
protected String help;
/**
* Construct a new instance of the object.
*
* @param name Name of the option, including the leading `-'.
* @param numArgs Number of arguments the option takes.
* @param multipleAllowed Can the option be specified multiple times?
* @param help A string that is used in generating the help message.
* It should include the arguments, a `-', then one line message. The
* option name and a space will be prepended.
*/
public Option(String name,
int numArgs,
boolean multipleAllowed,
String help) {
this.name = name;
this.numArgs = numArgs;
this.multipleAllowed = multipleAllowed;
this.help = help;
}
/**
* Get the option name, including leading `-'.
*/
public String getName() {
return name;
}
/**
* Get the number of arguments for a single instance of the option. If
* the option repeats, the actual number of arguments is a multiple of
* this.
*/
public int getNumArgs() {
return numArgs;
}
/**
* Return true if the option maybe specified multiple times, resulting
* in a list of values. Return false if the option can only be specified
* once.
*/
public boolean getMultipleAllowed() {
return multipleAllowed;
}
/**
* Get the help string, including option name.
*/
public String getHelp() {
return name + " " + help;
}
/**
* Determine if two options are equal. Only the name is considered. It
* is illegal to have options with the same name and different attributes.
*
* @param obj This can be either another Option object or a string. If
* an Option object is supplied, the names are compared.
* @return True if this object name is the same as the name in
*/
public boolean equals(Object obj) {
if (obj instanceof Option) {
return name.equals(((Option)obj).getName());
} else if (obj instanceof String) {
return name.equals((String)obj);
} else {
return false;
}
}
/**
* Get the hashcode for the object.
*/
public int hashCode() {
return name.hashCode();
}
/**
* Parse an instance of the option.
*
* @param args The option's arguments. Will always have the number of
* arguments defined for an option.
* @param errorReporter Used to report warnings, such as deprecated
* options. Errors should be thrown.
* @param clientData Object that is passed to the parser. Normally
* contains the object to store the configuration in.
*/
abstract protected void parse(String[] args,
ErrorReporter errorReporter,
Object clientData) throws XMLCException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy