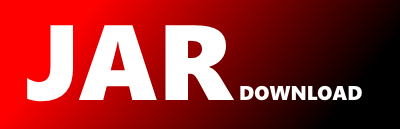
org.enhydra.xml.xmlc.commands.options.OptionFileParser Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: OptionFileParser.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.commands.options;
import java.io.IOException;
import java.io.Reader;
import java.io.StreamTokenizer;
import java.util.Vector;
import org.enhydra.xml.io.InputSourceOps;
import org.enhydra.xml.xmlc.XMLCException;
import org.xml.sax.InputSource;
/**
* Parse an XMLC options file. This creates a vector of options and
* arguments. It does no other processing on options.
*/
class OptionFileParser {
// Vector of entries in the file.
private Vector entries = new Vector();
/**
* Parse a line in the file.
*
* @return true if a line was parsed, false on EOF.
*/
private boolean parseLine(StreamTokenizer in) throws IOException {
in.nextToken();
if (in.ttype == StreamTokenizer.TT_EOF) {
return false; // EOF
}
// Check for comment or blank line.
if (in.ttype == StreamTokenizer.TT_EOL) {
return true; // EOL
}
if (in.sval.startsWith("#")) {
// Eat comment line
do {
in.nextToken();
} while (!((in.ttype == StreamTokenizer.TT_EOF)
|| (in.ttype == StreamTokenizer.TT_EOL)));
return true;
}
// Parse option and arguments.
Vector tokens = new Vector();
do {
tokens.addElement(in.sval);
in.nextToken();
} while (!((in.ttype == StreamTokenizer.TT_EOF)
|| (in.ttype == StreamTokenizer.TT_EOL)));
if (tokens.size() >= 0) {
String[] optEntry = new String[tokens.size()];
tokens.copyInto(optEntry);
entries.addElement(optEntry);
}
return true;
}
/**
* Parse an open options file.
*/
private void parse(Reader reader) throws IOException {
StreamTokenizer in = new StreamTokenizer(reader);
in.resetSyntax();
in.eolIsSignificant(true);
in.whitespaceChars('\u0000', '\u0020');
in.wordChars('\u0021', '\uffff');
in.quoteChar('"');
in.quoteChar('\'');
while (parseLine(in)) {
continue;
}
}
/**
* Construct, parsing contents of file.
*/
public OptionFileParser(Reader reader)
throws XMLCException, IOException {
parse(reader);
}
/**
* Construct, parsing contents of file.
*/
public OptionFileParser(InputSource inputSource)
throws XMLCException {
try {
Reader reader = InputSourceOps.open(inputSource);
try {
parse(reader);
} finally {
InputSourceOps.closeIfOpened(inputSource, reader);
}
} catch (IOException except) {
throw new XMLCException("parse of option failed" + inputSource,
except);
}
}
/**
* Get the options that were parsed.
*
* @return An array of string arrays, each representing an
* option and its arguments.
*/
public String[][] getOptions() {
String[][] opts = new String[entries.size()][];
entries.copyInto(opts);
return opts;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy