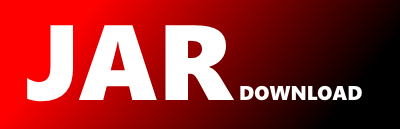
org.enhydra.xml.xmlc.commands.xmlc.ParserCmdOptions Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: ParserCmdOptions.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.commands.xmlc;
import org.enhydra.xml.io.ErrorReporter;
import org.enhydra.xml.xmlc.XMLCException;
import org.enhydra.xml.xmlc.commands.options.BooleanOption;
import org.enhydra.xml.xmlc.commands.options.Option;
import org.enhydra.xml.xmlc.commands.options.OptionSet;
import org.enhydra.xml.xmlc.metadata.MetaData;
import org.enhydra.xml.xmlc.metadata.ParserType;
import org.enhydra.xml.xmlc.metadata.XCatalog;
/**
* Command line options parsing parser options.
*/
class ParserCmdOptions extends BaseCmdOptions {
/**
* Class for the -parser option.
*/
private class ParserOption extends Option {
/**
* Constructor.
*/
public ParserOption() {
super("-parser", 1, false,
"type - Specify the parser to use (tidy, swing, xerces).");
}
/**
* Parse an instance of the option.
*/
protected void parse(String[] args,
ErrorReporter errorReporter,
Object clientData) throws XMLCException {
ParserType parser;
try {
parser = ParserType.getType(args[0].toLowerCase());
} catch (IllegalArgumentException except) {
throw new XMLCException(except.getMessage(),
except);
}
((MetaData)clientData).getParser().setName(parser);
}
}
/**
* Class for the -xcatalog option.
*/
private class XCatalogOption extends Option {
/**
* Constructor.
*/
public XCatalogOption() {
super("-xcatalog", 1, true,
"catalog - XCatalog file to resolve external entities.");
}
/**
* Parse an instance of the option.
*/
protected void parse(String[] args,
ErrorReporter errorReporter,
Object clientData) throws XMLCException {
XCatalog xCatalog = ((MetaData)clientData).getParser().addXCatalog();
xCatalog.setUrl(args[0]);
}
}
/**
* Class for the -validate option.
*/
private class ValidateOption extends BooleanOption {
/**
* Constructor.
*/
public ValidateOption() {
super("-validate",
"yes|no|true|false - Changes the default document validation mode of the parser");
}
/**
* Set the value.
*/
protected void set(boolean value,
Object clientData) throws XMLCException {
((MetaData)clientData).getParser().setValidate(value ? Boolean.TRUE : Boolean.FALSE);
}
}
/**
* Constructor. Add options to option set.
*/
public ParserCmdOptions(OptionSet optionSet) {
super(optionSet);
optionSet.addOption(new ParserOption());
optionSet.addOption(new XCatalogOption());
optionSet.addOption(new ValidateOption());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy