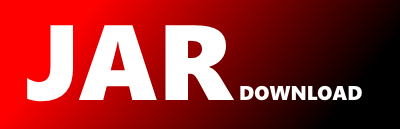
org.enhydra.xml.xmlc.commands.xmlc.XMLC Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: XMLC.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.commands.xmlc;
import java.io.IOException;
import java.io.PrintWriter;
import org.enhydra.xml.io.ErrorReporter;
import org.enhydra.xml.xmlc.XMLCException;
import org.enhydra.xml.xmlc.XMLCVersion;
import org.enhydra.xml.xmlc.commands.ErrorHandling;
import org.enhydra.xml.xmlc.compiler.Compiler;
import org.enhydra.xml.xmlc.metadata.CompileOptions;
import org.enhydra.xml.xmlc.metadata.JavaCompilerSection;
import org.enhydra.xml.xmlc.metadata.MetaData;
/**
* XMLC compiler program. See the user manual for details on the command
* line. Will look for a system property `org.enhydra.xml.xmlc.javac' to find
* the compiler to use if -javac is not specified.
*/
public class XMLC {
/**
* System property to pass default javac.
*/
public static final String JAVAC_PROPERTY = "org.enhydra.xml.xmlc.javac";
/**
* Command line parsing object.
*/
private XMLCOptionsParser fOptionsParser = new XMLCOptionsParser();
/**
* Enable verbose output.
*/
private boolean fVerbose = false;
/*
* Stream to use for stdout.
*/
private PrintWriter fStdout;
/*
* Error output.
*/
private ErrorReporter fErrorReporter;
/*
* Trace and verbose output.
*/
private PrintWriter fTraceOut;
/**
* Constructor specifying output stream for error messages.
*/
public XMLC(PrintWriter stdout,
PrintWriter stderr) {
fStdout = stdout;
fErrorReporter = new ErrorReporter(stderr);
fTraceOut = stderr;
}
/**
* Constructor with standard output streams.
*/
public XMLC() {
this(new PrintWriter(System.out, true),
new PrintWriter(System.err, true));
}
/*
* Print the XMLC version number.
*/
private void printVersion() {
fStdout.println("Enhydra XMLC version " + XMLCVersion.VERSION);
fStdout.println("See http://xmlc.enhydra.org for latest distribution");
}
/**
* Parse arguments.
*/
private MetaData parseArgs(String[] args) throws XMLCException, IOException {
MetaData metaData = fOptionsParser.parse(args, fErrorReporter);
// Default javac if supplied by a property.
String defaultJavac = System.getProperty(JAVAC_PROPERTY);
if (defaultJavac != null) {
JavaCompilerSection compilerSection = metaData.getJavaCompilerSection();
if (!compilerSection.isJavacSpecified()) {
compilerSection.setJavac(defaultJavac);
}
}
return metaData;
}
/**
* Parse arguments and compile the page. If this method is used,
* errors are thrown, exit will not be called.
*/
public void compile(String[] args) throws XMLCException, IOException {
// Setup and document parsing.
MetaData metaData = parseArgs(args);
CompileOptions compileOptions = metaData.getCompileOptions();
fVerbose = compileOptions.getVerbose();
if (compileOptions.getPrintVersion()) {
printVersion();
}
if (metaData.getInputDocument().getUrl() != null) {
Compiler compiler = new Compiler(fErrorReporter,
fTraceOut);
compiler.compile(metaData);
}
}
/*
* Parse arguments and compile the page. Print errors that
* are thrown.
*/
private void compileHandleErrors(String[] args) {
try {
compile(args);
} catch (Exception except) {
ErrorHandling.handleException(except, fVerbose);
}
}
/*
* Program entry point.
*/
public static void main(String[] args) {
XMLC xmlc = new XMLC();
xmlc.compileHandleErrors(args);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy