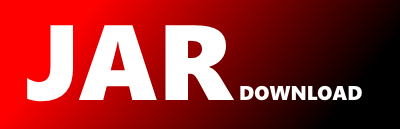
org.enhydra.xml.xmlc.compiler.AccessConsts Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: AccessConsts.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
/*
* Enhydra Java Application Server
* The Initial Developer of the Original Code is Lutris Technologies Inc.
* Portions created by Lutris are Copyright (C) 1997-2000 Lutris Technologies
* Inc.
* All Rights Reserved.
*
* The contents of this file are subject to the Enhydra Public License Version
* 1.0 (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at
* http://www.enhydra.org/software/license/epl.html
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
* License for the specific language governing rights and limitations under the
* License.
*
* $Id: AccessConsts.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.compiler;
import java.io.PrintWriter;
import java.util.Iterator;
import java.util.TreeMap;
import org.enhydra.xml.xmlc.codegen.JavaClass;
import org.enhydra.xml.xmlc.codegen.JavaField;
import org.enhydra.xml.xmlc.codegen.JavaLang;
import org.enhydra.xml.xmlc.codegen.JavaModifiers;
import org.enhydra.xml.xmlc.metadata.HTMLCompatibility;
import org.enhydra.xml.xmlc.metadata.HTMLSection;
import org.enhydra.xml.xmlc.metadata.MetaData;
/**
* Data collection and code generation for constants used to access the
* DOM.
*/
class AccessConsts {
/**
* Information collected about each element.
*/
private ElementTable fElementTable;
/*
* Sorted table of element class fields that have been created, with
* class as the key.
*/
private TreeMap fClassNameFields = new TreeMap();
/**
* Table of element class that are not legal Java identifiers.
*/
private TreeMap fInvalidElementClassNames = null;
/*
* Sorted table of element name fields that have been created, with
* element name as the key.
*/
private TreeMap fElementNameFields = new TreeMap();
/**
* Table of element names that are not legal Java identifiers.
*/
private TreeMap fInvalidElementNames = null;
/**
* Generate old-style, all upper-case class constants.
*/
private boolean fOldClassConstants;
/**
* Generate old-style, all upper-case name constants.
*/
private boolean fOldNameConstants;
/**
* Class being generated.
*/
private JavaClass fDocClass;
/**
* Constructor.
* @param metaData Document metadata.
* @param xmlcDoc Must contain parsed document.
* @param docClass Object containing the class being generated.
*/
public AccessConsts(MetaData metaData,
ElementTable elementTable) {
fElementTable = elementTable;
//FIXME: Would really rather get defaults..
HTMLSection htmlSection = metaData.getHTMLSection();
HTMLCompatibility htmlCompat = htmlSection.getCompatibility();
if (htmlCompat != null) {
fOldClassConstants = htmlCompat.getOldClassConstants();
fOldNameConstants = htmlCompat.getOldNameConstants();
}
}
/**
* Create a field for a single element class name.
*/
private void createElementClassNameField(String className) {
String fieldName = "CLASS_"
+ (fOldClassConstants ? className.toUpperCase() : className);
JavaField field
= new JavaField(fieldName,
"String",
JavaModifiers.PUBLIC_CONST,
"Class attribute constant for element class " + className,
JavaLang.createStringConst(className));
fDocClass.addField(field);
fClassNameFields.put(className, field);
}
/**
* Create class name fields for an element.
*/
private void processElementClassNames(ElementInfo elementInfo) {
String[] names = elementInfo.getElementClassNames();
if (names != null) {
for (int idx = 0; idx < names.length; idx++) {
if (!fClassNameFields.containsKey(names[idx])) {
if (!JavaLang.legalJavaIdentifier(names[idx])) {
if (fInvalidElementClassNames == null) {
fInvalidElementClassNames = new TreeMap();
}
fInvalidElementClassNames.put(names[idx], names[idx]);
} else {
createElementClassNameField(names[idx]);
}
}
}
}
}
/**
* Create a field for an element name.
*/
private void createElementNameField(String name) {
String fieldName = "NAME_"
+ (fOldNameConstants ? name.toUpperCase() : name);
JavaField field
= new JavaField(fieldName,
"String",
JavaModifiers.PUBLIC_CONST,
"Element name constant for " + name,
JavaLang.createStringConst(name));
fDocClass.addField(field);
fElementNameFields.put(name, field);
}
/**
* Create element name fieldsfor an element.
*/
private void processElementName(ElementInfo elementInfo) {
String name = elementInfo.getElementName();
if ((name != null) && (name.length() > 0)) {
if (!fElementNameFields.containsKey(name)) {
if (!JavaLang.legalJavaIdentifier(name)) {
if (fInvalidElementNames == null) {
fInvalidElementNames = new TreeMap();
}
fInvalidElementNames.put(name, name);
} else {
createElementNameField(name);
}
}
}
}
/**
* Generate the code for the access methods.
* @param docClass Object containing the class being generated.
*/
public void generateCode(JavaClass docClass) {
fDocClass = docClass;
Iterator elements = fElementTable.getElements();
while (elements.hasNext()) {
ElementInfo elementInfo = (ElementInfo)elements.next();
processElementClassNames(elementInfo);
processElementName(elementInfo);
}
}
/**
* Print the signatures of the access constants are created.
*/
public void printAccessConstants(PrintWriter out) {
Iterator classes = fClassNameFields.values().iterator();
while (classes.hasNext()) {
((JavaField)classes.next()).printDefinition(out);
out.println(';');
}
Iterator names = fElementNameFields.values().iterator();
while (names.hasNext()) {
((JavaField)names.next()).printDefinition(out);
out.println(';');
}
}
/**
* Print names that didn't have access constants generated due to
* being illegal Java identifiers. Sort so tests can be reproduced.
*/
public void printOmittedConstants(PrintWriter out) {
if (fInvalidElementClassNames != null) {
out.println("Element class name constants not created for these class names");
out.println("since they are not legal Java identifiers:");
Iterator badClasses = fInvalidElementClassNames.keySet().iterator();
while (badClasses.hasNext()) {
out.print("`");
out.print((String)badClasses.next());
out.println("'");
}
}
if (fInvalidElementNames != null) {
out.println("Element name constants not created for these names");
out.println("since they are not legal Java identifiers:");
Iterator badNames = fInvalidElementNames.keySet().iterator();
while (badNames.hasNext()) {
out.print("`");
out.print((String)badNames.next());
out.println("'");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy