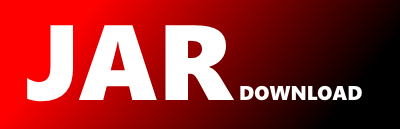
org.enhydra.xml.xmlc.compiler.Compiler Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: Compiler.java,v 1.3 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.compiler;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import org.enhydra.xml.dom.DOMInfo;
import org.enhydra.xml.io.DOMFormatter;
import org.enhydra.xml.io.ErrorReporter;
import org.enhydra.xml.io.InputSourceOps;
import org.enhydra.xml.io.OutputOptions;
import org.enhydra.xml.xmlc.XMLCException;
import org.enhydra.xml.xmlc.dom.XMLCDocument;
import org.enhydra.xml.xmlc.metadata.CompileOptions;
import org.enhydra.xml.xmlc.metadata.DocumentClass;
import org.enhydra.xml.xmlc.metadata.MetaData;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
//FIXME: InputSource is obtained both here and in Parse...
/**
* XMLC compiler class. This class implements the steps in compiling a
* document. This is a programatic interface and does not have a main or
* handle command line options.
*/
public class Compiler {
/*
* Document metadata.
*/
private MetaData fMetaData;
/*
* Compile options from metadata.
*/
private CompileOptions fCompileOptions;
/**
* Enable verbose output.
*/
private boolean fVerbose = false;
/**
* Input source being compiled.
*/
private InputSource fInputSource;
/*
* Document being compiled.
*/
private Document fDocument;
/*
* Error output.
*/
ErrorReporter fErrorReporter;
/*
* Trace and verbose output.
*/
private PrintWriter fTraceOut;
/*
* XML document management object.
*/
private XMLCDocument fXmlcDoc;
/**
* Constructor.
* @param errorReporter Object called to handle and output errors.
* @param traceOut Object to write trace and document information to.
*/
public Compiler(ErrorReporter errorReporter,
PrintWriter traceOut) {
fErrorReporter = errorReporter;
fTraceOut = traceOut;
}
/*
* Parse the page into the DOM and perform various checks and edits.
*/
private void parsePage() throws XMLCException, IOException {
if (fVerbose) {
fTraceOut.println(">>> parsing " + InputSourceOps.getName(fInputSource));
}
Parse parser = new Parse(fErrorReporter, fTraceOut);
fXmlcDoc = parser.parse(fMetaData);
fDocument = fXmlcDoc.getDocument();
if (fCompileOptions.getPrintDocumentInfo()) {
if (fVerbose) {
fTraceOut.println(">>> printing document info");
}
PrintInfo info = new PrintInfo(fDocument, fXmlcDoc);
info.printInfo(fTraceOut);
}
EditDOM domEditor = new EditDOM(fMetaData);
domEditor.edit(fXmlcDoc);
if (fCompileOptions.getPrintDOM()) {
if (fVerbose) {
fTraceOut.println(">>> dumping document hierarchy");
}
DOMInfo.printTree("DOM hierarchy", fDocument, fTraceOut);
}
}
/*
* Generate the Java source files.
*/
private void generateJavaSource() throws XMLCException, IOException {
if (fVerbose) {
fTraceOut.println(">>> generating code");
}
ClassGenerator codeGen =
new ClassGenerator(fMetaData, fXmlcDoc,
(fCompileOptions.getPrintAccessorInfo()
? fTraceOut : null));
codeGen.generateJavaSource(fVerbose ? fTraceOut : null);
}
/*
* Compile the generate source.
*/
private void compileJavaSource() throws XMLCException {
if (fVerbose) {
fTraceOut.println(">>> compiling code");
}
Javac javac = new Javac();
javac.compile(fMetaData, fErrorReporter,
(fVerbose ? fTraceOut : null));
}
/*
* Write the DOM to a file.
*/
private void writeDOM() throws IOException {
File docOut = new File(fCompileOptions.getDocumentOutput());
String dir = docOut.getParent();
if (dir != null) {
new File(dir).mkdirs();
}
OutputOptions options = DOMFormatter.getDefaultOutputOptions(fXmlcDoc.getDocument());
options.setEncoding(fXmlcDoc.getEncoding());
DOMFormatter formatter = new DOMFormatter(options);
formatter.write(fXmlcDoc.getDocument(), docOut);
}
/*
* Generate the Jave source and compile the page.
*/
private void compileDocument()
throws XMLCException, IOException {
DocumentClass documentClass = fMetaData.getDocumentClass();
boolean saveWarnings = fErrorReporter.getPrintWarnings();
fErrorReporter.setPrintWarnings(fCompileOptions.getWarnings());
try {
generateJavaSource();
if (fCompileOptions.getCompileSource()) {
compileJavaSource();
}
/* generate the meta data file for recompiling/deferred deferred parsing */
if (documentClass.getRecompilation() || documentClass.getDeferredParsing()) {
if (fVerbose) {
fTraceOut.println(">>> output metadata to " + fMetaData.getDocument().getMetadataOutputFile().getAbsolutePath());
}
fMetaData.getDocument().serialize();
}
} finally {
fErrorReporter.setPrintWarnings(saveWarnings);
if (!fCompileOptions.getKeepGeneratedSource()) {
if (documentClass.getJavaClassSource() != null) {
documentClass.getJavaClassSource().delete();
}
if (documentClass.getJavaInterfaceSource() != null) {
documentClass.getJavaInterfaceSource().delete();
}
}
}
}
/*
* Parse arguments and compile the page.
*/
public void compile(MetaData metaData)
throws XMLCException, IOException {
fMetaData = metaData;
fCompileOptions = fMetaData.getCompileOptions();
fVerbose = fCompileOptions.getVerbose();
String inputDocument = fMetaData.getInputDocument().getUrl();
if (inputDocument == null) {
throw new XMLCException("input document not specified");
}
fInputSource = new InputSource(inputDocument);
parsePage();
if (fCompileOptions.getCreateSource()) {
compileDocument();
}
// Write document after edits, if requested.
if (fCompileOptions.getDocumentOutput() != null) {
writeDOM();
}
if (fVerbose) {
fTraceOut.println(">>> completed");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy