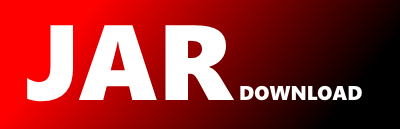
org.enhydra.xml.xmlc.compiler.Javac Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: Javac.java,v 1.4 2005/10/23 03:17:49 jkjome Exp $
*/
package org.enhydra.xml.xmlc.compiler;
import java.io.PrintWriter;
import org.enhydra.xml.io.ErrorReporter;
import org.enhydra.xml.xmlc.XMLCException;
import org.enhydra.xml.xmlc.codegen.JavaCompile;
import org.enhydra.xml.xmlc.metadata.DocumentClass;
import org.enhydra.xml.xmlc.metadata.GenerateType;
import org.enhydra.xml.xmlc.metadata.JavaCompilerSection;
import org.enhydra.xml.xmlc.metadata.MetaData;
/**
* Compile generated classes.
*/
public class Javac {
/** Should errors be passed back in an exception? */
private boolean fThrowErrorMsgs;
/**
* Enable collecting errors and throwing them in an exception.
*/
public void setThrowErrorMsgs(boolean enable) {
fThrowErrorMsgs = enable;
}
/**
* Run a javac process. Stdout/stderr are written to the standard
* descriptors.
*
* @param metaData Document metadata.
* @param errorReporter Write errors to this object.
* @param verboseOut Write verbose message to this file if not null.
*/
public void compile(MetaData metaData,
ErrorReporter errorReporter,
PrintWriter verboseOut)
throws XMLCException {
DocumentClass documentClass = metaData.getDocumentClass();
GenerateType generate = documentClass.getGenerate();
JavaCompilerSection compilerSection = metaData.getJavaCompilerSection();
String classOutputRoot = metaData.getCompileOptions().getClassOutputRoot();
JavaCompile compile = new JavaCompile(errorReporter,
compilerSection.getJavac());
if (fThrowErrorMsgs) {
compile.setOptions(JavaCompile.COLLECT_STDOUT
|JavaCompile.COLLECT_STDERR
|JavaCompile.DUMP_STDERR_ON_FAIL);
}
if (classOutputRoot != null) {
compile.setClassOutputRoot(classOutputRoot);
}
compile.addArgs(compilerSection.getJavacArgs());
compile.addArg("-encoding", "ISO-8859-1");
if (generate.generateClass()) {
compile.addSrc(documentClass.getJavaClassSource().getPath());
}
if (generate.generateInterface()) {
compile.addSrc(documentClass.getJavaInterfaceSource().getPath());
}
compile.compile(verboseOut);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy