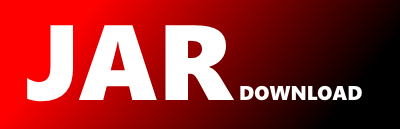
org.enhydra.xml.xmlc.compiler.PrintInfo Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: PrintInfo.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.compiler;
import java.io.PrintWriter;
import java.util.Vector;
import org.enhydra.xml.xmlc.dom.XMLCDocument;
import org.w3c.dom.Attr;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
/**
* Gather and print information about an document.
*/
class PrintInfo {
/**
* Table URLs.
*/
private Vector urls = new Vector();
/**
* Table of element ids.
*/
private Vector ids = new Vector();
/**
* Construct document info from document.
*/
public PrintInfo(Document doc, XMLCDocument xmlcDoc) {
getNodeInfo(doc, xmlcDoc);
}
/**
* Append URLs from an element.
*/
private void getElementURLs(Element element, XMLCDocument xmlcDoc) {
NamedNodeMap attrs = element.getAttributes();
if (attrs != null) {
for (int idx = 0; idx < attrs.getLength(); idx++) {
Attr attr = (Attr)attrs.item(idx);
if (xmlcDoc.isURLAttribute(element, attr.getNodeName())) {
urls.addElement(attr.getValue());
}
}
}
}
/**
* Recursively scan nodes nodes and accumulate information.
*/
private void getNodeInfo(Node node, XMLCDocument xmlcDoc) {
if (node instanceof Element) {
Element elem = (Element)node;
getElementURLs(elem, xmlcDoc);
String id = xmlcDoc.getElementId(elem);
if ((id != null) && (id.length() > 0)) {
ids.addElement(id + " => "
+ xmlcDoc.nodeClassToInterface(node));
}
}
for (Node child = node.getFirstChild(); child != null;
child = child.getNextSibling()) {
getNodeInfo(child, xmlcDoc);
}
}
/**
* Print document information.
*/
protected void printInfo(PrintWriter out) {
int idx;
out.println("Element IDs:");
for (idx = 0; idx < ids.size(); idx++) {
out.println(" " + ids.elementAt(idx));
}
out.println("Document URLs:");
for (idx = 0; idx < urls.size(); idx++) {
out.println(" " + urls.elementAt(idx));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy