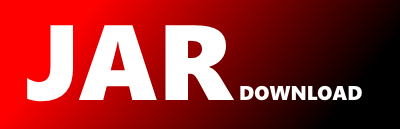
org.enhydra.xml.xmlc.deferredparsing.DynamicMLCreatorBCELImpl Maven / Gradle / Ivy
The newest version!
package org.enhydra.xml.xmlc.deferredparsing;
import org.apache.bcel.Constants;
import org.apache.bcel.classfile.JavaClass;
import org.apache.bcel.generic.BranchInstruction;
import org.apache.bcel.generic.ClassGen;
import org.apache.bcel.generic.ConstantPoolGen;
import org.apache.bcel.generic.FieldGen;
import org.apache.bcel.generic.INSTANCEOF;
import org.apache.bcel.generic.InstructionConstants;
import org.apache.bcel.generic.InstructionFactory;
import org.apache.bcel.generic.InstructionHandle;
import org.apache.bcel.generic.InstructionList;
import org.apache.bcel.generic.MethodGen;
import org.apache.bcel.generic.ObjectType;
import org.apache.bcel.generic.PUSH;
import org.apache.bcel.generic.Type;
public class DynamicMLCreatorBCELImpl implements Constants, DynamicMLCreator {
private String className;
private String className$;
private String sourcePath;
private InstructionFactory _factory;
private ConstantPoolGen _cp;
private ClassGen _cg;
private String superClass;
private String mimeType;
private String encoding;
public byte[] getByteArrayForClass() {
JavaClass javaClass = create();
return javaClass.getBytes();
}
public DynamicMLCreatorBCELImpl(String name, String path) {
className = name;
className$ = "class$" + className.replace('.', '$');
sourcePath = path;
String type = (path.endsWith(".html") || path.endsWith(".htm") || path.endsWith(".xhtml") || path
.endsWith(".chtml")) ? "html" : "xml";
if (type.equals("html")) {
// xhtml is actually xml, but generated xhtml classes look like
// this rather than the xml below, so including xhtml for this
// definition
superClass = "org.enhydra.xml.xmlc.html.HTMLObjectImpl";
_cg = new ClassGen(className, superClass, "", ACC_PUBLIC | ACC_SUPER, new String[] {
"org.enhydra.xml.xmlc.XMLObject", "org.enhydra.xml.xmlc.html.HTMLObject" });
mimeType = "text/html";
encoding = "ISO-8859-1";
} else {
superClass = "org.enhydra.xml.xmlc.XMLObjectImpl";
_cg = new ClassGen(className, superClass, "", ACC_PUBLIC | ACC_SUPER,
new String[] { "org.enhydra.xml.xmlc.XMLObject" });
mimeType = "text/xml";
encoding = "UTF-8";
}
_cp = _cg.getConstantPool();
_factory = new InstructionFactory(_cg, _cp);
}
public JavaClass create() {
createFields();
createMethod_0();
createMethod_1();
createMethod_2();
createMethod_3();
createMethod_4();
createMethod_5();
createMethod_6();
createMethod_7();
createMethod_8();
createMethod_9();
createMethod_10();
createMethod_11();
return _cg.getJavaClass();
}
private void createFields() {
FieldGen field;
field = new FieldGen(ACC_PUBLIC | ACC_STATIC | ACC_FINAL, new ObjectType("java.lang.Class"),
"XMLC_GENERATED_CLASS", _cp);
_cg.addField(field.getField());
field = new FieldGen(ACC_PUBLIC | ACC_STATIC | ACC_FINAL, Type.STRING, "XMLC_SOURCE_FILE", _cp);
field.setInitValue(sourcePath);
_cg.addField(field.getField());
field = new FieldGen(ACC_PRIVATE | ACC_STATIC | ACC_FINAL, new ObjectType(
"org.enhydra.xml.xmlc.dom.XMLCDomFactory"), "fDOMFactory", _cp);
_cg.addField(field.getField());
field = new FieldGen(ACC_PRIVATE | ACC_FINAL, new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), "fDocumentLoader", _cp);
_cg.addField(field.getField());
field = new FieldGen(ACC_STATIC, new ObjectType("java.lang.Class"), className$, _cp);
_cg.addField(field.getField());
field = new FieldGen(ACC_STATIC, new ObjectType("java.lang.Class"),
"class$org$enhydra$xml$xmlc$dom$xerces$XercesHTMLDomFactory", _cp);
_cg.addField(field.getField());
}
private void createMethod_0() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PUBLIC, Type.VOID, Type.NO_ARGS, new String[] {}, "", className, il,
_cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke("org.enhydra.xml.xmlc.deferredparsing.StandardDocumentLoader", "getInstance",
new ObjectType("org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.NO_ARGS,
Constants.INVOKESTATIC));
il.append(_factory.createInvoke(className, "", Type.VOID, new Type[] { new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader") }, Constants.INVOKESPECIAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(className, "buildDocument", Type.VOID, Type.NO_ARGS, Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createReturn(Type.VOID));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_1() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PUBLIC, Type.VOID, new Type[] { Type.BOOLEAN },
new String[] { "buildDOM" }, "", className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke("org.enhydra.xml.xmlc.deferredparsing.StandardDocumentLoader", "getInstance",
new ObjectType("org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.NO_ARGS,
Constants.INVOKESTATIC));
il.append(InstructionFactory.createLoad(Type.INT, 1));
il.append(_factory.createInvoke(className, "", Type.VOID, new Type[] {
new ObjectType("org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.BOOLEAN },
Constants.INVOKESPECIAL));
il.append(InstructionFactory.createReturn(Type.VOID));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_2() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PUBLIC, Type.VOID, new Type[] { new ObjectType(className) },
new String[] { "src" }, "", className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(superClass, "", Type.VOID, Type.NO_ARGS, Constants.INVOKESPECIAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createInvoke(className, "getDocumentLoader", new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.NO_ARGS, Constants.INVOKEVIRTUAL));
il.append(_factory.createFieldAccess(className, "fDocumentLoader", new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Constants.PUTFIELD));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createInvoke(className, "getDocument", new ObjectType("org.w3c.dom.Document"), Type.NO_ARGS,
Constants.INVOKEVIRTUAL));
il.append(new PUSH(_cp, 1));
il.append(_factory.createInvoke("org.w3c.dom.Document", "cloneNode", new ObjectType("org.w3c.dom.Node"),
new Type[] { Type.BOOLEAN }, Constants.INVOKEINTERFACE));
il.append(_factory.createCheckCast(new ObjectType("org.w3c.dom.Document")));
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createInvoke(className, "getMIMEType", Type.STRING, Type.NO_ARGS, Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createInvoke(className, "getEncoding", Type.STRING, Type.NO_ARGS, Constants.INVOKEVIRTUAL));
il.append(_factory.createInvoke(className, "setDocument", Type.VOID, new Type[] {
new ObjectType("org.w3c.dom.Document"), Type.STRING, Type.STRING }, Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(className, "syncAccessMethods", Type.VOID, Type.NO_ARGS,
Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createReturn(Type.VOID));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_3() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PUBLIC, Type.VOID, new Type[] {
new ObjectType("org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.BOOLEAN }, new String[] {
"loader", "buildDOM" }, "", className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(superClass, "", Type.VOID, Type.NO_ARGS, Constants.INVOKESPECIAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createFieldAccess(className, "fDocumentLoader", new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Constants.PUTFIELD));
il.append(InstructionFactory.createLoad(Type.INT, 2));
BranchInstruction ifeq_10 = InstructionFactory.createBranchInstruction(Constants.IFEQ, null);
il.append(ifeq_10);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(className, "buildDocument", Type.VOID, Type.NO_ARGS, Constants.INVOKEVIRTUAL));
InstructionHandle ih_17 = il.append(InstructionFactory.createReturn(Type.VOID));
ifeq_10.setTarget(ih_17);
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_4() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PUBLIC, Type.VOID, new Type[] { new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader") }, new String[] { "loader" }, "",
className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(new PUSH(_cp, 1));
il.append(_factory.createInvoke(className, "", Type.VOID, new Type[] {
new ObjectType("org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.BOOLEAN },
Constants.INVOKESPECIAL));
il.append(InstructionFactory.createReturn(Type.VOID));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_5() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PUBLIC, Type.VOID, Type.NO_ARGS, new String[] {}, "buildDocument",
className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(className, "getDocumentLoader", new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.NO_ARGS, Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke("java.lang.Object", "getClass", new ObjectType("java.lang.Class"),
Type.NO_ARGS, Constants.INVOKEVIRTUAL));
il.append(_factory.createInvoke("org.enhydra.xml.xmlc.deferredparsing.DocumentLoader", "getDocument",
new ObjectType("org.w3c.dom.Document"), new Type[] { new ObjectType("java.lang.Class") },
Constants.INVOKEINTERFACE));
il.append(new PUSH(_cp, mimeType));
il.append(new PUSH(_cp, encoding));
il.append(_factory.createInvoke(className, "setDocument", Type.VOID, new Type[] {
new ObjectType("org.w3c.dom.Document"), Type.STRING, Type.STRING }, Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(className, "syncAccessMethods", Type.VOID, Type.NO_ARGS,
Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createReturn(Type.VOID));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_6() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PUBLIC, new ObjectType("org.w3c.dom.Node"), new Type[] { Type.BOOLEAN },
new String[] { "deep" }, "cloneNode", className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(InstructionFactory.createLoad(Type.INT, 1));
il.append(_factory.createInvoke(className, "cloneDeepCheck", Type.VOID, new Type[] { Type.BOOLEAN },
Constants.INVOKEVIRTUAL));
il.append(_factory.createNew(className));
il.append(InstructionConstants.DUP);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createInvoke(className, "", Type.VOID, new Type[] { new ObjectType(className) },
Constants.INVOKESPECIAL));
il.append(InstructionFactory.createReturn(Type.OBJECT));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_7() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PROTECTED | ACC_FINAL, new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Type.NO_ARGS, new String[] {},
"getDocumentLoader", className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(_factory.createFieldAccess(className, "fDocumentLoader", new ObjectType(
"org.enhydra.xml.xmlc.deferredparsing.DocumentLoader"), Constants.GETFIELD));
il.append(InstructionFactory.createReturn(Type.OBJECT));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_8() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PROTECTED | ACC_FINAL, new ObjectType(
"org.enhydra.xml.xmlc.dom.XMLCDomFactory"), Type.NO_ARGS, new String[] {}, "getDomFactory", className,
il, _cp);
il.append(_factory.createFieldAccess(className, "fDOMFactory", new ObjectType(
"org.enhydra.xml.xmlc.dom.XMLCDomFactory"), Constants.GETSTATIC));
il.append(InstructionFactory.createReturn(Type.OBJECT));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_9() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_PROTECTED, Type.VOID, new Type[] { new ObjectType("org.w3c.dom.Node") },
new String[] { "node" }, "syncWithDocument", className, il, _cp);
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(new INSTANCEOF(_cp.addClass(new ObjectType("org.w3c.dom.Element"))));
BranchInstruction ifeq_4 = InstructionFactory.createBranchInstruction(Constants.IFEQ, null);
il.append(ifeq_4);
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createCheckCast(new ObjectType("org.w3c.dom.Element")));
il.append(new PUSH(_cp, "id"));
il.append(_factory.createInvoke("org.w3c.dom.Element", "getAttribute", Type.STRING, new Type[] { Type.STRING },
Constants.INVOKEINTERFACE));
il.append(InstructionFactory.createStore(Type.OBJECT, 2));
il.append(InstructionFactory.createLoad(Type.OBJECT, 2));
il.append(_factory.createInvoke("java.lang.String", "length", Type.INT, Type.NO_ARGS, Constants.INVOKEVIRTUAL));
BranchInstruction ifne_23 = InstructionFactory.createBranchInstruction(Constants.IFNE, null);
il.append(ifne_23);
InstructionHandle ih_26 = il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createInvoke("org.w3c.dom.Node", "getFirstChild", new ObjectType("org.w3c.dom.Node"),
Type.NO_ARGS, Constants.INVOKEINTERFACE));
il.append(InstructionFactory.createStore(Type.OBJECT, 2));
InstructionHandle ih_33 = il.append(InstructionFactory.createLoad(Type.OBJECT, 2));
BranchInstruction ifnull_34 = InstructionFactory.createBranchInstruction(Constants.IFNULL, null);
il.append(ifnull_34);
il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
il.append(InstructionFactory.createLoad(Type.OBJECT, 2));
il.append(_factory.createInvoke(className, "syncWithDocument", Type.VOID, new Type[] { new ObjectType(
"org.w3c.dom.Node") }, Constants.INVOKEVIRTUAL));
il.append(InstructionFactory.createLoad(Type.OBJECT, 2));
il.append(_factory.createInvoke("org.w3c.dom.Node", "getNextSibling", new ObjectType("org.w3c.dom.Node"),
Type.NO_ARGS, Constants.INVOKEINTERFACE));
il.append(InstructionFactory.createStore(Type.OBJECT, 2));
BranchInstruction goto_49 = InstructionFactory.createBranchInstruction(Constants.GOTO, ih_33);
il.append(goto_49);
InstructionHandle ih_52 = il.append(InstructionFactory.createReturn(Type.VOID));
ifeq_4.setTarget(ih_26);
ifne_23.setTarget(ih_26);
ifnull_34.setTarget(ih_52);
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_10() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_STATIC, new ObjectType("java.lang.Class"), new Type[] { Type.STRING },
new String[] { "x0" }, "class$", className, il, _cp);
InstructionHandle ih_0 = il.append(InstructionFactory.createLoad(Type.OBJECT, 0));
InstructionHandle ih_1 = il.append(_factory.createInvoke("java.lang.Class", "forName", new ObjectType(
"java.lang.Class"), new Type[] { Type.STRING }, Constants.INVOKESTATIC));
il.append(InstructionFactory.createReturn(Type.OBJECT));
InstructionHandle ih_5 = il.append(InstructionFactory.createStore(Type.OBJECT, 1));
il.append(_factory.createNew("java.lang.NoClassDefFoundError"));
il.append(InstructionConstants.DUP);
il.append(InstructionFactory.createLoad(Type.OBJECT, 1));
il.append(_factory.createInvoke("java.lang.ClassNotFoundException", "getMessage", Type.STRING, Type.NO_ARGS,
Constants.INVOKEVIRTUAL));
il.append(_factory.createInvoke("java.lang.NoClassDefFoundError", "", Type.VOID,
new Type[] { Type.STRING }, Constants.INVOKESPECIAL));
il.append(InstructionConstants.ATHROW);
method.addExceptionHandler(ih_0, ih_1, ih_5, new ObjectType("java.lang.ClassNotFoundException"));
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
private void createMethod_11() {
InstructionList il = new InstructionList();
MethodGen method = new MethodGen(ACC_STATIC, Type.VOID, Type.NO_ARGS, new String[] {}, "", className,
il, _cp);
il.append(_factory.createFieldAccess(className, className$, new ObjectType("java.lang.Class"),
Constants.GETSTATIC));
BranchInstruction ifnonnull_3 = InstructionFactory.createBranchInstruction(Constants.IFNONNULL, null);
il.append(ifnonnull_3);
il.append(new PUSH(_cp, className));
il.append(_factory.createInvoke(className, "class$", new ObjectType("java.lang.Class"),
new Type[] { Type.STRING }, Constants.INVOKESTATIC));
il.append(InstructionConstants.DUP);
il.append(_factory.createFieldAccess(className, className$, new ObjectType("java.lang.Class"),
Constants.PUTSTATIC));
BranchInstruction goto_15 = InstructionFactory.createBranchInstruction(Constants.GOTO, null);
il.append(goto_15);
InstructionHandle ih_18 = il.append(_factory.createFieldAccess(className, className$, new ObjectType(
"java.lang.Class"), Constants.GETSTATIC));
InstructionHandle ih_21 = il.append(_factory.createFieldAccess(className, "XMLC_GENERATED_CLASS",
new ObjectType("java.lang.Class"), Constants.PUTSTATIC));
il.append(_factory.createFieldAccess(className, "class$org$enhydra$xml$xmlc$dom$xerces$XercesHTMLDomFactory",
new ObjectType("java.lang.Class"), Constants.GETSTATIC));
BranchInstruction ifnonnull_27 = InstructionFactory.createBranchInstruction(Constants.IFNONNULL, null);
il.append(ifnonnull_27);
il.append(new PUSH(_cp, "org.enhydra.xml.xmlc.dom.xerces.XercesHTMLDomFactory"));
il.append(_factory.createInvoke(className, "class$", new ObjectType("java.lang.Class"),
new Type[] { Type.STRING }, Constants.INVOKESTATIC));
il.append(InstructionConstants.DUP);
il.append(_factory.createFieldAccess(className, "class$org$enhydra$xml$xmlc$dom$xerces$XercesHTMLDomFactory",
new ObjectType("java.lang.Class"), Constants.PUTSTATIC));
BranchInstruction goto_39 = InstructionFactory.createBranchInstruction(Constants.GOTO, null);
il.append(goto_39);
InstructionHandle ih_42 = il.append(_factory.createFieldAccess(className,
"class$org$enhydra$xml$xmlc$dom$xerces$XercesHTMLDomFactory", new ObjectType("java.lang.Class"),
Constants.GETSTATIC));
InstructionHandle ih_45 = il.append(_factory.createInvoke("org.enhydra.xml.xmlc.dom.XMLCDomFactoryCache",
"getFactory", new ObjectType("org.enhydra.xml.xmlc.dom.XMLCDomFactory"), new Type[] { new ObjectType(
"java.lang.Class") }, Constants.INVOKESTATIC));
il.append(_factory.createFieldAccess(className, "fDOMFactory", new ObjectType(
"org.enhydra.xml.xmlc.dom.XMLCDomFactory"), Constants.PUTSTATIC));
il.append(InstructionFactory.createReturn(Type.VOID));
ifnonnull_3.setTarget(ih_18);
goto_15.setTarget(ih_21);
ifnonnull_27.setTarget(ih_42);
goto_39.setTarget(ih_45);
method.setMaxStack();
method.setMaxLocals();
_cg.addMethod(method.getMethod());
il.dispose();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy