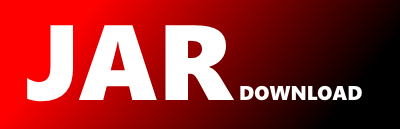
org.enhydra.xml.xmlc.dom.HTMLDomFactoryMethods Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: HTMLDomFactoryMethods.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.dom;
import java.util.HashSet;
import java.util.StringTokenizer;
import org.w3c.dom.Element;
/**
* Support for implementing DOM factories for HTML. Since a HTML
* DOM factory normally extends the XML DOM factory, there isn't
* a base class for HTML support.
*/
public final class HTMLDomFactoryMethods {
/**
* HTML URL attributes.
*/
private static String[] URL_ATTRIBUTES = {
"action", "archive", "background", "cite", "classid", "codebase",
"data", "href", "longdesc", "onblur", "onchange", "onclick",
"ondblclick", "onfocus", "onkeydown", "onkeypress", "onkeyup",
"onload", "onmousedown", "onmousemove", "onmouseout", "onmouseover",
"onmouseup","onreset", "onselect", "onsubmit", "onunload", "profile",
"src", "usemap"
};
/**
* HashSet built from URL_ATTRIBUTES.
*/
private static final HashSet urlAttributes;
/**
* Class initializer.
*/
static {
urlAttributes = new HashSet(URL_ATTRIBUTES.length);
for (int idx = 0; idx < URL_ATTRIBUTES.length; idx++) {
urlAttributes.add(URL_ATTRIBUTES[idx]);
}
}
/**
* @see XMLCDomFactory#getBaseClassName
*/
public static final String getBaseClassName() {
return "org.enhydra.xml.xmlc.html.HTMLObjectImpl";
}
/**
* @see XMLCDomFactory#getInterfaceNames
*/
public static final String[] getInterfaceNames() {
return new String[] {"org.enhydra.xml.xmlc.html.HTMLObject"};
}
/**
* @see XMLCDomFactory#getElementClassNames
*/
public static final String[] getElementClassNames(Element element) {
//FIXME: This code would be real useful in XMLCUtil or HTMLObject.
String classNames = element.getAttribute("class");
if ((classNames == null) || (classNames.length() ==0)) {
return null;
} else {
// Parse the class name.
StringTokenizer tokens = new StringTokenizer(classNames);
String[] names = new String[tokens.countTokens()];
for (int idx = 0; idx < names.length; idx++) {
names[idx] = tokens.nextToken();
}
return names;
}
}
/**
* @see XMLCDomFactory#isURLAttribute
*/
public static final boolean isURLAttribute(Element element,
String attrName) {
return urlAttributes.contains(attrName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy