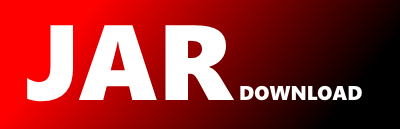
org.enhydra.xml.xmlc.dom.XMLCDomFactory Maven / Gradle / Ivy
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: XMLCDomFactory.java,v 1.3 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.dom;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
/**
* Interface for a factory class that can create DocumentType and Document
* objects. It also provides document-type specific functions. This is
* used in implementing subclassed DOMs.
*
* Classes implementing this must:
*
* - Provide a constructor that takes no arguments.
*
- Be thread-safe (reentrant is preferred).
*
* When a DTD-specific DOM is created, an class implementing this interface
* must be supplied to XMLC. This would normally be created by extending the
* XMLCDomDefaultFactory class.
*
* This class is designed to be build using a DOMImplementation object.
* However, since the DOM doesn't specify how DOMImplementation objects
* are obtained, we hide that detail. The methods are somewhat different
* for historic reasons.
*
* @see org.w3c.dom.DOMImplementation
* @see org.w3c.dom.html.HTMLDOMImplementation
*/
public interface XMLCDomFactory {
//FIXME: Way of returning URL attribute names would allow for
// more efficient code.
/**
* Creates an empty DocumentType
node.
*
* @param qualifiedName The document type name (same as the root element).
* @param publicID The document type public identifier.
* @param systemID The document type system identifier.
* @param internalSubset The internal subset as a string.
* @return A new DocumentType
node.
*/
public DocumentType createDocumentType(String qualifiedName,
String publicID,
String systemID,
String internalSubset);
/**
* Creates an XML Document
object of the specified type. The
* document element should be created. A HTML document should
* only have the document element, which differs from the
* DOMImplementation
specification, however it makes
* code generation easier and its not expected that there will be
* many custom HTML DOM factories.
*
* @param namespaceURI The namespace URI of the document element to
* create, or null.
* @param qualifiedName The document type name (same as the root element).
* Maybe null for HTML documents or documents without DTDs.
* @param doctype The type of document to be created or null
.
* When doctype
is not null
, its
* Node.ownerDocument
attribute is set to the document being
* created. Maybe null for for documents without DTDs or HTML documents.
* @return A new Document
object.
* @see org.w3c.dom.DOMImplementation
* @see org.w3c.dom.html.HTMLDOMImplementation
*/
public Document createDocument(String namespaceURI,
String qualifiedName,
DocumentType doctype);
/**
* Get the MIME type to associated with the document, or null
* if none should be associated.
*/
public String getMIMEType();
/**
* Get the base class name for generated classes. It must extend
* XMLObjectImpl. This class maybe overridden for individual documents
* that are compiled.
*/
public String getBaseClassName();
/**
* Get the interface names that will automatically be added to all
* generated classes and interfaces. This class maybe overridden for
* individual documents that are compiled. It XMLObject is not
* part of the list, it will be added automatically.
*
* @return An array of fully-qualified interface names, or null
* if none, other than XMLObject, are to be added.
*/
public String[] getInterfaceNames();
/**
* Convert an implementation-specific DOM node class name to the
* external interface or class name that should be used to
* reference it. This could be a org.w3c.dom
interface or
* other interface or class.
*
* @see org.enhydra.xml.xmlc#XMLObject
*/
public String nodeClassToInterface(Node node);
/**
* Extract the class names for an element. This is a class for grouping
* elements, not the Java class. In HTML, the class is specified with the
* class
attribute and with a value of a white-space
* separated list of class names. Its not specified for XML, however this
* method can be implemented in DTD-specifc XMLDomFactories to enable this
* functionality.
*
* @return An array of class names or null if the node has no classes.
* XML returns null.
*/
public String[] getElementClassNames(Element element);
/**
* Determine if an an attribute of an element may contain a URL and should
* be subject to URL editing at compile time(or rewriting at run
* time. This method is required, as there is not way to define this in a
* XML DTD. With HTML, the attributes returned should have values of type
* %URL, %URI or %Script.
*
* @param element The element object the attribute is associated with.
* @param attrName The name of the attribute.
* @return True if the attribute may contain a URL (although it could
* contain JavaScript, etc. False if it can't and shouldn't be edited.
*/
public boolean isURLAttribute(Element element,
String attrName);
/**
* Create an AccessorGenerator object for this DOM.
* Normally, this method is inherited from the DOM that the base
* DOM implementation a.
*/
public AccessorGenerator createAccessorGenerator(Document document);
/**
* Create an DocBuilderGenerator object for this DOM.
* Normally, this method is inherited from the DOM that the base
* DOM implementation a.
*/
public DocBuilderGenerator createDocBuilderGenerator(Document document);
}