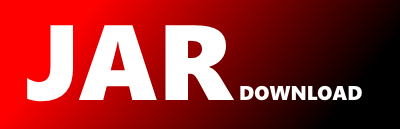
org.enhydra.xml.xmlc.dom.XMLCDomFactoryCache Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: XMLCDomFactoryCache.java,v 1.3 2005/11/02 06:30:31 jkjome Exp $
*/
package org.enhydra.xml.xmlc.dom;
import java.util.HashMap;
import org.enhydra.xml.xmlc.XMLCError;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
/**
* Class that provides a cache of XMLCDomFactory objects. Since these classes
* are simple, they are normally reentrant. This cache provides a mechanism
* to maintain only one instance of each class. Currently, instances are
* never freed from this cache. Also creates factories for use at
* compile-time.
*/
public class XMLCDomFactoryCache {
/**
* Class name of default XML DOM factory.
*/
private static final String DEFAULT_XML_DOM_FACTORY_CLASS
= "org.enhydra.xml.xmlc.dom.lazydom.LazyDomFactory";
/**
* Class name of default HTML DOM factory.
*/
private static final String DEFAULT_HTML_DOM_FACTORY_CLASS
= "org.enhydra.xml.xmlc.dom.lazydom.LazyHTMLDomFactory";
/**
* Cache of factories, keyed by class object.
*/
private static HashMap cache = new HashMap();
/**
* Disallow instanciation.
*/
private XMLCDomFactoryCache() {
}
/**
* Generate an error message when we can't create an instance of an
* XMLCDomFactory class.
*/
private static void handleCreateError(Class factoryClass,
Exception except) {
throw new XMLCError("Can't create an instance of \""
+ factoryClass.getName() + "\": "
+ except.getClass().getName(),
except);
}
/**
* Create an instance of an XMLCDomFactory. Don't synchronize, as its ok
* to create more than one.
*/
private static XMLCDomFactory createFactory(Class factoryClass) {
try {
return (XMLCDomFactory)factoryClass.newInstance();
} catch (IllegalAccessException except) {
handleCreateError(factoryClass, except);
} catch (InstantiationException except) {
handleCreateError(factoryClass, except);
} catch (ClassCastException except) {
handleCreateError(factoryClass, except);
}
return null; // Never makes it here.
}
/**
* Create an instance of an XMLCDomFactory, however don't add it
* to the cache. This is used at compile time to get a factory.
*
* @param factoryClassName Name of factory to create, if null, the
* default is user.
* @param isHtmlDocument Use only to determine the default.
*/
public static XMLCDomFactory createFactory(String factoryClassName,
boolean isHtmlDocument) {
if (factoryClassName == null) {
if (isHtmlDocument) {
factoryClassName = DEFAULT_HTML_DOM_FACTORY_CLASS;
} else {
factoryClassName = DEFAULT_XML_DOM_FACTORY_CLASS;
}
}
try {
return createFactory(Class.forName(factoryClassName));
} catch (Exception except) {
throw new XMLCError("Can't load class \""
+ factoryClassName + "\": "
+ except.getClass().getName(),
except);
}
}
/**
* Get an instance of an XMLCDomFactory given it's class.
*/
public static XMLCDomFactory getFactory(Class factoryClass) {
XMLCDomFactory factory = (XMLCDomFactory)cache.get(factoryClass);
if (factory == null) {
factory = createFactory(factoryClass);
cache.put(factoryClass, factory);
}
//System.out.println("factory is: " + factory);
return factory;
}
/**
* Check for an outdate DOM factory method,
*/
private static void checkForOutdateMethod(Class factoryClass,
String methodName,
Class[] methodArgTypes,
String methodDesc) {
try {
factoryClass.getMethod(methodName, methodArgTypes);
throw new XMLCError("XMLCDomFactory implementation "
+ factoryClass.getName()
+ " contains outdated method "
+ methodDesc
+ "; please update your code");
} catch (NoSuchMethodException except) {
// Good, don't have it...
}
}
/**
* Check a DOM factory for outdated methods. Several methods were
* changed in the XMLCDomFactory. Since few users implement these
* classes, its was decided to just obsolute the methods. However,
* since most XMLCDomFactories extend the base one, one just got
* weird behavior (generic documents instead of specific ones).
* To work around this, this method checks for outdated factory
* methods and generates errors if found.
*/
public static void checkForOutdatedClass(XMLCDomFactory domFactory) {
//FIXME: Added in XMLC 2.0, delete at some future date
Class factoryClass = domFactory.getClass();
// createDocument(String qualifiedName, DocumentType doctype);
checkForOutdateMethod(factoryClass, "createDocument",
new Class[] {String.class, DocumentType.class},
"createDocument(String,DocumentType)");
// getElementURLAttrs(Element element);
checkForOutdateMethod(factoryClass, "getElementURLAttrs",
new Class[] {Element.class},
"getElementURLAttrs(Element)");
// getBaseInterfaceName()
checkForOutdateMethod(factoryClass, "getBaseInterfaceName",
null, "getBaseInterfaceName()");
// needsCreateTextSetMethod(Element)
checkForOutdateMethod(factoryClass, "needsCreateTextSetMethod",
new Class[] {Element.class},
"needsCreateTextSetMethod(Element)");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy