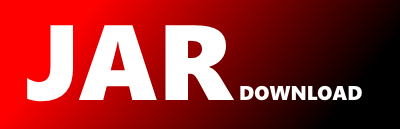
org.enhydra.xml.xmlc.dom.generic.ContainedNodeEnum Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: ContainedNodeEnum.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.dom.generic;
import java.util.Enumeration;
import java.util.NoSuchElementException;
import org.w3c.dom.DocumentType;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
/**
* Enumeration over the nodes contained by another node. This provides a way
* of find traversing standard children, attributes and document type entity
* and notation nodes in the same way.
*/
public class ContainedNodeEnum implements Enumeration {
/**
* Types of contained nodes to enumerate. This is both a bit set
* and values that define the order contained nodes are traversed.
*/
public static final int NONE = 0x0;
public static final int CHILDREN = 0x1;
public static final int ATTRIBUTES = 0x2;
public static final int ENTITIES = 0x4;
public static final int NOTATIONS = 0x8;
public static final int ALL = 0xF;
/** Node who's children are being enumerated */
private Node fParent;
/** Is this a DocumentType node */
private boolean fIsDocType;
/** Filter on types of nodes to process. */
private int fFilterSet;
/** Flag indicating the type of next contained node. */
private int fNextNodeType = NONE;
/** Next contained node */
private Node fNextNode;
/** Node map and index for other than children */
private NamedNodeMap fNextNodeMap;
private int fNextNodeIdx;
/** Constructor with filter for node types */
public ContainedNodeEnum(Node parent,
int filterSet) {
fParent = parent;
fIsDocType = (fParent instanceof DocumentType);
fFilterSet = filterSet;
// Find the first contained node
advance();
}
/** Constructor for enumerating all nodes types */
public ContainedNodeEnum(Node parent) {
this(parent, ALL);
}
/** Does the filter accept the specified type */
private boolean accepts(int type) {
return ((type & fFilterSet) != 0);
}
/**
* Advance to the next contained node. Also handles initialization.
*/
private void advance() {
if ((fNextNodeType <= CHILDREN) && accepts(CHILDREN)) {
if (fNextNodeType != CHILDREN) {
fNextNode = fParent.getFirstChild();
} else {
fNextNode = fNextNode.getNextSibling();
}
if (fNextNode != null) {
fNextNodeType = CHILDREN;
return; // done
}
}
if ((fNextNodeType <= ATTRIBUTES) && accepts(ATTRIBUTES)) {
if (fNextNodeType != ATTRIBUTES) {
fNextNodeMap = fParent.getAttributes();
fNextNodeIdx = 0;
} else {
fNextNodeIdx++;
}
if ((fNextNodeMap != null)
&& (fNextNodeIdx < fNextNodeMap.getLength())) {
fNextNodeType = ATTRIBUTES;
fNextNode = fNextNodeMap.item(fNextNodeIdx);
return; // done
}
}
if (fIsDocType && (fNextNodeType <= ENTITIES) && accepts(ENTITIES)) {
if (fNextNodeType != ENTITIES) {
fNextNodeMap = ((DocumentType)fParent).getEntities();
fNextNodeIdx = 0;
} else {
fNextNodeIdx++;
}
if ((fNextNodeMap != null)
&& (fNextNodeIdx < fNextNodeMap.getLength())) {
fNextNodeType = ENTITIES;
fNextNode = fNextNodeMap.item(fNextNodeIdx);
return; // done
}
}
if (fIsDocType && (fNextNodeType <= NOTATIONS) && accepts(NOTATIONS)) {
if (fNextNodeType != NOTATIONS) {
fNextNodeMap = ((DocumentType)fParent).getNotations();
fNextNodeIdx = 0;
} else {
fNextNodeIdx++;
}
if ((fNextNodeMap != null)
&& (fNextNodeIdx < fNextNodeMap.getLength())) {
fNextNodeType = NOTATIONS;
fNextNode = fNextNodeMap.item(fNextNodeIdx);
return; // done
}
}
// that' all folks...
fNextNodeType = NONE;
}
/** Tests if this enumeration contains more elements. */
public boolean hasMoreElements() {
return (fNextNodeType != NONE);
}
/** Returns the next node of this enumeration, or null if no more. */
public Node nextNode() throws NoSuchElementException {
if (fNextNodeType == NONE) {
return null;
} else {
Node node = fNextNode;
advance();
return node;
}
}
/** Returns the next element of this enumeration */
public Object nextElement() throws NoSuchElementException {
if (fNextNodeType == NONE) {
throw new NoSuchElementException("no more contained nodes");
}
return nextNode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy