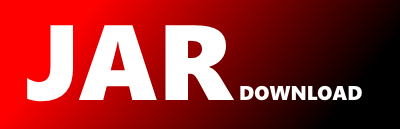
org.enhydra.xml.xmlc.metadata.CompileOptions Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: CompileOptions.java,v 1.3 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.metadata;
import java.io.File;
import org.enhydra.xml.xmlc.XMLCException;
import org.enhydra.xml.xmlc.codegen.JavaLang;
import org.w3c.dom.Document;
/**
* Specifies options for the document compiler.
*/
public class CompileOptions extends MetaDataElement {
/**
* Element name.
*/
public static final String TAG_NAME = "compileOptions";
/**
* Attribute names.
*/
private static final String VERBOSE_ATTR = "verbose";
private static final String PRINT_VERSION_ATTR = "printVersion";
private static final String PRINT_DOCUMENT_INFO_ATTR = "printDocumentInfo";
private static final String PRINT_PARSE_INFO_ATTR = "printParseInfo";
private static final String PRINT_DOM_ATTR = "printDOM";
private static final String PRINT_ACCESSOR_INFO_ATTR = "printAccessorInfo";
private static final String KEEP_GENERATED_SOURCE_ATTR = "keepGeneratedSource";
private static final String CREATE_SOURCE_ATTR = "createSource";
private static final String COMPILE_SOURCE_ATTR = "compileSource";
private static final String SOURCE_OUTPUT_ROOT_ATTR = "sourceOutputRoot";
private static final String CLASS_OUTPUT_ROOT_ATTR = "classOutputRoot";
private static final String DOCUMENT_OUTPUT_ATTR = "documentOutput";
private static final String WARNINGS_ATTR = "warnings";
/**
* Deprecated attributes that have been moved to the InputDocument
* object. If a file is parsed that has these options, their values
* are moved to the InputDocument object.
*/
private static final String INPUT_DOCUMENT_ATTR = "inputDocument";
private static final String PROCESS_SSI_ATTR = "processSSI";
private static final String DOCUMENT_FORMAT_ATTR = "documentFormat";
/**
* Constructor.
*/
public CompileOptions(Document ownerDoc) {
super(ownerDoc, TAG_NAME);
}
/**
* Get the verbose attribute.
*/
public boolean getVerbose() {
return getBooleanAttribute(VERBOSE_ATTR);
}
/**
* Set the verbose attribute.
*/
public void setVerbose(boolean value) {
setBooleanAttribute(VERBOSE_ATTR, value);
}
/**
* Get the printVersion attribute.
*/
public boolean getPrintVersion() {
return getBooleanAttribute(PRINT_VERSION_ATTR);
}
/**
* Set the printVersion attribute.
*/
public void setPrintVersion(boolean value) {
setBooleanAttribute(PRINT_VERSION_ATTR, value);
}
/**
* Get the printDocumentInfo attribute.
*/
public boolean getPrintDocumentInfo() {
return getBooleanAttribute(PRINT_DOCUMENT_INFO_ATTR);
}
/**
* Set the printDocumentInfo attribute.
*/
public void setPrintDocumentInfo(boolean value) {
setBooleanAttribute(PRINT_DOCUMENT_INFO_ATTR, value);
}
/**
* Get the printParseInfo attribute.
*/
public boolean getPrintParseInfo() {
return getBooleanAttribute(PRINT_PARSE_INFO_ATTR);
}
/**
* Set the printParseInfo attribute.
*/
public void setPrintParseInfo(boolean value) {
setBooleanAttribute(PRINT_PARSE_INFO_ATTR, value);
}
/**
* Get the printDOM attribute.
*/
public boolean getPrintDOM() {
return getBooleanAttribute(PRINT_DOM_ATTR);
}
/**
* Set the printDOM attribute.
*/
public void setPrintDOM(boolean value) {
setBooleanAttribute(PRINT_DOM_ATTR, value);
}
/**
* Get the printAccessorInfo attribute.
*/
public boolean getPrintAccessorInfo() {
return getBooleanAttribute(PRINT_ACCESSOR_INFO_ATTR);
}
/**
* Set the printAccessorInfo attribute.
*/
public void setPrintAccessorInfo(boolean value) {
setBooleanAttribute(PRINT_ACCESSOR_INFO_ATTR, value);
}
/**
* Get the keepGeneratedSource attribute.
*/
public boolean getKeepGeneratedSource() {
return getBooleanAttribute(KEEP_GENERATED_SOURCE_ATTR);
}
/**
* Set the keepGeneratedSource attribute.
*/
public void setKeepGeneratedSource(boolean value) {
setBooleanAttribute(KEEP_GENERATED_SOURCE_ATTR, value);
}
/**
* Get the compileSource attribute or the default value if not specified.
* The default for this attribute is true.
*/
public boolean getCompileSource() {
return getBooleanAttribute(COMPILE_SOURCE_ATTR, true);
}
/**
* Set the compileSource attribute.
*/
public void setCompileSource(boolean value) {
setBooleanAttribute(COMPILE_SOURCE_ATTR, value, true);
}
/**
* Determine if the compileSource attribute is specified.
*/
public boolean isCompileSourceSpecified() {
return isAttributeSpecified(COMPILE_SOURCE_ATTR);
}
/**
* Get the createSource attribute or the default value if not specified.
* The default for this attribute is true.
*/
public boolean getCreateSource() {
return getBooleanAttribute(CREATE_SOURCE_ATTR, true);
}
/**
* Set the createSource attribute.
*/
public void setCreateSource(boolean value) {
setBooleanAttribute(CREATE_SOURCE_ATTR, value, true);
}
/**
* Determine if the createSource attribute is specified.
*/
public boolean isCreateSourceSpecified() {
return isAttributeSpecified(CREATE_SOURCE_ATTR);
}
/**
* Get the sourceOutputRoot attribute.
*/
public String getSourceOutputRoot() {
return getAttributeNull(SOURCE_OUTPUT_ROOT_ATTR);
}
/**
* Set the sourceOutputRoot attribute.
*/
public void setSourceOutputRoot(String value) {
setRemoveAttribute(SOURCE_OUTPUT_ROOT_ATTR, value);
}
/**
* Get the classOutputRoot attribute.
*/
public String getClassOutputRoot() {
return getAttributeNull(CLASS_OUTPUT_ROOT_ATTR);
}
/**
* Set the classOutputRoot attribute.
*/
public void setClassOutputRoot(String value) {
setRemoveAttribute(CLASS_OUTPUT_ROOT_ATTR, value);
}
/**
* Get the package output directory, obtained from the classOutputRoot
* attribute and package name. Null if returned classOutputRoot is not
* specified.
*/
public File getPackageOutputDir() {
String outputRoot = getClassOutputRoot();
if (outputRoot == null) {
outputRoot = getSourceOutputRoot();
if (outputRoot == null) {
return null;
}
}
String packageName = getMetaData().getDocumentClass().getPackageName();
return new File(new File(outputRoot),
JavaLang.packageNameToFileName(packageName));
}
/**
* Get the documentOutput attribute.
*/
public String getDocumentOutput() {
return getAttributeNull(DOCUMENT_OUTPUT_ATTR);
}
/**
* Set the documentOutput attribute.
*/
public void setDocumentOutput(String value) {
setRemoveAttribute(DOCUMENT_OUTPUT_ATTR, value);
}
/**
* Get the parser warning flag. Returns null if not specified.
*/
public boolean getWarnings() {
return getBooleanAttribute(WARNINGS_ATTR, true);
}
/**
* Set the document validation flag.
*/
public void setWarnings(boolean warnings) {
setBooleanAttribute(WARNINGS_ATTR, warnings, true);
}
/**
* Move deprecated attributes to InputDocument.
*/
private void moveToInputDocument() {
InputDocument inputDoc = getMetaData().getInputDocument();
String value = getAttributeNull(INPUT_DOCUMENT_ATTR);
if (value != null) {
inputDoc.setUrl(value);
removeAttribute(INPUT_DOCUMENT_ATTR);
}
if (isAttributeSpecified(PROCESS_SSI_ATTR)) {
inputDoc.setProcessSSI(getBooleanAttribute(PROCESS_SSI_ATTR));
removeAttribute(PROCESS_SSI_ATTR);
}
value = getAttributeNull(DOCUMENT_FORMAT_ATTR);
if (value != null) {
inputDoc.setDocumentFormat(DocumentFormat.getType(value));
removeAttribute(DOCUMENT_FORMAT_ATTR);
}
}
/**
* Complete modifications to DOM. Move d
*/
protected void completeModifications() throws XMLCException {
if (isAttributeSpecified(INPUT_DOCUMENT_ATTR)
|| isAttributeSpecified(PROCESS_SSI_ATTR)
|| isAttributeSpecified(DOCUMENT_FORMAT_ATTR)) {
moveToInputDocument();
}
}
/**
* Get the inputDocument attribute.
* @deprecated use InputDocument#getUrl()
* @see InputDocument#getUrl()
*/
public String getInputDocument() {
return getMetaData().getInputDocument().getUrl();
}
/**
* Set the inputDocument attribute.
* @deprecated use InputDocument#setUrl(String)
* @see InputDocument#setUrl(String)
*/
public void setInputDocument(String value) {
getMetaData().getInputDocument().setUrl(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy