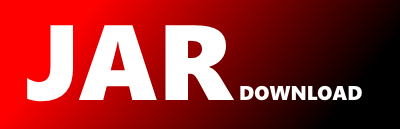
org.enhydra.xml.xmlc.metadata.DocumentClass Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: DocumentClass.java,v 1.4 2005/11/19 21:30:41 jkjome Exp $
*/
package org.enhydra.xml.xmlc.metadata;
import java.io.File;
import org.enhydra.xml.xmlc.XMLCError;
import org.enhydra.xml.xmlc.XMLCException;
import org.enhydra.xml.xmlc.codegen.JavaLang;
import org.w3c.dom.Document;
/**
* Specify properties of the XMLC document class to generate.
*/
public class DocumentClass extends MetaDataElement {
/**
* Element name.
*/
public static final String TAG_NAME = "documentClass";
/**
* Attribute names.
*/
private static final String NAME_ATTR = "name";
private static final String GENERATE_ATTR = "generate";
private static final String DELEGATE_SUPPORT_ATTR = "delegateSupport";
private static final String CREATE_META_DATA_ATTR = "createMetaData";
private static final String RECOMPILATION_ATTR = "recompilation";
private static final String DEFERRED_PARSING_ATTR = "deferParsing";
private static final String EXTENDS_ATTR = "extends";
private static final String DOM_FACTORY_ATTR = "domFactory";
private static final String DOM_ATTR = "dom";
private static final String CREATE_GET_TAG_METHODS_ATTR = "createGetTagMethods";
private static final String GET_TAG_RETURN_TYPE_ATTR = "getTagReturnType";
private static final String SUPPRESS_GET_ELEMENT_METHODS_ATTR = "suppressGetElementMethods";
/**
* Deprecated attribute that has been moved to the InputDocument object.
* If a file is parsed that has these options, its value is moved to the
* InputDocument object.
*/
private static final String RECOMPILE_SOURCE_FILE_ATTR = "recompileSourceFile";
/**
* Suffix for interface implementations.
*/
public static final String IMPLEMENTATION_SUFFIX = "Impl";
/**
* Default DOM if neither -domfactory or -dom are specified.
*/
public static final DOMType DEFAULT_DOM_TYPE = DOMType.LAZYDOM;
/*
* Argument to getTagReturnType as a short-cut for
* org.w3c.dom.Element.
*/
public static final String ACCESSOR_TYPE_ELEMENT = "Element";
/*
* Argument to getTagReturnType as a short-cut for
* org.w3c.dom.html.HTMLElement.
*/
public static final String ACCESSOR_TYPE_HTML_ELEMENT = "HTMLElement";
/*
* Argument to getTagReturnType to indicate the actual
* class name of the element should be used.
*/
public static final String ACCESSOR_TYPE_CLASS = "class";
/*
* Argument to getTagReturnType to indicate the value obtained
* from the nodeClassToInterface method in the XMLCDomFactory object being
* used to compile the document should be used.
*/
public static final String ACCESSOR_TYPE_INTERFACE = "interface";
/*
* Base class/interface name. This name is take from either the
* name attribute or determined from the source file. Its
* calculated at modification completion time to avoid throwing
* errors in get method if calculated on the fly.
* FIXME: had to go back to calculating on the fly, due to
* ordering problems in handling defaults.
*/
private transient boolean determinedBaseClassName;
private transient String baseClassName;
/**
* Constructor.
*/
public DocumentClass(Document ownerDoc) {
super(ownerDoc, TAG_NAME);
}
/**
* Get the base class name, calculating if its not already been done.
* Returns null if there is no base class.
*/
private String getBaseClassName() {
if (!determinedBaseClassName) {
if (getMetaData().getCompileOptions().getCreateSource()) {
baseClassName = classNameFromSourceName();
}
determinedBaseClassName = true;
}
return baseClassName;
}
/**
* Get the name attribute value, or the default value if its not
* specified.
*/
public String getName() {
String name = getAttributeNull(NAME_ATTR);
if (name == null) {
return getBaseClassName();
} else {
return name;
}
}
/**
* Set the name attribute value.
*/
public void setName(String value) {
setRemoveAttribute(NAME_ATTR, value);
}
/**
* Determine if the name attribute is specified.
*/
public boolean isNameSpecified() {
return isAttributeSpecified(NAME_ATTR);
}
/**
* Get the generate attribute value or the default if it is not specified.
*/
public GenerateType getGenerate() {
String value = getAttributeNull(GENERATE_ATTR);
if (value == null) {
return GenerateType.CLASS;
} else {
return GenerateType.getType(value);
}
}
/**
* Set the generate attribute value.
*/
public void setGenerate(GenerateType value) {
if (value == null) {
removeAttribute(GENERATE_ATTR);
} else {
setAttribute(GENERATE_ATTR, value.toString());
}
}
/**
* Determine if the generate attribute is specified.
*/
public boolean isGenerateSpecified() {
return isAttributeSpecified(GENERATE_ATTR);
}
/**
* Get the delegateSupport attribute value.
*/
public boolean getDelegateSupport() {
return getBooleanAttribute(DELEGATE_SUPPORT_ATTR);
}
/**
* Set the delegateSupport attribute value.
*/
public void setDelegateSupport(boolean value) {
setBooleanAttribute(DELEGATE_SUPPORT_ATTR, value);
}
/**
* Get the createMetaData attribute value.
*/
public boolean getCreateMetaData() {
return getBooleanAttribute(CREATE_META_DATA_ATTR);
}
/**
* Set the createMetaData attribute value.
*/
public void setCreateMetaData(boolean value) {
setBooleanAttribute(CREATE_META_DATA_ATTR, value);
}
/**
* Get the recompilation attribute value.
*/
public boolean getRecompilation() {
return getBooleanAttribute(RECOMPILATION_ATTR);
}
/**
* Set the recompilation attribute value. This attribute is a short cut
* for setting other attributes. Setting this to true has the
* following affects, however setting it to false doesn't modify
* any other attributes.
*
* - Sets generate to BOTH.
*
- Sets delegate support to true.
*
- Sets deferred parsing support to false;
*
*/
public void setRecompilation(boolean value) {
setBooleanAttribute(RECOMPILATION_ATTR, value);
if (value) {
setGenerate(GenerateType.BOTH);
setDelegateSupport(true);
setDeferredParsing(false);
}
}
/**
* Get the deferred parsing attribute.
*/
public boolean getDeferredParsing() {
return getBooleanAttribute(DEFERRED_PARSING_ATTR);
}
/**
* Set the deferred parsing attribute value. This attribute is a short cut
* for setting other attributes. Setting this to true has the
* following effects, however setting it to false doesn't modify
* any other attributes.
*
* - Sets generate to CLASS.
*
- Sets delegate support to false.
*
- Sets recompilation support to false.
*
*/
public void setDeferredParsing(boolean value) {
setBooleanAttribute(DEFERRED_PARSING_ATTR, value);
if (value) {
setGenerate(GenerateType.CLASS);
setDelegateSupport(false);
setRecompilation(false);
}
}
/**
* Get the extends attribute value.
*/
public String getExtends() {
return getAttributeNull(EXTENDS_ATTR);
}
/**
* Set the extends attribute value.
*/
public void setExtends(String value) {
setRemoveAttribute(EXTENDS_ATTR, value);
}
/**
* Get the domFactory attribute value.
*/
public String getDomFactory() {
return getAttributeNull(DOM_FACTORY_ATTR);
}
/**
* Set the domFactory attribute value. If not null, clears the
* dom attribute.
*/
public void setDomFactory(String value) {
setRemoveAttribute(DOM_FACTORY_ATTR, value);
if (value != null) {
removeAttribute(DOM_ATTR);
}
}
/**
* Get the dom attribute value.
*/
public DOMType getDom() {
return DOMType.getType(getAttributeNull(DOM_ATTR));
}
/**
* Set the dom attribute value. If not null, clears the
* domFactory attribute.
*/
public void setDom(DOMType value) {
if (value == null) {
removeAttribute(DOM_ATTR);
} else {
setAttribute(DOM_ATTR, value.getName());
removeAttribute(DOM_FACTORY_ATTR);
}
}
/**
* Get the XMLCDomFactory class to use. This is chosen based on
* the dom or domFactory attributes.
*/
public String getDomFactoryClass(boolean html) {
String domFactory = getAttributeNull(DOM_FACTORY_ATTR);
if (domFactory == null) {
DOMType domType = getDom();
Class dfClass;
if (domType == null) {
domType = DEFAULT_DOM_TYPE;
}
if (html) {
dfClass = domType.getHTMLDomFactoryClass();
} else {
dfClass = domType.getXMLDomFactoryClass();
}
if (dfClass == null) {
throw new XMLCError("The '" + domType.getName() +
"' DOM type only supports " +
(html?"XML":"HTML") + " documents.");
}
domFactory = dfClass.getName();
}
return domFactory;
}
/**
* Get the list of interfaces that the class will implement.
*/
public String[] getImplements() {
Implements[] impls = (Implements[])getChildren(Implements.class);
String[] implNames = new String[impls.length];
for (int idx = 0; idx < impls.length; idx++) {
implNames[idx] = impls[idx].getName();
}
return implNames;
}
/**
* Add an interface to the list of interfaces the class will implement.
*/
public void addImplements(String interfaceName) {
Implements impl
= (Implements)getOwnerDocument().createElement(Implements.TAG_NAME);
impl.setName(interfaceName);
appendChild(impl);
}
/**
* Get the package name, or null if not set.
*/
public String getPackageName() {
String base = getName();
if (base == null) {
return null;
} else {
return JavaLang.getPackageName(base);
}
}
/**
* Get the unqualified class name attribute. If only a class is being
* generated, this is the base name, otherwise its the interface name with
* `Impl" appended.
*/
public String getUnqualClassName() {
String simpleName = JavaLang.simpleClassName(getName());
if (getGenerate() == GenerateType.CLASS) {
return simpleName;
} else {
return simpleName + IMPLEMENTATION_SUFFIX;
}
}
/**
* Get the unqualified interface name, or null if there is no interface
* associated with the class.
*/
public String getUnqualInterfaceName() {
if (getGenerate() == GenerateType.CLASS) {
return null;
} else {
return JavaLang.simpleClassName(getName());
}
}
/**
* Get the source output directory (under sourceOutputRoot).
*/
private File getSourceOutputDir() {
String sourceOutputRoot = getMetaData().getCompileOptions().getSourceOutputRoot();
File sourceDirectory = null;
String packageName = getPackageName();
if ((sourceOutputRoot != null) && (packageName != null)) {
sourceDirectory = new File(sourceOutputRoot);
if (packageName != null) {
String[] packageParts = JavaLang.parseClassName(packageName);
for (int i = 0; i < packageParts.length; i++) {
sourceDirectory = new File(sourceDirectory, packageParts[i]);
}
}
}
return sourceDirectory;
}
/*
* Construct a source file path for the given unqualified name.
*/
private File getSourceFile(String unqualName) {
File sourceDirectory = getSourceOutputDir();
String fileName = unqualName + ".java";
if (sourceDirectory == null) {
return new File(fileName);
} else {
return new File(sourceDirectory, fileName);
}
}
/*
* Get the name of the generated java class source file.
*/
public File getJavaClassSource() {
return getSourceFile(getUnqualClassName());
}
/*
* Get the name of the generated java interface source file.
*/
public File getJavaInterfaceSource() {
return getSourceFile(getUnqualInterfaceName());
}
/**
* Get the createGetTagMethods attribute value.
*/
public boolean getCreateGetTagMethods() {
return getBooleanAttribute(CREATE_GET_TAG_METHODS_ATTR);
}
/**
* Set the createGetTagMethods attribute value.
*/
public void setCreateGetTagMethods(boolean value) {
setBooleanAttribute(CREATE_GET_TAG_METHODS_ATTR, value);
}
/**
* Get the return type of getTagXXX() methods or the default if not
* specified.
*/
public String getGetTagReturnType() {
String type = getAttributeNull(GET_TAG_RETURN_TYPE_ATTR);
if (type == null) {
return ACCESSOR_TYPE_ELEMENT;
} else {
return type;
}
}
/**
* Set the return type for getTagXXX() methods or null to delete
* attribute.
*/
public void setGetTagReturnType(String value) {
if (ACCESSOR_TYPE_ELEMENT.equals(value)) {
value = null;
}
setRemoveAttribute(GET_TAG_RETURN_TYPE_ATTR, value);
}
/**
* Get the createGetElementMethods attribute value.
*/
public boolean getSuppressGetElementMethods() {
return getBooleanAttribute(SUPPRESS_GET_ELEMENT_METHODS_ATTR);
}
/**
* Set the createGetElementMethods attribute value.
*/
public void setSuppressGetElementMethods(boolean value) {
setBooleanAttribute(SUPPRESS_GET_ELEMENT_METHODS_ATTR, value);
}
/**
* Get the class name from the source file name.
*/
private String classNameFromSourceName() {
String srcName = getMetaData().getInputDocument().getUrl();
if (srcName == null) {
//FIXME: should be an Exception
throw new XMLCError("No input document name from which to determine the class name");
}
String className = new File(srcName).getName();
int dotIdx = className.lastIndexOf('.');
if (dotIdx >= 0) {
className = className.substring(0, dotIdx);
}
if (!JavaLang.legalJavaIdentifier(className)) {
//FIXME: should be an Exception
throw new XMLCError("Can't use source file name \""
+ className
+ "\" as class name: "
+ "not a legal Java identifier");
}
return className;
}
/**
* Move deprecated attribute to InputDocument.
*/
private void moveToInputDocument() {
InputDocument inputDoc = getMetaData().getInputDocument();
String value = getAttributeNull(RECOMPILE_SOURCE_FILE_ATTR);
if (value != null) {
inputDoc.setRecompileSource(value);
removeAttribute(RECOMPILE_SOURCE_FILE_ATTR);
}
}
/**
* Complete modifications to DOM, check some error cases.
* @see MetaDataElement#completeModifications
*/
protected void completeModifications() throws XMLCException {
if (isAttributeSpecified(DOM_FACTORY_ATTR)
&& isAttributeSpecified(DOM_ATTR)) {
throw new XMLCException("Can't specify both the "
+ DOM_FACTORY_ATTR
+ " and " + DOM_ATTR
+ " attributes in <"
+ TAG_NAME + ">");
}
if (isAttributeSpecified(RECOMPILE_SOURCE_FILE_ATTR)) {
moveToInputDocument();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy