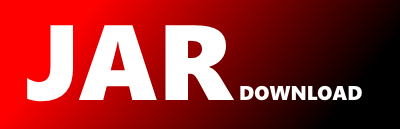
org.enhydra.xml.xmlc.metadata.HTMLSection Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: HTMLSection.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.metadata;
import java.util.ArrayList;
import org.enhydra.xml.io.Encodings;
import org.enhydra.xml.xmlc.XMLCException;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
/**
* Metadata specific to HTML documents.
*/
public class HTMLSection extends MetaDataElement {
/**
* Element name.
*/
public static final String TAG_NAME = "html";
/**
* Attribute names.
*/
private static final String ENCODING_ATTR = "encoding";
/**
* The file encoding for this platform.
*/
private String defaultFileEncoding = System.getProperty("file.encoding");
/**
* Constructor.
*/
public HTMLSection(Document ownerDoc) {
super(ownerDoc, TAG_NAME);
}
/**
* Get the default encoding. This is the default system file encoding
* unless its ASCII, in which case ISO_8859-1 is used. The name is
* converted to the MIME-preferred name.
*/
private String getDefaultEncoding() {
if (defaultFileEncoding == null) {
return Encodings.ISO_8859_1;
}
Encodings encodings = Encodings.getEncodings();
String encoding = encodings.getMIMEPreferred(defaultFileEncoding);
if (encoding != null) {
if (encodings.sameEncodings(encoding, Encodings.US_ASCII)) {
return Encodings.ISO_8859_1;
}
return encoding;
} else {
return Encodings.ISO_8859_1;
}
}
/**
* Get the encoding attribute value, or
*/
public String getEncoding() {
String encoding = getAttributeNull(ENCODING_ATTR);
if (encoding == null) {
encoding = getDefaultEncoding();
}
return encoding;
}
/**
* Set the encoding attribute value.
*/
public void setEncoding(String value) {
setRemoveAttribute(ENCODING_ATTR, value);
}
/**
* Get the htmlTagSet child elements.
*/
public HTMLTagSet[] getHTMLTagSets() {
return (HTMLTagSet[])getChildren(HTMLTagSet.class);
}
/**
* Add a htmlTagSet child element.
*/
public void addHTMLTagSet(HTMLTagSet htmlTagSet) {
appendChild(htmlTagSet);
}
/**
* Create and add a htmlTagSet child element.
*/
public HTMLTagSet addHTMLTagSet() {
HTMLTagSet htmlTagSet = new HTMLTagSet(getOwnerDocument());
appendChild(htmlTagSet);
return htmlTagSet;
}
/**
* Delete a htmlTagSet child element.
*/
public void deleteTagSet(HTMLTagSet htmlTagSet) {
removeChild(htmlTagSet);
}
/**
* Get the htmlTag child elements.
*/
public HTMLTag[] getHTMLTags() {
return (HTMLTag[])getChildren(HTMLTag.class);
}
/**
* Add a htmlTag child element.
*/
public void addHTMLTag(HTMLTag htmlTag) {
appendChild(htmlTag);
}
/**
* Create and add a htmlTag child element.
*/
public HTMLTag addHTMLTag() {
HTMLTag htmlTag = new HTMLTag(getOwnerDocument());
appendChild(htmlTag);
return htmlTag;
}
/**
* Delete a htmlTag child element.
*/
public void deleteHTMLTag(HTMLTag htmlTag) {
removeChild(htmlTag);
}
/**
* Get the htmlAttr child elements.
*/
public HTMLAttr[] getHTMLAttr() {
return (HTMLAttr[])getChildren(HTMLAttr.class);
}
/**
* Add a htmlAttr child element.
*/
public void addHTMLAttr(HTMLAttr htmlAttr) {
appendChild(htmlAttr);
}
/**
* Create and add a htmlAttr child element.
*/
public HTMLAttr addHTMLAttr() {
HTMLAttr htmlAttr = new HTMLAttr(getOwnerDocument());
appendChild(htmlAttr);
return htmlAttr;
}
/**
* Delete a htmlAttr child element.
*/
public void deleteHTMLAttr(HTMLAttr htmlAttr) {
removeChild(htmlAttr);
}
/*
* Tags from a tag set.
*/
private void addTagSetTagDefs(HTMLTagSetDef tagSet,
ArrayList tagDefs) {
HTMLTagDef[] defs = tagSet.getTagDefs();
for (int idx = 0; idx < defs.length; idx++) {
tagDefs.add(defs[idx]);
}
}
/*
* Get a list of the proprietary tags to recognize.
*/
public HTMLTagDef[] getHTMLTagDefs() {
ArrayList tagDefs = new ArrayList();
HTMLTagSet[] tagSets = getHTMLTagSets();
for (int idx = 0; idx < tagSets.length; idx++) {
addTagSetTagDefs(tagSets[idx].getTagSetDef(), tagDefs);
}
HTMLTag[] tags = getHTMLTags();
for (int idx = 0; idx < tags.length; idx++) {
tagDefs.add(tags[idx]);
}
return (HTMLTagDef[])tagDefs.toArray(new HTMLTagDef[tagDefs.size()]);
}
/*
* Add attributes from a tag set.
*/
private void addTagSetAttrDefs(HTMLTagSetDef tagSet,
ArrayList attrDefs) {
HTMLAttrDef[] defs = tagSet.getAttrDefs();
for (int idx = 0; idx < defs.length; idx++) {
attrDefs.add(defs[idx]);
}
}
/*
* Get a list of the proprietary attributes to recognize.
*/
public HTMLAttrDef[] getHTMLAttrDefs() {
ArrayList attrDefs = new ArrayList();
HTMLTagSet[] tagSets = getHTMLTagSets();
for (int idx = 0; idx < tagSets.length; idx++) {
addTagSetAttrDefs(tagSets[idx].getTagSetDef(), attrDefs);
}
HTMLAttr[] attrs = getHTMLAttr();
for (int idx = 0; idx < attrs.length; idx++) {
attrDefs.add(attrs[idx]);
}
return (HTMLAttrDef[])attrDefs.toArray(new HTMLAttrDef[attrDefs.size()]);
}
/**
* Get the compatibility child element or null if it doesn't exist.
*/
public HTMLCompatibility getCompatibility() {
return (HTMLCompatibility)getChild(HTMLCompatibility.class);
}
/**
* Get the compatibility child element, creating if it doesn't exist.
*/
public HTMLCompatibility getCreateCompatibility() {
return (HTMLCompatibility)getCreateChild(HTMLCompatibility.class);
}
/**
* Set the compatibility child element, or delete with null.
*/
public void setCompatibility(HTMLCompatibility compatibility) {
setChild(compatibility);
}
/**
* Complete modifications to DOM.
* @see MetaDataElement#completeModifications
*/
protected void completeModifications() throws XMLCException {
super.completeModifications();
}
/**
* Merge another element into this element.
*/
protected void mergeElement(MetaDataElement srcElement) {
mergeAttributes(srcElement);
mergeSingletonChild(HTMLCompatibility.class, srcElement);
// Import other children
Document document = getOwnerDocument();
for (Node child = srcElement.getFirstChild();
child != null;
child = child.getNextSibling()) {
if (!(child instanceof HTMLCompatibility)) {
appendChild(document.importNode(child, true));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy