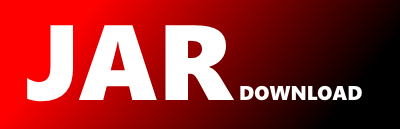
org.enhydra.xml.xmlc.metadata.InputDocument Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: InputDocument.java,v 1.3 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.metadata;
import java.io.File;
import org.enhydra.xml.xmlc.codegen.JavaLang;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
/**
* Specifies the document to compile.
*/
public class InputDocument extends Include {
/**
* Element name.
*/
public static final String TAG_NAME = "inputDocument";
/**
* Attribute names.
*/
private static final String PROCESS_SSI_ATTR = "processSSI";
private static final String DOCUMENT_FORMAT_ATTR = "documentFormat";
private static final String RECOMPILE_SOURCE_ATTR = "recompileSource";
// dbr_20020128.2_start
private static final String SSI_BASE_ATTR = "SSIBase";
// dbr_20020128.2_end
/**
* Constructor.
*/
public InputDocument(Document ownerDoc) {
super(ownerDoc, TAG_NAME);
}
/**
* Get the input source. Derived from the inputDocument attribute,
* plus explictly specified encoding if HTML.
*/
public InputSource getInputSource() {
String url = getUrl();
if (url == null) {
return null;
}
InputSource inputSource = new InputSource(url);
HTMLSection htmlMetaData = getMetaData().getHTMLSection();
if (htmlMetaData != null) {
String htmlEncoding = htmlMetaData.getEncoding();
if (htmlEncoding != null) {
inputSource.setEncoding(htmlEncoding);
}
}
return inputSource;
}
/**
* Normalize a file to be a unix path name.
*/
private String normalizeToUnixFile(String name) {
return name.replace('\\', '/');
}
/**
* Get the processSSI attribute.
*/
public boolean getProcessSSI() {
return getBooleanAttribute(PROCESS_SSI_ATTR);
}
/**
* Set the processSSI attribute.
*/
public void setProcessSSI(boolean value) {
setBooleanAttribute(PROCESS_SSI_ATTR, value);
}
// dbr_20020128.1_start
/**
* Set the SSIBase attribute.
*/
public void setSSIBase(String value) {
setRemoveAttribute(SSI_BASE_ATTR, value);
}
/**
* Get the SSIBase attribute.
*/
public String getSSIBase() {
return getAttributeNull(SSI_BASE_ATTR);
}
// dbr_20020128.1_end
/**
* Get the documentFormat attribute.
*/
public DocumentFormat getDocumentFormat() {
return DocumentFormat.getType(getAttributeNull(DOCUMENT_FORMAT_ATTR));
}
/**
* Set the documentFormat attribute.
*/
public void setDocumentFormat(DocumentFormat docType) {
setRemoveAttribute(DOCUMENT_FORMAT_ATTR, docType.getName());
}
/**
* Get the default recompile source.
*/
private String getDefaultRecompileSource() {
String inputFile = getUrl();
if (inputFile == null) {
return null; // No name...
}
String name = new File(inputFile).getName();
// Add in package name, if available.
String packageName = getMetaData().getDocumentClass().getPackageName();
if (packageName != null) {
name = JavaLang.packageNameToUnixFileName(packageName)
+ "/" + name;
}
return name;
}
/**
* Get the recompileSource attribute value. Value is always
* normalized to a Unix-style pathname. Returns the default
* if the attribute is not specified or null if there is no
* default.
*/
public String getRecompileSource() {
String name = getAttributeNull(RECOMPILE_SOURCE_ATTR);
if (name == null) {
name = getDefaultRecompileSource();
}
if (name != null) {
return normalizeToUnixFile(name);
} else {
return null;
}
}
/**
* Set the recompileSource attribute value.
*/
public void setRecompileSource(String value) {
if (value != null) {
value = normalizeToUnixFile(value);
}
setRemoveAttribute(RECOMPILE_SOURCE_ATTR, value);
}
/**
* Determine if the recompileSource attribute is specified.
*/
public boolean isRecompileSourceSpecified() {
return isAttributeSpecified(RECOMPILE_SOURCE_ATTR);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy