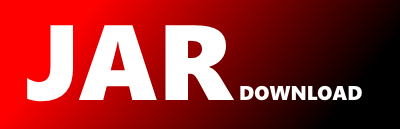
org.enhydra.xml.xmlc.metadata.JavaCompilerSection Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: JavaCompilerSection.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.metadata;
import java.util.ArrayList;
import org.w3c.dom.Document;
/**
* Java compiler metadata.
*/
public class JavaCompilerSection extends MetaDataElement {
/**
* Element name.
*/
public static final String TAG_NAME = "javaCompiler";
/**
* Attribute names.
*/
private static final String JAVAC_ATTR = "javac";
/**
* Default javac compiler.
*/
public static final String DEFAULT_JAVAC = "javac";
/**
* Constructor.
*/
public JavaCompilerSection(Document ownerDoc) {
super(ownerDoc, TAG_NAME);
}
/**
* Get the value of the javac attribute or the default if not specified.
*/
public String getJavac() {
String javac = getAttributeNull(JAVAC_ATTR);
if (javac == null) {
return DEFAULT_JAVAC;
} else {
return javac;
}
}
/**
* Set the value of the javac attribute.
*/
public void setJavac(String value) {
setAttribute(JAVAC_ATTR, value);
}
/**
* Determine if the javac attribute is specified.
*/
public boolean isJavacSpecified() {
return isAttributeSpecified(JAVAC_ATTR);
}
/**
* Get the JavacOption child elements.
*/
public JavacOption[] getJavacOptions() {
return (JavacOption[])getChildren(JavacOption.class);
}
/**
* Add a JavacOption child element.
*/
public void addJavacOption(JavacOption javacOption) {
appendChild(javacOption);
}
/**
* Create and add a new JavacOption child element.
*/
public JavacOption addJavacOption() {
JavacOption javacOption = new JavacOption(getOwnerDocument());
appendChild(javacOption);
return javacOption;
}
/**
* Delete a JavacOption child element.
*/
public void deleteJavacOption(JavacOption javacOption) {
removeChild(javacOption);
}
/**
* Delete a JavacOption child element by name. Ignore if it doesn't
* exist. Deletes all copies if specified multiple times.
*/
public void deleteJavacOption(String optionName) {
JavacOption[] options = getJavacOptions();
for (int idx = 0; idx < options.length; idx++) {
if (options[idx].getName().equals(optionName)) {
removeChild(options[idx]);
}
}
}
/*
* Get the javac arguments (flags + options), in command line form.
*/
public String[] getJavacArgs() {
ArrayList args = new ArrayList();
JavacOption[] options = getJavacOptions();
for (int idx = 0; idx < options.length; idx++) {
args.add(options[idx].getName());
String value = options[idx].getValue();
if (value != null) {
args.add(value);
}
}
return (String[])args.toArray(new String[args.size()]);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy