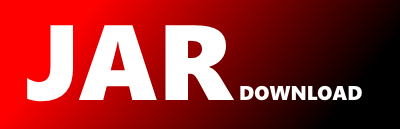
org.enhydra.xml.xmlc.metadata.MetaData Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: MetaData.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.metadata;
import org.w3c.dom.Document;
/*
* Notes:
* Can't create section elements in constructor or we will get in
* a second when added by the parser. Instead, create them on the
* fly.
*/
/**
* Root element of XMLC metadata. The child elements of this class represent
* the major sections of the XMLC metadata. Only one instance of a section
* element object can exist. All sections are created on first reference
* if they don't exist to make it easy to use them to get defaults.
*/
public class MetaData extends MetaDataElement {
/**
* Element name.
*/
public static final String TAG_NAME = "xmlc";
/**
* Constructor.
*/
public MetaData(Document ownerDoc) {
super(ownerDoc, TAG_NAME);
}
/**
* Get the parser metadata or null if it doesn't exist.
*/
public Parser getParser() {
return (Parser)getCreateChild(Parser.class);
}
/**
* Set the parser metadata, or delete by setting to null.
*/
public void setParser(Parser parser) {
if (parser == null) {
deleteChild(Parser.class);
} else {
setChild(parser);
}
}
/**
* Get the document class metadata or null if it doesn't exist.
*/
public DocumentClass getDocumentClass() {
return (DocumentClass)getCreateChild(DocumentClass.class);
}
/**
* Set the document class metdata, or delete by specifying null.
*/
public void setDocumentClass(DocumentClass documentClass) {
if (documentClass == null) {
deleteChild(DocumentClass.class);
} else {
setChild(documentClass);
}
}
/**
* Get the HTML metadata or null if it doesn't exist.
*/
public HTMLSection getHTMLSection() {
return (HTMLSection)getCreateChild(HTMLSection.class);
}
/**
* Set the HTML metadata, or delete by specifying null.
*/
public void setHTMLSection(HTMLSection htmlMetaData) {
if (htmlMetaData == null) {
deleteChild(HTMLSection.class);
} else {
setChild(htmlMetaData);
}
}
/**
* Get the DOM edits metadata or null if it doesn't exist.
*/
public DOMEdits getDOMEdits() {
return (DOMEdits)getCreateChild(DOMEdits.class);
}
/**
* Set the DOM edits metadata, or delete by specifying null.
*/
public void setDOMEdits(DOMEdits domEditsMetaData) {
if (domEditsMetaData == null) {
deleteChild(DOMEdits.class);
} else {
setChild(domEditsMetaData);
}
}
/**
* Get the java compiler metadata or null if it doesn't exist.
*/
public JavaCompilerSection getJavaCompilerSection() {
return (JavaCompilerSection)getCreateChild(JavaCompilerSection.class);
}
/**
* Set the java compiler metadata, or delete by specifying null.
*/
public void setJavaCompiler(JavaCompilerSection compilerSection) {
if (compilerSection == null) {
deleteChild(JavaCompilerSection.class);
} else {
setChild(compilerSection);
}
}
/**
* Get the document metadata or null if it doesn't exist.
*/
public DocumentSection getDocumentSection() {
return (DocumentSection)getCreateChild(DocumentSection.class);
}
/**
* Set the document metadata, or delete by specifying null.
*/
public void setDocumentSection(DocumentSection documentMetaData) {
if (documentMetaData == null) {
deleteChild(DocumentSection.class);
} else {
setChild(documentMetaData);
}
}
/**
* Get the compile options or null if it doesn't exist.
*/
public CompileOptions getCompileOptions() {
return (CompileOptions)getCreateChild(CompileOptions.class);
}
/**
* Set the compile options, or delete by specifying null.
*/
public void setCompileOptions(CompileOptions compileOptions) {
if (compileOptions == null) {
deleteChild(CompileOptions.class);
} else {
setChild(compileOptions);
}
}
/**
* Get the input document or null if it doesn't exist.
*/
public InputDocument getInputDocument() {
return (InputDocument)getCreateChild(InputDocument.class);
}
/**
* Set the input document, or delete by specifying null.
*/
public void setInputDocument(InputDocument inputDocument) {
if (inputDocument == null) {
deleteChild(InputDocument.class);
} else {
setChild(inputDocument);
}
}
/**
* Merge another element into this element. Don't append clones of the
* children, merge them.
*/
protected void mergeElement(MetaDataElement srcElement) {
mergeAttributes(srcElement);
mergeSingletonChild(Parser.class, srcElement);
mergeSingletonChild(DocumentClass.class, srcElement);
mergeSingletonChild(HTMLSection.class, srcElement);
mergeSingletonChild(DOMEdits.class, srcElement);
mergeSingletonChild(JavaCompilerSection.class, srcElement);
mergeSingletonChild(DocumentSection.class, srcElement);
mergeSingletonChild(CompileOptions.class, srcElement);
mergeSingletonChild(InputDocument.class, srcElement);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy