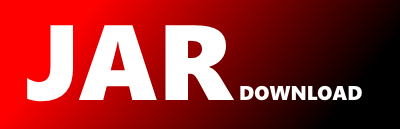
org.enhydra.xml.xmlc.metadata.Parser Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: Parser.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.metadata;
import org.w3c.dom.Document;
//FIXME: modify Xerces to take a already created document class.
/**
* Specifies the parser and parsing options.
*/
public class Parser extends MetaDataElement {
/**
* Element name.
*/
public static final String TAG_NAME = "parser";
/**
* Attribute names.
*/
private static final String NAME_ATTR = "name";
private static final String VALIDATE_ATTR = "validate";
private static final String WARNINGS_ATTR = "warnings";
/**
* Constructor.
*/
public Parser(Document ownerDoc) {
super(ownerDoc, TAG_NAME);
}
/**
* Get the xcatalog metadata.
*/
public XCatalog[] getXCatalog() {
return (XCatalog[])getChildren(XCatalog.class);
}
/**
* Add a XCatalog metadata object.
*/
public void addXCatalog(XCatalog xCatalog) {
appendChild(xCatalog);
}
/**
* Create and add a XCatalog metadata object.
*/
public XCatalog addXCatalog() {
XCatalog xCatalog = new XCatalog(getOwnerDocument());
appendChild(xCatalog);
return xCatalog;
}
/**
* Delete a XCatalog metadata object.
*/
public void deleteXCatalog(XCatalog xCatalog) {
removeChild(xCatalog);
}
/**
* Get the list of XCatalog file URLs.
*/
public String[] getXCatalogURLs() {
XCatalog[] xCatalogs = getXCatalog();
String[] urls = new String[xCatalogs.length];
for (int idx = 0; idx < xCatalogs.length; idx++) {
urls[idx] = xCatalogs[idx].getUrl();
}
return urls;
}
/**
* Get the parser to use.
*/
public ParserType getName() {
return ParserType.getType(getAttributeNull(NAME_ATTR));
}
/**
* Set the parser to use.
*/
public void setName(ParserType value) {
if (value == null) {
removeAttribute(NAME_ATTR);
} else {
setAttribute(NAME_ATTR, value.getName());
}
}
/**
* Get the document validation flag. Returns null if not specified.
*/
public Boolean getValidate() {
return getBooleanObjectAttribute(VALIDATE_ATTR);
}
/**
* Set the document validation flag. Use a null value to make
* attribute unspecified.
*/
public void setValidate(Boolean validate) {
setBooleanObjectAttribute(VALIDATE_ATTR, validate);
}
/**
* Set the document validation flag.
*/
public void setValidate(boolean validate) {
setBooleanAttribute(VALIDATE_ATTR, validate);
}
/**
* Get the parser warning flag. Returns null if not specified.
*/
public boolean getWarnings() {
return getBooleanAttribute(WARNINGS_ATTR, true);
}
/**
* Set the document validation flag.
*/
public void setWarnings(boolean warnings) {
setBooleanAttribute(WARNINGS_ATTR, warnings, true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy