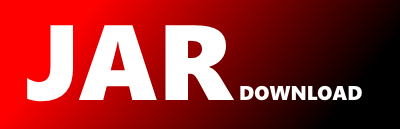
org.enhydra.xml.xmlc.misc.LineNumberMap Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: LineNumberMap.java,v 1.1.1.1 2003/03/10 16:36:23 taweili Exp $
*/
package org.enhydra.xml.xmlc.misc;
import java.util.ArrayList;
/**
* Table that keeps a map of character offsets and line count in an input
* stream to file name and line numbers in source files. This is used to
* handle mapping of indexes or line numbers in a stream generted by including
* files to those in the original files.
*/
public class LineNumberMap {
/**
* Structure to record the char offset in an input stream for
* a line. Objects of this class are immutable.
*/
public final class Line {
/** File were encountered. */
private final String fFileName;
/** One-based line number in the source file. */
private final int fLineNum;
/** One-based line number in the char stream */
private final int fStreamLineNum;
/** Zero-based stream char offset. */
private final int fStreamCharOffset;
/**
* Constructor
*/
Line(String fileName,
int lineNum,
int streamLineNum,
int streamCharOffset) {
fFileName = fileName;
fLineNum = lineNum;
fStreamLineNum = streamLineNum;
fStreamCharOffset = streamCharOffset;
}
//FIXME: should really use the term systemId rather than fileName.
/** Get the file name. */
public String getFileName() {
return fFileName;
}
/** Get one-based source line number. */
public int getLineNum() {
return fLineNum;
}
/** Get one-based stream line number. */
public int getStreamLineNum() {
return fStreamLineNum;
}
/** Zero-based stream char offset. */
public int getStreamCharOffset() {
return fStreamCharOffset;
}
/** Get String reprsentation for debugging */
public String toString() {
return "(" + fFileName + " " + fLineNum + ") => ("
+ fStreamLineNum + " " + fStreamCharOffset + ")";
}
}
/**
* Array of line records. We don't currently provide indexing as
* this is normally search only on warnings or errors, so the overhead
* of keeping an index is avoided.
*/
private ArrayList fLines = new ArrayList();
/**
* Add a line to the map. This object currently assumes that
* all lines are added in sequential order.
*/
public final void addLine(String fileName,
int lineNum,
int streamLineNum,
int streamCharOffset) {
fLines.add(new Line(fileName, lineNum,
streamLineNum, streamCharOffset));
}
/**
* Get the filname end line number for a stream line number.
*/
public final Line getLineFromOffset(int streamCharOffset) {
// Start from the end of the list, as this is normally
// used to find errors that just occured.
for (int idx = fLines.size() - 1; idx >= 0; idx--) {
Line line = (Line)fLines.get(idx);
if (streamCharOffset >= line.getStreamCharOffset()) {
return line;
}
}
return null;
}
/**
* Get the filname end line number for a stream character offset.
*/
public final Line getLineFromLineNum(int streamLineNum) {
// Start from the end of the list, as this is normally
// used to find errors that just occured.
for (int idx = fLines.size() - 1; idx >= 0; idx--) {
Line line = (Line)fLines.get(idx);
if (streamLineNum >= line.getStreamLineNum()) {
return line;
}
}
return null;
}
/** Get String reprsentation for debugging */
public String toString() {
StringBuffer buf = new StringBuffer(4096); // Something bigger than default.
for (int idx = 0; idx < fLines.size(); idx++) {
buf.append(fLines.get(idx).toString());
buf.append('\n');
}
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy