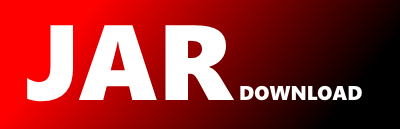
org.enhydra.xml.xmlc.misc.ReadIterator Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: ReadIterator.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.misc;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* Wrapper around Iterator objects that doesn't allow modification.
*/
public class ReadIterator implements Iterator {
/**
* Wrapper iterator.
*/
private Iterator fIter;
/**
* Constructor.
* @param iter Iterator to wrap.
*/
public ReadIterator(Iterator iter) {
fIter = iter;
}
/**
* Returns true if the iteration has more elements. (In other words,
* returns true if next would return an element rather than throwing
* an exception).
* @return true if the iterator has more elements.
* @see Iterator#hasNext
*/
public boolean hasNext() {
return fIter.hasNext();
}
/**
* Returns the next element in the interation.
* @exception NoSuchElementException iteration has no more elements.
*/
public Object next() {
return fIter.next();
}
/**
* Throws an exception, preventing modification of the underlying
* structure.
* @exception UnsupportedOperationException Always thrown.
*/
public void remove() {
throw new UnsupportedOperationException("Modification through this iterator is not allowed");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy