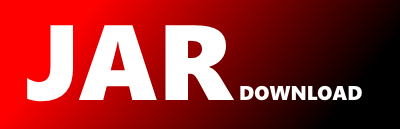
org.enhydra.xml.xmlc.parsers.ParseTracer Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: ParseTracer.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc.parsers;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.Writer;
import org.enhydra.xml.xmlc.codegen.IndentWriter;
/**
* Object used to trace parser calls. Handles indentation for logging the
* parsing of nested elements. This also is a PrintWriter, so it can be
* be passed to methods not expecting a ParseTracer.
* @see PrintWriter
*/
public class ParseTracer extends IndentWriter {
/**
* Is tracing enabled?
*/
private boolean fEnabled;
/**
* Writer that discards all output, used to pass to super class if
* disabled. Normally not called, as explict enable checks are done
* in in commonly used methods.
*/
private static class NullWriter extends Writer {
/** Constructor */
public NullWriter() {
}
/** @see Writer#write(int) */
public void write(int c) throws IOException {
}
/** @see Writer#write(char[],int,int) */
public void write(char[] cbuf, int off, int len) throws IOException {
}
/** @see Writer#flush */
public void flush() throws IOException {
}
/** @see Writer#close */
public void close() throws IOException {
}
}
/**
* Create tracer. If traceOut is null, it is created
* disabled.
*/
public ParseTracer(PrintWriter traceOut) {
super(((traceOut != null) ? traceOut
: new PrintWriter(new NullWriter())), true);
fEnabled = (traceOut != null);
setZeroCheck(false);
}
/**
* Determine if tracing is enabled.
*/
public final boolean enabled() {
return fEnabled;
}
/**
* Format a right-justified number.
* FIXME: should be a utility somewhere.
*/
private String formatNumber(int number,
int width) {
String num = Integer.toString(number);
StringBuffer buf = new StringBuffer(num.length()+width);
for (int cnt = width-num.length(); cnt >= 0; cnt--) {
buf.append(' ');
}
buf.append(num);
return buf.toString();
}
/**
* Generate trace output with indentation.
*/
public void trace(String str) {
if (fEnabled) {
printPrefix(formatNumber(getIndentLevel(), 2) + ">");
super.println(str);
}
}
/**
* Print a string, indenting at the beginning of lines.
*/
public void print(String str) {
if (fEnabled) {
super.print(str);
}
}
/**
* Print a newline.
*/
public void println() {
if (fEnabled) {
super.println();
}
}
/**
* Print a string and newline, indenting at the beginning of lines.
*/
public void println(String str) {
if (fEnabled) {
super.println(str);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy