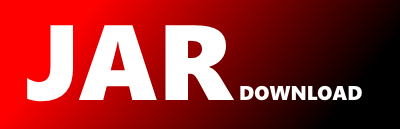
net.opengis.gml._3.ObjectFactory Maven / Gradle / Ivy
Show all versions of netex-java-model Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package net.opengis.gml._3;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the net.opengis.gml._3 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AbstractObject_QNAME = new QName("http://www.opengis.net/gml/3.2", "AbstractObject");
private final static QName _AbstractGML_QNAME = new QName("http://www.opengis.net/gml/3.2", "AbstractGML");
private final static QName _AbstractAssociationRole_QNAME = new QName("http://www.opengis.net/gml/3.2", "abstractAssociationRole");
private final static QName _AbstractStrictAssociationRole_QNAME = new QName("http://www.opengis.net/gml/3.2", "abstractStrictAssociationRole");
private final static QName _AbstractReference_QNAME = new QName("http://www.opengis.net/gml/3.2", "abstractReference");
private final static QName _AbstractInlineProperty_QNAME = new QName("http://www.opengis.net/gml/3.2", "abstractInlineProperty");
private final static QName _ReversePropertyName_QNAME = new QName("http://www.opengis.net/gml/3.2", "reversePropertyName");
private final static QName _DescriptionReference_QNAME = new QName("http://www.opengis.net/gml/3.2", "descriptionReference");
private final static QName _Name_QNAME = new QName("http://www.opengis.net/gml/3.2", "name");
private final static QName _Identifier_QNAME = new QName("http://www.opengis.net/gml/3.2", "identifier");
private final static QName _TargetElement_QNAME = new QName("http://www.opengis.net/gml/3.2", "targetElement");
private final static QName _AssociationName_QNAME = new QName("http://www.opengis.net/gml/3.2", "associationName");
private final static QName _DefaultCodeSpace_QNAME = new QName("http://www.opengis.net/gml/3.2", "defaultCodeSpace");
private final static QName _GmlProfileSchema_QNAME = new QName("http://www.opengis.net/gml/3.2", "gmlProfileSchema");
private final static QName _AbstractGeometry_QNAME = new QName("http://www.opengis.net/gml/3.2", "AbstractGeometry");
private final static QName _Pos_QNAME = new QName("http://www.opengis.net/gml/3.2", "pos");
private final static QName _PosList_QNAME = new QName("http://www.opengis.net/gml/3.2", "posList");
private final static QName _Vector_QNAME = new QName("http://www.opengis.net/gml/3.2", "vector");
private final static QName _Envelope_QNAME = new QName("http://www.opengis.net/gml/3.2", "Envelope");
private final static QName _AbstractGeometricPrimitive_QNAME = new QName("http://www.opengis.net/gml/3.2", "AbstractGeometricPrimitive");
private final static QName _Point_QNAME = new QName("http://www.opengis.net/gml/3.2", "Point");
private final static QName _PointProperty_QNAME = new QName("http://www.opengis.net/gml/3.2", "pointProperty");
private final static QName _AbstractCurve_QNAME = new QName("http://www.opengis.net/gml/3.2", "AbstractCurve");
private final static QName _CurveProperty_QNAME = new QName("http://www.opengis.net/gml/3.2", "curveProperty");
private final static QName _LineString_QNAME = new QName("http://www.opengis.net/gml/3.2", "LineString");
private final static QName _AbstractSurface_QNAME = new QName("http://www.opengis.net/gml/3.2", "AbstractSurface");
private final static QName _SurfaceProperty_QNAME = new QName("http://www.opengis.net/gml/3.2", "surfaceProperty");
private final static QName _Polygon_QNAME = new QName("http://www.opengis.net/gml/3.2", "Polygon");
private final static QName _Exterior_QNAME = new QName("http://www.opengis.net/gml/3.2", "exterior");
private final static QName _Interior_QNAME = new QName("http://www.opengis.net/gml/3.2", "interior");
private final static QName _AbstractRing_QNAME = new QName("http://www.opengis.net/gml/3.2", "AbstractRing");
private final static QName _LinearRing_QNAME = new QName("http://www.opengis.net/gml/3.2", "LinearRing");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: net.opengis.gml._3
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link AssociationRoleType }
*
*/
public AssociationRoleType createAssociationRoleType() {
return new AssociationRoleType();
}
/**
* Create an instance of {@link ReferenceType }
*
*/
public ReferenceType createReferenceType() {
return new ReferenceType();
}
/**
* Create an instance of {@link InlinePropertyType }
*
*/
public InlinePropertyType createInlinePropertyType() {
return new InlinePropertyType();
}
/**
* Create an instance of {@link CodeType }
*
*/
public CodeType createCodeType() {
return new CodeType();
}
/**
* Create an instance of {@link CodeWithAuthorityType }
*
*/
public CodeWithAuthorityType createCodeWithAuthorityType() {
return new CodeWithAuthorityType();
}
/**
* Create an instance of {@link DirectPositionType }
*
*/
public DirectPositionType createDirectPositionType() {
return new DirectPositionType();
}
/**
* Create an instance of {@link DirectPositionListType }
*
*/
public DirectPositionListType createDirectPositionListType() {
return new DirectPositionListType();
}
/**
* Create an instance of {@link VectorType }
*
*/
public VectorType createVectorType() {
return new VectorType();
}
/**
* Create an instance of {@link EnvelopeType }
*
*/
public EnvelopeType createEnvelopeType() {
return new EnvelopeType();
}
/**
* Create an instance of {@link PointType }
*
*/
public PointType createPointType() {
return new PointType();
}
/**
* Create an instance of {@link PointPropertyType }
*
*/
public PointPropertyType createPointPropertyType() {
return new PointPropertyType();
}
/**
* Create an instance of {@link CurvePropertyType }
*
*/
public CurvePropertyType createCurvePropertyType() {
return new CurvePropertyType();
}
/**
* Create an instance of {@link LineStringType }
*
*/
public LineStringType createLineStringType() {
return new LineStringType();
}
/**
* Create an instance of {@link SurfacePropertyType }
*
*/
public SurfacePropertyType createSurfacePropertyType() {
return new SurfacePropertyType();
}
/**
* Create an instance of {@link PolygonType }
*
*/
public PolygonType createPolygonType() {
return new PolygonType();
}
/**
* Create an instance of {@link AbstractRingPropertyType }
*
*/
public AbstractRingPropertyType createAbstractRingPropertyType() {
return new AbstractRingPropertyType();
}
/**
* Create an instance of {@link LinearRingType }
*
*/
public LinearRingType createLinearRingType() {
return new LinearRingType();
}
/**
* Create an instance of {@link MeasureType }
*
*/
public MeasureType createMeasureType() {
return new MeasureType();
}
/**
* Create an instance of {@link CoordinatesType }
*
*/
public CoordinatesType createCoordinatesType() {
return new CoordinatesType();
}
/**
* Create an instance of {@link CodeListType }
*
*/
public CodeListType createCodeListType() {
return new CodeListType();
}
/**
* Create an instance of {@link CodeOrNilReasonListType }
*
*/
public CodeOrNilReasonListType createCodeOrNilReasonListType() {
return new CodeOrNilReasonListType();
}
/**
* Create an instance of {@link MeasureListType }
*
*/
public MeasureListType createMeasureListType() {
return new MeasureListType();
}
/**
* Create an instance of {@link MeasureOrNilReasonListType }
*
*/
public MeasureOrNilReasonListType createMeasureOrNilReasonListType() {
return new MeasureOrNilReasonListType();
}
/**
* Create an instance of {@link GeometryPropertyType }
*
*/
public GeometryPropertyType createGeometryPropertyType() {
return new GeometryPropertyType();
}
/**
* Create an instance of {@link GeometryArrayPropertyType }
*
*/
public GeometryArrayPropertyType createGeometryArrayPropertyType() {
return new GeometryArrayPropertyType();
}
/**
* Create an instance of {@link GeometricPrimitivePropertyType }
*
*/
public GeometricPrimitivePropertyType createGeometricPrimitivePropertyType() {
return new GeometricPrimitivePropertyType();
}
/**
* Create an instance of {@link PointArrayPropertyType }
*
*/
public PointArrayPropertyType createPointArrayPropertyType() {
return new PointArrayPropertyType();
}
/**
* Create an instance of {@link CurveArrayPropertyType }
*
*/
public CurveArrayPropertyType createCurveArrayPropertyType() {
return new CurveArrayPropertyType();
}
/**
* Create an instance of {@link SurfaceArrayPropertyType }
*
*/
public SurfaceArrayPropertyType createSurfaceArrayPropertyType() {
return new SurfaceArrayPropertyType();
}
/**
* Create an instance of {@link LinearRingPropertyType }
*
*/
public LinearRingPropertyType createLinearRingPropertyType() {
return new LinearRingPropertyType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/gml/3.2", name = "AbstractObject")
public JAXBElement