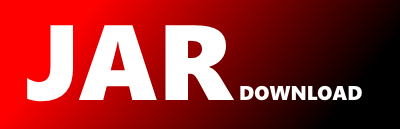
org.rutebanken.netex.model.Block_VersionStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.math.BigInteger;
import java.time.Duration;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.util.Collection;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.migesok.jaxb.adapter.javatime.DurationXmlAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
import org.rutebanken.util.OffsetTimeISO8601XmlAdapter;
/**
* Java class for Block_VersionStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Block_VersionStructure">
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}DataManagedObjectStructure">
* <sequence>
* <group ref="{http://www.netex.org.uk/netex}BlockGroup"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Block_VersionStructure", propOrder = {
"name",
"description",
"privateCode",
"preparationDuration",
"startTime",
"startTimeDayOffset",
"finishingDuration",
"endTime",
"endTimeDayOffset",
"dayTypes",
"vehicleServicePartRef",
"vehicleTypeRef",
"startPointRef",
"endPointRef",
"journeys",
"coursesOfJourneys",
"blockParts",
"reliefOpportunities"
})
@XmlSeeAlso({
Block.class,
TrainBlock_VersionStructure.class
})
public class Block_VersionStructure
extends DataManagedObjectStructure
{
@XmlElement(name = "Name")
protected MultilingualString name;
@XmlElement(name = "Description")
protected MultilingualString description;
@XmlElement(name = "PrivateCode")
protected PrivateCodeStructure privateCode;
@XmlElement(name = "PreparationDuration", type = String.class)
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration preparationDuration;
@XmlElement(name = "StartTime", type = String.class)
@XmlJavaTypeAdapter(OffsetTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime startTime;
@XmlElement(name = "StartTimeDayOffset")
protected BigInteger startTimeDayOffset;
@XmlElement(name = "FinishingDuration", type = String.class)
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration finishingDuration;
@XmlElement(name = "EndTime", type = String.class)
@XmlJavaTypeAdapter(OffsetTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime endTime;
@XmlElement(name = "EndTimeDayOffset")
protected BigInteger endTimeDayOffset;
protected Block_VersionStructure.DayTypes dayTypes;
@XmlElement(name = "VehicleServicePartRef")
protected VehicleServicePartRefStructure vehicleServicePartRef;
@XmlElementRef(name = "VehicleTypeRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends VehicleTypeRefStructure> vehicleTypeRef;
@XmlElement(name = "StartPointRef")
protected PointRefStructure startPointRef;
@XmlElement(name = "EndPointRef")
protected PointRefStructure endPointRef;
protected JourneyRefs_RelStructure journeys;
protected CoursesOfJourneys_RelStructure coursesOfJourneys;
protected BlockParts_RelStructure blockParts;
protected ReliefOpportunities_RelStructure reliefOpportunities;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link MultilingualString }
*
*/
public MultilingualString getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link MultilingualString }
*
*/
public void setName(MultilingualString value) {
this.name = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link MultilingualString }
*
*/
public MultilingualString getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link MultilingualString }
*
*/
public void setDescription(MultilingualString value) {
this.description = value;
}
/**
* Gets the value of the privateCode property.
*
* @return
* possible object is
* {@link PrivateCodeStructure }
*
*/
public PrivateCodeStructure getPrivateCode() {
return privateCode;
}
/**
* Sets the value of the privateCode property.
*
* @param value
* allowed object is
* {@link PrivateCodeStructure }
*
*/
public void setPrivateCode(PrivateCodeStructure value) {
this.privateCode = value;
}
/**
* Gets the value of the preparationDuration property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getPreparationDuration() {
return preparationDuration;
}
/**
* Sets the value of the preparationDuration property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPreparationDuration(Duration value) {
this.preparationDuration = value;
}
/**
* Gets the value of the startTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getStartTime() {
return startTime;
}
/**
* Sets the value of the startTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStartTime(OffsetTime value) {
this.startTime = value;
}
/**
* Gets the value of the startTimeDayOffset property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getStartTimeDayOffset() {
return startTimeDayOffset;
}
/**
* Sets the value of the startTimeDayOffset property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setStartTimeDayOffset(BigInteger value) {
this.startTimeDayOffset = value;
}
/**
* Gets the value of the finishingDuration property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getFinishingDuration() {
return finishingDuration;
}
/**
* Sets the value of the finishingDuration property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFinishingDuration(Duration value) {
this.finishingDuration = value;
}
/**
* Gets the value of the endTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getEndTime() {
return endTime;
}
/**
* Sets the value of the endTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEndTime(OffsetTime value) {
this.endTime = value;
}
/**
* Gets the value of the endTimeDayOffset property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getEndTimeDayOffset() {
return endTimeDayOffset;
}
/**
* Sets the value of the endTimeDayOffset property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setEndTimeDayOffset(BigInteger value) {
this.endTimeDayOffset = value;
}
/**
* Gets the value of the dayTypes property.
*
* @return
* possible object is
* {@link Block_VersionStructure.DayTypes }
*
*/
public Block_VersionStructure.DayTypes getDayTypes() {
return dayTypes;
}
/**
* Sets the value of the dayTypes property.
*
* @param value
* allowed object is
* {@link Block_VersionStructure.DayTypes }
*
*/
public void setDayTypes(Block_VersionStructure.DayTypes value) {
this.dayTypes = value;
}
/**
* Gets the value of the vehicleServicePartRef property.
*
* @return
* possible object is
* {@link VehicleServicePartRefStructure }
*
*/
public VehicleServicePartRefStructure getVehicleServicePartRef() {
return vehicleServicePartRef;
}
/**
* Sets the value of the vehicleServicePartRef property.
*
* @param value
* allowed object is
* {@link VehicleServicePartRefStructure }
*
*/
public void setVehicleServicePartRef(VehicleServicePartRefStructure value) {
this.vehicleServicePartRef = value;
}
/**
* Gets the value of the vehicleTypeRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link CompoundTrainRef }{@code >}
* {@link JAXBElement }{@code <}{@link TrainRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link VehicleTypeRefStructure }{@code >}
*
*/
public JAXBElement extends VehicleTypeRefStructure> getVehicleTypeRef() {
return vehicleTypeRef;
}
/**
* Sets the value of the vehicleTypeRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link CompoundTrainRef }{@code >}
* {@link JAXBElement }{@code <}{@link TrainRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link VehicleTypeRefStructure }{@code >}
*
*/
public void setVehicleTypeRef(JAXBElement extends VehicleTypeRefStructure> value) {
this.vehicleTypeRef = value;
}
/**
* Gets the value of the startPointRef property.
*
* @return
* possible object is
* {@link PointRefStructure }
*
*/
public PointRefStructure getStartPointRef() {
return startPointRef;
}
/**
* Sets the value of the startPointRef property.
*
* @param value
* allowed object is
* {@link PointRefStructure }
*
*/
public void setStartPointRef(PointRefStructure value) {
this.startPointRef = value;
}
/**
* Gets the value of the endPointRef property.
*
* @return
* possible object is
* {@link PointRefStructure }
*
*/
public PointRefStructure getEndPointRef() {
return endPointRef;
}
/**
* Sets the value of the endPointRef property.
*
* @param value
* allowed object is
* {@link PointRefStructure }
*
*/
public void setEndPointRef(PointRefStructure value) {
this.endPointRef = value;
}
/**
* Gets the value of the journeys property.
*
* @return
* possible object is
* {@link JourneyRefs_RelStructure }
*
*/
public JourneyRefs_RelStructure getJourneys() {
return journeys;
}
/**
* Sets the value of the journeys property.
*
* @param value
* allowed object is
* {@link JourneyRefs_RelStructure }
*
*/
public void setJourneys(JourneyRefs_RelStructure value) {
this.journeys = value;
}
/**
* Gets the value of the coursesOfJourneys property.
*
* @return
* possible object is
* {@link CoursesOfJourneys_RelStructure }
*
*/
public CoursesOfJourneys_RelStructure getCoursesOfJourneys() {
return coursesOfJourneys;
}
/**
* Sets the value of the coursesOfJourneys property.
*
* @param value
* allowed object is
* {@link CoursesOfJourneys_RelStructure }
*
*/
public void setCoursesOfJourneys(CoursesOfJourneys_RelStructure value) {
this.coursesOfJourneys = value;
}
/**
* Gets the value of the blockParts property.
*
* @return
* possible object is
* {@link BlockParts_RelStructure }
*
*/
public BlockParts_RelStructure getBlockParts() {
return blockParts;
}
/**
* Sets the value of the blockParts property.
*
* @param value
* allowed object is
* {@link BlockParts_RelStructure }
*
*/
public void setBlockParts(BlockParts_RelStructure value) {
this.blockParts = value;
}
/**
* Gets the value of the reliefOpportunities property.
*
* @return
* possible object is
* {@link ReliefOpportunities_RelStructure }
*
*/
public ReliefOpportunities_RelStructure getReliefOpportunities() {
return reliefOpportunities;
}
/**
* Sets the value of the reliefOpportunities property.
*
* @param value
* allowed object is
* {@link ReliefOpportunities_RelStructure }
*
*/
public void setReliefOpportunities(ReliefOpportunities_RelStructure value) {
this.reliefOpportunities = value;
}
public Block_VersionStructure withName(MultilingualString value) {
setName(value);
return this;
}
public Block_VersionStructure withDescription(MultilingualString value) {
setDescription(value);
return this;
}
public Block_VersionStructure withPrivateCode(PrivateCodeStructure value) {
setPrivateCode(value);
return this;
}
public Block_VersionStructure withPreparationDuration(Duration value) {
setPreparationDuration(value);
return this;
}
public Block_VersionStructure withStartTime(OffsetTime value) {
setStartTime(value);
return this;
}
public Block_VersionStructure withStartTimeDayOffset(BigInteger value) {
setStartTimeDayOffset(value);
return this;
}
public Block_VersionStructure withFinishingDuration(Duration value) {
setFinishingDuration(value);
return this;
}
public Block_VersionStructure withEndTime(OffsetTime value) {
setEndTime(value);
return this;
}
public Block_VersionStructure withEndTimeDayOffset(BigInteger value) {
setEndTimeDayOffset(value);
return this;
}
public Block_VersionStructure withDayTypes(Block_VersionStructure.DayTypes value) {
setDayTypes(value);
return this;
}
public Block_VersionStructure withVehicleServicePartRef(VehicleServicePartRefStructure value) {
setVehicleServicePartRef(value);
return this;
}
public Block_VersionStructure withVehicleTypeRef(JAXBElement extends VehicleTypeRefStructure> value) {
setVehicleTypeRef(value);
return this;
}
public Block_VersionStructure withStartPointRef(PointRefStructure value) {
setStartPointRef(value);
return this;
}
public Block_VersionStructure withEndPointRef(PointRefStructure value) {
setEndPointRef(value);
return this;
}
public Block_VersionStructure withJourneys(JourneyRefs_RelStructure value) {
setJourneys(value);
return this;
}
public Block_VersionStructure withCoursesOfJourneys(CoursesOfJourneys_RelStructure value) {
setCoursesOfJourneys(value);
return this;
}
public Block_VersionStructure withBlockParts(BlockParts_RelStructure value) {
setBlockParts(value);
return this;
}
public Block_VersionStructure withReliefOpportunities(ReliefOpportunities_RelStructure value) {
setReliefOpportunities(value);
return this;
}
@Override
public Block_VersionStructure withKeyList(KeyListStructure value) {
setKeyList(value);
return this;
}
@Override
public Block_VersionStructure withExtensions(ExtensionsStructure value) {
setExtensions(value);
return this;
}
@Override
public Block_VersionStructure withBrandingRef(BrandingRefStructure value) {
setBrandingRef(value);
return this;
}
@Override
public Block_VersionStructure withAlternativeTexts(AlternativeTexts_RelStructure value) {
setAlternativeTexts(value);
return this;
}
@Override
public Block_VersionStructure withResponsibilitySetRef(String value) {
setResponsibilitySetRef(value);
return this;
}
@Override
public Block_VersionStructure withValidityConditions(ValidityConditions_RelStructure value) {
setValidityConditions(value);
return this;
}
@Override
public Block_VersionStructure withValidBetween(ValidBetween... values) {
if (values!= null) {
for (ValidBetween value: values) {
getValidBetween().add(value);
}
}
return this;
}
@Override
public Block_VersionStructure withValidBetween(Collection values) {
if (values!= null) {
getValidBetween().addAll(values);
}
return this;
}
@Override
public Block_VersionStructure withDataSourceRef(String value) {
setDataSourceRef(value);
return this;
}
@Override
public Block_VersionStructure withCreated(OffsetDateTime value) {
setCreated(value);
return this;
}
@Override
public Block_VersionStructure withChanged(OffsetDateTime value) {
setChanged(value);
return this;
}
@Override
public Block_VersionStructure withModification(ModificationEnumeration value) {
setModification(value);
return this;
}
@Override
public Block_VersionStructure withVersion(String value) {
setVersion(value);
return this;
}
@Override
public Block_VersionStructure withStatus_BasicModificationDetailsGroup(StatusEnumeration value) {
setStatus_BasicModificationDetailsGroup(value);
return this;
}
@Override
public Block_VersionStructure withDerivedFromVersionRef_BasicModificationDetailsGroup(String value) {
setDerivedFromVersionRef_BasicModificationDetailsGroup(value);
return this;
}
@Override
public Block_VersionStructure withCompatibleWithVersionFrameVersionRef(String value) {
setCompatibleWithVersionFrameVersionRef(value);
return this;
}
@Override
public Block_VersionStructure withDerivedFromObjectRef(String value) {
setDerivedFromObjectRef(value);
return this;
}
@Override
public Block_VersionStructure withNameOfClass(String value) {
setNameOfClass(value);
return this;
}
@Override
public Block_VersionStructure withId(String value) {
setId(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}dayTypeRefs_RelStructure">
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class DayTypes
extends DayTypeRefs_RelStructure
{
@Override
public Block_VersionStructure.DayTypes withDayTypeRef(JAXBElement extends DayTypeRefStructure> ... values) {
if (values!= null) {
for (JAXBElement extends DayTypeRefStructure> value: values) {
getDayTypeRef().add(value);
}
}
return this;
}
@Override
public Block_VersionStructure.DayTypes withDayTypeRef(Collection> values) {
if (values!= null) {
getDayTypeRef().addAll(values);
}
return this;
}
@Override
public Block_VersionStructure.DayTypes withModificationSet(ModificationSetEnumeration value) {
setModificationSet(value);
return this;
}
@Override
public Block_VersionStructure.DayTypes withId(String value) {
setId(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy