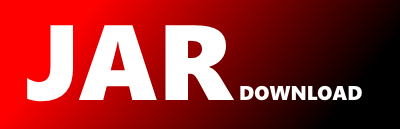
org.rutebanken.netex.model.Cell_VersionedChildStructure Maven / Gradle / Ivy
Show all versions of netex-java-model Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.math.BigInteger;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
/**
* Java class for Cell_VersionedChildStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Cell_VersionedChildStructure">
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}VersionedChildStructure">
* <sequence>
* <group ref="{http://www.netex.org.uk/netex}CellGroup"/>
* </sequence>
* <attribute name="order" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Cell_VersionedChildStructure", propOrder = {
"name",
"description",
"cellPrice",
"farePriceRef",
"priceGroupRef",
"priceableObjectRef",
"groupOfDistanceMatrixElementsRef",
"directionType",
"routingType",
"groupOfLinesRef",
"lineRef",
"siteRef",
"fareClass",
"classOfUseRef",
"facilitySetRef",
"typeOfProductCategoryRef",
"typeOfServiceRef",
"serviceJourneyRef",
"trainNumberRef",
"groupOfServicesRef",
"typeOfFareProductRef",
"distributionChannelRef",
"groupOfDistributionChannelsRef",
"fareTableRef",
"columnRef",
"rowRef"
})
@XmlSeeAlso({
Cell.class,
org.rutebanken.netex.model.Cells_RelStructure.CellInContext.class
})
public class Cell_VersionedChildStructure
extends VersionedChildStructure
{
@XmlElement(name = "Name")
protected MultilingualString name;
@XmlElement(name = "Description")
protected MultilingualString description;
@XmlElement(name = "CellPrice")
protected FarePrice_VersionedChildStructure cellPrice;
@XmlElementRef(name = "FarePriceRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends FarePriceRefStructure> farePriceRef;
@XmlElement(name = "PriceGroupRef")
protected PriceGroupRefStructure priceGroupRef;
@XmlElementRef(name = "PriceableObjectRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected List> priceableObjectRef;
@XmlElement(name = "GroupOfDistanceMatrixElementsRef")
protected GroupOfDistanceMatrixElementsRefStructureElement groupOfDistanceMatrixElementsRef;
@XmlElement(name = "DirectionType", defaultValue = "both")
@XmlSchemaType(name = "normalizedString")
protected RelativeDirectionEnumeration directionType;
@XmlElement(name = "RoutingType", defaultValue = "both")
@XmlSchemaType(name = "normalizedString")
protected RoutingTypeEnumeration routingType;
@XmlElementRef(name = "GroupOfLinesRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends GroupOfLinesRefStructure> groupOfLinesRef;
@XmlElementRef(name = "LineRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends LineRefStructure> lineRef;
@XmlElementRef(name = "SiteRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends SiteRefStructure> siteRef;
@XmlElement(name = "FareClass", defaultValue = "unknown")
@XmlSchemaType(name = "NMTOKEN")
protected FareClassEnumeration fareClass;
@XmlElement(name = "ClassOfUseRef")
protected ClassOfUseRef classOfUseRef;
@XmlElementRef(name = "FacilitySetRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends FacilitySetRefStructure> facilitySetRef;
@XmlElement(name = "TypeOfProductCategoryRef")
protected TypeOfProductCategoryRefStructure typeOfProductCategoryRef;
@XmlElement(name = "TypeOfServiceRef")
protected TypeOfServiceRefStructure typeOfServiceRef;
@XmlElementRef(name = "ServiceJourneyRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends ServiceJourneyRefStructure> serviceJourneyRef;
@XmlElement(name = "TrainNumberRef")
protected TrainNumberRefStructure trainNumberRef;
@XmlElement(name = "GroupOfServicesRef")
protected GroupOfServicesRefStructure groupOfServicesRef;
@XmlElement(name = "TypeOfFareProductRef")
protected TypeOfFareProductRefStructure typeOfFareProductRef;
@XmlElement(name = "DistributionChannelRef")
protected DistributionChannelRefStructureElement distributionChannelRef;
@XmlElement(name = "GroupOfDistributionChannelsRef")
protected GroupOfDistributionChannelsRefStructure groupOfDistributionChannelsRef;
@XmlElementRef(name = "FareTableRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends FareTableRefStructure> fareTableRef;
@XmlElement(name = "ColumnRef")
protected FareTableColumnRefStructure columnRef;
@XmlElement(name = "RowRef")
protected FareTableRowRefStructure rowRef;
@XmlAttribute(name = "order")
protected BigInteger order;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link MultilingualString }
*
*/
public MultilingualString getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link MultilingualString }
*
*/
public void setName(MultilingualString value) {
this.name = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link MultilingualString }
*
*/
public MultilingualString getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link MultilingualString }
*
*/
public void setDescription(MultilingualString value) {
this.description = value;
}
/**
* Gets the value of the cellPrice property.
*
* @return
* possible object is
* {@link FarePrice_VersionedChildStructure }
*
*/
public FarePrice_VersionedChildStructure getCellPrice() {
return cellPrice;
}
/**
* Sets the value of the cellPrice property.
*
* @param value
* allowed object is
* {@link FarePrice_VersionedChildStructure }
*
*/
public void setCellPrice(FarePrice_VersionedChildStructure value) {
this.cellPrice = value;
}
/**
* Gets the value of the farePriceRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link QualityStructureFactorPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ValidableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SalesPackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CustomerPurchasePackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareProductPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FarePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ControllableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageParameterPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FulfilmentMethodPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareStructureElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DistanceMatrixElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SeriesConstraintPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CappingRulePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ParkingPriceRefStructure }{@code >}
*
*/
public JAXBElement extends FarePriceRefStructure> getFarePriceRef() {
return farePriceRef;
}
/**
* Sets the value of the farePriceRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link QualityStructureFactorPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ValidableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SalesPackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CustomerPurchasePackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareProductPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FarePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ControllableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageParameterPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FulfilmentMethodPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareStructureElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DistanceMatrixElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SeriesConstraintPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CappingRulePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ParkingPriceRefStructure }{@code >}
*
*/
public void setFarePriceRef(JAXBElement extends FarePriceRefStructure> value) {
this.farePriceRef = value;
}
/**
* Gets the value of the priceGroupRef property.
*
* @return
* possible object is
* {@link PriceGroupRefStructure }
*
*/
public PriceGroupRefStructure getPriceGroupRef() {
return priceGroupRef;
}
/**
* Sets the value of the priceGroupRef property.
*
* @param value
* allowed object is
* {@link PriceGroupRefStructure }
*
*/
public void setPriceGroupRef(PriceGroupRefStructure value) {
this.priceGroupRef = value;
}
/**
* Gets the value of the priceableObjectRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the priceableObjectRef property.
*
*
* For example, to add a new item, do as follows:
*
* getPriceableObjectRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link JAXBElement }{@code <}{@link ServiceAccessRightRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ReplacingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link RoundTripRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FrequencyOfUseRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link StepLimitRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareIntervalRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ControllableElementRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareStructureElementRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeIntervalRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeStructureFactorRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ReservingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalStructureFactorRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareStructureFactorRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ResellingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SalesPackageRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageParameterRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalIntervalRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link QualityStructureFactorRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CancellingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TransferabilityRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UserProfileRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PurchaseWindowRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SaleDiscountRightRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SupplementProductRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageParameterRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareProductRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link RefundingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ValidableElementRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link RoutingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CommercialProfileRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CappedDiscountRightRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SalesPackageElementRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link AmountOfPriceUnitProductRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ExchangingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ChargingPolicyRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareQuotaFactorRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GroupTicketRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FulfilmentMethodRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareDemandFactorRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CustomerPurchasePackageElementRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SeriesConstraintRef }{@code >}
* {@link JAXBElement }{@code <}{@link LuggageAllowanceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PenaltyPolicyRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DistanceMatrixElementRefStructureElement }{@code >}
* {@link JAXBElement }{@code <}{@link CappingRuleRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link EntitlementProductRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link EntitlementRequiredRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link EntitlementGivenRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CustomerPurchasePackageRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link MinimumStayRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageDiscountRightRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageValidityPeriodRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ThirdPartyProductRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link InterchangingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PreassignedFareProductRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PriceableObjectRefStructure }{@code >}
*
*
*/
public List> getPriceableObjectRef() {
if (priceableObjectRef == null) {
priceableObjectRef = new ArrayList>();
}
return this.priceableObjectRef;
}
/**
* Gets the value of the groupOfDistanceMatrixElementsRef property.
*
* @return
* possible object is
* {@link GroupOfDistanceMatrixElementsRefStructureElement }
*
*/
public GroupOfDistanceMatrixElementsRefStructureElement getGroupOfDistanceMatrixElementsRef() {
return groupOfDistanceMatrixElementsRef;
}
/**
* Sets the value of the groupOfDistanceMatrixElementsRef property.
*
* @param value
* allowed object is
* {@link GroupOfDistanceMatrixElementsRefStructureElement }
*
*/
public void setGroupOfDistanceMatrixElementsRef(GroupOfDistanceMatrixElementsRefStructureElement value) {
this.groupOfDistanceMatrixElementsRef = value;
}
/**
* Gets the value of the directionType property.
*
* @return
* possible object is
* {@link RelativeDirectionEnumeration }
*
*/
public RelativeDirectionEnumeration getDirectionType() {
return directionType;
}
/**
* Sets the value of the directionType property.
*
* @param value
* allowed object is
* {@link RelativeDirectionEnumeration }
*
*/
public void setDirectionType(RelativeDirectionEnumeration value) {
this.directionType = value;
}
/**
* Gets the value of the routingType property.
*
* @return
* possible object is
* {@link RoutingTypeEnumeration }
*
*/
public RoutingTypeEnumeration getRoutingType() {
return routingType;
}
/**
* Sets the value of the routingType property.
*
* @param value
* allowed object is
* {@link RoutingTypeEnumeration }
*
*/
public void setRoutingType(RoutingTypeEnumeration value) {
this.routingType = value;
}
/**
* Gets the value of the groupOfLinesRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link GroupOfLinesRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link NetworkRefStructure }{@code >}
*
*/
public JAXBElement extends GroupOfLinesRefStructure> getGroupOfLinesRef() {
return groupOfLinesRef;
}
/**
* Sets the value of the groupOfLinesRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link GroupOfLinesRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link NetworkRefStructure }{@code >}
*
*/
public void setGroupOfLinesRef(JAXBElement extends GroupOfLinesRefStructure> value) {
this.groupOfLinesRef = value;
}
/**
* Gets the value of the lineRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link FlexibleLineRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link LineRefStructure }{@code >}
*
*/
public JAXBElement extends LineRefStructure> getLineRef() {
return lineRef;
}
/**
* Sets the value of the lineRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link FlexibleLineRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link LineRefStructure }{@code >}
*
*/
public void setLineRef(JAXBElement extends LineRefStructure> value) {
this.lineRef = value;
}
/**
* Gets the value of the siteRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link PointOfInterestRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link StopPlaceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SiteRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ParkingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServiceSIteRefStructure }{@code >}
*
*/
public JAXBElement extends SiteRefStructure> getSiteRef() {
return siteRef;
}
/**
* Sets the value of the siteRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link PointOfInterestRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link StopPlaceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SiteRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ParkingRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServiceSIteRefStructure }{@code >}
*
*/
public void setSiteRef(JAXBElement extends SiteRefStructure> value) {
this.siteRef = value;
}
/**
* Gets the value of the fareClass property.
*
* @return
* possible object is
* {@link FareClassEnumeration }
*
*/
public FareClassEnumeration getFareClass() {
return fareClass;
}
/**
* Sets the value of the fareClass property.
*
* @param value
* allowed object is
* {@link FareClassEnumeration }
*
*/
public void setFareClass(FareClassEnumeration value) {
this.fareClass = value;
}
/**
* Gets the value of the classOfUseRef property.
*
* @return
* possible object is
* {@link ClassOfUseRef }
*
*/
public ClassOfUseRef getClassOfUseRef() {
return classOfUseRef;
}
/**
* Sets the value of the classOfUseRef property.
*
* @param value
* allowed object is
* {@link ClassOfUseRef }
*
*/
public void setClassOfUseRef(ClassOfUseRef value) {
this.classOfUseRef = value;
}
/**
* Gets the value of the facilitySetRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link SiteFacilitySetRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServiceFacilitySetRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FacilitySetRefStructure }{@code >}
*
*/
public JAXBElement extends FacilitySetRefStructure> getFacilitySetRef() {
return facilitySetRef;
}
/**
* Sets the value of the facilitySetRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link SiteFacilitySetRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServiceFacilitySetRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FacilitySetRefStructure }{@code >}
*
*/
public void setFacilitySetRef(JAXBElement extends FacilitySetRefStructure> value) {
this.facilitySetRef = value;
}
/**
* Gets the value of the typeOfProductCategoryRef property.
*
* @return
* possible object is
* {@link TypeOfProductCategoryRefStructure }
*
*/
public TypeOfProductCategoryRefStructure getTypeOfProductCategoryRef() {
return typeOfProductCategoryRef;
}
/**
* Sets the value of the typeOfProductCategoryRef property.
*
* @param value
* allowed object is
* {@link TypeOfProductCategoryRefStructure }
*
*/
public void setTypeOfProductCategoryRef(TypeOfProductCategoryRefStructure value) {
this.typeOfProductCategoryRef = value;
}
/**
* Gets the value of the typeOfServiceRef property.
*
* @return
* possible object is
* {@link TypeOfServiceRefStructure }
*
*/
public TypeOfServiceRefStructure getTypeOfServiceRef() {
return typeOfServiceRef;
}
/**
* Sets the value of the typeOfServiceRef property.
*
* @param value
* allowed object is
* {@link TypeOfServiceRefStructure }
*
*/
public void setTypeOfServiceRef(TypeOfServiceRefStructure value) {
this.typeOfServiceRef = value;
}
/**
* Gets the value of the serviceJourneyRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link ServiceJourneyRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TemplateServiceJourneyRefStructure }{@code >}
*
*/
public JAXBElement extends ServiceJourneyRefStructure> getServiceJourneyRef() {
return serviceJourneyRef;
}
/**
* Sets the value of the serviceJourneyRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link ServiceJourneyRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TemplateServiceJourneyRefStructure }{@code >}
*
*/
public void setServiceJourneyRef(JAXBElement extends ServiceJourneyRefStructure> value) {
this.serviceJourneyRef = value;
}
/**
* Gets the value of the trainNumberRef property.
*
* @return
* possible object is
* {@link TrainNumberRefStructure }
*
*/
public TrainNumberRefStructure getTrainNumberRef() {
return trainNumberRef;
}
/**
* Sets the value of the trainNumberRef property.
*
* @param value
* allowed object is
* {@link TrainNumberRefStructure }
*
*/
public void setTrainNumberRef(TrainNumberRefStructure value) {
this.trainNumberRef = value;
}
/**
* Gets the value of the groupOfServicesRef property.
*
* @return
* possible object is
* {@link GroupOfServicesRefStructure }
*
*/
public GroupOfServicesRefStructure getGroupOfServicesRef() {
return groupOfServicesRef;
}
/**
* Sets the value of the groupOfServicesRef property.
*
* @param value
* allowed object is
* {@link GroupOfServicesRefStructure }
*
*/
public void setGroupOfServicesRef(GroupOfServicesRefStructure value) {
this.groupOfServicesRef = value;
}
/**
* Gets the value of the typeOfFareProductRef property.
*
* @return
* possible object is
* {@link TypeOfFareProductRefStructure }
*
*/
public TypeOfFareProductRefStructure getTypeOfFareProductRef() {
return typeOfFareProductRef;
}
/**
* Sets the value of the typeOfFareProductRef property.
*
* @param value
* allowed object is
* {@link TypeOfFareProductRefStructure }
*
*/
public void setTypeOfFareProductRef(TypeOfFareProductRefStructure value) {
this.typeOfFareProductRef = value;
}
/**
* Gets the value of the distributionChannelRef property.
*
* @return
* possible object is
* {@link DistributionChannelRefStructureElement }
*
*/
public DistributionChannelRefStructureElement getDistributionChannelRef() {
return distributionChannelRef;
}
/**
* Sets the value of the distributionChannelRef property.
*
* @param value
* allowed object is
* {@link DistributionChannelRefStructureElement }
*
*/
public void setDistributionChannelRef(DistributionChannelRefStructureElement value) {
this.distributionChannelRef = value;
}
/**
* Gets the value of the groupOfDistributionChannelsRef property.
*
* @return
* possible object is
* {@link GroupOfDistributionChannelsRefStructure }
*
*/
public GroupOfDistributionChannelsRefStructure getGroupOfDistributionChannelsRef() {
return groupOfDistributionChannelsRef;
}
/**
* Sets the value of the groupOfDistributionChannelsRef property.
*
* @param value
* allowed object is
* {@link GroupOfDistributionChannelsRefStructure }
*
*/
public void setGroupOfDistributionChannelsRef(GroupOfDistributionChannelsRefStructure value) {
this.groupOfDistributionChannelsRef = value;
}
/**
* Gets the value of the fareTableRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link StandardFareTableRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareTableRefStructure }{@code >}
*
*/
public JAXBElement extends FareTableRefStructure> getFareTableRef() {
return fareTableRef;
}
/**
* Sets the value of the fareTableRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link StandardFareTableRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareTableRefStructure }{@code >}
*
*/
public void setFareTableRef(JAXBElement extends FareTableRefStructure> value) {
this.fareTableRef = value;
}
/**
* Gets the value of the columnRef property.
*
* @return
* possible object is
* {@link FareTableColumnRefStructure }
*
*/
public FareTableColumnRefStructure getColumnRef() {
return columnRef;
}
/**
* Sets the value of the columnRef property.
*
* @param value
* allowed object is
* {@link FareTableColumnRefStructure }
*
*/
public void setColumnRef(FareTableColumnRefStructure value) {
this.columnRef = value;
}
/**
* Gets the value of the rowRef property.
*
* @return
* possible object is
* {@link FareTableRowRefStructure }
*
*/
public FareTableRowRefStructure getRowRef() {
return rowRef;
}
/**
* Sets the value of the rowRef property.
*
* @param value
* allowed object is
* {@link FareTableRowRefStructure }
*
*/
public void setRowRef(FareTableRowRefStructure value) {
this.rowRef = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setOrder(BigInteger value) {
this.order = value;
}
public Cell_VersionedChildStructure withName(MultilingualString value) {
setName(value);
return this;
}
public Cell_VersionedChildStructure withDescription(MultilingualString value) {
setDescription(value);
return this;
}
public Cell_VersionedChildStructure withCellPrice(FarePrice_VersionedChildStructure value) {
setCellPrice(value);
return this;
}
public Cell_VersionedChildStructure withFarePriceRef(JAXBElement extends FarePriceRefStructure> value) {
setFarePriceRef(value);
return this;
}
public Cell_VersionedChildStructure withPriceGroupRef(PriceGroupRefStructure value) {
setPriceGroupRef(value);
return this;
}
public Cell_VersionedChildStructure withPriceableObjectRef(JAXBElement extends PriceableObjectRefStructure> ... values) {
if (values!= null) {
for (JAXBElement extends PriceableObjectRefStructure> value: values) {
getPriceableObjectRef().add(value);
}
}
return this;
}
public Cell_VersionedChildStructure withPriceableObjectRef(Collection> values) {
if (values!= null) {
getPriceableObjectRef().addAll(values);
}
return this;
}
public Cell_VersionedChildStructure withGroupOfDistanceMatrixElementsRef(GroupOfDistanceMatrixElementsRefStructureElement value) {
setGroupOfDistanceMatrixElementsRef(value);
return this;
}
public Cell_VersionedChildStructure withDirectionType(RelativeDirectionEnumeration value) {
setDirectionType(value);
return this;
}
public Cell_VersionedChildStructure withRoutingType(RoutingTypeEnumeration value) {
setRoutingType(value);
return this;
}
public Cell_VersionedChildStructure withGroupOfLinesRef(JAXBElement extends GroupOfLinesRefStructure> value) {
setGroupOfLinesRef(value);
return this;
}
public Cell_VersionedChildStructure withLineRef(JAXBElement extends LineRefStructure> value) {
setLineRef(value);
return this;
}
public Cell_VersionedChildStructure withSiteRef(JAXBElement extends SiteRefStructure> value) {
setSiteRef(value);
return this;
}
public Cell_VersionedChildStructure withFareClass(FareClassEnumeration value) {
setFareClass(value);
return this;
}
public Cell_VersionedChildStructure withClassOfUseRef(ClassOfUseRef value) {
setClassOfUseRef(value);
return this;
}
public Cell_VersionedChildStructure withFacilitySetRef(JAXBElement extends FacilitySetRefStructure> value) {
setFacilitySetRef(value);
return this;
}
public Cell_VersionedChildStructure withTypeOfProductCategoryRef(TypeOfProductCategoryRefStructure value) {
setTypeOfProductCategoryRef(value);
return this;
}
public Cell_VersionedChildStructure withTypeOfServiceRef(TypeOfServiceRefStructure value) {
setTypeOfServiceRef(value);
return this;
}
public Cell_VersionedChildStructure withServiceJourneyRef(JAXBElement extends ServiceJourneyRefStructure> value) {
setServiceJourneyRef(value);
return this;
}
public Cell_VersionedChildStructure withTrainNumberRef(TrainNumberRefStructure value) {
setTrainNumberRef(value);
return this;
}
public Cell_VersionedChildStructure withGroupOfServicesRef(GroupOfServicesRefStructure value) {
setGroupOfServicesRef(value);
return this;
}
public Cell_VersionedChildStructure withTypeOfFareProductRef(TypeOfFareProductRefStructure value) {
setTypeOfFareProductRef(value);
return this;
}
public Cell_VersionedChildStructure withDistributionChannelRef(DistributionChannelRefStructureElement value) {
setDistributionChannelRef(value);
return this;
}
public Cell_VersionedChildStructure withGroupOfDistributionChannelsRef(GroupOfDistributionChannelsRefStructure value) {
setGroupOfDistributionChannelsRef(value);
return this;
}
public Cell_VersionedChildStructure withFareTableRef(JAXBElement extends FareTableRefStructure> value) {
setFareTableRef(value);
return this;
}
public Cell_VersionedChildStructure withColumnRef(FareTableColumnRefStructure value) {
setColumnRef(value);
return this;
}
public Cell_VersionedChildStructure withRowRef(FareTableRowRefStructure value) {
setRowRef(value);
return this;
}
public Cell_VersionedChildStructure withOrder(BigInteger value) {
setOrder(value);
return this;
}
@Override
public Cell_VersionedChildStructure withExtensions(ExtensionsStructure value) {
setExtensions(value);
return this;
}
@Override
public Cell_VersionedChildStructure withValidityConditions(ValidityConditions_RelStructure value) {
setValidityConditions(value);
return this;
}
@Override
public Cell_VersionedChildStructure withValidBetween(ValidBetween... values) {
if (values!= null) {
for (ValidBetween value: values) {
getValidBetween().add(value);
}
}
return this;
}
@Override
public Cell_VersionedChildStructure withValidBetween(Collection values) {
if (values!= null) {
getValidBetween().addAll(values);
}
return this;
}
@Override
public Cell_VersionedChildStructure withDataSourceRef(String value) {
setDataSourceRef(value);
return this;
}
@Override
public Cell_VersionedChildStructure withCreated(OffsetDateTime value) {
setCreated(value);
return this;
}
@Override
public Cell_VersionedChildStructure withChanged(OffsetDateTime value) {
setChanged(value);
return this;
}
@Override
public Cell_VersionedChildStructure withModification(ModificationEnumeration value) {
setModification(value);
return this;
}
@Override
public Cell_VersionedChildStructure withVersion(String value) {
setVersion(value);
return this;
}
@Override
public Cell_VersionedChildStructure withStatus_BasicModificationDetailsGroup(StatusEnumeration value) {
setStatus_BasicModificationDetailsGroup(value);
return this;
}
@Override
public Cell_VersionedChildStructure withDerivedFromVersionRef_BasicModificationDetailsGroup(String value) {
setDerivedFromVersionRef_BasicModificationDetailsGroup(value);
return this;
}
@Override
public Cell_VersionedChildStructure withCompatibleWithVersionFrameVersionRef(String value) {
setCompatibleWithVersionFrameVersionRef(value);
return this;
}
@Override
public Cell_VersionedChildStructure withDerivedFromObjectRef(String value) {
setDerivedFromObjectRef(value);
return this;
}
@Override
public Cell_VersionedChildStructure withNameOfClass(String value) {
setNameOfClass(value);
return this;
}
@Override
public Cell_VersionedChildStructure withId(String value) {
setId(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}