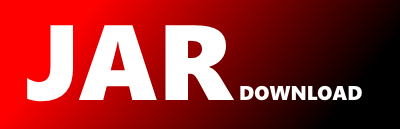
org.rutebanken.netex.model.ConditionSummaryStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
/**
* Java class for ConditionSummaryStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ConditionSummaryStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="FareStructureType" type="{http://www.netex.org.uk/netex}FareStructureTypeEnumeration" minOccurs="0"/>
* <element name="TariffBasis" type="{http://www.netex.org.uk/netex}TariffBasisEnumeration" minOccurs="0"/>
* <element name="HasNotices" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <group ref="{http://www.netex.org.uk/netex}ConditionSummaryCardGroup"/>
* <group ref="{http://www.netex.org.uk/netex}ConditionSummaryEntitlementGroup"/>
* <group ref="{http://www.netex.org.uk/netex}ConditionSummaryTravelGroup"/>
* <group ref="{http://www.netex.org.uk/netex}ConditionSummaryCommercialGroup"/>
* <group ref="{http://www.netex.org.uk/netex}ConditionSummaryReservationGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ConditionSummaryStructure", propOrder = {
"fareStructureType",
"tariffBasis",
"hasNotices",
"providesCard",
"goesOnCard",
"isPersonal",
"requiresPhoto",
"mustCarry",
"requiresAccount",
"isSupplement",
"requiresEntitlement",
"givesEntitlement",
"hasOperatorRestrictions",
"hasTravelTimeRestrictions",
"hasRouteRestrictions",
"trainRestrictions",
"hasZoneRestrictions",
"canBreakJourney",
"returnTripsOnly",
"nightTrain",
"canChangeClass",
"isRefundable",
"isExchangable",
"hasExchangeFee",
"hasDiscountedFares",
"allowAdditionalDiscounts",
"allowCompanionDiscounts",
"hasMinimumPrice",
"requiresPositiveBalance",
"hasPurchaseConditions",
"hasDynamicPricing",
"requiresReservation",
"hasReservationFee",
"hasQuota"
})
public class ConditionSummaryStructure {
@XmlElement(name = "FareStructureType")
@XmlSchemaType(name = "normalizedString")
protected FareStructureTypeEnumeration fareStructureType;
@XmlElement(name = "TariffBasis")
@XmlSchemaType(name = "normalizedString")
protected TariffBasisEnumeration tariffBasis;
@XmlElement(name = "HasNotices", defaultValue = "false")
protected Boolean hasNotices;
@XmlElement(name = "ProvidesCard", defaultValue = "false")
protected Boolean providesCard;
@XmlElement(name = "GoesOnCard", defaultValue = "false")
protected Boolean goesOnCard;
@XmlElement(name = "IsPersonal", defaultValue = "false")
protected Boolean isPersonal;
@XmlElement(name = "RequiresPhoto", defaultValue = "false")
protected Boolean requiresPhoto;
@XmlElement(name = "MustCarry", defaultValue = "false")
protected Boolean mustCarry;
@XmlElement(name = "RequiresAccount", defaultValue = "false")
protected Boolean requiresAccount;
@XmlElement(name = "IsSupplement", defaultValue = "false")
protected Boolean isSupplement;
@XmlElement(name = "RequiresEntitlement", defaultValue = "false")
protected Boolean requiresEntitlement;
@XmlElement(name = "GivesEntitlement", defaultValue = "false")
protected Boolean givesEntitlement;
@XmlElement(name = "HasOperatorRestrictions", defaultValue = "anyTrain")
@XmlSchemaType(name = "normalizedString")
protected OperatorRestrictionsEnumeration hasOperatorRestrictions;
@XmlElement(name = "HasTravelTimeRestrictions", defaultValue = "false")
protected Boolean hasTravelTimeRestrictions;
@XmlElement(name = "HasRouteRestrictions", defaultValue = "false")
protected Boolean hasRouteRestrictions;
@XmlElement(name = "TrainRestrictions", defaultValue = "anyTrain")
@XmlSchemaType(name = "normalizedString")
protected TrainRestrictionsEnumeration trainRestrictions;
@XmlElement(name = "HasZoneRestrictions", defaultValue = "false")
protected Boolean hasZoneRestrictions;
@XmlElement(name = "CanBreakJourney", defaultValue = "false")
protected Boolean canBreakJourney;
@XmlElement(name = "ReturnTripsOnly", defaultValue = "false")
protected Boolean returnTripsOnly;
@XmlElement(name = "NightTrain", defaultValue = "false")
protected Boolean nightTrain;
@XmlElement(name = "CanChangeClass", defaultValue = "false")
protected Boolean canChangeClass;
@XmlElement(name = "IsRefundable", defaultValue = "true")
protected Boolean isRefundable;
@XmlElement(name = "IsExchangable", defaultValue = "true")
protected Boolean isExchangable;
@XmlElement(name = "HasExchangeFee", defaultValue = "false")
protected Boolean hasExchangeFee;
@XmlElement(name = "HasDiscountedFares", defaultValue = "false")
protected Boolean hasDiscountedFares;
@XmlElement(name = "AllowAdditionalDiscounts", defaultValue = "false")
protected Boolean allowAdditionalDiscounts;
@XmlElement(name = "AllowCompanionDiscounts", defaultValue = "false")
protected Boolean allowCompanionDiscounts;
@XmlElement(name = "HasMinimumPrice", defaultValue = "false")
protected Boolean hasMinimumPrice;
@XmlElement(name = "RequiresPositiveBalance", defaultValue = "true")
protected Boolean requiresPositiveBalance;
@XmlElement(name = "HasPurchaseConditions", defaultValue = "false")
protected Boolean hasPurchaseConditions;
@XmlElement(name = "HasDynamicPricing", defaultValue = "false")
protected Boolean hasDynamicPricing;
@XmlElement(name = "RequiresReservation", defaultValue = "false")
protected Boolean requiresReservation;
@XmlElement(name = "HasReservationFee", defaultValue = "false")
protected Boolean hasReservationFee;
@XmlElement(name = "HasQuota", defaultValue = "false")
protected Boolean hasQuota;
/**
* Gets the value of the fareStructureType property.
*
* @return
* possible object is
* {@link FareStructureTypeEnumeration }
*
*/
public FareStructureTypeEnumeration getFareStructureType() {
return fareStructureType;
}
/**
* Sets the value of the fareStructureType property.
*
* @param value
* allowed object is
* {@link FareStructureTypeEnumeration }
*
*/
public void setFareStructureType(FareStructureTypeEnumeration value) {
this.fareStructureType = value;
}
/**
* Gets the value of the tariffBasis property.
*
* @return
* possible object is
* {@link TariffBasisEnumeration }
*
*/
public TariffBasisEnumeration getTariffBasis() {
return tariffBasis;
}
/**
* Sets the value of the tariffBasis property.
*
* @param value
* allowed object is
* {@link TariffBasisEnumeration }
*
*/
public void setTariffBasis(TariffBasisEnumeration value) {
this.tariffBasis = value;
}
/**
* Gets the value of the hasNotices property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasNotices() {
return hasNotices;
}
/**
* Sets the value of the hasNotices property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasNotices(Boolean value) {
this.hasNotices = value;
}
/**
* Gets the value of the providesCard property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isProvidesCard() {
return providesCard;
}
/**
* Sets the value of the providesCard property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setProvidesCard(Boolean value) {
this.providesCard = value;
}
/**
* Gets the value of the goesOnCard property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isGoesOnCard() {
return goesOnCard;
}
/**
* Sets the value of the goesOnCard property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setGoesOnCard(Boolean value) {
this.goesOnCard = value;
}
/**
* Gets the value of the isPersonal property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsPersonal() {
return isPersonal;
}
/**
* Sets the value of the isPersonal property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsPersonal(Boolean value) {
this.isPersonal = value;
}
/**
* Gets the value of the requiresPhoto property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRequiresPhoto() {
return requiresPhoto;
}
/**
* Sets the value of the requiresPhoto property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRequiresPhoto(Boolean value) {
this.requiresPhoto = value;
}
/**
* Gets the value of the mustCarry property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isMustCarry() {
return mustCarry;
}
/**
* Sets the value of the mustCarry property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setMustCarry(Boolean value) {
this.mustCarry = value;
}
/**
* Gets the value of the requiresAccount property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRequiresAccount() {
return requiresAccount;
}
/**
* Sets the value of the requiresAccount property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRequiresAccount(Boolean value) {
this.requiresAccount = value;
}
/**
* Gets the value of the isSupplement property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsSupplement() {
return isSupplement;
}
/**
* Sets the value of the isSupplement property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsSupplement(Boolean value) {
this.isSupplement = value;
}
/**
* Gets the value of the requiresEntitlement property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRequiresEntitlement() {
return requiresEntitlement;
}
/**
* Sets the value of the requiresEntitlement property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRequiresEntitlement(Boolean value) {
this.requiresEntitlement = value;
}
/**
* Gets the value of the givesEntitlement property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isGivesEntitlement() {
return givesEntitlement;
}
/**
* Sets the value of the givesEntitlement property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setGivesEntitlement(Boolean value) {
this.givesEntitlement = value;
}
/**
* Gets the value of the hasOperatorRestrictions property.
*
* @return
* possible object is
* {@link OperatorRestrictionsEnumeration }
*
*/
public OperatorRestrictionsEnumeration getHasOperatorRestrictions() {
return hasOperatorRestrictions;
}
/**
* Sets the value of the hasOperatorRestrictions property.
*
* @param value
* allowed object is
* {@link OperatorRestrictionsEnumeration }
*
*/
public void setHasOperatorRestrictions(OperatorRestrictionsEnumeration value) {
this.hasOperatorRestrictions = value;
}
/**
* Gets the value of the hasTravelTimeRestrictions property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasTravelTimeRestrictions() {
return hasTravelTimeRestrictions;
}
/**
* Sets the value of the hasTravelTimeRestrictions property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasTravelTimeRestrictions(Boolean value) {
this.hasTravelTimeRestrictions = value;
}
/**
* Gets the value of the hasRouteRestrictions property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasRouteRestrictions() {
return hasRouteRestrictions;
}
/**
* Sets the value of the hasRouteRestrictions property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasRouteRestrictions(Boolean value) {
this.hasRouteRestrictions = value;
}
/**
* Gets the value of the trainRestrictions property.
*
* @return
* possible object is
* {@link TrainRestrictionsEnumeration }
*
*/
public TrainRestrictionsEnumeration getTrainRestrictions() {
return trainRestrictions;
}
/**
* Sets the value of the trainRestrictions property.
*
* @param value
* allowed object is
* {@link TrainRestrictionsEnumeration }
*
*/
public void setTrainRestrictions(TrainRestrictionsEnumeration value) {
this.trainRestrictions = value;
}
/**
* Gets the value of the hasZoneRestrictions property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasZoneRestrictions() {
return hasZoneRestrictions;
}
/**
* Sets the value of the hasZoneRestrictions property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasZoneRestrictions(Boolean value) {
this.hasZoneRestrictions = value;
}
/**
* Gets the value of the canBreakJourney property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCanBreakJourney() {
return canBreakJourney;
}
/**
* Sets the value of the canBreakJourney property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCanBreakJourney(Boolean value) {
this.canBreakJourney = value;
}
/**
* Gets the value of the returnTripsOnly property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isReturnTripsOnly() {
return returnTripsOnly;
}
/**
* Sets the value of the returnTripsOnly property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setReturnTripsOnly(Boolean value) {
this.returnTripsOnly = value;
}
/**
* Gets the value of the nightTrain property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isNightTrain() {
return nightTrain;
}
/**
* Sets the value of the nightTrain property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setNightTrain(Boolean value) {
this.nightTrain = value;
}
/**
* Gets the value of the canChangeClass property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCanChangeClass() {
return canChangeClass;
}
/**
* Sets the value of the canChangeClass property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCanChangeClass(Boolean value) {
this.canChangeClass = value;
}
/**
* Gets the value of the isRefundable property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsRefundable() {
return isRefundable;
}
/**
* Sets the value of the isRefundable property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsRefundable(Boolean value) {
this.isRefundable = value;
}
/**
* Gets the value of the isExchangable property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsExchangable() {
return isExchangable;
}
/**
* Sets the value of the isExchangable property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsExchangable(Boolean value) {
this.isExchangable = value;
}
/**
* Gets the value of the hasExchangeFee property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasExchangeFee() {
return hasExchangeFee;
}
/**
* Sets the value of the hasExchangeFee property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasExchangeFee(Boolean value) {
this.hasExchangeFee = value;
}
/**
* Gets the value of the hasDiscountedFares property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasDiscountedFares() {
return hasDiscountedFares;
}
/**
* Sets the value of the hasDiscountedFares property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasDiscountedFares(Boolean value) {
this.hasDiscountedFares = value;
}
/**
* Gets the value of the allowAdditionalDiscounts property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAllowAdditionalDiscounts() {
return allowAdditionalDiscounts;
}
/**
* Sets the value of the allowAdditionalDiscounts property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setAllowAdditionalDiscounts(Boolean value) {
this.allowAdditionalDiscounts = value;
}
/**
* Gets the value of the allowCompanionDiscounts property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAllowCompanionDiscounts() {
return allowCompanionDiscounts;
}
/**
* Sets the value of the allowCompanionDiscounts property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setAllowCompanionDiscounts(Boolean value) {
this.allowCompanionDiscounts = value;
}
/**
* Gets the value of the hasMinimumPrice property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasMinimumPrice() {
return hasMinimumPrice;
}
/**
* Sets the value of the hasMinimumPrice property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasMinimumPrice(Boolean value) {
this.hasMinimumPrice = value;
}
/**
* Gets the value of the requiresPositiveBalance property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRequiresPositiveBalance() {
return requiresPositiveBalance;
}
/**
* Sets the value of the requiresPositiveBalance property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRequiresPositiveBalance(Boolean value) {
this.requiresPositiveBalance = value;
}
/**
* Gets the value of the hasPurchaseConditions property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasPurchaseConditions() {
return hasPurchaseConditions;
}
/**
* Sets the value of the hasPurchaseConditions property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasPurchaseConditions(Boolean value) {
this.hasPurchaseConditions = value;
}
/**
* Gets the value of the hasDynamicPricing property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasDynamicPricing() {
return hasDynamicPricing;
}
/**
* Sets the value of the hasDynamicPricing property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasDynamicPricing(Boolean value) {
this.hasDynamicPricing = value;
}
/**
* Gets the value of the requiresReservation property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRequiresReservation() {
return requiresReservation;
}
/**
* Sets the value of the requiresReservation property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRequiresReservation(Boolean value) {
this.requiresReservation = value;
}
/**
* Gets the value of the hasReservationFee property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasReservationFee() {
return hasReservationFee;
}
/**
* Sets the value of the hasReservationFee property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasReservationFee(Boolean value) {
this.hasReservationFee = value;
}
/**
* Gets the value of the hasQuota property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasQuota() {
return hasQuota;
}
/**
* Sets the value of the hasQuota property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasQuota(Boolean value) {
this.hasQuota = value;
}
public ConditionSummaryStructure withFareStructureType(FareStructureTypeEnumeration value) {
setFareStructureType(value);
return this;
}
public ConditionSummaryStructure withTariffBasis(TariffBasisEnumeration value) {
setTariffBasis(value);
return this;
}
public ConditionSummaryStructure withHasNotices(Boolean value) {
setHasNotices(value);
return this;
}
public ConditionSummaryStructure withProvidesCard(Boolean value) {
setProvidesCard(value);
return this;
}
public ConditionSummaryStructure withGoesOnCard(Boolean value) {
setGoesOnCard(value);
return this;
}
public ConditionSummaryStructure withIsPersonal(Boolean value) {
setIsPersonal(value);
return this;
}
public ConditionSummaryStructure withRequiresPhoto(Boolean value) {
setRequiresPhoto(value);
return this;
}
public ConditionSummaryStructure withMustCarry(Boolean value) {
setMustCarry(value);
return this;
}
public ConditionSummaryStructure withRequiresAccount(Boolean value) {
setRequiresAccount(value);
return this;
}
public ConditionSummaryStructure withIsSupplement(Boolean value) {
setIsSupplement(value);
return this;
}
public ConditionSummaryStructure withRequiresEntitlement(Boolean value) {
setRequiresEntitlement(value);
return this;
}
public ConditionSummaryStructure withGivesEntitlement(Boolean value) {
setGivesEntitlement(value);
return this;
}
public ConditionSummaryStructure withHasOperatorRestrictions(OperatorRestrictionsEnumeration value) {
setHasOperatorRestrictions(value);
return this;
}
public ConditionSummaryStructure withHasTravelTimeRestrictions(Boolean value) {
setHasTravelTimeRestrictions(value);
return this;
}
public ConditionSummaryStructure withHasRouteRestrictions(Boolean value) {
setHasRouteRestrictions(value);
return this;
}
public ConditionSummaryStructure withTrainRestrictions(TrainRestrictionsEnumeration value) {
setTrainRestrictions(value);
return this;
}
public ConditionSummaryStructure withHasZoneRestrictions(Boolean value) {
setHasZoneRestrictions(value);
return this;
}
public ConditionSummaryStructure withCanBreakJourney(Boolean value) {
setCanBreakJourney(value);
return this;
}
public ConditionSummaryStructure withReturnTripsOnly(Boolean value) {
setReturnTripsOnly(value);
return this;
}
public ConditionSummaryStructure withNightTrain(Boolean value) {
setNightTrain(value);
return this;
}
public ConditionSummaryStructure withCanChangeClass(Boolean value) {
setCanChangeClass(value);
return this;
}
public ConditionSummaryStructure withIsRefundable(Boolean value) {
setIsRefundable(value);
return this;
}
public ConditionSummaryStructure withIsExchangable(Boolean value) {
setIsExchangable(value);
return this;
}
public ConditionSummaryStructure withHasExchangeFee(Boolean value) {
setHasExchangeFee(value);
return this;
}
public ConditionSummaryStructure withHasDiscountedFares(Boolean value) {
setHasDiscountedFares(value);
return this;
}
public ConditionSummaryStructure withAllowAdditionalDiscounts(Boolean value) {
setAllowAdditionalDiscounts(value);
return this;
}
public ConditionSummaryStructure withAllowCompanionDiscounts(Boolean value) {
setAllowCompanionDiscounts(value);
return this;
}
public ConditionSummaryStructure withHasMinimumPrice(Boolean value) {
setHasMinimumPrice(value);
return this;
}
public ConditionSummaryStructure withRequiresPositiveBalance(Boolean value) {
setRequiresPositiveBalance(value);
return this;
}
public ConditionSummaryStructure withHasPurchaseConditions(Boolean value) {
setHasPurchaseConditions(value);
return this;
}
public ConditionSummaryStructure withHasDynamicPricing(Boolean value) {
setHasDynamicPricing(value);
return this;
}
public ConditionSummaryStructure withRequiresReservation(Boolean value) {
setRequiresReservation(value);
return this;
}
public ConditionSummaryStructure withHasReservationFee(Boolean value) {
setHasReservationFee(value);
return this;
}
public ConditionSummaryStructure withHasQuota(Boolean value) {
setHasQuota(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy