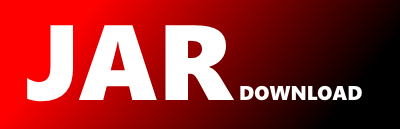
org.rutebanken.netex.model.DepartureStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.math.BigInteger;
import java.time.Duration;
import java.time.OffsetTime;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.migesok.jaxb.adapter.javatime.DurationXmlAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
import org.rutebanken.util.OffsetTimeISO8601XmlAdapter;
/**
* Java class for DepartureStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="DepartureStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Time" type="{http://www.w3.org/2001/XMLSchema}time" minOccurs="0"/>
* <element name="DayOffset" type="{http://www.netex.org.uk/netex}DayOffsetType" minOccurs="0"/>
* <element name="ForBoarding" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="IsFlexible" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="WaitTime" type="{http://www.w3.org/2001/XMLSchema}duration" minOccurs="0"/>
* <group ref="{http://www.netex.org.uk/netex}CallPartGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "DepartureStructure", propOrder = {
"time",
"dayOffset",
"forBoarding",
"isFlexible",
"waitTime",
"journeyPartRef",
"journeyMeetings",
"interchanges",
"interchangeRules",
"timeDemandTypeRef",
"timebandRef",
"dutyPartRef",
"passengerStopAssignmentRef",
"quayAssignmentView",
"dynamicStopAssignment",
"accessibilityAssessment",
"checkConstraint",
"noticeAssignments"
})
public class DepartureStructure {
@XmlElement(name = "Time", type = String.class)
@XmlJavaTypeAdapter(OffsetTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime time;
@XmlElement(name = "DayOffset", defaultValue = "0")
protected BigInteger dayOffset;
@XmlElement(name = "ForBoarding", defaultValue = "true")
protected Boolean forBoarding;
@XmlElement(name = "IsFlexible", defaultValue = "false")
protected Boolean isFlexible;
@XmlElement(name = "WaitTime", type = String.class)
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration waitTime;
@XmlElement(name = "JourneyPartRef")
protected JourneyPartRefStructure journeyPartRef;
protected JourneyMeetingViews_RelStructure journeyMeetings;
protected ServiceJourneyInterchanges_RelStructure interchanges;
protected InterchangeRules_RelStructure interchangeRules;
@XmlElement(name = "TimeDemandTypeRef")
protected TimeDemandTypeRefStructure timeDemandTypeRef;
@XmlElement(name = "TimebandRef")
protected TimebandRefStructure timebandRef;
@XmlElement(name = "DutyPartRef")
protected DutyPartRefStructure dutyPartRef;
@XmlElementRef(name = "PassengerStopAssignmentRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends PassengerStopAssignmentRefStructure> passengerStopAssignmentRef;
@XmlElement(name = "QuayAssignmentView")
protected QuayAssignmentView quayAssignmentView;
@XmlElement(name = "DynamicStopAssignment")
protected DynamicStopAssignment dynamicStopAssignment;
@XmlElement(name = "AccessibilityAssessment")
protected AccessibilityAssessment accessibilityAssessment;
@XmlElement(name = "CheckConstraint")
protected CheckConstraint checkConstraint;
protected NoticeAssignments_RelStructure noticeAssignments;
/**
* Gets the value of the time property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getTime() {
return time;
}
/**
* Sets the value of the time property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTime(OffsetTime value) {
this.time = value;
}
/**
* Gets the value of the dayOffset property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getDayOffset() {
return dayOffset;
}
/**
* Sets the value of the dayOffset property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setDayOffset(BigInteger value) {
this.dayOffset = value;
}
/**
* Gets the value of the forBoarding property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isForBoarding() {
return forBoarding;
}
/**
* Sets the value of the forBoarding property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setForBoarding(Boolean value) {
this.forBoarding = value;
}
/**
* Gets the value of the isFlexible property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsFlexible() {
return isFlexible;
}
/**
* Sets the value of the isFlexible property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsFlexible(Boolean value) {
this.isFlexible = value;
}
/**
* Gets the value of the waitTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getWaitTime() {
return waitTime;
}
/**
* Sets the value of the waitTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWaitTime(Duration value) {
this.waitTime = value;
}
/**
* Gets the value of the journeyPartRef property.
*
* @return
* possible object is
* {@link JourneyPartRefStructure }
*
*/
public JourneyPartRefStructure getJourneyPartRef() {
return journeyPartRef;
}
/**
* Sets the value of the journeyPartRef property.
*
* @param value
* allowed object is
* {@link JourneyPartRefStructure }
*
*/
public void setJourneyPartRef(JourneyPartRefStructure value) {
this.journeyPartRef = value;
}
/**
* Gets the value of the journeyMeetings property.
*
* @return
* possible object is
* {@link JourneyMeetingViews_RelStructure }
*
*/
public JourneyMeetingViews_RelStructure getJourneyMeetings() {
return journeyMeetings;
}
/**
* Sets the value of the journeyMeetings property.
*
* @param value
* allowed object is
* {@link JourneyMeetingViews_RelStructure }
*
*/
public void setJourneyMeetings(JourneyMeetingViews_RelStructure value) {
this.journeyMeetings = value;
}
/**
* Gets the value of the interchanges property.
*
* @return
* possible object is
* {@link ServiceJourneyInterchanges_RelStructure }
*
*/
public ServiceJourneyInterchanges_RelStructure getInterchanges() {
return interchanges;
}
/**
* Sets the value of the interchanges property.
*
* @param value
* allowed object is
* {@link ServiceJourneyInterchanges_RelStructure }
*
*/
public void setInterchanges(ServiceJourneyInterchanges_RelStructure value) {
this.interchanges = value;
}
/**
* Gets the value of the interchangeRules property.
*
* @return
* possible object is
* {@link InterchangeRules_RelStructure }
*
*/
public InterchangeRules_RelStructure getInterchangeRules() {
return interchangeRules;
}
/**
* Sets the value of the interchangeRules property.
*
* @param value
* allowed object is
* {@link InterchangeRules_RelStructure }
*
*/
public void setInterchangeRules(InterchangeRules_RelStructure value) {
this.interchangeRules = value;
}
/**
* Gets the value of the timeDemandTypeRef property.
*
* @return
* possible object is
* {@link TimeDemandTypeRefStructure }
*
*/
public TimeDemandTypeRefStructure getTimeDemandTypeRef() {
return timeDemandTypeRef;
}
/**
* Sets the value of the timeDemandTypeRef property.
*
* @param value
* allowed object is
* {@link TimeDemandTypeRefStructure }
*
*/
public void setTimeDemandTypeRef(TimeDemandTypeRefStructure value) {
this.timeDemandTypeRef = value;
}
/**
* Gets the value of the timebandRef property.
*
* @return
* possible object is
* {@link TimebandRefStructure }
*
*/
public TimebandRefStructure getTimebandRef() {
return timebandRef;
}
/**
* Sets the value of the timebandRef property.
*
* @param value
* allowed object is
* {@link TimebandRefStructure }
*
*/
public void setTimebandRef(TimebandRefStructure value) {
this.timebandRef = value;
}
/**
* Gets the value of the dutyPartRef property.
*
* @return
* possible object is
* {@link DutyPartRefStructure }
*
*/
public DutyPartRefStructure getDutyPartRef() {
return dutyPartRef;
}
/**
* Sets the value of the dutyPartRef property.
*
* @param value
* allowed object is
* {@link DutyPartRefStructure }
*
*/
public void setDutyPartRef(DutyPartRefStructure value) {
this.dutyPartRef = value;
}
/**
* Gets the value of the passengerStopAssignmentRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link DynamicStopAssignmentRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PassengerStopAssignmentRefStructure }{@code >}
*
*/
public JAXBElement extends PassengerStopAssignmentRefStructure> getPassengerStopAssignmentRef() {
return passengerStopAssignmentRef;
}
/**
* Sets the value of the passengerStopAssignmentRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link DynamicStopAssignmentRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PassengerStopAssignmentRefStructure }{@code >}
*
*/
public void setPassengerStopAssignmentRef(JAXBElement extends PassengerStopAssignmentRefStructure> value) {
this.passengerStopAssignmentRef = value;
}
/**
* Gets the value of the quayAssignmentView property.
*
* @return
* possible object is
* {@link QuayAssignmentView }
*
*/
public QuayAssignmentView getQuayAssignmentView() {
return quayAssignmentView;
}
/**
* Sets the value of the quayAssignmentView property.
*
* @param value
* allowed object is
* {@link QuayAssignmentView }
*
*/
public void setQuayAssignmentView(QuayAssignmentView value) {
this.quayAssignmentView = value;
}
/**
* Gets the value of the dynamicStopAssignment property.
*
* @return
* possible object is
* {@link DynamicStopAssignment }
*
*/
public DynamicStopAssignment getDynamicStopAssignment() {
return dynamicStopAssignment;
}
/**
* Sets the value of the dynamicStopAssignment property.
*
* @param value
* allowed object is
* {@link DynamicStopAssignment }
*
*/
public void setDynamicStopAssignment(DynamicStopAssignment value) {
this.dynamicStopAssignment = value;
}
/**
* Gets the value of the accessibilityAssessment property.
*
* @return
* possible object is
* {@link AccessibilityAssessment }
*
*/
public AccessibilityAssessment getAccessibilityAssessment() {
return accessibilityAssessment;
}
/**
* Sets the value of the accessibilityAssessment property.
*
* @param value
* allowed object is
* {@link AccessibilityAssessment }
*
*/
public void setAccessibilityAssessment(AccessibilityAssessment value) {
this.accessibilityAssessment = value;
}
/**
* Gets the value of the checkConstraint property.
*
* @return
* possible object is
* {@link CheckConstraint }
*
*/
public CheckConstraint getCheckConstraint() {
return checkConstraint;
}
/**
* Sets the value of the checkConstraint property.
*
* @param value
* allowed object is
* {@link CheckConstraint }
*
*/
public void setCheckConstraint(CheckConstraint value) {
this.checkConstraint = value;
}
/**
* Gets the value of the noticeAssignments property.
*
* @return
* possible object is
* {@link NoticeAssignments_RelStructure }
*
*/
public NoticeAssignments_RelStructure getNoticeAssignments() {
return noticeAssignments;
}
/**
* Sets the value of the noticeAssignments property.
*
* @param value
* allowed object is
* {@link NoticeAssignments_RelStructure }
*
*/
public void setNoticeAssignments(NoticeAssignments_RelStructure value) {
this.noticeAssignments = value;
}
public DepartureStructure withTime(OffsetTime value) {
setTime(value);
return this;
}
public DepartureStructure withDayOffset(BigInteger value) {
setDayOffset(value);
return this;
}
public DepartureStructure withForBoarding(Boolean value) {
setForBoarding(value);
return this;
}
public DepartureStructure withIsFlexible(Boolean value) {
setIsFlexible(value);
return this;
}
public DepartureStructure withWaitTime(Duration value) {
setWaitTime(value);
return this;
}
public DepartureStructure withJourneyPartRef(JourneyPartRefStructure value) {
setJourneyPartRef(value);
return this;
}
public DepartureStructure withJourneyMeetings(JourneyMeetingViews_RelStructure value) {
setJourneyMeetings(value);
return this;
}
public DepartureStructure withInterchanges(ServiceJourneyInterchanges_RelStructure value) {
setInterchanges(value);
return this;
}
public DepartureStructure withInterchangeRules(InterchangeRules_RelStructure value) {
setInterchangeRules(value);
return this;
}
public DepartureStructure withTimeDemandTypeRef(TimeDemandTypeRefStructure value) {
setTimeDemandTypeRef(value);
return this;
}
public DepartureStructure withTimebandRef(TimebandRefStructure value) {
setTimebandRef(value);
return this;
}
public DepartureStructure withDutyPartRef(DutyPartRefStructure value) {
setDutyPartRef(value);
return this;
}
public DepartureStructure withPassengerStopAssignmentRef(JAXBElement extends PassengerStopAssignmentRefStructure> value) {
setPassengerStopAssignmentRef(value);
return this;
}
public DepartureStructure withQuayAssignmentView(QuayAssignmentView value) {
setQuayAssignmentView(value);
return this;
}
public DepartureStructure withDynamicStopAssignment(DynamicStopAssignment value) {
setDynamicStopAssignment(value);
return this;
}
public DepartureStructure withAccessibilityAssessment(AccessibilityAssessment value) {
setAccessibilityAssessment(value);
return this;
}
public DepartureStructure withCheckConstraint(CheckConstraint value) {
setCheckConstraint(value);
return this;
}
public DepartureStructure withNoticeAssignments(NoticeAssignments_RelStructure value) {
setNoticeAssignments(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy