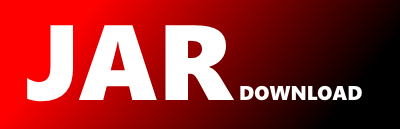
org.rutebanken.netex.model.FarePrice_VersionedChildStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.OffsetDateTime;
import java.util.Collection;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
import org.rutebanken.util.OffsetDateXmlAdapter;
/**
* Java class for FarePrice_VersionedChildStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FarePrice_VersionedChildStructure">
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}VersionedChildStructure">
* <sequence>
* <group ref="{http://www.netex.org.uk/netex}FarePriceGroup"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FarePrice_VersionedChildStructure", propOrder = {
"name",
"startDate",
"endDate",
"amount",
"currency",
"units",
"priceUnitRef",
"ruleStepResults",
"isAllowed",
"pricingServiceRef",
"farePriceRef",
"pricingRuleRef",
"pricingRule_",
"canBeCumulative",
"roundingRef",
"ranking"
})
@XmlSeeAlso({
FarePrice.class,
UsageParameterPrice_VersionedChildStructure.class,
SeriesConstraintPrice_VersionedChildStructure.class,
GeographicalUnitPrice_VersionedChildStructure.class,
GeographicalIntervalPrice_VersionedChildStructure.class,
DistanceMatrixElementPrice_VersionedChildStructure.class,
ValidableElementPrice_VersionedChildStructure.class,
ControllableElementPrice_VersionedChildStructure.class,
FareProductPrice_VersionedChildStructure.class,
CappingRulePrice_VersionedChildStructure.class,
QualityStructureFactorPrice_VersionedChildStructure.class,
TimeUnitPrice_VersionedChildStructure.class,
TimeIntervalPrice_VersionedChildStructure.class,
FareStructureElementPrice_VersionedChildStructure.class,
FulfilmentMethodPrice_VersionedChildStructure.class,
SalesPackagePrice_VersionedChildStructure.class,
ParkingPrice_VersionedChildStructure.class,
CustomerPurchasePackagePrice_VersionedChildStructure.class
})
public class FarePrice_VersionedChildStructure
extends VersionedChildStructure
{
@XmlElement(name = "Name")
protected MultilingualString name;
@XmlElement(name = "StartDate", type = String.class)
@XmlJavaTypeAdapter(OffsetDateXmlAdapter.class)
@XmlSchemaType(name = "date")
protected OffsetDateTime startDate;
@XmlElement(name = "EndDate", type = String.class)
@XmlJavaTypeAdapter(OffsetDateXmlAdapter.class)
@XmlSchemaType(name = "date")
protected OffsetDateTime endDate;
@XmlElement(name = "Amount")
protected BigDecimal amount;
@XmlElement(name = "Currency")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String currency;
@XmlElement(name = "Units")
protected BigDecimal units;
@XmlElement(name = "PriceUnitRef")
protected PriceUnitRefStructure priceUnitRef;
protected PriceRuleStepResults_RelStructure ruleStepResults;
@XmlElement(name = "IsAllowed")
protected Boolean isAllowed;
@XmlElement(name = "PricingServiceRef")
protected PricingServiceRefStructure pricingServiceRef;
@XmlElementRef(name = "FarePriceRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends FarePriceRefStructure> farePriceRef;
@XmlElementRef(name = "PricingRuleRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends PricingRuleRefStructure> pricingRuleRef;
@XmlElementRef(name = "PricingRule_", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends DataManagedObjectStructure> pricingRule_;
@XmlElement(name = "CanBeCumulative", defaultValue = "true")
protected Boolean canBeCumulative;
@XmlElement(name = "RoundingRef")
protected RoundingRefStructure roundingRef;
@XmlElement(name = "Ranking")
protected BigInteger ranking;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link MultilingualString }
*
*/
public MultilingualString getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link MultilingualString }
*
*/
public void setName(MultilingualString value) {
this.name = value;
}
/**
* Gets the value of the startDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getStartDate() {
return startDate;
}
/**
* Sets the value of the startDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStartDate(OffsetDateTime value) {
this.startDate = value;
}
/**
* Gets the value of the endDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getEndDate() {
return endDate;
}
/**
* Sets the value of the endDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEndDate(OffsetDateTime value) {
this.endDate = value;
}
/**
* Gets the value of the amount property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAmount() {
return amount;
}
/**
* Sets the value of the amount property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setAmount(BigDecimal value) {
this.amount = value;
}
/**
* Gets the value of the currency property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCurrency() {
return currency;
}
/**
* Sets the value of the currency property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCurrency(String value) {
this.currency = value;
}
/**
* Gets the value of the units property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getUnits() {
return units;
}
/**
* Sets the value of the units property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setUnits(BigDecimal value) {
this.units = value;
}
/**
* Gets the value of the priceUnitRef property.
*
* @return
* possible object is
* {@link PriceUnitRefStructure }
*
*/
public PriceUnitRefStructure getPriceUnitRef() {
return priceUnitRef;
}
/**
* Sets the value of the priceUnitRef property.
*
* @param value
* allowed object is
* {@link PriceUnitRefStructure }
*
*/
public void setPriceUnitRef(PriceUnitRefStructure value) {
this.priceUnitRef = value;
}
/**
* Gets the value of the ruleStepResults property.
*
* @return
* possible object is
* {@link PriceRuleStepResults_RelStructure }
*
*/
public PriceRuleStepResults_RelStructure getRuleStepResults() {
return ruleStepResults;
}
/**
* Sets the value of the ruleStepResults property.
*
* @param value
* allowed object is
* {@link PriceRuleStepResults_RelStructure }
*
*/
public void setRuleStepResults(PriceRuleStepResults_RelStructure value) {
this.ruleStepResults = value;
}
/**
* Gets the value of the isAllowed property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsAllowed() {
return isAllowed;
}
/**
* Sets the value of the isAllowed property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsAllowed(Boolean value) {
this.isAllowed = value;
}
/**
* Gets the value of the pricingServiceRef property.
*
* @return
* possible object is
* {@link PricingServiceRefStructure }
*
*/
public PricingServiceRefStructure getPricingServiceRef() {
return pricingServiceRef;
}
/**
* Sets the value of the pricingServiceRef property.
*
* @param value
* allowed object is
* {@link PricingServiceRefStructure }
*
*/
public void setPricingServiceRef(PricingServiceRefStructure value) {
this.pricingServiceRef = value;
}
/**
* Gets the value of the farePriceRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link QualityStructureFactorPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ValidableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SalesPackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CustomerPurchasePackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareProductPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FarePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ControllableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageParameterPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FulfilmentMethodPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareStructureElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DistanceMatrixElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SeriesConstraintPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CappingRulePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ParkingPriceRefStructure }{@code >}
*
*/
public JAXBElement extends FarePriceRefStructure> getFarePriceRef() {
return farePriceRef;
}
/**
* Sets the value of the farePriceRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link QualityStructureFactorPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ValidableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SalesPackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CustomerPurchasePackagePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareProductPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FarePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ControllableElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimeIntervalPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link UsageParameterPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeographicalUnitPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FulfilmentMethodPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareStructureElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DistanceMatrixElementPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SeriesConstraintPriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CappingRulePriceRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ParkingPriceRefStructure }{@code >}
*
*/
public void setFarePriceRef(JAXBElement extends FarePriceRefStructure> value) {
this.farePriceRef = value;
}
/**
* Gets the value of the pricingRuleRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link LimitingRuleRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DiscountingRuleRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PricingRuleRefStructure }{@code >}
*
*/
public JAXBElement extends PricingRuleRefStructure> getPricingRuleRef() {
return pricingRuleRef;
}
/**
* Sets the value of the pricingRuleRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link LimitingRuleRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DiscountingRuleRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link PricingRuleRefStructure }{@code >}
*
*/
public void setPricingRuleRef(JAXBElement extends PricingRuleRefStructure> value) {
this.pricingRuleRef = value;
}
/**
* Gets the value of the pricingRule_ property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link DiscountingRule }{@code >}
* {@link JAXBElement }{@code <}{@link LimitingRule }{@code >}
* {@link JAXBElement }{@code <}{@link PricingRule }{@code >}
* {@link JAXBElement }{@code <}{@link DataManagedObjectStructure }{@code >}
* {@link JAXBElement }{@code <}{@link LimitingRuleInContext }{@code >}
*
*/
public JAXBElement extends DataManagedObjectStructure> getPricingRule_() {
return pricingRule_;
}
/**
* Sets the value of the pricingRule_ property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link DiscountingRule }{@code >}
* {@link JAXBElement }{@code <}{@link LimitingRule }{@code >}
* {@link JAXBElement }{@code <}{@link PricingRule }{@code >}
* {@link JAXBElement }{@code <}{@link DataManagedObjectStructure }{@code >}
* {@link JAXBElement }{@code <}{@link LimitingRuleInContext }{@code >}
*
*/
public void setPricingRule_(JAXBElement extends DataManagedObjectStructure> value) {
this.pricingRule_ = value;
}
/**
* Gets the value of the canBeCumulative property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCanBeCumulative() {
return canBeCumulative;
}
/**
* Sets the value of the canBeCumulative property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCanBeCumulative(Boolean value) {
this.canBeCumulative = value;
}
/**
* Gets the value of the roundingRef property.
*
* @return
* possible object is
* {@link RoundingRefStructure }
*
*/
public RoundingRefStructure getRoundingRef() {
return roundingRef;
}
/**
* Sets the value of the roundingRef property.
*
* @param value
* allowed object is
* {@link RoundingRefStructure }
*
*/
public void setRoundingRef(RoundingRefStructure value) {
this.roundingRef = value;
}
/**
* Gets the value of the ranking property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getRanking() {
return ranking;
}
/**
* Sets the value of the ranking property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setRanking(BigInteger value) {
this.ranking = value;
}
public FarePrice_VersionedChildStructure withName(MultilingualString value) {
setName(value);
return this;
}
public FarePrice_VersionedChildStructure withStartDate(OffsetDateTime value) {
setStartDate(value);
return this;
}
public FarePrice_VersionedChildStructure withEndDate(OffsetDateTime value) {
setEndDate(value);
return this;
}
public FarePrice_VersionedChildStructure withAmount(BigDecimal value) {
setAmount(value);
return this;
}
public FarePrice_VersionedChildStructure withCurrency(String value) {
setCurrency(value);
return this;
}
public FarePrice_VersionedChildStructure withUnits(BigDecimal value) {
setUnits(value);
return this;
}
public FarePrice_VersionedChildStructure withPriceUnitRef(PriceUnitRefStructure value) {
setPriceUnitRef(value);
return this;
}
public FarePrice_VersionedChildStructure withRuleStepResults(PriceRuleStepResults_RelStructure value) {
setRuleStepResults(value);
return this;
}
public FarePrice_VersionedChildStructure withIsAllowed(Boolean value) {
setIsAllowed(value);
return this;
}
public FarePrice_VersionedChildStructure withPricingServiceRef(PricingServiceRefStructure value) {
setPricingServiceRef(value);
return this;
}
public FarePrice_VersionedChildStructure withFarePriceRef(JAXBElement extends FarePriceRefStructure> value) {
setFarePriceRef(value);
return this;
}
public FarePrice_VersionedChildStructure withPricingRuleRef(JAXBElement extends PricingRuleRefStructure> value) {
setPricingRuleRef(value);
return this;
}
public FarePrice_VersionedChildStructure withPricingRule_(JAXBElement extends DataManagedObjectStructure> value) {
setPricingRule_(value);
return this;
}
public FarePrice_VersionedChildStructure withCanBeCumulative(Boolean value) {
setCanBeCumulative(value);
return this;
}
public FarePrice_VersionedChildStructure withRoundingRef(RoundingRefStructure value) {
setRoundingRef(value);
return this;
}
public FarePrice_VersionedChildStructure withRanking(BigInteger value) {
setRanking(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withExtensions(ExtensionsStructure value) {
setExtensions(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withValidityConditions(ValidityConditions_RelStructure value) {
setValidityConditions(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withValidBetween(ValidBetween... values) {
if (values!= null) {
for (ValidBetween value: values) {
getValidBetween().add(value);
}
}
return this;
}
@Override
public FarePrice_VersionedChildStructure withValidBetween(Collection values) {
if (values!= null) {
getValidBetween().addAll(values);
}
return this;
}
@Override
public FarePrice_VersionedChildStructure withDataSourceRef(String value) {
setDataSourceRef(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withCreated(OffsetDateTime value) {
setCreated(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withChanged(OffsetDateTime value) {
setChanged(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withModification(ModificationEnumeration value) {
setModification(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withVersion(String value) {
setVersion(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withStatus_BasicModificationDetailsGroup(StatusEnumeration value) {
setStatus_BasicModificationDetailsGroup(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withDerivedFromVersionRef_BasicModificationDetailsGroup(String value) {
setDerivedFromVersionRef_BasicModificationDetailsGroup(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withCompatibleWithVersionFrameVersionRef(String value) {
setCompatibleWithVersionFrameVersionRef(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withDerivedFromObjectRef(String value) {
setDerivedFromObjectRef(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withNameOfClass(String value) {
setNameOfClass(value);
return this;
}
@Override
public FarePrice_VersionedChildStructure withId(String value) {
setId(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy