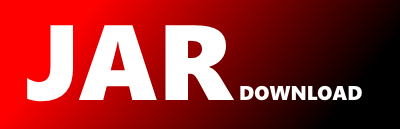
org.rutebanken.netex.model.Infrastructure_VersionFrameStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.time.OffsetDateTime;
import java.util.Collection;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
/**
* Java class for Infrastructure_VersionFrameStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Infrastructure_VersionFrameStructure">
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}Common_VersionFrameStructure">
* <sequence>
* <group ref="{http://www.netex.org.uk/netex}InfrastructureFrameGroup"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Infrastructure_VersionFrameStructure", propOrder = {
"meetingsRestricted",
"restrictedManoeuvres",
"overtakingPossibilitiesRestricted",
"spatialFeatures",
"junctions",
"elements",
"restrictions",
"crewBases",
"garages",
"vehicleAndCrewPoints",
"trafficControlPoints",
"activationPoints",
"activationLinks",
"activatedEquipments",
"vehicleTypes",
"vehicleModels",
"vehicleEquipmentProfiles",
"vehicles"
})
@XmlSeeAlso({
InfrastructureFrame.class
})
public class Infrastructure_VersionFrameStructure
extends Common_VersionFrameStructure
{
@XmlElement(name = "MeetingsRestricted", defaultValue = "false")
protected Boolean meetingsRestricted;
@XmlElement(name = "RestrictedManoeuvres", defaultValue = "false")
protected Boolean restrictedManoeuvres;
@XmlElement(name = "OvertakingPossibilitiesRestricted", defaultValue = "false")
protected Boolean overtakingPossibilitiesRestricted;
protected SpatialFeaturesInFrame_RelStructure spatialFeatures;
protected InfrastructureJunctionsInFrame_RelStructure junctions;
protected InfrastructureElementsInFrame_RelStructure elements;
protected NetworkRestrictionsInFrame_RelStructure restrictions;
protected CrewBasesInFrame_RelStructure crewBases;
protected GaragesInFrame_RelStructure garages;
protected ReliefPointsInFrame_RelStructure vehicleAndCrewPoints;
protected TrafficControlPointsInFrame_RelStructure trafficControlPoints;
protected ActivationPointsInFrame_RelStructure activationPoints;
protected ActivationLinksInFrame_RelStructure activationLinks;
protected ActivatedEquipmentsInFrame_RelStructure activatedEquipments;
protected VehicleTypesInFrame_RelStructure vehicleTypes;
protected VehicleModelsInFrame_RelStructure vehicleModels;
protected VehicleEquipmenProfilesInFrame_RelStructure vehicleEquipmentProfiles;
protected VehiclesInFrame_RelStructure vehicles;
/**
* Gets the value of the meetingsRestricted property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isMeetingsRestricted() {
return meetingsRestricted;
}
/**
* Sets the value of the meetingsRestricted property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setMeetingsRestricted(Boolean value) {
this.meetingsRestricted = value;
}
/**
* Gets the value of the restrictedManoeuvres property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRestrictedManoeuvres() {
return restrictedManoeuvres;
}
/**
* Sets the value of the restrictedManoeuvres property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRestrictedManoeuvres(Boolean value) {
this.restrictedManoeuvres = value;
}
/**
* Gets the value of the overtakingPossibilitiesRestricted property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isOvertakingPossibilitiesRestricted() {
return overtakingPossibilitiesRestricted;
}
/**
* Sets the value of the overtakingPossibilitiesRestricted property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setOvertakingPossibilitiesRestricted(Boolean value) {
this.overtakingPossibilitiesRestricted = value;
}
/**
* Gets the value of the spatialFeatures property.
*
* @return
* possible object is
* {@link SpatialFeaturesInFrame_RelStructure }
*
*/
public SpatialFeaturesInFrame_RelStructure getSpatialFeatures() {
return spatialFeatures;
}
/**
* Sets the value of the spatialFeatures property.
*
* @param value
* allowed object is
* {@link SpatialFeaturesInFrame_RelStructure }
*
*/
public void setSpatialFeatures(SpatialFeaturesInFrame_RelStructure value) {
this.spatialFeatures = value;
}
/**
* Gets the value of the junctions property.
*
* @return
* possible object is
* {@link InfrastructureJunctionsInFrame_RelStructure }
*
*/
public InfrastructureJunctionsInFrame_RelStructure getJunctions() {
return junctions;
}
/**
* Sets the value of the junctions property.
*
* @param value
* allowed object is
* {@link InfrastructureJunctionsInFrame_RelStructure }
*
*/
public void setJunctions(InfrastructureJunctionsInFrame_RelStructure value) {
this.junctions = value;
}
/**
* Gets the value of the elements property.
*
* @return
* possible object is
* {@link InfrastructureElementsInFrame_RelStructure }
*
*/
public InfrastructureElementsInFrame_RelStructure getElements() {
return elements;
}
/**
* Sets the value of the elements property.
*
* @param value
* allowed object is
* {@link InfrastructureElementsInFrame_RelStructure }
*
*/
public void setElements(InfrastructureElementsInFrame_RelStructure value) {
this.elements = value;
}
/**
* Gets the value of the restrictions property.
*
* @return
* possible object is
* {@link NetworkRestrictionsInFrame_RelStructure }
*
*/
public NetworkRestrictionsInFrame_RelStructure getRestrictions() {
return restrictions;
}
/**
* Sets the value of the restrictions property.
*
* @param value
* allowed object is
* {@link NetworkRestrictionsInFrame_RelStructure }
*
*/
public void setRestrictions(NetworkRestrictionsInFrame_RelStructure value) {
this.restrictions = value;
}
/**
* Gets the value of the crewBases property.
*
* @return
* possible object is
* {@link CrewBasesInFrame_RelStructure }
*
*/
public CrewBasesInFrame_RelStructure getCrewBases() {
return crewBases;
}
/**
* Sets the value of the crewBases property.
*
* @param value
* allowed object is
* {@link CrewBasesInFrame_RelStructure }
*
*/
public void setCrewBases(CrewBasesInFrame_RelStructure value) {
this.crewBases = value;
}
/**
* Gets the value of the garages property.
*
* @return
* possible object is
* {@link GaragesInFrame_RelStructure }
*
*/
public GaragesInFrame_RelStructure getGarages() {
return garages;
}
/**
* Sets the value of the garages property.
*
* @param value
* allowed object is
* {@link GaragesInFrame_RelStructure }
*
*/
public void setGarages(GaragesInFrame_RelStructure value) {
this.garages = value;
}
/**
* Gets the value of the vehicleAndCrewPoints property.
*
* @return
* possible object is
* {@link ReliefPointsInFrame_RelStructure }
*
*/
public ReliefPointsInFrame_RelStructure getVehicleAndCrewPoints() {
return vehicleAndCrewPoints;
}
/**
* Sets the value of the vehicleAndCrewPoints property.
*
* @param value
* allowed object is
* {@link ReliefPointsInFrame_RelStructure }
*
*/
public void setVehicleAndCrewPoints(ReliefPointsInFrame_RelStructure value) {
this.vehicleAndCrewPoints = value;
}
/**
* Gets the value of the trafficControlPoints property.
*
* @return
* possible object is
* {@link TrafficControlPointsInFrame_RelStructure }
*
*/
public TrafficControlPointsInFrame_RelStructure getTrafficControlPoints() {
return trafficControlPoints;
}
/**
* Sets the value of the trafficControlPoints property.
*
* @param value
* allowed object is
* {@link TrafficControlPointsInFrame_RelStructure }
*
*/
public void setTrafficControlPoints(TrafficControlPointsInFrame_RelStructure value) {
this.trafficControlPoints = value;
}
/**
* Gets the value of the activationPoints property.
*
* @return
* possible object is
* {@link ActivationPointsInFrame_RelStructure }
*
*/
public ActivationPointsInFrame_RelStructure getActivationPoints() {
return activationPoints;
}
/**
* Sets the value of the activationPoints property.
*
* @param value
* allowed object is
* {@link ActivationPointsInFrame_RelStructure }
*
*/
public void setActivationPoints(ActivationPointsInFrame_RelStructure value) {
this.activationPoints = value;
}
/**
* Gets the value of the activationLinks property.
*
* @return
* possible object is
* {@link ActivationLinksInFrame_RelStructure }
*
*/
public ActivationLinksInFrame_RelStructure getActivationLinks() {
return activationLinks;
}
/**
* Sets the value of the activationLinks property.
*
* @param value
* allowed object is
* {@link ActivationLinksInFrame_RelStructure }
*
*/
public void setActivationLinks(ActivationLinksInFrame_RelStructure value) {
this.activationLinks = value;
}
/**
* Gets the value of the activatedEquipments property.
*
* @return
* possible object is
* {@link ActivatedEquipmentsInFrame_RelStructure }
*
*/
public ActivatedEquipmentsInFrame_RelStructure getActivatedEquipments() {
return activatedEquipments;
}
/**
* Sets the value of the activatedEquipments property.
*
* @param value
* allowed object is
* {@link ActivatedEquipmentsInFrame_RelStructure }
*
*/
public void setActivatedEquipments(ActivatedEquipmentsInFrame_RelStructure value) {
this.activatedEquipments = value;
}
/**
* Gets the value of the vehicleTypes property.
*
* @return
* possible object is
* {@link VehicleTypesInFrame_RelStructure }
*
*/
public VehicleTypesInFrame_RelStructure getVehicleTypes() {
return vehicleTypes;
}
/**
* Sets the value of the vehicleTypes property.
*
* @param value
* allowed object is
* {@link VehicleTypesInFrame_RelStructure }
*
*/
public void setVehicleTypes(VehicleTypesInFrame_RelStructure value) {
this.vehicleTypes = value;
}
/**
* Gets the value of the vehicleModels property.
*
* @return
* possible object is
* {@link VehicleModelsInFrame_RelStructure }
*
*/
public VehicleModelsInFrame_RelStructure getVehicleModels() {
return vehicleModels;
}
/**
* Sets the value of the vehicleModels property.
*
* @param value
* allowed object is
* {@link VehicleModelsInFrame_RelStructure }
*
*/
public void setVehicleModels(VehicleModelsInFrame_RelStructure value) {
this.vehicleModels = value;
}
/**
* Gets the value of the vehicleEquipmentProfiles property.
*
* @return
* possible object is
* {@link VehicleEquipmenProfilesInFrame_RelStructure }
*
*/
public VehicleEquipmenProfilesInFrame_RelStructure getVehicleEquipmentProfiles() {
return vehicleEquipmentProfiles;
}
/**
* Sets the value of the vehicleEquipmentProfiles property.
*
* @param value
* allowed object is
* {@link VehicleEquipmenProfilesInFrame_RelStructure }
*
*/
public void setVehicleEquipmentProfiles(VehicleEquipmenProfilesInFrame_RelStructure value) {
this.vehicleEquipmentProfiles = value;
}
/**
* Gets the value of the vehicles property.
*
* @return
* possible object is
* {@link VehiclesInFrame_RelStructure }
*
*/
public VehiclesInFrame_RelStructure getVehicles() {
return vehicles;
}
/**
* Sets the value of the vehicles property.
*
* @param value
* allowed object is
* {@link VehiclesInFrame_RelStructure }
*
*/
public void setVehicles(VehiclesInFrame_RelStructure value) {
this.vehicles = value;
}
public Infrastructure_VersionFrameStructure withMeetingsRestricted(Boolean value) {
setMeetingsRestricted(value);
return this;
}
public Infrastructure_VersionFrameStructure withRestrictedManoeuvres(Boolean value) {
setRestrictedManoeuvres(value);
return this;
}
public Infrastructure_VersionFrameStructure withOvertakingPossibilitiesRestricted(Boolean value) {
setOvertakingPossibilitiesRestricted(value);
return this;
}
public Infrastructure_VersionFrameStructure withSpatialFeatures(SpatialFeaturesInFrame_RelStructure value) {
setSpatialFeatures(value);
return this;
}
public Infrastructure_VersionFrameStructure withJunctions(InfrastructureJunctionsInFrame_RelStructure value) {
setJunctions(value);
return this;
}
public Infrastructure_VersionFrameStructure withElements(InfrastructureElementsInFrame_RelStructure value) {
setElements(value);
return this;
}
public Infrastructure_VersionFrameStructure withRestrictions(NetworkRestrictionsInFrame_RelStructure value) {
setRestrictions(value);
return this;
}
public Infrastructure_VersionFrameStructure withCrewBases(CrewBasesInFrame_RelStructure value) {
setCrewBases(value);
return this;
}
public Infrastructure_VersionFrameStructure withGarages(GaragesInFrame_RelStructure value) {
setGarages(value);
return this;
}
public Infrastructure_VersionFrameStructure withVehicleAndCrewPoints(ReliefPointsInFrame_RelStructure value) {
setVehicleAndCrewPoints(value);
return this;
}
public Infrastructure_VersionFrameStructure withTrafficControlPoints(TrafficControlPointsInFrame_RelStructure value) {
setTrafficControlPoints(value);
return this;
}
public Infrastructure_VersionFrameStructure withActivationPoints(ActivationPointsInFrame_RelStructure value) {
setActivationPoints(value);
return this;
}
public Infrastructure_VersionFrameStructure withActivationLinks(ActivationLinksInFrame_RelStructure value) {
setActivationLinks(value);
return this;
}
public Infrastructure_VersionFrameStructure withActivatedEquipments(ActivatedEquipmentsInFrame_RelStructure value) {
setActivatedEquipments(value);
return this;
}
public Infrastructure_VersionFrameStructure withVehicleTypes(VehicleTypesInFrame_RelStructure value) {
setVehicleTypes(value);
return this;
}
public Infrastructure_VersionFrameStructure withVehicleModels(VehicleModelsInFrame_RelStructure value) {
setVehicleModels(value);
return this;
}
public Infrastructure_VersionFrameStructure withVehicleEquipmentProfiles(VehicleEquipmenProfilesInFrame_RelStructure value) {
setVehicleEquipmentProfiles(value);
return this;
}
public Infrastructure_VersionFrameStructure withVehicles(VehiclesInFrame_RelStructure value) {
setVehicles(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withName(MultilingualString value) {
setName(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withDescription(MultilingualString value) {
setDescription(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withTypeOfFrameRef(TypeOfFrameRefStructure value) {
setTypeOfFrameRef(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withBaselineVersionFrameRef(VersionRefStructure value) {
setBaselineVersionFrameRef(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withCodespaces(Codespaces_RelStructure value) {
setCodespaces(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withFrameDefaults(VersionFrameDefaultsStructure value) {
setFrameDefaults(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withVersions(Versions_RelStructure value) {
setVersions(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withTraces(Traces_RelStructure value) {
setTraces(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withContentValidityConditions(ValidityConditions_RelStructure value) {
setContentValidityConditions(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withKeyList(KeyListStructure value) {
setKeyList(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withExtensions(ExtensionsStructure value) {
setExtensions(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withBrandingRef(BrandingRefStructure value) {
setBrandingRef(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withAlternativeTexts(AlternativeTexts_RelStructure value) {
setAlternativeTexts(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withResponsibilitySetRef(String value) {
setResponsibilitySetRef(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withValidityConditions(ValidityConditions_RelStructure value) {
setValidityConditions(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withValidBetween(ValidBetween... values) {
if (values!= null) {
for (ValidBetween value: values) {
getValidBetween().add(value);
}
}
return this;
}
@Override
public Infrastructure_VersionFrameStructure withValidBetween(Collection values) {
if (values!= null) {
getValidBetween().addAll(values);
}
return this;
}
@Override
public Infrastructure_VersionFrameStructure withDataSourceRef(String value) {
setDataSourceRef(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withCreated(OffsetDateTime value) {
setCreated(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withChanged(OffsetDateTime value) {
setChanged(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withModification(ModificationEnumeration value) {
setModification(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withVersion(String value) {
setVersion(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withStatus_BasicModificationDetailsGroup(StatusEnumeration value) {
setStatus_BasicModificationDetailsGroup(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withDerivedFromVersionRef_BasicModificationDetailsGroup(String value) {
setDerivedFromVersionRef_BasicModificationDetailsGroup(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withCompatibleWithVersionFrameVersionRef(String value) {
setCompatibleWithVersionFrameVersionRef(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withDerivedFromObjectRef(String value) {
setDerivedFromObjectRef(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withNameOfClass(String value) {
setNameOfClass(value);
return this;
}
@Override
public Infrastructure_VersionFrameStructure withId(String value) {
setId(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy