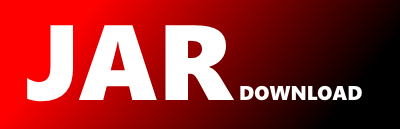
org.rutebanken.netex.model.JourneyPartCouple_VersionStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.math.BigInteger;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.util.Collection;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
import org.rutebanken.util.OffsetTimeISO8601XmlAdapter;
/**
* Java class for JourneyPartCouple_VersionStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="JourneyPartCouple_VersionStructure">
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}DataManagedObjectStructure">
* <sequence>
* <group ref="{http://www.netex.org.uk/netex}JourneyPartCoupleGroup"/>
* </sequence>
* <attribute name="order" use="required" type="{http://www.w3.org/2001/XMLSchema}positiveInteger" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "JourneyPartCouple_VersionStructure", propOrder = {
"description",
"startTime",
"endTime",
"fromStopPointRef",
"toStopPointRef",
"mainPartRef",
"journeyParts",
"trainNumberRef"
})
@XmlSeeAlso({
JourneyPartCouple.class
})
public class JourneyPartCouple_VersionStructure
extends DataManagedObjectStructure
{
@XmlElement(name = "Description")
protected MultilingualString description;
@XmlElement(name = "StartTime", required = true, type = String.class)
@XmlJavaTypeAdapter(OffsetTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime startTime;
@XmlElement(name = "EndTime", required = true, type = String.class)
@XmlJavaTypeAdapter(OffsetTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime endTime;
@XmlElement(name = "FromStopPointRef", required = true)
protected ScheduledStopPointRefStructure fromStopPointRef;
@XmlElement(name = "ToStopPointRef", required = true)
protected ScheduledStopPointRefStructure toStopPointRef;
@XmlElement(name = "MainPartRef", required = true)
protected JourneyPartRefStructure mainPartRef;
protected JourneyPartRefs_RelStructure journeyParts;
@XmlElement(name = "TrainNumberRef")
protected TrainNumberRefStructure trainNumberRef;
@XmlAttribute(name = "order", required = true)
@XmlSchemaType(name = "positiveInteger")
protected BigInteger order;
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link MultilingualString }
*
*/
public MultilingualString getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link MultilingualString }
*
*/
public void setDescription(MultilingualString value) {
this.description = value;
}
/**
* Gets the value of the startTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getStartTime() {
return startTime;
}
/**
* Sets the value of the startTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStartTime(OffsetTime value) {
this.startTime = value;
}
/**
* Gets the value of the endTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getEndTime() {
return endTime;
}
/**
* Sets the value of the endTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEndTime(OffsetTime value) {
this.endTime = value;
}
/**
* Gets the value of the fromStopPointRef property.
*
* @return
* possible object is
* {@link ScheduledStopPointRefStructure }
*
*/
public ScheduledStopPointRefStructure getFromStopPointRef() {
return fromStopPointRef;
}
/**
* Sets the value of the fromStopPointRef property.
*
* @param value
* allowed object is
* {@link ScheduledStopPointRefStructure }
*
*/
public void setFromStopPointRef(ScheduledStopPointRefStructure value) {
this.fromStopPointRef = value;
}
/**
* Gets the value of the toStopPointRef property.
*
* @return
* possible object is
* {@link ScheduledStopPointRefStructure }
*
*/
public ScheduledStopPointRefStructure getToStopPointRef() {
return toStopPointRef;
}
/**
* Sets the value of the toStopPointRef property.
*
* @param value
* allowed object is
* {@link ScheduledStopPointRefStructure }
*
*/
public void setToStopPointRef(ScheduledStopPointRefStructure value) {
this.toStopPointRef = value;
}
/**
* Gets the value of the mainPartRef property.
*
* @return
* possible object is
* {@link JourneyPartRefStructure }
*
*/
public JourneyPartRefStructure getMainPartRef() {
return mainPartRef;
}
/**
* Sets the value of the mainPartRef property.
*
* @param value
* allowed object is
* {@link JourneyPartRefStructure }
*
*/
public void setMainPartRef(JourneyPartRefStructure value) {
this.mainPartRef = value;
}
/**
* Gets the value of the journeyParts property.
*
* @return
* possible object is
* {@link JourneyPartRefs_RelStructure }
*
*/
public JourneyPartRefs_RelStructure getJourneyParts() {
return journeyParts;
}
/**
* Sets the value of the journeyParts property.
*
* @param value
* allowed object is
* {@link JourneyPartRefs_RelStructure }
*
*/
public void setJourneyParts(JourneyPartRefs_RelStructure value) {
this.journeyParts = value;
}
/**
* Gets the value of the trainNumberRef property.
*
* @return
* possible object is
* {@link TrainNumberRefStructure }
*
*/
public TrainNumberRefStructure getTrainNumberRef() {
return trainNumberRef;
}
/**
* Sets the value of the trainNumberRef property.
*
* @param value
* allowed object is
* {@link TrainNumberRefStructure }
*
*/
public void setTrainNumberRef(TrainNumberRefStructure value) {
this.trainNumberRef = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setOrder(BigInteger value) {
this.order = value;
}
public JourneyPartCouple_VersionStructure withDescription(MultilingualString value) {
setDescription(value);
return this;
}
public JourneyPartCouple_VersionStructure withStartTime(OffsetTime value) {
setStartTime(value);
return this;
}
public JourneyPartCouple_VersionStructure withEndTime(OffsetTime value) {
setEndTime(value);
return this;
}
public JourneyPartCouple_VersionStructure withFromStopPointRef(ScheduledStopPointRefStructure value) {
setFromStopPointRef(value);
return this;
}
public JourneyPartCouple_VersionStructure withToStopPointRef(ScheduledStopPointRefStructure value) {
setToStopPointRef(value);
return this;
}
public JourneyPartCouple_VersionStructure withMainPartRef(JourneyPartRefStructure value) {
setMainPartRef(value);
return this;
}
public JourneyPartCouple_VersionStructure withJourneyParts(JourneyPartRefs_RelStructure value) {
setJourneyParts(value);
return this;
}
public JourneyPartCouple_VersionStructure withTrainNumberRef(TrainNumberRefStructure value) {
setTrainNumberRef(value);
return this;
}
public JourneyPartCouple_VersionStructure withOrder(BigInteger value) {
setOrder(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withKeyList(KeyListStructure value) {
setKeyList(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withExtensions(ExtensionsStructure value) {
setExtensions(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withBrandingRef(BrandingRefStructure value) {
setBrandingRef(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withAlternativeTexts(AlternativeTexts_RelStructure value) {
setAlternativeTexts(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withResponsibilitySetRef(String value) {
setResponsibilitySetRef(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withValidityConditions(ValidityConditions_RelStructure value) {
setValidityConditions(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withValidBetween(ValidBetween... values) {
if (values!= null) {
for (ValidBetween value: values) {
getValidBetween().add(value);
}
}
return this;
}
@Override
public JourneyPartCouple_VersionStructure withValidBetween(Collection values) {
if (values!= null) {
getValidBetween().addAll(values);
}
return this;
}
@Override
public JourneyPartCouple_VersionStructure withDataSourceRef(String value) {
setDataSourceRef(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withCreated(OffsetDateTime value) {
setCreated(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withChanged(OffsetDateTime value) {
setChanged(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withModification(ModificationEnumeration value) {
setModification(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withVersion(String value) {
setVersion(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withStatus_BasicModificationDetailsGroup(StatusEnumeration value) {
setStatus_BasicModificationDetailsGroup(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withDerivedFromVersionRef_BasicModificationDetailsGroup(String value) {
setDerivedFromVersionRef_BasicModificationDetailsGroup(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withCompatibleWithVersionFrameVersionRef(String value) {
setCompatibleWithVersionFrameVersionRef(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withDerivedFromObjectRef(String value) {
setDerivedFromObjectRef(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withNameOfClass(String value) {
setNameOfClass(value);
return this;
}
@Override
public JourneyPartCouple_VersionStructure withId(String value) {
setId(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy