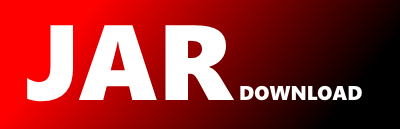
org.rutebanken.netex.model.NetworkFrameTopicStructure Maven / Gradle / Ivy
Show all versions of netex-java-model Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
import org.rutebanken.util.OffsetDateTimeISO8601XmlAdapter;
/**
* Java class for NetworkFrameTopicStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="NetworkFrameTopicStructure">
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}TopicStructure">
* <sequence>
* <group ref="{http://www.netex.org.uk/netex}TopicTemporalScopeGroup"/>
* <element ref="{http://www.netex.org.uk/netex}TypeOfFrameRef" minOccurs="0"/>
* <group ref="{http://www.netex.org.uk/netex}TopicFrameScopeGroup" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "NetworkFrameTopicStructure", propOrder = {
"current",
"changedSince",
"currentAt",
"historicBetween",
"selectionValidityConditions",
"typeOfFrameRef",
"versionFrameRef",
"networkFilterByValue"
})
@XmlSeeAlso({
NetworkFrameTopic.class
})
public class NetworkFrameTopicStructure
extends TopicStructure
{
@XmlElement(name = "Current")
protected String current;
@XmlElement(name = "ChangedSince", type = String.class)
@XmlJavaTypeAdapter(OffsetDateTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime changedSince;
@XmlElement(name = "CurrentAt", type = String.class)
@XmlJavaTypeAdapter(OffsetDateTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime currentAt;
@XmlElement(name = "HistoricBetween")
protected ClosedTimestampRangeStructure historicBetween;
protected NetworkFrameTopicStructure.SelectionValidityConditions selectionValidityConditions;
@XmlElement(name = "TypeOfFrameRef")
protected TypeOfFrameRefStructure typeOfFrameRef;
@XmlElementRef(name = "VersionFrameRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected List> versionFrameRef;
@XmlElement(name = "NetworkFilterByValue")
protected NetworkFilterByValueStructure networkFilterByValue;
/**
* Gets the value of the current property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCurrent() {
return current;
}
/**
* Sets the value of the current property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCurrent(String value) {
this.current = value;
}
/**
* Gets the value of the changedSince property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getChangedSince() {
return changedSince;
}
/**
* Sets the value of the changedSince property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setChangedSince(OffsetDateTime value) {
this.changedSince = value;
}
/**
* Gets the value of the currentAt property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getCurrentAt() {
return currentAt;
}
/**
* Sets the value of the currentAt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCurrentAt(OffsetDateTime value) {
this.currentAt = value;
}
/**
* Gets the value of the historicBetween property.
*
* @return
* possible object is
* {@link ClosedTimestampRangeStructure }
*
*/
public ClosedTimestampRangeStructure getHistoricBetween() {
return historicBetween;
}
/**
* Sets the value of the historicBetween property.
*
* @param value
* allowed object is
* {@link ClosedTimestampRangeStructure }
*
*/
public void setHistoricBetween(ClosedTimestampRangeStructure value) {
this.historicBetween = value;
}
/**
* Gets the value of the selectionValidityConditions property.
*
* @return
* possible object is
* {@link NetworkFrameTopicStructure.SelectionValidityConditions }
*
*/
public NetworkFrameTopicStructure.SelectionValidityConditions getSelectionValidityConditions() {
return selectionValidityConditions;
}
/**
* Sets the value of the selectionValidityConditions property.
*
* @param value
* allowed object is
* {@link NetworkFrameTopicStructure.SelectionValidityConditions }
*
*/
public void setSelectionValidityConditions(NetworkFrameTopicStructure.SelectionValidityConditions value) {
this.selectionValidityConditions = value;
}
/**
* Gets the value of the typeOfFrameRef property.
*
* @return
* possible object is
* {@link TypeOfFrameRefStructure }
*
*/
public TypeOfFrameRefStructure getTypeOfFrameRef() {
return typeOfFrameRef;
}
/**
* Sets the value of the typeOfFrameRef property.
*
* @param value
* allowed object is
* {@link TypeOfFrameRefStructure }
*
*/
public void setTypeOfFrameRef(TypeOfFrameRefStructure value) {
this.typeOfFrameRef = value;
}
/**
* Gets the value of the versionFrameRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the versionFrameRef property.
*
*
* For example, to add a new item, do as follows:
*
* getVersionFrameRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link JAXBElement }{@code <}{@link DriverScheduleFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link TimetableFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link FareFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link CompositeFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link VehicleScheduleFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServiceCalendarFrameRef }{@code >}
* {@link JAXBElement }{@code <}{@link SalesTransactionFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link GeneralFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link SiteFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link VersionFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link InfrastructureFrameRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServiceFrameRefStructure }{@code >}
*
*
*/
public List> getVersionFrameRef() {
if (versionFrameRef == null) {
versionFrameRef = new ArrayList>();
}
return this.versionFrameRef;
}
/**
* Gets the value of the networkFilterByValue property.
*
* @return
* possible object is
* {@link NetworkFilterByValueStructure }
*
*/
public NetworkFilterByValueStructure getNetworkFilterByValue() {
return networkFilterByValue;
}
/**
* Sets the value of the networkFilterByValue property.
*
* @param value
* allowed object is
* {@link NetworkFilterByValueStructure }
*
*/
public void setNetworkFilterByValue(NetworkFilterByValueStructure value) {
this.networkFilterByValue = value;
}
public NetworkFrameTopicStructure withCurrent(String value) {
setCurrent(value);
return this;
}
public NetworkFrameTopicStructure withChangedSince(OffsetDateTime value) {
setChangedSince(value);
return this;
}
public NetworkFrameTopicStructure withCurrentAt(OffsetDateTime value) {
setCurrentAt(value);
return this;
}
public NetworkFrameTopicStructure withHistoricBetween(ClosedTimestampRangeStructure value) {
setHistoricBetween(value);
return this;
}
public NetworkFrameTopicStructure withSelectionValidityConditions(NetworkFrameTopicStructure.SelectionValidityConditions value) {
setSelectionValidityConditions(value);
return this;
}
public NetworkFrameTopicStructure withTypeOfFrameRef(TypeOfFrameRefStructure value) {
setTypeOfFrameRef(value);
return this;
}
public NetworkFrameTopicStructure withVersionFrameRef(JAXBElement extends VersionFrameRefStructure> ... values) {
if (values!= null) {
for (JAXBElement extends VersionFrameRefStructure> value: values) {
getVersionFrameRef().add(value);
}
}
return this;
}
public NetworkFrameTopicStructure withVersionFrameRef(Collection> values) {
if (values!= null) {
getVersionFrameRef().addAll(values);
}
return this;
}
public NetworkFrameTopicStructure withNetworkFilterByValue(NetworkFilterByValueStructure value) {
setNetworkFilterByValue(value);
return this;
}
@Override
public NetworkFrameTopicStructure withDescription(MultilingualString value) {
setDescription(value);
return this;
}
@Override
public NetworkFrameTopicStructure withSources(DataSources_RelStructure value) {
setSources(value);
return this;
}
@Override
public NetworkFrameTopicStructure withCodespaceRef(CodespaceRefStructure value) {
setCodespaceRef(value);
return this;
}
@Override
public NetworkFrameTopicStructure withResponsibilityRoleAssignment(ResponsibilityRoleAssignment_VersionedChildStructure value) {
setResponsibilityRoleAssignment(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice maxOccurs="unbounded">
* <element ref="{http://www.netex.org.uk/netex}ValidityCondition_"/>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"validityCondition_"
})
public static class SelectionValidityConditions {
@XmlElementRef(name = "ValidityCondition_", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected List> validityCondition_;
/**
* Gets the value of the validityCondition property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the validityCondition property.
*
*
* For example, to add a new item, do as follows:
*
* getValidityCondition_().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link JAXBElement }{@code <}{@link ValidityRuleParameter }{@code >}
* {@link JAXBElement }{@code <}{@link ValidityCondition }{@code >}
* {@link JAXBElement }{@code <}{@link ValidityTrigger }{@code >}
* {@link JAXBElement }{@code <}{@link SimpleAvailabilityCondition }{@code >}
* {@link JAXBElement }{@code <}{@link DataManagedObjectStructure }{@code >}
* {@link JAXBElement }{@code <}{@link AvailabilityCondition }{@code >}
* {@link JAXBElement }{@code <}{@link ValidDuring }{@code >}
*
*
*/
public List> getValidityCondition_() {
if (validityCondition_ == null) {
validityCondition_ = new ArrayList>();
}
return this.validityCondition_;
}
public NetworkFrameTopicStructure.SelectionValidityConditions withValidityCondition_(JAXBElement extends DataManagedObjectStructure> ... values) {
if (values!= null) {
for (JAXBElement extends DataManagedObjectStructure> value: values) {
getValidityCondition_().add(value);
}
}
return this;
}
public NetworkFrameTopicStructure.SelectionValidityConditions withValidityCondition_(Collection> values) {
if (values!= null) {
getValidityCondition_().addAll(values);
}
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
}