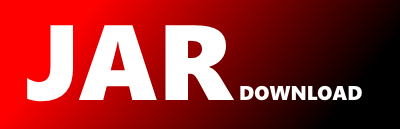
org.rutebanken.netex.model.PresentationStructure Maven / Gradle / Ivy
Show all versions of netex-java-model Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.HexBinaryAdapter;
import javax.xml.bind.annotation.adapters.NormalizedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
/**
* Java class for PresentationStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PresentationStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Colour" type="{http://www.netex.org.uk/netex}ColourValueType" minOccurs="0"/>
* <element name="ColourName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TextColour" type="{http://www.netex.org.uk/netex}ColourValueType" minOccurs="0"/>
* <element name="TextColourName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TextFont" type="{http://www.w3.org/2001/XMLSchema}normalizedString" minOccurs="0"/>
* <element name="TextFontName" type="{http://www.w3.org/2001/XMLSchema}normalizedString" minOccurs="0"/>
* <element name="TextLanguage" type="{http://www.w3.org/2001/XMLSchema}language" minOccurs="0"/>
* <element name="infoLinks" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.netex.org.uk/netex}InfoLink" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PresentationStructure", propOrder = {
"colour",
"colourName",
"textColour",
"textColourName",
"textFont",
"textFontName",
"textLanguage",
"infoLinks"
})
public class PresentationStructure {
@XmlElement(name = "Colour", type = String.class)
@XmlJavaTypeAdapter(HexBinaryAdapter.class)
@XmlSchemaType(name = "hexBinary")
protected byte[] colour;
@XmlElement(name = "ColourName")
protected String colourName;
@XmlElement(name = "TextColour", type = String.class)
@XmlJavaTypeAdapter(HexBinaryAdapter.class)
@XmlSchemaType(name = "hexBinary")
protected byte[] textColour;
@XmlElement(name = "TextColourName")
protected String textColourName;
@XmlElement(name = "TextFont")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String textFont;
@XmlElement(name = "TextFontName")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String textFontName;
@XmlElement(name = "TextLanguage")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String textLanguage;
protected PresentationStructure.InfoLinks infoLinks;
/**
* Gets the value of the colour property.
*
* @return
* possible object is
* {@link String }
*
*/
public byte[] getColour() {
return colour;
}
/**
* Sets the value of the colour property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setColour(byte[] value) {
this.colour = value;
}
/**
* Gets the value of the colourName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getColourName() {
return colourName;
}
/**
* Sets the value of the colourName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setColourName(String value) {
this.colourName = value;
}
/**
* Gets the value of the textColour property.
*
* @return
* possible object is
* {@link String }
*
*/
public byte[] getTextColour() {
return textColour;
}
/**
* Sets the value of the textColour property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextColour(byte[] value) {
this.textColour = value;
}
/**
* Gets the value of the textColourName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextColourName() {
return textColourName;
}
/**
* Sets the value of the textColourName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextColourName(String value) {
this.textColourName = value;
}
/**
* Gets the value of the textFont property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextFont() {
return textFont;
}
/**
* Sets the value of the textFont property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextFont(String value) {
this.textFont = value;
}
/**
* Gets the value of the textFontName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextFontName() {
return textFontName;
}
/**
* Sets the value of the textFontName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextFontName(String value) {
this.textFontName = value;
}
/**
* Gets the value of the textLanguage property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextLanguage() {
return textLanguage;
}
/**
* Sets the value of the textLanguage property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextLanguage(String value) {
this.textLanguage = value;
}
/**
* Gets the value of the infoLinks property.
*
* @return
* possible object is
* {@link PresentationStructure.InfoLinks }
*
*/
public PresentationStructure.InfoLinks getInfoLinks() {
return infoLinks;
}
/**
* Sets the value of the infoLinks property.
*
* @param value
* allowed object is
* {@link PresentationStructure.InfoLinks }
*
*/
public void setInfoLinks(PresentationStructure.InfoLinks value) {
this.infoLinks = value;
}
public PresentationStructure withColour(byte[] value) {
setColour(value);
return this;
}
public PresentationStructure withColourName(String value) {
setColourName(value);
return this;
}
public PresentationStructure withTextColour(byte[] value) {
setTextColour(value);
return this;
}
public PresentationStructure withTextColourName(String value) {
setTextColourName(value);
return this;
}
public PresentationStructure withTextFont(String value) {
setTextFont(value);
return this;
}
public PresentationStructure withTextFontName(String value) {
setTextFontName(value);
return this;
}
public PresentationStructure withTextLanguage(String value) {
setTextLanguage(value);
return this;
}
public PresentationStructure withInfoLinks(PresentationStructure.InfoLinks value) {
setInfoLinks(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.netex.org.uk/netex}InfoLink" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"infoLink"
})
public static class InfoLinks {
@XmlElement(name = "InfoLink")
protected List infoLink;
/**
* Gets the value of the infoLink property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the infoLink property.
*
*
* For example, to add a new item, do as follows:
*
* getInfoLink().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InfoLinkStructure }
*
*
*/
public List getInfoLink() {
if (infoLink == null) {
infoLink = new ArrayList();
}
return this.infoLink;
}
public PresentationStructure.InfoLinks withInfoLink(InfoLinkStructure... values) {
if (values!= null) {
for (InfoLinkStructure value: values) {
getInfoLink().add(value);
}
}
return this;
}
public PresentationStructure.InfoLinks withInfoLink(Collection values) {
if (values!= null) {
getInfoLink().addAll(values);
}
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
}