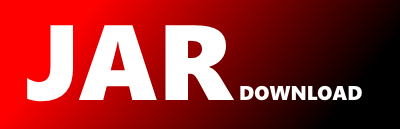
org.rutebanken.netex.model.PrintPresentationStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.NormalizedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
/**
* Java class for PrintPresentationStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PrintPresentationStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Colour" type="{http://www.netex.org.uk/netex}PrintColourValueType" minOccurs="0"/>
* <element name="ColourName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TextColour" type="{http://www.netex.org.uk/netex}PrintColourValueType" minOccurs="0"/>
* <element name="TextColourName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TextFont" type="{http://www.w3.org/2001/XMLSchema}normalizedString" minOccurs="0"/>
* <element name="TextFontName" type="{http://www.w3.org/2001/XMLSchema}normalizedString" minOccurs="0"/>
* <element name="TextLanguage" type="{http://www.w3.org/2001/XMLSchema}language" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PrintPresentationStructure", propOrder = {
"colour",
"colourName",
"textColour",
"textColourName",
"textFont",
"textFontName",
"textLanguage"
})
public class PrintPresentationStructure {
@XmlElement(name = "Colour")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String colour;
@XmlElement(name = "ColourName")
protected String colourName;
@XmlElement(name = "TextColour")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String textColour;
@XmlElement(name = "TextColourName")
protected String textColourName;
@XmlElement(name = "TextFont")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String textFont;
@XmlElement(name = "TextFontName")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String textFontName;
@XmlElement(name = "TextLanguage")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String textLanguage;
/**
* Gets the value of the colour property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getColour() {
return colour;
}
/**
* Sets the value of the colour property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setColour(String value) {
this.colour = value;
}
/**
* Gets the value of the colourName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getColourName() {
return colourName;
}
/**
* Sets the value of the colourName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setColourName(String value) {
this.colourName = value;
}
/**
* Gets the value of the textColour property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextColour() {
return textColour;
}
/**
* Sets the value of the textColour property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextColour(String value) {
this.textColour = value;
}
/**
* Gets the value of the textColourName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextColourName() {
return textColourName;
}
/**
* Sets the value of the textColourName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextColourName(String value) {
this.textColourName = value;
}
/**
* Gets the value of the textFont property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextFont() {
return textFont;
}
/**
* Sets the value of the textFont property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextFont(String value) {
this.textFont = value;
}
/**
* Gets the value of the textFontName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextFontName() {
return textFontName;
}
/**
* Sets the value of the textFontName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextFontName(String value) {
this.textFontName = value;
}
/**
* Gets the value of the textLanguage property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTextLanguage() {
return textLanguage;
}
/**
* Sets the value of the textLanguage property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTextLanguage(String value) {
this.textLanguage = value;
}
public PrintPresentationStructure withColour(String value) {
setColour(value);
return this;
}
public PrintPresentationStructure withColourName(String value) {
setColourName(value);
return this;
}
public PrintPresentationStructure withTextColour(String value) {
setTextColour(value);
return this;
}
public PrintPresentationStructure withTextColourName(String value) {
setTextColourName(value);
return this;
}
public PrintPresentationStructure withTextFont(String value) {
setTextFont(value);
return this;
}
public PrintPresentationStructure withTextFontName(String value) {
setTextFontName(value);
return this;
}
public PrintPresentationStructure withTextLanguage(String value) {
setTextLanguage(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy