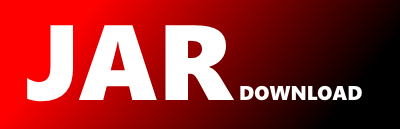
org.rutebanken.netex.model.ServiceJourney_VersionStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netex-java-model Show documentation
Show all versions of netex-java-model Show documentation
Generates Java model from NeTEx XSDs using JAXB.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.09.21 at 10:53:23 AM CEST
//
package org.rutebanken.netex.model;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.Duration;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.util.Collection;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.NormalizedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.migesok.jaxb.adapter.javatime.DurationXmlAdapter;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.rutebanken.netex.OmitNullsToStringStyle;
import org.rutebanken.util.OffsetTimeISO8601XmlAdapter;
/**
* Java class for ServiceJourney_VersionStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ServiceJourney_VersionStructure">
* <complexContent>
* <extension base="{http://www.netex.org.uk/netex}Journey_VersionStructure">
* <group ref="{http://www.netex.org.uk/netex}ServiceJourneyGroup"/>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ServiceJourney_VersionStructure", propOrder = {
"serviceAlteration",
"departureTime",
"departureDayOffset",
"frequency",
"journeyDuration",
"dayTypes",
"routeRef",
"journeyPatternRef",
"timeDemandTypeRef",
"timingAlgorithmTypeRef",
"journeyFrequencyGroupRef",
"vehicleTypeRef",
"operationalContextRef",
"blockRef",
"courseOfJourneysRef",
"publicCode",
"operatorRef",
"operatorView",
"lineRef",
"lineView",
"flexibleLineView",
"directionType",
"journeyPatternView",
"groupsOfServices",
"timeDemandTypes",
"trainNumbers",
"origin",
"destination",
"print",
"dynamic",
"waitTimes",
"runTimes",
"layovers",
"passingTimes",
"parts",
"calls",
"facilities",
"checkConstraints",
"passengerCarryingRequirementRef",
"passengerCarryingRequirementsView",
"trainSize",
"equipments",
"flexibleServicePropertiesRef",
"flexibleServiceProperties"
})
@XmlSeeAlso({
ServiceJourney.class,
TemplateServiceJourney_VersionStructure.class,
DatedServiceJourney_VersionStructure.class
})
public class ServiceJourney_VersionStructure
extends Journey_VersionStructure
{
@XmlElement(name = "ServiceAlteration", defaultValue = "planned")
@XmlSchemaType(name = "NMTOKEN")
protected ServiceAlterationEnumeration serviceAlteration;
@XmlElement(name = "DepartureTime", type = String.class)
@XmlJavaTypeAdapter(OffsetTimeISO8601XmlAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime departureTime;
@XmlElement(name = "DepartureDayOffset")
protected BigInteger departureDayOffset;
@XmlElement(name = "Frequency")
protected FrequencyStructure frequency;
@XmlElement(name = "JourneyDuration", type = String.class)
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration journeyDuration;
protected DayTypeRefs_RelStructure dayTypes;
@XmlElement(name = "RouteRef")
protected RouteRefStructure routeRef;
@XmlElementRef(name = "JourneyPatternRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends JourneyPatternRefStructure> journeyPatternRef;
@XmlElement(name = "TimeDemandTypeRef")
protected TimeDemandTypeRefStructure timeDemandTypeRef;
@XmlElement(name = "TimingAlgorithmTypeRef")
protected TimingAlgorithmTypeRefStructure timingAlgorithmTypeRef;
@XmlElementRef(name = "JourneyFrequencyGroupRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends JourneyFrequencyGroupRefStructure> journeyFrequencyGroupRef;
@XmlElementRef(name = "VehicleTypeRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends VehicleTypeRefStructure> vehicleTypeRef;
@XmlElement(name = "OperationalContextRef")
protected OperationalContextRefStructure operationalContextRef;
@XmlElementRef(name = "BlockRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends BlockRefStructure> blockRef;
@XmlElement(name = "CourseOfJourneysRef")
protected CourseOfJourneysRefStructure courseOfJourneysRef;
@XmlElement(name = "PublicCode")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String publicCode;
@XmlElement(name = "OperatorRef")
protected OperatorRefStructure operatorRef;
@XmlElement(name = "OperatorView")
protected OperatorView operatorView;
@XmlElementRef(name = "LineRef", namespace = "http://www.netex.org.uk/netex", type = JAXBElement.class, required = false)
protected JAXBElement extends LineRefStructure> lineRef;
@XmlElement(name = "LineView")
protected LineView lineView;
@XmlElement(name = "FlexibleLineView")
protected FlexibleLineView flexibleLineView;
@XmlElement(name = "DirectionType", defaultValue = "outbound")
@XmlSchemaType(name = "normalizedString")
protected DirectionTypeEnumeration directionType;
@XmlElement(name = "JourneyPatternView")
protected JourneyPattern_DerivedViewStructure journeyPatternView;
protected GroupOfServicesRefs_RelStructure groupsOfServices;
protected TimeDemandTypeRefs_RelStructure timeDemandTypes;
protected TrainNumberRefs_RelStructure trainNumbers;
@XmlElement(name = "Origin")
protected JourneyEndpointStructure origin;
@XmlElement(name = "Destination")
protected JourneyEndpointStructure destination;
@XmlElement(name = "Print", defaultValue = "true")
protected Boolean print;
@XmlElement(name = "Dynamic", defaultValue = "always")
@XmlSchemaType(name = "NMTOKEN")
protected DynamicAdvertisementEnumeration dynamic;
protected VehicleJourneyWaitTimes_RelStructure waitTimes;
protected VehicleJourneyRunTimes_RelStructure runTimes;
protected VehicleJourneyLayovers_RelStructure layovers;
protected TimetabledPassingTimes_RelStructure passingTimes;
protected JourneyParts_RelStructure parts;
protected Calls_RelStructure calls;
protected ServiceFacilitySets_RelStructure facilities;
protected CheckConstraints_RelStructure checkConstraints;
@XmlElement(name = "PassengerCarryingRequirementRef")
protected PassengerCarryingRequirementRefStructure passengerCarryingRequirementRef;
@XmlElement(name = "PassengerCarryingRequirementsView")
protected PassengerCarryingRequirementsView passengerCarryingRequirementsView;
@XmlElement(name = "TrainSize")
protected TrainSizeStructure trainSize;
protected VehicleEquipments_RelStructure equipments;
@XmlElement(name = "FlexibleServicePropertiesRef")
protected FlexibleServicePropertiesRefStructure flexibleServicePropertiesRef;
@XmlElement(name = "FlexibleServiceProperties")
protected FlexibleServiceProperties flexibleServiceProperties;
/**
* Gets the value of the serviceAlteration property.
*
* @return
* possible object is
* {@link ServiceAlterationEnumeration }
*
*/
public ServiceAlterationEnumeration getServiceAlteration() {
return serviceAlteration;
}
/**
* Sets the value of the serviceAlteration property.
*
* @param value
* allowed object is
* {@link ServiceAlterationEnumeration }
*
*/
public void setServiceAlteration(ServiceAlterationEnumeration value) {
this.serviceAlteration = value;
}
/**
* Gets the value of the departureTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getDepartureTime() {
return departureTime;
}
/**
* Sets the value of the departureTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDepartureTime(OffsetTime value) {
this.departureTime = value;
}
/**
* Gets the value of the departureDayOffset property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getDepartureDayOffset() {
return departureDayOffset;
}
/**
* Sets the value of the departureDayOffset property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setDepartureDayOffset(BigInteger value) {
this.departureDayOffset = value;
}
/**
* Gets the value of the frequency property.
*
* @return
* possible object is
* {@link FrequencyStructure }
*
*/
public FrequencyStructure getFrequency() {
return frequency;
}
/**
* Sets the value of the frequency property.
*
* @param value
* allowed object is
* {@link FrequencyStructure }
*
*/
public void setFrequency(FrequencyStructure value) {
this.frequency = value;
}
/**
* Gets the value of the journeyDuration property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getJourneyDuration() {
return journeyDuration;
}
/**
* Sets the value of the journeyDuration property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJourneyDuration(Duration value) {
this.journeyDuration = value;
}
/**
* Gets the value of the dayTypes property.
*
* @return
* possible object is
* {@link DayTypeRefs_RelStructure }
*
*/
public DayTypeRefs_RelStructure getDayTypes() {
return dayTypes;
}
/**
* Sets the value of the dayTypes property.
*
* @param value
* allowed object is
* {@link DayTypeRefs_RelStructure }
*
*/
public void setDayTypes(DayTypeRefs_RelStructure value) {
this.dayTypes = value;
}
/**
* Gets the value of the routeRef property.
*
* @return
* possible object is
* {@link RouteRefStructure }
*
*/
public RouteRefStructure getRouteRef() {
return routeRef;
}
/**
* Sets the value of the routeRef property.
*
* @param value
* allowed object is
* {@link RouteRefStructure }
*
*/
public void setRouteRef(RouteRefStructure value) {
this.routeRef = value;
}
/**
* Gets the value of the journeyPatternRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link ServiceJourneyPatternRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link JourneyPatternRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServicePatternRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DeadRunJourneyPatternRefStructure }{@code >}
*
*/
public JAXBElement extends JourneyPatternRefStructure> getJourneyPatternRef() {
return journeyPatternRef;
}
/**
* Sets the value of the journeyPatternRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link ServiceJourneyPatternRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link JourneyPatternRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link ServicePatternRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link DeadRunJourneyPatternRefStructure }{@code >}
*
*/
public void setJourneyPatternRef(JAXBElement extends JourneyPatternRefStructure> value) {
this.journeyPatternRef = value;
}
/**
* Gets the value of the timeDemandTypeRef property.
*
* @return
* possible object is
* {@link TimeDemandTypeRefStructure }
*
*/
public TimeDemandTypeRefStructure getTimeDemandTypeRef() {
return timeDemandTypeRef;
}
/**
* Sets the value of the timeDemandTypeRef property.
*
* @param value
* allowed object is
* {@link TimeDemandTypeRefStructure }
*
*/
public void setTimeDemandTypeRef(TimeDemandTypeRefStructure value) {
this.timeDemandTypeRef = value;
}
/**
* Gets the value of the timingAlgorithmTypeRef property.
*
* @return
* possible object is
* {@link TimingAlgorithmTypeRefStructure }
*
*/
public TimingAlgorithmTypeRefStructure getTimingAlgorithmTypeRef() {
return timingAlgorithmTypeRef;
}
/**
* Sets the value of the timingAlgorithmTypeRef property.
*
* @param value
* allowed object is
* {@link TimingAlgorithmTypeRefStructure }
*
*/
public void setTimingAlgorithmTypeRef(TimingAlgorithmTypeRefStructure value) {
this.timingAlgorithmTypeRef = value;
}
/**
* Gets the value of the journeyFrequencyGroupRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link HeadwayJourneyGroupRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link RhythmicalJourneyGroupRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link JourneyFrequencyGroupRefStructure }{@code >}
*
*/
public JAXBElement extends JourneyFrequencyGroupRefStructure> getJourneyFrequencyGroupRef() {
return journeyFrequencyGroupRef;
}
/**
* Sets the value of the journeyFrequencyGroupRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link HeadwayJourneyGroupRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link RhythmicalJourneyGroupRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link JourneyFrequencyGroupRefStructure }{@code >}
*
*/
public void setJourneyFrequencyGroupRef(JAXBElement extends JourneyFrequencyGroupRefStructure> value) {
this.journeyFrequencyGroupRef = value;
}
/**
* Gets the value of the vehicleTypeRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link CompoundTrainRef }{@code >}
* {@link JAXBElement }{@code <}{@link TrainRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link VehicleTypeRefStructure }{@code >}
*
*/
public JAXBElement extends VehicleTypeRefStructure> getVehicleTypeRef() {
return vehicleTypeRef;
}
/**
* Sets the value of the vehicleTypeRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link CompoundTrainRef }{@code >}
* {@link JAXBElement }{@code <}{@link TrainRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link VehicleTypeRefStructure }{@code >}
*
*/
public void setVehicleTypeRef(JAXBElement extends VehicleTypeRefStructure> value) {
this.vehicleTypeRef = value;
}
/**
* Gets the value of the operationalContextRef property.
*
* @return
* possible object is
* {@link OperationalContextRefStructure }
*
*/
public OperationalContextRefStructure getOperationalContextRef() {
return operationalContextRef;
}
/**
* Sets the value of the operationalContextRef property.
*
* @param value
* allowed object is
* {@link OperationalContextRefStructure }
*
*/
public void setOperationalContextRef(OperationalContextRefStructure value) {
this.operationalContextRef = value;
}
/**
* Gets the value of the blockRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link TrainBlockRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link BlockRefStructure }{@code >}
*
*/
public JAXBElement extends BlockRefStructure> getBlockRef() {
return blockRef;
}
/**
* Sets the value of the blockRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link TrainBlockRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link BlockRefStructure }{@code >}
*
*/
public void setBlockRef(JAXBElement extends BlockRefStructure> value) {
this.blockRef = value;
}
/**
* Gets the value of the courseOfJourneysRef property.
*
* @return
* possible object is
* {@link CourseOfJourneysRefStructure }
*
*/
public CourseOfJourneysRefStructure getCourseOfJourneysRef() {
return courseOfJourneysRef;
}
/**
* Sets the value of the courseOfJourneysRef property.
*
* @param value
* allowed object is
* {@link CourseOfJourneysRefStructure }
*
*/
public void setCourseOfJourneysRef(CourseOfJourneysRefStructure value) {
this.courseOfJourneysRef = value;
}
/**
* Gets the value of the publicCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPublicCode() {
return publicCode;
}
/**
* Sets the value of the publicCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPublicCode(String value) {
this.publicCode = value;
}
/**
* Gets the value of the operatorRef property.
*
* @return
* possible object is
* {@link OperatorRefStructure }
*
*/
public OperatorRefStructure getOperatorRef() {
return operatorRef;
}
/**
* Sets the value of the operatorRef property.
*
* @param value
* allowed object is
* {@link OperatorRefStructure }
*
*/
public void setOperatorRef(OperatorRefStructure value) {
this.operatorRef = value;
}
/**
* Gets the value of the operatorView property.
*
* @return
* possible object is
* {@link OperatorView }
*
*/
public OperatorView getOperatorView() {
return operatorView;
}
/**
* Sets the value of the operatorView property.
*
* @param value
* allowed object is
* {@link OperatorView }
*
*/
public void setOperatorView(OperatorView value) {
this.operatorView = value;
}
/**
* Gets the value of the lineRef property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link FlexibleLineRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link LineRefStructure }{@code >}
*
*/
public JAXBElement extends LineRefStructure> getLineRef() {
return lineRef;
}
/**
* Sets the value of the lineRef property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link FlexibleLineRefStructure }{@code >}
* {@link JAXBElement }{@code <}{@link LineRefStructure }{@code >}
*
*/
public void setLineRef(JAXBElement extends LineRefStructure> value) {
this.lineRef = value;
}
/**
* Gets the value of the lineView property.
*
* @return
* possible object is
* {@link LineView }
*
*/
public LineView getLineView() {
return lineView;
}
/**
* Sets the value of the lineView property.
*
* @param value
* allowed object is
* {@link LineView }
*
*/
public void setLineView(LineView value) {
this.lineView = value;
}
/**
* Gets the value of the flexibleLineView property.
*
* @return
* possible object is
* {@link FlexibleLineView }
*
*/
public FlexibleLineView getFlexibleLineView() {
return flexibleLineView;
}
/**
* Sets the value of the flexibleLineView property.
*
* @param value
* allowed object is
* {@link FlexibleLineView }
*
*/
public void setFlexibleLineView(FlexibleLineView value) {
this.flexibleLineView = value;
}
/**
* Gets the value of the directionType property.
*
* @return
* possible object is
* {@link DirectionTypeEnumeration }
*
*/
public DirectionTypeEnumeration getDirectionType() {
return directionType;
}
/**
* Sets the value of the directionType property.
*
* @param value
* allowed object is
* {@link DirectionTypeEnumeration }
*
*/
public void setDirectionType(DirectionTypeEnumeration value) {
this.directionType = value;
}
/**
* Gets the value of the journeyPatternView property.
*
* @return
* possible object is
* {@link JourneyPattern_DerivedViewStructure }
*
*/
public JourneyPattern_DerivedViewStructure getJourneyPatternView() {
return journeyPatternView;
}
/**
* Sets the value of the journeyPatternView property.
*
* @param value
* allowed object is
* {@link JourneyPattern_DerivedViewStructure }
*
*/
public void setJourneyPatternView(JourneyPattern_DerivedViewStructure value) {
this.journeyPatternView = value;
}
/**
* Gets the value of the groupsOfServices property.
*
* @return
* possible object is
* {@link GroupOfServicesRefs_RelStructure }
*
*/
public GroupOfServicesRefs_RelStructure getGroupsOfServices() {
return groupsOfServices;
}
/**
* Sets the value of the groupsOfServices property.
*
* @param value
* allowed object is
* {@link GroupOfServicesRefs_RelStructure }
*
*/
public void setGroupsOfServices(GroupOfServicesRefs_RelStructure value) {
this.groupsOfServices = value;
}
/**
* Gets the value of the timeDemandTypes property.
*
* @return
* possible object is
* {@link TimeDemandTypeRefs_RelStructure }
*
*/
public TimeDemandTypeRefs_RelStructure getTimeDemandTypes() {
return timeDemandTypes;
}
/**
* Sets the value of the timeDemandTypes property.
*
* @param value
* allowed object is
* {@link TimeDemandTypeRefs_RelStructure }
*
*/
public void setTimeDemandTypes(TimeDemandTypeRefs_RelStructure value) {
this.timeDemandTypes = value;
}
/**
* Gets the value of the trainNumbers property.
*
* @return
* possible object is
* {@link TrainNumberRefs_RelStructure }
*
*/
public TrainNumberRefs_RelStructure getTrainNumbers() {
return trainNumbers;
}
/**
* Sets the value of the trainNumbers property.
*
* @param value
* allowed object is
* {@link TrainNumberRefs_RelStructure }
*
*/
public void setTrainNumbers(TrainNumberRefs_RelStructure value) {
this.trainNumbers = value;
}
/**
* Gets the value of the origin property.
*
* @return
* possible object is
* {@link JourneyEndpointStructure }
*
*/
public JourneyEndpointStructure getOrigin() {
return origin;
}
/**
* Sets the value of the origin property.
*
* @param value
* allowed object is
* {@link JourneyEndpointStructure }
*
*/
public void setOrigin(JourneyEndpointStructure value) {
this.origin = value;
}
/**
* Gets the value of the destination property.
*
* @return
* possible object is
* {@link JourneyEndpointStructure }
*
*/
public JourneyEndpointStructure getDestination() {
return destination;
}
/**
* Sets the value of the destination property.
*
* @param value
* allowed object is
* {@link JourneyEndpointStructure }
*
*/
public void setDestination(JourneyEndpointStructure value) {
this.destination = value;
}
/**
* Gets the value of the print property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isPrint() {
return print;
}
/**
* Sets the value of the print property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setPrint(Boolean value) {
this.print = value;
}
/**
* Gets the value of the dynamic property.
*
* @return
* possible object is
* {@link DynamicAdvertisementEnumeration }
*
*/
public DynamicAdvertisementEnumeration getDynamic() {
return dynamic;
}
/**
* Sets the value of the dynamic property.
*
* @param value
* allowed object is
* {@link DynamicAdvertisementEnumeration }
*
*/
public void setDynamic(DynamicAdvertisementEnumeration value) {
this.dynamic = value;
}
/**
* Gets the value of the waitTimes property.
*
* @return
* possible object is
* {@link VehicleJourneyWaitTimes_RelStructure }
*
*/
public VehicleJourneyWaitTimes_RelStructure getWaitTimes() {
return waitTimes;
}
/**
* Sets the value of the waitTimes property.
*
* @param value
* allowed object is
* {@link VehicleJourneyWaitTimes_RelStructure }
*
*/
public void setWaitTimes(VehicleJourneyWaitTimes_RelStructure value) {
this.waitTimes = value;
}
/**
* Gets the value of the runTimes property.
*
* @return
* possible object is
* {@link VehicleJourneyRunTimes_RelStructure }
*
*/
public VehicleJourneyRunTimes_RelStructure getRunTimes() {
return runTimes;
}
/**
* Sets the value of the runTimes property.
*
* @param value
* allowed object is
* {@link VehicleJourneyRunTimes_RelStructure }
*
*/
public void setRunTimes(VehicleJourneyRunTimes_RelStructure value) {
this.runTimes = value;
}
/**
* Gets the value of the layovers property.
*
* @return
* possible object is
* {@link VehicleJourneyLayovers_RelStructure }
*
*/
public VehicleJourneyLayovers_RelStructure getLayovers() {
return layovers;
}
/**
* Sets the value of the layovers property.
*
* @param value
* allowed object is
* {@link VehicleJourneyLayovers_RelStructure }
*
*/
public void setLayovers(VehicleJourneyLayovers_RelStructure value) {
this.layovers = value;
}
/**
* Gets the value of the passingTimes property.
*
* @return
* possible object is
* {@link TimetabledPassingTimes_RelStructure }
*
*/
public TimetabledPassingTimes_RelStructure getPassingTimes() {
return passingTimes;
}
/**
* Sets the value of the passingTimes property.
*
* @param value
* allowed object is
* {@link TimetabledPassingTimes_RelStructure }
*
*/
public void setPassingTimes(TimetabledPassingTimes_RelStructure value) {
this.passingTimes = value;
}
/**
* Gets the value of the parts property.
*
* @return
* possible object is
* {@link JourneyParts_RelStructure }
*
*/
public JourneyParts_RelStructure getParts() {
return parts;
}
/**
* Sets the value of the parts property.
*
* @param value
* allowed object is
* {@link JourneyParts_RelStructure }
*
*/
public void setParts(JourneyParts_RelStructure value) {
this.parts = value;
}
/**
* Gets the value of the calls property.
*
* @return
* possible object is
* {@link Calls_RelStructure }
*
*/
public Calls_RelStructure getCalls() {
return calls;
}
/**
* Sets the value of the calls property.
*
* @param value
* allowed object is
* {@link Calls_RelStructure }
*
*/
public void setCalls(Calls_RelStructure value) {
this.calls = value;
}
/**
* Gets the value of the facilities property.
*
* @return
* possible object is
* {@link ServiceFacilitySets_RelStructure }
*
*/
public ServiceFacilitySets_RelStructure getFacilities() {
return facilities;
}
/**
* Sets the value of the facilities property.
*
* @param value
* allowed object is
* {@link ServiceFacilitySets_RelStructure }
*
*/
public void setFacilities(ServiceFacilitySets_RelStructure value) {
this.facilities = value;
}
/**
* Gets the value of the checkConstraints property.
*
* @return
* possible object is
* {@link CheckConstraints_RelStructure }
*
*/
public CheckConstraints_RelStructure getCheckConstraints() {
return checkConstraints;
}
/**
* Sets the value of the checkConstraints property.
*
* @param value
* allowed object is
* {@link CheckConstraints_RelStructure }
*
*/
public void setCheckConstraints(CheckConstraints_RelStructure value) {
this.checkConstraints = value;
}
/**
* Gets the value of the passengerCarryingRequirementRef property.
*
* @return
* possible object is
* {@link PassengerCarryingRequirementRefStructure }
*
*/
public PassengerCarryingRequirementRefStructure getPassengerCarryingRequirementRef() {
return passengerCarryingRequirementRef;
}
/**
* Sets the value of the passengerCarryingRequirementRef property.
*
* @param value
* allowed object is
* {@link PassengerCarryingRequirementRefStructure }
*
*/
public void setPassengerCarryingRequirementRef(PassengerCarryingRequirementRefStructure value) {
this.passengerCarryingRequirementRef = value;
}
/**
* Gets the value of the passengerCarryingRequirementsView property.
*
* @return
* possible object is
* {@link PassengerCarryingRequirementsView }
*
*/
public PassengerCarryingRequirementsView getPassengerCarryingRequirementsView() {
return passengerCarryingRequirementsView;
}
/**
* Sets the value of the passengerCarryingRequirementsView property.
*
* @param value
* allowed object is
* {@link PassengerCarryingRequirementsView }
*
*/
public void setPassengerCarryingRequirementsView(PassengerCarryingRequirementsView value) {
this.passengerCarryingRequirementsView = value;
}
/**
* Gets the value of the trainSize property.
*
* @return
* possible object is
* {@link TrainSizeStructure }
*
*/
public TrainSizeStructure getTrainSize() {
return trainSize;
}
/**
* Sets the value of the trainSize property.
*
* @param value
* allowed object is
* {@link TrainSizeStructure }
*
*/
public void setTrainSize(TrainSizeStructure value) {
this.trainSize = value;
}
/**
* Gets the value of the equipments property.
*
* @return
* possible object is
* {@link VehicleEquipments_RelStructure }
*
*/
public VehicleEquipments_RelStructure getEquipments() {
return equipments;
}
/**
* Sets the value of the equipments property.
*
* @param value
* allowed object is
* {@link VehicleEquipments_RelStructure }
*
*/
public void setEquipments(VehicleEquipments_RelStructure value) {
this.equipments = value;
}
/**
* Gets the value of the flexibleServicePropertiesRef property.
*
* @return
* possible object is
* {@link FlexibleServicePropertiesRefStructure }
*
*/
public FlexibleServicePropertiesRefStructure getFlexibleServicePropertiesRef() {
return flexibleServicePropertiesRef;
}
/**
* Sets the value of the flexibleServicePropertiesRef property.
*
* @param value
* allowed object is
* {@link FlexibleServicePropertiesRefStructure }
*
*/
public void setFlexibleServicePropertiesRef(FlexibleServicePropertiesRefStructure value) {
this.flexibleServicePropertiesRef = value;
}
/**
* Gets the value of the flexibleServiceProperties property.
*
* @return
* possible object is
* {@link FlexibleServiceProperties }
*
*/
public FlexibleServiceProperties getFlexibleServiceProperties() {
return flexibleServiceProperties;
}
/**
* Sets the value of the flexibleServiceProperties property.
*
* @param value
* allowed object is
* {@link FlexibleServiceProperties }
*
*/
public void setFlexibleServiceProperties(FlexibleServiceProperties value) {
this.flexibleServiceProperties = value;
}
public ServiceJourney_VersionStructure withServiceAlteration(ServiceAlterationEnumeration value) {
setServiceAlteration(value);
return this;
}
public ServiceJourney_VersionStructure withDepartureTime(OffsetTime value) {
setDepartureTime(value);
return this;
}
public ServiceJourney_VersionStructure withDepartureDayOffset(BigInteger value) {
setDepartureDayOffset(value);
return this;
}
public ServiceJourney_VersionStructure withFrequency(FrequencyStructure value) {
setFrequency(value);
return this;
}
public ServiceJourney_VersionStructure withJourneyDuration(Duration value) {
setJourneyDuration(value);
return this;
}
public ServiceJourney_VersionStructure withDayTypes(DayTypeRefs_RelStructure value) {
setDayTypes(value);
return this;
}
public ServiceJourney_VersionStructure withRouteRef(RouteRefStructure value) {
setRouteRef(value);
return this;
}
public ServiceJourney_VersionStructure withJourneyPatternRef(JAXBElement extends JourneyPatternRefStructure> value) {
setJourneyPatternRef(value);
return this;
}
public ServiceJourney_VersionStructure withTimeDemandTypeRef(TimeDemandTypeRefStructure value) {
setTimeDemandTypeRef(value);
return this;
}
public ServiceJourney_VersionStructure withTimingAlgorithmTypeRef(TimingAlgorithmTypeRefStructure value) {
setTimingAlgorithmTypeRef(value);
return this;
}
public ServiceJourney_VersionStructure withJourneyFrequencyGroupRef(JAXBElement extends JourneyFrequencyGroupRefStructure> value) {
setJourneyFrequencyGroupRef(value);
return this;
}
public ServiceJourney_VersionStructure withVehicleTypeRef(JAXBElement extends VehicleTypeRefStructure> value) {
setVehicleTypeRef(value);
return this;
}
public ServiceJourney_VersionStructure withOperationalContextRef(OperationalContextRefStructure value) {
setOperationalContextRef(value);
return this;
}
public ServiceJourney_VersionStructure withBlockRef(JAXBElement extends BlockRefStructure> value) {
setBlockRef(value);
return this;
}
public ServiceJourney_VersionStructure withCourseOfJourneysRef(CourseOfJourneysRefStructure value) {
setCourseOfJourneysRef(value);
return this;
}
public ServiceJourney_VersionStructure withPublicCode(String value) {
setPublicCode(value);
return this;
}
public ServiceJourney_VersionStructure withOperatorRef(OperatorRefStructure value) {
setOperatorRef(value);
return this;
}
public ServiceJourney_VersionStructure withOperatorView(OperatorView value) {
setOperatorView(value);
return this;
}
public ServiceJourney_VersionStructure withLineRef(JAXBElement extends LineRefStructure> value) {
setLineRef(value);
return this;
}
public ServiceJourney_VersionStructure withLineView(LineView value) {
setLineView(value);
return this;
}
public ServiceJourney_VersionStructure withFlexibleLineView(FlexibleLineView value) {
setFlexibleLineView(value);
return this;
}
public ServiceJourney_VersionStructure withDirectionType(DirectionTypeEnumeration value) {
setDirectionType(value);
return this;
}
public ServiceJourney_VersionStructure withJourneyPatternView(JourneyPattern_DerivedViewStructure value) {
setJourneyPatternView(value);
return this;
}
public ServiceJourney_VersionStructure withGroupsOfServices(GroupOfServicesRefs_RelStructure value) {
setGroupsOfServices(value);
return this;
}
public ServiceJourney_VersionStructure withTimeDemandTypes(TimeDemandTypeRefs_RelStructure value) {
setTimeDemandTypes(value);
return this;
}
public ServiceJourney_VersionStructure withTrainNumbers(TrainNumberRefs_RelStructure value) {
setTrainNumbers(value);
return this;
}
public ServiceJourney_VersionStructure withOrigin(JourneyEndpointStructure value) {
setOrigin(value);
return this;
}
public ServiceJourney_VersionStructure withDestination(JourneyEndpointStructure value) {
setDestination(value);
return this;
}
public ServiceJourney_VersionStructure withPrint(Boolean value) {
setPrint(value);
return this;
}
public ServiceJourney_VersionStructure withDynamic(DynamicAdvertisementEnumeration value) {
setDynamic(value);
return this;
}
public ServiceJourney_VersionStructure withWaitTimes(VehicleJourneyWaitTimes_RelStructure value) {
setWaitTimes(value);
return this;
}
public ServiceJourney_VersionStructure withRunTimes(VehicleJourneyRunTimes_RelStructure value) {
setRunTimes(value);
return this;
}
public ServiceJourney_VersionStructure withLayovers(VehicleJourneyLayovers_RelStructure value) {
setLayovers(value);
return this;
}
public ServiceJourney_VersionStructure withPassingTimes(TimetabledPassingTimes_RelStructure value) {
setPassingTimes(value);
return this;
}
public ServiceJourney_VersionStructure withParts(JourneyParts_RelStructure value) {
setParts(value);
return this;
}
public ServiceJourney_VersionStructure withCalls(Calls_RelStructure value) {
setCalls(value);
return this;
}
public ServiceJourney_VersionStructure withFacilities(ServiceFacilitySets_RelStructure value) {
setFacilities(value);
return this;
}
public ServiceJourney_VersionStructure withCheckConstraints(CheckConstraints_RelStructure value) {
setCheckConstraints(value);
return this;
}
public ServiceJourney_VersionStructure withPassengerCarryingRequirementRef(PassengerCarryingRequirementRefStructure value) {
setPassengerCarryingRequirementRef(value);
return this;
}
public ServiceJourney_VersionStructure withPassengerCarryingRequirementsView(PassengerCarryingRequirementsView value) {
setPassengerCarryingRequirementsView(value);
return this;
}
public ServiceJourney_VersionStructure withTrainSize(TrainSizeStructure value) {
setTrainSize(value);
return this;
}
public ServiceJourney_VersionStructure withEquipments(VehicleEquipments_RelStructure value) {
setEquipments(value);
return this;
}
public ServiceJourney_VersionStructure withFlexibleServicePropertiesRef(FlexibleServicePropertiesRefStructure value) {
setFlexibleServicePropertiesRef(value);
return this;
}
public ServiceJourney_VersionStructure withFlexibleServiceProperties(FlexibleServiceProperties value) {
setFlexibleServiceProperties(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withDescription(MultilingualString value) {
setDescription(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withTransportMode(AllVehicleModesOfTransportEnumeration value) {
setTransportMode(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withTransportSubmode(TransportSubmodeStructure value) {
setTransportSubmode(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withExternalVehicleJourneyRef(ExternalObjectRefStructure value) {
setExternalVehicleJourneyRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withTypeOfProductCategoryRef(TypeOfProductCategoryRefStructure value) {
setTypeOfProductCategoryRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withTypeOfServiceRef(TypeOfServiceRefStructure value) {
setTypeOfServiceRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withLinkSequenceProjectionRef(LinkSequenceProjectionRefStructure value) {
setLinkSequenceProjectionRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withLinkSequenceProjection(LinkSequenceProjection value) {
setLinkSequenceProjection(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withMonitored(Boolean value) {
setMonitored(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withAccessibilityAssessment(AccessibilityAssessment value) {
setAccessibilityAssessment(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withJourneyAccountings(JourneyAccountings_RelStructure value) {
setJourneyAccountings(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withNoticeAssignments(NoticeAssignments_RelStructure value) {
setNoticeAssignments(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withName(MultilingualString value) {
setName(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withShortName(MultilingualString value) {
setShortName(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withDistance(BigDecimal value) {
setDistance(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withPrivateCode(PrivateCodeStructure value) {
setPrivateCode(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withSectionsInSequence(SectionsInSequence_RelStructure value) {
setSectionsInSequence(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withKeyList(KeyListStructure value) {
setKeyList(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withExtensions(ExtensionsStructure value) {
setExtensions(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withBrandingRef(BrandingRefStructure value) {
setBrandingRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withAlternativeTexts(AlternativeTexts_RelStructure value) {
setAlternativeTexts(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withResponsibilitySetRef(String value) {
setResponsibilitySetRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withValidityConditions(ValidityConditions_RelStructure value) {
setValidityConditions(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withValidBetween(ValidBetween... values) {
if (values!= null) {
for (ValidBetween value: values) {
getValidBetween().add(value);
}
}
return this;
}
@Override
public ServiceJourney_VersionStructure withValidBetween(Collection values) {
if (values!= null) {
getValidBetween().addAll(values);
}
return this;
}
@Override
public ServiceJourney_VersionStructure withDataSourceRef(String value) {
setDataSourceRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withCreated(OffsetDateTime value) {
setCreated(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withChanged(OffsetDateTime value) {
setChanged(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withModification(ModificationEnumeration value) {
setModification(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withVersion(String value) {
setVersion(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withStatus_BasicModificationDetailsGroup(StatusEnumeration value) {
setStatus_BasicModificationDetailsGroup(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withDerivedFromVersionRef_BasicModificationDetailsGroup(String value) {
setDerivedFromVersionRef_BasicModificationDetailsGroup(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withCompatibleWithVersionFrameVersionRef(String value) {
setCompatibleWithVersionFrameVersionRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withDerivedFromObjectRef(String value) {
setDerivedFromObjectRef(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withNameOfClass(String value) {
setNameOfClass(value);
return this;
}
@Override
public ServiceJourney_VersionStructure withId(String value) {
setId(value);
return this;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, OmitNullsToStringStyle.INSTANCE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy