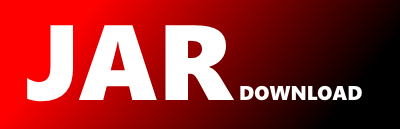
uk.org.siri.siri20.AffectedStopPointStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import uk.org.acbs.siri20.AccessibilityAssessmentStructure;
/**
* Type for an SCHEDUELD STOP POINT affected by a SITUATION.
*
* Java class for AffectedStopPointStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AffectedStopPointStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}StopPointRef" minOccurs="0"/>
* <element name="PrivateRef" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="StopPointName" type="{http://www.siri.org.uk/siri}NaturalLanguageStringStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="StopPointType" type="{http://www.siri.org.uk/siri}StopPointTypeEnumeration" minOccurs="0"/>
* <element name="Location" type="{http://www.siri.org.uk/siri}LocationStructure" minOccurs="0"/>
* <element name="AffectedModes" type="{http://www.siri.org.uk/siri}AffectedModesStructure" minOccurs="0"/>
* <element name="PlaceRef" type="{http://www.siri.org.uk/siri}ZoneRefStructure" minOccurs="0"/>
* <element name="PlaceName" type="{http://www.siri.org.uk/siri}NaturalLanguageStringStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="AccessibilityAssessment" type="{http://www.ifopt.org.uk/acsb}AccessibilityAssessmentStructure" minOccurs="0"/>
* <element name="StopCondition" type="{http://www.siri.org.uk/siri}RoutePointTypeEnumeration" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ConnectionLinks" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedConnectionLink" type="{http://www.siri.org.uk/siri}AffectedConnectionLinkStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AffectedStopPointStructure", propOrder = {
"stopPointRef",
"privateRef",
"stopPointNames",
"stopPointType",
"location",
"affectedModes",
"placeRef",
"placeNames",
"accessibilityAssessment",
"stopConditions",
"connectionLinks",
"extensions"
})
@XmlSeeAlso({
AffectedCallStructure.class
})
public class AffectedStopPointStructure implements Serializable
{
@XmlElement(name = "StopPointRef")
protected StopPointRef stopPointRef;
@XmlElement(name = "PrivateRef")
protected String privateRef;
@XmlElement(name = "StopPointName")
protected List stopPointNames;
@XmlElement(name = "StopPointType")
@XmlSchemaType(name = "NMTOKEN")
protected StopPointTypeEnumeration stopPointType;
@XmlElement(name = "Location")
protected LocationStructure location;
@XmlElement(name = "AffectedModes")
protected AffectedModesStructure affectedModes;
@XmlElement(name = "PlaceRef")
protected ZoneRefStructure placeRef;
@XmlElement(name = "PlaceName")
protected List placeNames;
@XmlElement(name = "AccessibilityAssessment")
protected AccessibilityAssessmentStructure accessibilityAssessment;
@XmlElement(name = "StopCondition")
@XmlSchemaType(name = "NMTOKEN")
protected List stopConditions;
@XmlElement(name = "ConnectionLinks")
protected AffectedStopPointStructure.ConnectionLinks connectionLinks;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the stopPointRef property.
*
* @return
* possible object is
* {@link StopPointRef }
*
*/
public StopPointRef getStopPointRef() {
return stopPointRef;
}
/**
* Sets the value of the stopPointRef property.
*
* @param value
* allowed object is
* {@link StopPointRef }
*
*/
public void setStopPointRef(StopPointRef value) {
this.stopPointRef = value;
}
/**
* Gets the value of the privateRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrivateRef() {
return privateRef;
}
/**
* Sets the value of the privateRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrivateRef(String value) {
this.privateRef = value;
}
/**
* Gets the value of the stopPointNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the stopPointNames property.
*
*
* For example, to add a new item, do as follows:
*
* getStopPointNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getStopPointNames() {
if (stopPointNames == null) {
stopPointNames = new ArrayList();
}
return this.stopPointNames;
}
/**
* Gets the value of the stopPointType property.
*
* @return
* possible object is
* {@link StopPointTypeEnumeration }
*
*/
public StopPointTypeEnumeration getStopPointType() {
return stopPointType;
}
/**
* Sets the value of the stopPointType property.
*
* @param value
* allowed object is
* {@link StopPointTypeEnumeration }
*
*/
public void setStopPointType(StopPointTypeEnumeration value) {
this.stopPointType = value;
}
/**
* Gets the value of the location property.
*
* @return
* possible object is
* {@link LocationStructure }
*
*/
public LocationStructure getLocation() {
return location;
}
/**
* Sets the value of the location property.
*
* @param value
* allowed object is
* {@link LocationStructure }
*
*/
public void setLocation(LocationStructure value) {
this.location = value;
}
/**
* Gets the value of the affectedModes property.
*
* @return
* possible object is
* {@link AffectedModesStructure }
*
*/
public AffectedModesStructure getAffectedModes() {
return affectedModes;
}
/**
* Sets the value of the affectedModes property.
*
* @param value
* allowed object is
* {@link AffectedModesStructure }
*
*/
public void setAffectedModes(AffectedModesStructure value) {
this.affectedModes = value;
}
/**
* Gets the value of the placeRef property.
*
* @return
* possible object is
* {@link ZoneRefStructure }
*
*/
public ZoneRefStructure getPlaceRef() {
return placeRef;
}
/**
* Sets the value of the placeRef property.
*
* @param value
* allowed object is
* {@link ZoneRefStructure }
*
*/
public void setPlaceRef(ZoneRefStructure value) {
this.placeRef = value;
}
/**
* Gets the value of the placeNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the placeNames property.
*
*
* For example, to add a new item, do as follows:
*
* getPlaceNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getPlaceNames() {
if (placeNames == null) {
placeNames = new ArrayList();
}
return this.placeNames;
}
/**
* Gets the value of the accessibilityAssessment property.
*
* @return
* possible object is
* {@link AccessibilityAssessmentStructure }
*
*/
public AccessibilityAssessmentStructure getAccessibilityAssessment() {
return accessibilityAssessment;
}
/**
* Sets the value of the accessibilityAssessment property.
*
* @param value
* allowed object is
* {@link AccessibilityAssessmentStructure }
*
*/
public void setAccessibilityAssessment(AccessibilityAssessmentStructure value) {
this.accessibilityAssessment = value;
}
/**
* Gets the value of the stopConditions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the stopConditions property.
*
*
* For example, to add a new item, do as follows:
*
* getStopConditions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RoutePointTypeEnumeration }
*
*
*/
public List getStopConditions() {
if (stopConditions == null) {
stopConditions = new ArrayList();
}
return this.stopConditions;
}
/**
* Gets the value of the connectionLinks property.
*
* @return
* possible object is
* {@link AffectedStopPointStructure.ConnectionLinks }
*
*/
public AffectedStopPointStructure.ConnectionLinks getConnectionLinks() {
return connectionLinks;
}
/**
* Sets the value of the connectionLinks property.
*
* @param value
* allowed object is
* {@link AffectedStopPointStructure.ConnectionLinks }
*
*/
public void setConnectionLinks(AffectedStopPointStructure.ConnectionLinks value) {
this.connectionLinks = value;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedConnectionLink" type="{http://www.siri.org.uk/siri}AffectedConnectionLinkStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"affectedConnectionLinks"
})
public static class ConnectionLinks
implements Serializable
{
@XmlElement(name = "AffectedConnectionLink", required = true)
protected List affectedConnectionLinks;
/**
* Gets the value of the affectedConnectionLinks property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the affectedConnectionLinks property.
*
*
* For example, to add a new item, do as follows:
*
* getAffectedConnectionLinks().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedConnectionLinkStructure }
*
*
*/
public List getAffectedConnectionLinks() {
if (affectedConnectionLinks == null) {
affectedConnectionLinks = new ArrayList();
}
return this.affectedConnectionLinks;
}
}
}