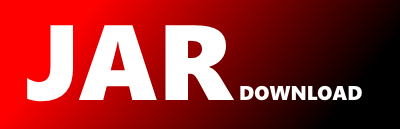
uk.org.siri.siri20.FacilityLocationStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import uk.org.ifopt.siri20.StopPlaceComponentRefStructure;
import uk.org.ifopt.siri20.StopPlaceRef;
/**
* Location of the MONITORED FACILITY.
*
* Java class for FacilityLocationStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FacilityLocationStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}FacilityScheduleRefGroup"/>
* <group ref="{http://www.siri.org.uk/siri}FacilityStopPlaceRefGroup"/>
* <group ref="{http://www.siri.org.uk/siri}ServiceInfoGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FacilityLocationStructure", propOrder = {
"lineRef",
"stopPointRef",
"vehicleRef",
"datedVehicleJourneyRef",
"connectionLinkRef",
"interchangeRef",
"stopPlaceRef",
"stopPlaceComponentId",
"operatorRef",
"productCategoryRef",
"serviceFeatureReves",
"vehicleFeatureReves"
})
public class FacilityLocationStructure
implements Serializable
{
@XmlElement(name = "LineRef")
protected LineRef lineRef;
@XmlElement(name = "StopPointRef")
protected StopPointRef stopPointRef;
@XmlElement(name = "VehicleRef")
protected VehicleRef vehicleRef;
@XmlElement(name = "DatedVehicleJourneyRef")
protected DatedVehicleJourneyRef datedVehicleJourneyRef;
@XmlElement(name = "ConnectionLinkRef")
protected ConnectionLinkRef connectionLinkRef;
@XmlElement(name = "InterchangeRef")
protected InterchangeRef interchangeRef;
@XmlElement(name = "StopPlaceRef")
protected StopPlaceRef stopPlaceRef;
@XmlElement(name = "StopPlaceComponentId")
protected StopPlaceComponentRefStructure stopPlaceComponentId;
@XmlElement(name = "OperatorRef")
protected OperatorRefStructure operatorRef;
@XmlElement(name = "ProductCategoryRef")
protected ProductCategoryRefStructure productCategoryRef;
@XmlElement(name = "ServiceFeatureRef")
protected List serviceFeatureReves;
@XmlElement(name = "VehicleFeatureRef")
protected List vehicleFeatureReves;
/**
* Gets the value of the lineRef property.
*
* @return
* possible object is
* {@link LineRef }
*
*/
public LineRef getLineRef() {
return lineRef;
}
/**
* Sets the value of the lineRef property.
*
* @param value
* allowed object is
* {@link LineRef }
*
*/
public void setLineRef(LineRef value) {
this.lineRef = value;
}
/**
* Gets the value of the stopPointRef property.
*
* @return
* possible object is
* {@link StopPointRef }
*
*/
public StopPointRef getStopPointRef() {
return stopPointRef;
}
/**
* Sets the value of the stopPointRef property.
*
* @param value
* allowed object is
* {@link StopPointRef }
*
*/
public void setStopPointRef(StopPointRef value) {
this.stopPointRef = value;
}
/**
* Gets the value of the vehicleRef property.
*
* @return
* possible object is
* {@link VehicleRef }
*
*/
public VehicleRef getVehicleRef() {
return vehicleRef;
}
/**
* Sets the value of the vehicleRef property.
*
* @param value
* allowed object is
* {@link VehicleRef }
*
*/
public void setVehicleRef(VehicleRef value) {
this.vehicleRef = value;
}
/**
* Gets the value of the datedVehicleJourneyRef property.
*
* @return
* possible object is
* {@link DatedVehicleJourneyRef }
*
*/
public DatedVehicleJourneyRef getDatedVehicleJourneyRef() {
return datedVehicleJourneyRef;
}
/**
* Sets the value of the datedVehicleJourneyRef property.
*
* @param value
* allowed object is
* {@link DatedVehicleJourneyRef }
*
*/
public void setDatedVehicleJourneyRef(DatedVehicleJourneyRef value) {
this.datedVehicleJourneyRef = value;
}
/**
* Gets the value of the connectionLinkRef property.
*
* @return
* possible object is
* {@link ConnectionLinkRef }
*
*/
public ConnectionLinkRef getConnectionLinkRef() {
return connectionLinkRef;
}
/**
* Sets the value of the connectionLinkRef property.
*
* @param value
* allowed object is
* {@link ConnectionLinkRef }
*
*/
public void setConnectionLinkRef(ConnectionLinkRef value) {
this.connectionLinkRef = value;
}
/**
* Gets the value of the interchangeRef property.
*
* @return
* possible object is
* {@link InterchangeRef }
*
*/
public InterchangeRef getInterchangeRef() {
return interchangeRef;
}
/**
* Sets the value of the interchangeRef property.
*
* @param value
* allowed object is
* {@link InterchangeRef }
*
*/
public void setInterchangeRef(InterchangeRef value) {
this.interchangeRef = value;
}
/**
* Gets the value of the stopPlaceRef property.
*
* @return
* possible object is
* {@link StopPlaceRef }
*
*/
public StopPlaceRef getStopPlaceRef() {
return stopPlaceRef;
}
/**
* Sets the value of the stopPlaceRef property.
*
* @param value
* allowed object is
* {@link StopPlaceRef }
*
*/
public void setStopPlaceRef(StopPlaceRef value) {
this.stopPlaceRef = value;
}
/**
* Gets the value of the stopPlaceComponentId property.
*
* @return
* possible object is
* {@link StopPlaceComponentRefStructure }
*
*/
public StopPlaceComponentRefStructure getStopPlaceComponentId() {
return stopPlaceComponentId;
}
/**
* Sets the value of the stopPlaceComponentId property.
*
* @param value
* allowed object is
* {@link StopPlaceComponentRefStructure }
*
*/
public void setStopPlaceComponentId(StopPlaceComponentRefStructure value) {
this.stopPlaceComponentId = value;
}
/**
* Gets the value of the operatorRef property.
*
* @return
* possible object is
* {@link OperatorRefStructure }
*
*/
public OperatorRefStructure getOperatorRef() {
return operatorRef;
}
/**
* Sets the value of the operatorRef property.
*
* @param value
* allowed object is
* {@link OperatorRefStructure }
*
*/
public void setOperatorRef(OperatorRefStructure value) {
this.operatorRef = value;
}
/**
* Gets the value of the productCategoryRef property.
*
* @return
* possible object is
* {@link ProductCategoryRefStructure }
*
*/
public ProductCategoryRefStructure getProductCategoryRef() {
return productCategoryRef;
}
/**
* Sets the value of the productCategoryRef property.
*
* @param value
* allowed object is
* {@link ProductCategoryRefStructure }
*
*/
public void setProductCategoryRef(ProductCategoryRefStructure value) {
this.productCategoryRef = value;
}
/**
* Classification of service into arbitrary Service categories, e.g. school bus. Recommended SIRI values based on TPEG are given in SIRI documentation and enumerated in the siri_facilities package.
* Corresponds to NeTEX TYPE OF SERVICe.Gets the value of the serviceFeatureReves property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the serviceFeatureReves property.
*
*
* For example, to add a new item, do as follows:
*
* getServiceFeatureReves().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ServiceFeatureRef }
*
*
*/
public List getServiceFeatureReves() {
if (serviceFeatureReves == null) {
serviceFeatureReves = new ArrayList();
}
return this.serviceFeatureReves;
}
/**
* Gets the value of the vehicleFeatureReves property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vehicleFeatureReves property.
*
*
* For example, to add a new item, do as follows:
*
* getVehicleFeatureReves().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VehicleFeatureRefStructure }
*
*
*/
public List getVehicleFeatureReves() {
if (vehicleFeatureReves == null) {
vehicleFeatureReves = new ArrayList();
}
return this.vehicleFeatureReves;
}
}