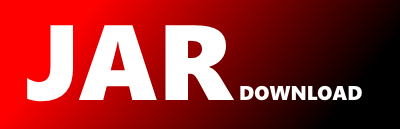
uk.org.siri.siri20.FacilityStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import uk.org.acbs.siri20.AccessibilityAssessmentStructure;
import uk.org.acbs.siri20.AccessibilityStructure;
import uk.org.acbs.siri20.SuitabilityStructure;
/**
* Type for sescription the MONITORED FACILITY itself.
*
* Java class for FacilityStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FacilityStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="FacilityCode" type="{http://www.siri.org.uk/siri}FacilityCodeType" minOccurs="0"/>
* <element name="Description" type="{http://www.siri.org.uk/siri}NaturalLanguageStringStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FacilityClass" type="{http://www.siri.org.uk/siri}FacilityCategoryEnumeration" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Features" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Feature" type="{http://www.siri.org.uk/siri}AllFacilitiesFeatureStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="OwnerRef" type="{http://www.siri.org.uk/siri}OrganisationRefStructure" minOccurs="0"/>
* <element name="OwnerName" type="{http://www.siri.org.uk/siri}NaturalLanguageStringStructure" minOccurs="0"/>
* <element name="ValidityCondition" type="{http://www.siri.org.uk/siri}MonitoringValidityConditionStructure" minOccurs="0"/>
* <element name="FacilityLocation" type="{http://www.siri.org.uk/siri}FacilityLocationStructure" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}FacilityAccessibilityGroup"/>
* <element name="AccessibilityAssessment" type="{http://www.ifopt.org.uk/acsb}AccessibilityAssessmentStructure" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FacilityStructure", propOrder = {
"facilityCode",
"descriptions",
"facilityClasses",
"features",
"ownerRef",
"ownerName",
"validityCondition",
"facilityLocation",
"limitations",
"suitabilities",
"accessibilityAssessment",
"extensions"
})
public class FacilityStructure
implements Serializable
{
@XmlElement(name = "FacilityCode")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String facilityCode;
@XmlElement(name = "Description")
protected List descriptions;
@XmlElement(name = "FacilityClass")
@XmlSchemaType(name = "NMTOKEN")
protected List facilityClasses;
@XmlElement(name = "Features")
protected FacilityStructure.Features features;
@XmlElement(name = "OwnerRef")
protected OrganisationRefStructure ownerRef;
@XmlElement(name = "OwnerName")
protected NaturalLanguageStringStructure ownerName;
@XmlElement(name = "ValidityCondition")
protected MonitoringValidityConditionStructure validityCondition;
@XmlElement(name = "FacilityLocation")
protected FacilityLocationStructure facilityLocation;
@XmlElement(name = "Limitations")
protected FacilityStructure.Limitations limitations;
@XmlElement(name = "Suitabilities")
protected FacilityStructure.Suitabilities suitabilities;
@XmlElement(name = "AccessibilityAssessment")
protected AccessibilityAssessmentStructure accessibilityAssessment;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the facilityCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFacilityCode() {
return facilityCode;
}
/**
* Sets the value of the facilityCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFacilityCode(String value) {
this.facilityCode = value;
}
/**
* Gets the value of the descriptions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the descriptions property.
*
*
* For example, to add a new item, do as follows:
*
* getDescriptions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getDescriptions() {
if (descriptions == null) {
descriptions = new ArrayList();
}
return this.descriptions;
}
/**
* Gets the value of the facilityClasses property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the facilityClasses property.
*
*
* For example, to add a new item, do as follows:
*
* getFacilityClasses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FacilityCategoryEnumeration }
*
*
*/
public List getFacilityClasses() {
if (facilityClasses == null) {
facilityClasses = new ArrayList();
}
return this.facilityClasses;
}
/**
* Gets the value of the features property.
*
* @return
* possible object is
* {@link FacilityStructure.Features }
*
*/
public FacilityStructure.Features getFeatures() {
return features;
}
/**
* Sets the value of the features property.
*
* @param value
* allowed object is
* {@link FacilityStructure.Features }
*
*/
public void setFeatures(FacilityStructure.Features value) {
this.features = value;
}
/**
* Gets the value of the ownerRef property.
*
* @return
* possible object is
* {@link OrganisationRefStructure }
*
*/
public OrganisationRefStructure getOwnerRef() {
return ownerRef;
}
/**
* Sets the value of the ownerRef property.
*
* @param value
* allowed object is
* {@link OrganisationRefStructure }
*
*/
public void setOwnerRef(OrganisationRefStructure value) {
this.ownerRef = value;
}
/**
* Gets the value of the ownerName property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getOwnerName() {
return ownerName;
}
/**
* Sets the value of the ownerName property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setOwnerName(NaturalLanguageStringStructure value) {
this.ownerName = value;
}
/**
* Gets the value of the validityCondition property.
*
* @return
* possible object is
* {@link MonitoringValidityConditionStructure }
*
*/
public MonitoringValidityConditionStructure getValidityCondition() {
return validityCondition;
}
/**
* Sets the value of the validityCondition property.
*
* @param value
* allowed object is
* {@link MonitoringValidityConditionStructure }
*
*/
public void setValidityCondition(MonitoringValidityConditionStructure value) {
this.validityCondition = value;
}
/**
* Gets the value of the facilityLocation property.
*
* @return
* possible object is
* {@link FacilityLocationStructure }
*
*/
public FacilityLocationStructure getFacilityLocation() {
return facilityLocation;
}
/**
* Sets the value of the facilityLocation property.
*
* @param value
* allowed object is
* {@link FacilityLocationStructure }
*
*/
public void setFacilityLocation(FacilityLocationStructure value) {
this.facilityLocation = value;
}
/**
* Gets the value of the limitations property.
*
* @return
* possible object is
* {@link FacilityStructure.Limitations }
*
*/
public FacilityStructure.Limitations getLimitations() {
return limitations;
}
/**
* Sets the value of the limitations property.
*
* @param value
* allowed object is
* {@link FacilityStructure.Limitations }
*
*/
public void setLimitations(FacilityStructure.Limitations value) {
this.limitations = value;
}
/**
* Gets the value of the suitabilities property.
*
* @return
* possible object is
* {@link FacilityStructure.Suitabilities }
*
*/
public FacilityStructure.Suitabilities getSuitabilities() {
return suitabilities;
}
/**
* Sets the value of the suitabilities property.
*
* @param value
* allowed object is
* {@link FacilityStructure.Suitabilities }
*
*/
public void setSuitabilities(FacilityStructure.Suitabilities value) {
this.suitabilities = value;
}
/**
* Gets the value of the accessibilityAssessment property.
*
* @return
* possible object is
* {@link AccessibilityAssessmentStructure }
*
*/
public AccessibilityAssessmentStructure getAccessibilityAssessment() {
return accessibilityAssessment;
}
/**
* Sets the value of the accessibilityAssessment property.
*
* @param value
* allowed object is
* {@link AccessibilityAssessmentStructure }
*
*/
public void setAccessibilityAssessment(AccessibilityAssessmentStructure value) {
this.accessibilityAssessment = value;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Feature" type="{http://www.siri.org.uk/siri}AllFacilitiesFeatureStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"features"
})
public static class Features
implements Serializable
{
@XmlElement(name = "Feature", required = true)
protected List features;
/**
* Gets the value of the features property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the features property.
*
*
* For example, to add a new item, do as follows:
*
* getFeatures().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AllFacilitiesFeatureStructure }
*
*
*/
public List getFeatures() {
if (features == null) {
features = new ArrayList();
}
return this.features;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{http://www.ifopt.org.uk/acsb}MobilityLimitationGroup"/>
* <group ref="{http://www.ifopt.org.uk/acsb}SensoryLimitationGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"wheelchairAccess",
"stepFreeAccess",
"escalatorFreeAccess",
"liftFreeAccess",
"audibleSignalsAvailable",
"visualSignsAvailable"
})
public static class Limitations
implements Serializable
{
@XmlElement(name = "WheelchairAccess", namespace = "http://www.ifopt.org.uk/acsb", required = true, defaultValue = "false")
protected AccessibilityStructure wheelchairAccess;
@XmlElement(name = "StepFreeAccess", namespace = "http://www.ifopt.org.uk/acsb", defaultValue = "unknown")
protected AccessibilityStructure stepFreeAccess;
@XmlElement(name = "EscalatorFreeAccess", namespace = "http://www.ifopt.org.uk/acsb", defaultValue = "unknown")
protected AccessibilityStructure escalatorFreeAccess;
@XmlElement(name = "LiftFreeAccess", namespace = "http://www.ifopt.org.uk/acsb", defaultValue = "unknown")
protected AccessibilityStructure liftFreeAccess;
@XmlElement(name = "AudibleSignalsAvailable", namespace = "http://www.ifopt.org.uk/acsb", defaultValue = "false")
protected AccessibilityStructure audibleSignalsAvailable;
@XmlElement(name = "VisualSignsAvailable", namespace = "http://www.ifopt.org.uk/acsb", defaultValue = "unknown")
protected AccessibilityStructure visualSignsAvailable;
/**
* Gets the value of the wheelchairAccess property.
*
* @return
* possible object is
* {@link AccessibilityStructure }
*
*/
public AccessibilityStructure getWheelchairAccess() {
return wheelchairAccess;
}
/**
* Sets the value of the wheelchairAccess property.
*
* @param value
* allowed object is
* {@link AccessibilityStructure }
*
*/
public void setWheelchairAccess(AccessibilityStructure value) {
this.wheelchairAccess = value;
}
/**
* Gets the value of the stepFreeAccess property.
*
* @return
* possible object is
* {@link AccessibilityStructure }
*
*/
public AccessibilityStructure getStepFreeAccess() {
return stepFreeAccess;
}
/**
* Sets the value of the stepFreeAccess property.
*
* @param value
* allowed object is
* {@link AccessibilityStructure }
*
*/
public void setStepFreeAccess(AccessibilityStructure value) {
this.stepFreeAccess = value;
}
/**
* Gets the value of the escalatorFreeAccess property.
*
* @return
* possible object is
* {@link AccessibilityStructure }
*
*/
public AccessibilityStructure getEscalatorFreeAccess() {
return escalatorFreeAccess;
}
/**
* Sets the value of the escalatorFreeAccess property.
*
* @param value
* allowed object is
* {@link AccessibilityStructure }
*
*/
public void setEscalatorFreeAccess(AccessibilityStructure value) {
this.escalatorFreeAccess = value;
}
/**
* Gets the value of the liftFreeAccess property.
*
* @return
* possible object is
* {@link AccessibilityStructure }
*
*/
public AccessibilityStructure getLiftFreeAccess() {
return liftFreeAccess;
}
/**
* Sets the value of the liftFreeAccess property.
*
* @param value
* allowed object is
* {@link AccessibilityStructure }
*
*/
public void setLiftFreeAccess(AccessibilityStructure value) {
this.liftFreeAccess = value;
}
/**
* Whether a PLACE / SITE ELEMENT has Audible signals for the viusally impaired.
*
* @return
* possible object is
* {@link AccessibilityStructure }
*
*/
public AccessibilityStructure getAudibleSignalsAvailable() {
return audibleSignalsAvailable;
}
/**
* Sets the value of the audibleSignalsAvailable property.
*
* @param value
* allowed object is
* {@link AccessibilityStructure }
*
*/
public void setAudibleSignalsAvailable(AccessibilityStructure value) {
this.audibleSignalsAvailable = value;
}
/**
* Whether a PLACE / SITE ELEMENT has Visual signals for the hearing impaired.
*
* @return
* possible object is
* {@link AccessibilityStructure }
*
*/
public AccessibilityStructure getVisualSignsAvailable() {
return visualSignsAvailable;
}
/**
* Sets the value of the visualSignsAvailable property.
*
* @param value
* allowed object is
* {@link AccessibilityStructure }
*
*/
public void setVisualSignsAvailable(AccessibilityStructure value) {
this.visualSignsAvailable = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Suitability" type="{http://www.ifopt.org.uk/acsb}SuitabilityStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"suitabilities"
})
public static class Suitabilities
implements Serializable
{
@XmlElement(name = "Suitability", required = true)
protected List suitabilities;
/**
* Gets the value of the suitabilities property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the suitabilities property.
*
*
* For example, to add a new item, do as follows:
*
* getSuitabilities().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SuitabilityStructure }
*
*
*/
public List getSuitabilities() {
if (suitabilities == null) {
suitabilities = new ArrayList();
}
return this.suitabilities;
}
}
}