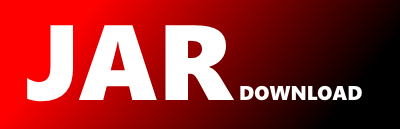
uk.org.siri.siri20.OnwardCallStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.math.BigInteger;
import java.time.Duration;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.entur.siri.adapter.DurationXmlAdapter;
import org.w3._2001.xmlschema.Adapter1;
/**
* Type Onwards CALLs at stop.
*
* Java class for OnwardCallStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="OnwardCallStructure">
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}AbstractMonitoredCallStructure">
* <sequence>
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}VehicleAtStop" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}TimingPoint" minOccurs="0"/>
* </sequence>
* <group ref="{http://www.siri.org.uk/siri}OnwardsCallGroup"/>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "OnwardCallStructure", propOrder = {
"vehicleAtStop",
"timingPoint",
"aimedArrivalTime",
"expectedArrivalTime",
"expectedArrivalPredictionQuality",
"arrivalStatus",
"arrivalProximityText",
"arrivalPlatformName",
"arrivalBoardingActivity",
"arrivalStopAssignment",
"arrivalOperatorRefs",
"aimedDepartureTime",
"expectedDepartureTime",
"provisionalExpectedDepartureTime",
"earliestExpectedDepartureTime",
"expectedDeparturePredictionQuality",
"aimedLatestPassengerAccessTime",
"expectedLatestPassengerAccessTime",
"departureStatus",
"departureProximityText",
"departurePlatformName",
"departureBoardingActivity",
"departureStopAssignment",
"departureOperatorRefs",
"aimedHeadwayInterval",
"expectedHeadwayInterval",
"distanceFromStop",
"numberOfStopsAway",
"extensions"
})
public class OnwardCallStructure
extends AbstractMonitoredCallStructure
implements Serializable
{
@XmlElement(name = "VehicleAtStop", defaultValue = "false")
protected Boolean vehicleAtStop;
@XmlElement(name = "TimingPoint", defaultValue = "true")
protected Boolean timingPoint;
@XmlElement(name = "AimedArrivalTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime aimedArrivalTime;
@XmlElement(name = "ExpectedArrivalTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime expectedArrivalTime;
@XmlElement(name = "ExpectedArrivalPredictionQuality")
protected PredictionQualityStructure expectedArrivalPredictionQuality;
@XmlElement(name = "ArrivalStatus")
@XmlSchemaType(name = "NMTOKEN")
protected CallStatusEnumeration arrivalStatus;
@XmlElement(name = "ArrivalProximityText")
protected NaturalLanguageStringStructure arrivalProximityText;
@XmlElement(name = "ArrivalPlatformName")
protected NaturalLanguageStringStructure arrivalPlatformName;
@XmlElement(name = "ArrivalBoardingActivity", defaultValue = "alighting")
@XmlSchemaType(name = "NMTOKEN")
protected ArrivalBoardingActivityEnumeration arrivalBoardingActivity;
@XmlElement(name = "ArrivalStopAssignment")
protected StopAssignmentStructure arrivalStopAssignment;
@XmlElement(name = "ArrivalOperatorRefs")
protected List arrivalOperatorRefs;
@XmlElement(name = "AimedDepartureTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime aimedDepartureTime;
@XmlElement(name = "ExpectedDepartureTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime expectedDepartureTime;
@XmlElement(name = "ProvisionalExpectedDepartureTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime provisionalExpectedDepartureTime;
@XmlElement(name = "EarliestExpectedDepartureTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime earliestExpectedDepartureTime;
@XmlElement(name = "ExpectedDeparturePredictionQuality")
protected PredictionQualityStructure expectedDeparturePredictionQuality;
@XmlElement(name = "AimedLatestPassengerAccessTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime aimedLatestPassengerAccessTime;
@XmlElement(name = "ExpectedLatestPassengerAccessTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime expectedLatestPassengerAccessTime;
@XmlElement(name = "DepartureStatus")
@XmlSchemaType(name = "NMTOKEN")
protected CallStatusEnumeration departureStatus;
@XmlElement(name = "DepartureProximityText")
protected NaturalLanguageStringStructure departureProximityText;
@XmlElement(name = "DeparturePlatformName")
protected NaturalLanguageStringStructure departurePlatformName;
@XmlElement(name = "DepartureBoardingActivity", defaultValue = "boarding")
@XmlSchemaType(name = "NMTOKEN")
protected DepartureBoardingActivityEnumeration departureBoardingActivity;
@XmlElement(name = "DepartureStopAssignment")
protected StopAssignmentStructure departureStopAssignment;
@XmlElement(name = "DepartureOperatorRefs")
protected List departureOperatorRefs;
@XmlElement(name = "AimedHeadwayInterval", type = String.class)
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration aimedHeadwayInterval;
@XmlElement(name = "ExpectedHeadwayInterval", type = String.class)
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration expectedHeadwayInterval;
@XmlElement(name = "DistanceFromStop")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger distanceFromStop;
@XmlElement(name = "NumberOfStopsAway")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger numberOfStopsAway;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the vehicleAtStop property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isVehicleAtStop() {
return vehicleAtStop;
}
/**
* Sets the value of the vehicleAtStop property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setVehicleAtStop(Boolean value) {
this.vehicleAtStop = value;
}
/**
* Gets the value of the timingPoint property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTimingPoint() {
return timingPoint;
}
/**
* Sets the value of the timingPoint property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTimingPoint(Boolean value) {
this.timingPoint = value;
}
/**
* Gets the value of the aimedArrivalTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getAimedArrivalTime() {
return aimedArrivalTime;
}
/**
* Sets the value of the aimedArrivalTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAimedArrivalTime(ZonedDateTime value) {
this.aimedArrivalTime = value;
}
/**
* Gets the value of the expectedArrivalTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getExpectedArrivalTime() {
return expectedArrivalTime;
}
/**
* Sets the value of the expectedArrivalTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExpectedArrivalTime(ZonedDateTime value) {
this.expectedArrivalTime = value;
}
/**
* Gets the value of the expectedArrivalPredictionQuality property.
*
* @return
* possible object is
* {@link PredictionQualityStructure }
*
*/
public PredictionQualityStructure getExpectedArrivalPredictionQuality() {
return expectedArrivalPredictionQuality;
}
/**
* Sets the value of the expectedArrivalPredictionQuality property.
*
* @param value
* allowed object is
* {@link PredictionQualityStructure }
*
*/
public void setExpectedArrivalPredictionQuality(PredictionQualityStructure value) {
this.expectedArrivalPredictionQuality = value;
}
/**
* Gets the value of the arrivalStatus property.
*
* @return
* possible object is
* {@link CallStatusEnumeration }
*
*/
public CallStatusEnumeration getArrivalStatus() {
return arrivalStatus;
}
/**
* Sets the value of the arrivalStatus property.
*
* @param value
* allowed object is
* {@link CallStatusEnumeration }
*
*/
public void setArrivalStatus(CallStatusEnumeration value) {
this.arrivalStatus = value;
}
/**
* Gets the value of the arrivalProximityText property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getArrivalProximityText() {
return arrivalProximityText;
}
/**
* Sets the value of the arrivalProximityText property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setArrivalProximityText(NaturalLanguageStringStructure value) {
this.arrivalProximityText = value;
}
/**
* Bay or platform (QUAY) name to show passenger i.e. expected platform for vehicel to arrive at.Inheritable property. Can be omitted if the same as the DeparturePlatformName If there no arrival platform name separate from the departure platform name, the precedence is
* (i) any arrival platform on any related dated timetable element,
* (ii) any departure platform name on this estimated element;
* (iii) any departure platform name on any related dated timetable CALL.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getArrivalPlatformName() {
return arrivalPlatformName;
}
/**
* Sets the value of the arrivalPlatformName property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setArrivalPlatformName(NaturalLanguageStringStructure value) {
this.arrivalPlatformName = value;
}
/**
* Nature of boarding and alighting allowed at stop. Default is 'Alighting'.
*
* @return
* possible object is
* {@link ArrivalBoardingActivityEnumeration }
*
*/
public ArrivalBoardingActivityEnumeration getArrivalBoardingActivity() {
return arrivalBoardingActivity;
}
/**
* Sets the value of the arrivalBoardingActivity property.
*
* @param value
* allowed object is
* {@link ArrivalBoardingActivityEnumeration }
*
*/
public void setArrivalBoardingActivity(ArrivalBoardingActivityEnumeration value) {
this.arrivalBoardingActivity = value;
}
/**
* Gets the value of the arrivalStopAssignment property.
*
* @return
* possible object is
* {@link StopAssignmentStructure }
*
*/
public StopAssignmentStructure getArrivalStopAssignment() {
return arrivalStopAssignment;
}
/**
* Sets the value of the arrivalStopAssignment property.
*
* @param value
* allowed object is
* {@link StopAssignmentStructure }
*
*/
public void setArrivalStopAssignment(StopAssignmentStructure value) {
this.arrivalStopAssignment = value;
}
/**
* Gets the value of the arrivalOperatorRefs property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the arrivalOperatorRefs property.
*
*
* For example, to add a new item, do as follows:
*
* getArrivalOperatorRefs().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OperatorRefStructure }
*
*
*/
public List getArrivalOperatorRefs() {
if (arrivalOperatorRefs == null) {
arrivalOperatorRefs = new ArrayList();
}
return this.arrivalOperatorRefs;
}
/**
* Target departure time of VEHICLE according to latest working timetable.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getAimedDepartureTime() {
return aimedDepartureTime;
}
/**
* Sets the value of the aimedDepartureTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAimedDepartureTime(ZonedDateTime value) {
this.aimedDepartureTime = value;
}
/**
* Estimated time of departure of VEHICLE, most likely taking into account all control actions such as waiting.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getExpectedDepartureTime() {
return expectedDepartureTime;
}
/**
* Sets the value of the expectedDepartureTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExpectedDepartureTime(ZonedDateTime value) {
this.expectedDepartureTime = value;
}
/**
* Gets the value of the provisionalExpectedDepartureTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getProvisionalExpectedDepartureTime() {
return provisionalExpectedDepartureTime;
}
/**
* Sets the value of the provisionalExpectedDepartureTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProvisionalExpectedDepartureTime(ZonedDateTime value) {
this.provisionalExpectedDepartureTime = value;
}
/**
* Gets the value of the earliestExpectedDepartureTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getEarliestExpectedDepartureTime() {
return earliestExpectedDepartureTime;
}
/**
* Sets the value of the earliestExpectedDepartureTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEarliestExpectedDepartureTime(ZonedDateTime value) {
this.earliestExpectedDepartureTime = value;
}
/**
* Gets the value of the expectedDeparturePredictionQuality property.
*
* @return
* possible object is
* {@link PredictionQualityStructure }
*
*/
public PredictionQualityStructure getExpectedDeparturePredictionQuality() {
return expectedDeparturePredictionQuality;
}
/**
* Sets the value of the expectedDeparturePredictionQuality property.
*
* @param value
* allowed object is
* {@link PredictionQualityStructure }
*
*/
public void setExpectedDeparturePredictionQuality(PredictionQualityStructure value) {
this.expectedDeparturePredictionQuality = value;
}
/**
* Target Latest time at which a PASSENGER should aim to arrive at the STOP PLACE containing the stop. This time may be earlier than the VEHICLE departure times as itmay include time for processes such as checkin, security, etc.(As specified by CHECK CONSTRAINT DELAYs in the underlying data) If absent assume to be the same as Earliest expected departure time, +SIRI v2.0
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getAimedLatestPassengerAccessTime() {
return aimedLatestPassengerAccessTime;
}
/**
* Sets the value of the aimedLatestPassengerAccessTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAimedLatestPassengerAccessTime(ZonedDateTime value) {
this.aimedLatestPassengerAccessTime = value;
}
/**
* Expected Latest time at which a PASSENGER should aim to arrive at the STOP PLACE containing the stop. This time may be earlier than the VEHICLE departure times as it may include time for processes such as checkin, security, etc.(As specified by CHECK CONSTRAINT DELAYs in the underlying data) If absent assume to be the same as Earliest expected departure time, +SIRI v2.0
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getExpectedLatestPassengerAccessTime() {
return expectedLatestPassengerAccessTime;
}
/**
* Sets the value of the expectedLatestPassengerAccessTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExpectedLatestPassengerAccessTime(ZonedDateTime value) {
this.expectedLatestPassengerAccessTime = value;
}
/**
* Gets the value of the departureStatus property.
*
* @return
* possible object is
* {@link CallStatusEnumeration }
*
*/
public CallStatusEnumeration getDepartureStatus() {
return departureStatus;
}
/**
* Sets the value of the departureStatus property.
*
* @param value
* allowed object is
* {@link CallStatusEnumeration }
*
*/
public void setDepartureStatus(CallStatusEnumeration value) {
this.departureStatus = value;
}
/**
* Gets the value of the departureProximityText property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getDepartureProximityText() {
return departureProximityText;
}
/**
* Sets the value of the departureProximityText property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setDepartureProximityText(NaturalLanguageStringStructure value) {
this.departureProximityText = value;
}
/**
* Departure QUAY ( Bay or platform) name. Defaulted taken from from planned timetable..
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getDeparturePlatformName() {
return departurePlatformName;
}
/**
* Sets the value of the departurePlatformName property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setDeparturePlatformName(NaturalLanguageStringStructure value) {
this.departurePlatformName = value;
}
/**
* Gets the value of the departureBoardingActivity property.
*
* @return
* possible object is
* {@link DepartureBoardingActivityEnumeration }
*
*/
public DepartureBoardingActivityEnumeration getDepartureBoardingActivity() {
return departureBoardingActivity;
}
/**
* Sets the value of the departureBoardingActivity property.
*
* @param value
* allowed object is
* {@link DepartureBoardingActivityEnumeration }
*
*/
public void setDepartureBoardingActivity(DepartureBoardingActivityEnumeration value) {
this.departureBoardingActivity = value;
}
/**
* Gets the value of the departureStopAssignment property.
*
* @return
* possible object is
* {@link StopAssignmentStructure }
*
*/
public StopAssignmentStructure getDepartureStopAssignment() {
return departureStopAssignment;
}
/**
* Sets the value of the departureStopAssignment property.
*
* @param value
* allowed object is
* {@link StopAssignmentStructure }
*
*/
public void setDepartureStopAssignment(StopAssignmentStructure value) {
this.departureStopAssignment = value;
}
/**
* Gets the value of the departureOperatorRefs property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the departureOperatorRefs property.
*
*
* For example, to add a new item, do as follows:
*
* getDepartureOperatorRefs().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OperatorRefStructure }
*
*
*/
public List getDepartureOperatorRefs() {
if (departureOperatorRefs == null) {
departureOperatorRefs = new ArrayList();
}
return this.departureOperatorRefs;
}
/**
* Gets the value of the aimedHeadwayInterval property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getAimedHeadwayInterval() {
return aimedHeadwayInterval;
}
/**
* Sets the value of the aimedHeadwayInterval property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAimedHeadwayInterval(Duration value) {
this.aimedHeadwayInterval = value;
}
/**
* Gets the value of the expectedHeadwayInterval property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getExpectedHeadwayInterval() {
return expectedHeadwayInterval;
}
/**
* Sets the value of the expectedHeadwayInterval property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExpectedHeadwayInterval(Duration value) {
this.expectedHeadwayInterval = value;
}
/**
* Gets the value of the distanceFromStop property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getDistanceFromStop() {
return distanceFromStop;
}
/**
* Sets the value of the distanceFromStop property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setDistanceFromStop(BigInteger value) {
this.distanceFromStop = value;
}
/**
* Gets the value of the numberOfStopsAway property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getNumberOfStopsAway() {
return numberOfStopsAway;
}
/**
* Sets the value of the numberOfStopsAway property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setNumberOfStopsAway(BigInteger value) {
this.numberOfStopsAway = value;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
}