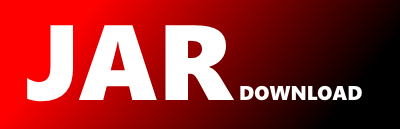
uk.org.siri.siri20.OptionalTrafficElementStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.math.BigInteger;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.List;
import eu.datex2.siri20.schema._2_0rc1._2_0.Cause;
import eu.datex2.siri20.schema._2_0rc1._2_0.Comment;
import eu.datex2.siri20.schema._2_0rc1._2_0.ExtensionType;
import eu.datex2.siri20.schema._2_0rc1._2_0.GroupOfLocations;
import eu.datex2.siri20.schema._2_0rc1._2_0.Impact;
import eu.datex2.siri20.schema._2_0rc1._2_0.Management;
import eu.datex2.siri20.schema._2_0rc1._2_0.ProbabilityOfOccurrenceEnum;
import eu.datex2.siri20.schema._2_0rc1._2_0.Source;
import eu.datex2.siri20.schema._2_0rc1._2_0.Validity;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.w3._2001.xmlschema.Adapter1;
/**
* An event which is not planned by the traffic OPERATOR, which is affecting, or has the potential to affect traffic flow. This SIRI-SX element embeds the Datex2 TrafficElement, making all elements optional because they may alternatvielky be specified by common SIRI-SRX SITUATION elements.
*
* Java class for OptionalTrafficElementStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="OptionalTrafficElementStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}Datex2ManagementGroup"/>
* <group ref="{http://www.siri.org.uk/siri}Datex2SituationGroup"/>
* <group ref="{http://www.siri.org.uk/siri}Datex2RoadGroup"/>
* <group ref="{http://www.siri.org.uk/siri}Datex2OtherGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "OptionalTrafficElementStructure", propOrder = {
"situationRecordCreationReference",
"situationRecordCreationTime",
"situationRecordObservationTime",
"situationRecordVersion",
"situationRecordVersionTime",
"situationRecordFirstSupplierVersionTime",
"probabilityOfOccurrence",
"source",
"validity",
"impact",
"cause",
"generalPublicComments",
"nonGeneralPublicComments",
"groupOfLocations",
"management",
"situationRecordExtension",
"trafficElementExtension"
})
public abstract class OptionalTrafficElementStructure
implements Serializable
{
@XmlElement(required = true)
protected String situationRecordCreationReference;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime situationRecordCreationTime;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime situationRecordObservationTime;
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger situationRecordVersion;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime situationRecordVersionTime;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime situationRecordFirstSupplierVersionTime;
@XmlSchemaType(name = "string")
protected ProbabilityOfOccurrenceEnum probabilityOfOccurrence;
@XmlElement(name = "Source")
protected Source source;
protected Validity validity;
protected Impact impact;
protected Cause cause;
@XmlElement(name = "generalPublicComment")
protected List generalPublicComments;
@XmlElement(name = "nonGeneralPublicComment")
protected List nonGeneralPublicComments;
protected GroupOfLocations groupOfLocations;
protected Management management;
protected ExtensionType situationRecordExtension;
protected ExtensionType trafficElementExtension;
/**
* Gets the value of the situationRecordCreationReference property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSituationRecordCreationReference() {
return situationRecordCreationReference;
}
/**
* Sets the value of the situationRecordCreationReference property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSituationRecordCreationReference(String value) {
this.situationRecordCreationReference = value;
}
/**
* Gets the value of the situationRecordCreationTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getSituationRecordCreationTime() {
return situationRecordCreationTime;
}
/**
* Sets the value of the situationRecordCreationTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSituationRecordCreationTime(ZonedDateTime value) {
this.situationRecordCreationTime = value;
}
/**
* Gets the value of the situationRecordObservationTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getSituationRecordObservationTime() {
return situationRecordObservationTime;
}
/**
* Sets the value of the situationRecordObservationTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSituationRecordObservationTime(ZonedDateTime value) {
this.situationRecordObservationTime = value;
}
/**
* Gets the value of the situationRecordVersion property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getSituationRecordVersion() {
return situationRecordVersion;
}
/**
* Sets the value of the situationRecordVersion property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setSituationRecordVersion(BigInteger value) {
this.situationRecordVersion = value;
}
/**
* Gets the value of the situationRecordVersionTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getSituationRecordVersionTime() {
return situationRecordVersionTime;
}
/**
* Sets the value of the situationRecordVersionTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSituationRecordVersionTime(ZonedDateTime value) {
this.situationRecordVersionTime = value;
}
/**
* Gets the value of the situationRecordFirstSupplierVersionTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getSituationRecordFirstSupplierVersionTime() {
return situationRecordFirstSupplierVersionTime;
}
/**
* Sets the value of the situationRecordFirstSupplierVersionTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSituationRecordFirstSupplierVersionTime(ZonedDateTime value) {
this.situationRecordFirstSupplierVersionTime = value;
}
/**
* Gets the value of the probabilityOfOccurrence property.
*
* @return
* possible object is
* {@link ProbabilityOfOccurrenceEnum }
*
*/
public ProbabilityOfOccurrenceEnum getProbabilityOfOccurrence() {
return probabilityOfOccurrence;
}
/**
* Sets the value of the probabilityOfOccurrence property.
*
* @param value
* allowed object is
* {@link ProbabilityOfOccurrenceEnum }
*
*/
public void setProbabilityOfOccurrence(ProbabilityOfOccurrenceEnum value) {
this.probabilityOfOccurrence = value;
}
/**
* Gets the value of the source property.
*
* @return
* possible object is
* {@link Source }
*
*/
public Source getSource() {
return source;
}
/**
* Sets the value of the source property.
*
* @param value
* allowed object is
* {@link Source }
*
*/
public void setSource(Source value) {
this.source = value;
}
/**
* Gets the value of the validity property.
*
* @return
* possible object is
* {@link Validity }
*
*/
public Validity getValidity() {
return validity;
}
/**
* Sets the value of the validity property.
*
* @param value
* allowed object is
* {@link Validity }
*
*/
public void setValidity(Validity value) {
this.validity = value;
}
/**
* Gets the value of the impact property.
*
* @return
* possible object is
* {@link Impact }
*
*/
public Impact getImpact() {
return impact;
}
/**
* Sets the value of the impact property.
*
* @param value
* allowed object is
* {@link Impact }
*
*/
public void setImpact(Impact value) {
this.impact = value;
}
/**
* Gets the value of the cause property.
*
* @return
* possible object is
* {@link Cause }
*
*/
public Cause getCause() {
return cause;
}
/**
* Sets the value of the cause property.
*
* @param value
* allowed object is
* {@link Cause }
*
*/
public void setCause(Cause value) {
this.cause = value;
}
/**
* Gets the value of the generalPublicComments property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the generalPublicComments property.
*
*
* For example, to add a new item, do as follows:
*
* getGeneralPublicComments().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Comment }
*
*
*/
public List getGeneralPublicComments() {
if (generalPublicComments == null) {
generalPublicComments = new ArrayList();
}
return this.generalPublicComments;
}
/**
* Gets the value of the nonGeneralPublicComments property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the nonGeneralPublicComments property.
*
*
* For example, to add a new item, do as follows:
*
* getNonGeneralPublicComments().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Comment }
*
*
*/
public List getNonGeneralPublicComments() {
if (nonGeneralPublicComments == null) {
nonGeneralPublicComments = new ArrayList();
}
return this.nonGeneralPublicComments;
}
/**
* Gets the value of the groupOfLocations property.
*
* @return
* possible object is
* {@link GroupOfLocations }
*
*/
public GroupOfLocations getGroupOfLocations() {
return groupOfLocations;
}
/**
* Sets the value of the groupOfLocations property.
*
* @param value
* allowed object is
* {@link GroupOfLocations }
*
*/
public void setGroupOfLocations(GroupOfLocations value) {
this.groupOfLocations = value;
}
/**
* Gets the value of the management property.
*
* @return
* possible object is
* {@link Management }
*
*/
public Management getManagement() {
return management;
}
/**
* Sets the value of the management property.
*
* @param value
* allowed object is
* {@link Management }
*
*/
public void setManagement(Management value) {
this.management = value;
}
/**
* Gets the value of the situationRecordExtension property.
*
* @return
* possible object is
* {@link ExtensionType }
*
*/
public ExtensionType getSituationRecordExtension() {
return situationRecordExtension;
}
/**
* Sets the value of the situationRecordExtension property.
*
* @param value
* allowed object is
* {@link ExtensionType }
*
*/
public void setSituationRecordExtension(ExtensionType value) {
this.situationRecordExtension = value;
}
/**
* Gets the value of the trafficElementExtension property.
*
* @return
* possible object is
* {@link ExtensionType }
*
*/
public ExtensionType getTrafficElementExtension() {
return trafficElementExtension;
}
/**
* Sets the value of the trafficElementExtension property.
*
* @param value
* allowed object is
* {@link ExtensionType }
*
*/
public void setTrafficElementExtension(ExtensionType value) {
this.trafficElementExtension = value;
}
}