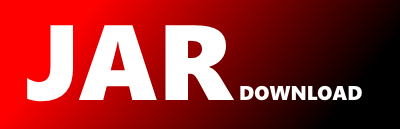
uk.org.siri.siri20.PtSituationElement Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import eu.datex2.siri20.schema._2_0rc1._2_0.InformationStatusEnum;
import eu.datex2.siri20.schema._2_0rc1._2_0.ProbabilityOfOccurrenceEnum;
import eu.datex2.siri20.schema._2_0rc1._2_0.PublicEventTypeEnum;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlList;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Type for individual PT SITUATION.
*
* Java class for PtSituationElementStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PtSituationElementStructure">
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}SituationElementStructure">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}PtSituationBodyGroup"/>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PtSituationElementStructure", propOrder = {
"verification",
"progress",
"qualityIndex",
"reality",
"likelihood",
"publications",
"validityPeriods",
"repetitions",
"publicationWindow",
"undefinedReason",
"environmentReason",
"equipmentReason",
"personnelReason",
"miscellaneousReason",
"unknownReason",
"environmentSubReason",
"equipmentSubReason",
"personnelSubReason",
"miscellaneousSubReason",
"publicEventReason",
"reasonNames",
"severity",
"priority",
"sensitivity",
"audience",
"scopeType",
"reportType",
"planned",
"keywords",
"secondaryReasons",
"language",
"summaries",
"descriptions",
"details",
"advices",
"internal",
"images",
"infoLinks",
"affects",
"consequences",
"publishingActions",
"extensions"
})
@XmlRootElement(name = "PtSituationElement")
public class PtSituationElement
extends SituationElementStructure
implements Serializable
{
@XmlElement(name = "Verification")
protected VerificationStatusEnumeration verification;
@XmlElement(name = "Progress", defaultValue = "open")
@XmlSchemaType(name = "NMTOKEN")
protected WorkflowStatusEnumeration progress;
@XmlElement(name = "QualityIndex")
@XmlSchemaType(name = "string")
protected QualityEnumeration qualityIndex;
@XmlElement(name = "Reality")
@XmlSchemaType(name = "string")
protected InformationStatusEnum reality;
@XmlElement(name = "Likelihood")
@XmlSchemaType(name = "string")
protected ProbabilityOfOccurrenceEnum likelihood;
@XmlElement(name = "Publication")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected List publications;
@XmlElement(name = "ValidityPeriod", required = true)
protected List validityPeriods;
@XmlElement(name = "Repetitions")
protected PtSituationElement.Repetitions repetitions;
@XmlElement(name = "PublicationWindow")
protected HalfOpenTimestampOutputRangeStructure publicationWindow;
@XmlElement(name = "UndefinedReason")
protected String undefinedReason;
@XmlElement(name = "EnvironmentReason")
@XmlSchemaType(name = "NMTOKEN")
protected EnvironmentReasonEnumeration environmentReason;
@XmlElement(name = "EquipmentReason")
@XmlSchemaType(name = "NMTOKEN")
protected EquipmentReasonEnumeration equipmentReason;
@XmlElement(name = "PersonnelReason")
@XmlSchemaType(name = "NMTOKEN")
protected PersonnelReasonEnumeration personnelReason;
@XmlElement(name = "MiscellaneousReason")
@XmlSchemaType(name = "NMTOKEN")
protected MiscellaneousReasonEnumeration miscellaneousReason;
@XmlElement(name = "UnknownReason")
protected String unknownReason;
@XmlElement(name = "EnvironmentSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected EnvironmentSubReasonEnumeration environmentSubReason;
@XmlElement(name = "EquipmentSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected EquipmentSubReasonEnumeration equipmentSubReason;
@XmlElement(name = "PersonnelSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected PersonnelSubReasonEnumeration personnelSubReason;
@XmlElement(name = "MiscellaneousSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected MiscellaneousSubReasonEnumeration miscellaneousSubReason;
@XmlElement(name = "PublicEventReason")
@XmlSchemaType(name = "string")
protected PublicEventTypeEnum publicEventReason;
@XmlElement(name = "ReasonName")
protected List reasonNames;
@XmlElement(name = "Severity", defaultValue = "normal")
@XmlSchemaType(name = "NMTOKEN")
protected SeverityEnumeration severity;
@XmlElement(name = "Priority")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger priority;
@XmlElement(name = "Sensitivity")
@XmlSchemaType(name = "NMTOKEN")
protected SensitivityEnumeration sensitivity;
@XmlElement(name = "Audience", defaultValue = "public")
@XmlSchemaType(name = "NMTOKEN")
protected AudienceEnumeration audience;
@XmlElement(name = "ScopeType")
protected ScopeTypeEnumeration scopeType;
@XmlElement(name = "ReportType", defaultValue = "unknown")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String reportType;
@XmlElement(name = "Planned", defaultValue = "false")
protected Boolean planned;
@XmlList
@XmlElement(name = "Keywords")
@XmlSchemaType(name = "NMTOKENS")
protected List keywords;
@XmlElement(name = "SecondaryReasons")
protected PtSituationElement.SecondaryReasons secondaryReasons;
@XmlElement(name = "Language", defaultValue = "en")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String language;
@XmlElement(name = "Summary")
protected List summaries;
@XmlElement(name = "Description")
protected List descriptions;
@XmlElement(name = "Detail")
protected List details;
@XmlElement(name = "Advice")
protected List advices;
@XmlElement(name = "Internal")
protected DefaultedTextStructure internal;
@XmlElement(name = "Images")
protected PtSituationElement.Images images;
@XmlElement(name = "InfoLinks")
protected PtSituationElement.InfoLinks infoLinks;
@XmlElement(name = "Affects")
protected AffectsScopeStructure affects;
@XmlElement(name = "Consequences")
protected PtConsequencesStructure consequences;
@XmlElement(name = "PublishingActions")
protected ActionsStructure publishingActions;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the verification property.
*
* @return
* possible object is
* {@link VerificationStatusEnumeration }
*
*/
public VerificationStatusEnumeration getVerification() {
return verification;
}
/**
* Sets the value of the verification property.
*
* @param value
* allowed object is
* {@link VerificationStatusEnumeration }
*
*/
public void setVerification(VerificationStatusEnumeration value) {
this.verification = value;
}
/**
* Gets the value of the progress property.
*
* @return
* possible object is
* {@link WorkflowStatusEnumeration }
*
*/
public WorkflowStatusEnumeration getProgress() {
return progress;
}
/**
* Sets the value of the progress property.
*
* @param value
* allowed object is
* {@link WorkflowStatusEnumeration }
*
*/
public void setProgress(WorkflowStatusEnumeration value) {
this.progress = value;
}
/**
* Gets the value of the qualityIndex property.
*
* @return
* possible object is
* {@link QualityEnumeration }
*
*/
public QualityEnumeration getQualityIndex() {
return qualityIndex;
}
/**
* Sets the value of the qualityIndex property.
*
* @param value
* allowed object is
* {@link QualityEnumeration }
*
*/
public void setQualityIndex(QualityEnumeration value) {
this.qualityIndex = value;
}
/**
* Gets the value of the reality property.
*
* @return
* possible object is
* {@link InformationStatusEnum }
*
*/
public InformationStatusEnum getReality() {
return reality;
}
/**
* Sets the value of the reality property.
*
* @param value
* allowed object is
* {@link InformationStatusEnum }
*
*/
public void setReality(InformationStatusEnum value) {
this.reality = value;
}
/**
* Gets the value of the likelihood property.
*
* @return
* possible object is
* {@link ProbabilityOfOccurrenceEnum }
*
*/
public ProbabilityOfOccurrenceEnum getLikelihood() {
return likelihood;
}
/**
* Sets the value of the likelihood property.
*
* @param value
* allowed object is
* {@link ProbabilityOfOccurrenceEnum }
*
*/
public void setLikelihood(ProbabilityOfOccurrenceEnum value) {
this.likelihood = value;
}
/**
* Gets the value of the publications property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publications property.
*
*
* For example, to add a new item, do as follows:
*
* getPublications().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getPublications() {
if (publications == null) {
publications = new ArrayList();
}
return this.publications;
}
/**
* Gets the value of the validityPeriods property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the validityPeriods property.
*
*
* For example, to add a new item, do as follows:
*
* getValidityPeriods().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HalfOpenTimestampOutputRangeStructure }
*
*
*/
public List getValidityPeriods() {
if (validityPeriods == null) {
validityPeriods = new ArrayList();
}
return this.validityPeriods;
}
/**
* Gets the value of the repetitions property.
*
* @return
* possible object is
* {@link PtSituationElement.Repetitions }
*
*/
public PtSituationElement.Repetitions getRepetitions() {
return repetitions;
}
/**
* Sets the value of the repetitions property.
*
* @param value
* allowed object is
* {@link PtSituationElement.Repetitions }
*
*/
public void setRepetitions(PtSituationElement.Repetitions value) {
this.repetitions = value;
}
/**
* Gets the value of the publicationWindow property.
*
* @return
* possible object is
* {@link HalfOpenTimestampOutputRangeStructure }
*
*/
public HalfOpenTimestampOutputRangeStructure getPublicationWindow() {
return publicationWindow;
}
/**
* Sets the value of the publicationWindow property.
*
* @param value
* allowed object is
* {@link HalfOpenTimestampOutputRangeStructure }
*
*/
public void setPublicationWindow(HalfOpenTimestampOutputRangeStructure value) {
this.publicationWindow = value;
}
/**
* Gets the value of the undefinedReason property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUndefinedReason() {
return undefinedReason;
}
/**
* Sets the value of the undefinedReason property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUndefinedReason(String value) {
this.undefinedReason = value;
}
/**
* Environment reason.
*
* @return
* possible object is
* {@link EnvironmentReasonEnumeration }
*
*/
public EnvironmentReasonEnumeration getEnvironmentReason() {
return environmentReason;
}
/**
* Sets the value of the environmentReason property.
*
* @param value
* allowed object is
* {@link EnvironmentReasonEnumeration }
*
*/
public void setEnvironmentReason(EnvironmentReasonEnumeration value) {
this.environmentReason = value;
}
/**
* Gets the value of the equipmentReason property.
*
* @return
* possible object is
* {@link EquipmentReasonEnumeration }
*
*/
public EquipmentReasonEnumeration getEquipmentReason() {
return equipmentReason;
}
/**
* Sets the value of the equipmentReason property.
*
* @param value
* allowed object is
* {@link EquipmentReasonEnumeration }
*
*/
public void setEquipmentReason(EquipmentReasonEnumeration value) {
this.equipmentReason = value;
}
/**
* Personnel reason.
*
* @return
* possible object is
* {@link PersonnelReasonEnumeration }
*
*/
public PersonnelReasonEnumeration getPersonnelReason() {
return personnelReason;
}
/**
* Sets the value of the personnelReason property.
*
* @param value
* allowed object is
* {@link PersonnelReasonEnumeration }
*
*/
public void setPersonnelReason(PersonnelReasonEnumeration value) {
this.personnelReason = value;
}
/**
* Gets the value of the miscellaneousReason property.
*
* @return
* possible object is
* {@link MiscellaneousReasonEnumeration }
*
*/
public MiscellaneousReasonEnumeration getMiscellaneousReason() {
return miscellaneousReason;
}
/**
* Sets the value of the miscellaneousReason property.
*
* @param value
* allowed object is
* {@link MiscellaneousReasonEnumeration }
*
*/
public void setMiscellaneousReason(MiscellaneousReasonEnumeration value) {
this.miscellaneousReason = value;
}
/**
* Gets the value of the unknownReason property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUnknownReason() {
return unknownReason;
}
/**
* Sets the value of the unknownReason property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUnknownReason(String value) {
this.unknownReason = value;
}
/**
* Environment reason.
*
* @return
* possible object is
* {@link EnvironmentSubReasonEnumeration }
*
*/
public EnvironmentSubReasonEnumeration getEnvironmentSubReason() {
return environmentSubReason;
}
/**
* Sets the value of the environmentSubReason property.
*
* @param value
* allowed object is
* {@link EnvironmentSubReasonEnumeration }
*
*/
public void setEnvironmentSubReason(EnvironmentSubReasonEnumeration value) {
this.environmentSubReason = value;
}
/**
* Gets the value of the equipmentSubReason property.
*
* @return
* possible object is
* {@link EquipmentSubReasonEnumeration }
*
*/
public EquipmentSubReasonEnumeration getEquipmentSubReason() {
return equipmentSubReason;
}
/**
* Sets the value of the equipmentSubReason property.
*
* @param value
* allowed object is
* {@link EquipmentSubReasonEnumeration }
*
*/
public void setEquipmentSubReason(EquipmentSubReasonEnumeration value) {
this.equipmentSubReason = value;
}
/**
* Personnel reason.
*
* @return
* possible object is
* {@link PersonnelSubReasonEnumeration }
*
*/
public PersonnelSubReasonEnumeration getPersonnelSubReason() {
return personnelSubReason;
}
/**
* Sets the value of the personnelSubReason property.
*
* @param value
* allowed object is
* {@link PersonnelSubReasonEnumeration }
*
*/
public void setPersonnelSubReason(PersonnelSubReasonEnumeration value) {
this.personnelSubReason = value;
}
/**
* Gets the value of the miscellaneousSubReason property.
*
* @return
* possible object is
* {@link MiscellaneousSubReasonEnumeration }
*
*/
public MiscellaneousSubReasonEnumeration getMiscellaneousSubReason() {
return miscellaneousSubReason;
}
/**
* Sets the value of the miscellaneousSubReason property.
*
* @param value
* allowed object is
* {@link MiscellaneousSubReasonEnumeration }
*
*/
public void setMiscellaneousSubReason(MiscellaneousSubReasonEnumeration value) {
this.miscellaneousSubReason = value;
}
/**
* Gets the value of the publicEventReason property.
*
* @return
* possible object is
* {@link PublicEventTypeEnum }
*
*/
public PublicEventTypeEnum getPublicEventReason() {
return publicEventReason;
}
/**
* Sets the value of the publicEventReason property.
*
* @param value
* allowed object is
* {@link PublicEventTypeEnum }
*
*/
public void setPublicEventReason(PublicEventTypeEnum value) {
this.publicEventReason = value;
}
/**
* Gets the value of the reasonNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the reasonNames property.
*
*
* For example, to add a new item, do as follows:
*
* getReasonNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getReasonNames() {
if (reasonNames == null) {
reasonNames = new ArrayList();
}
return this.reasonNames;
}
/**
* Gets the value of the severity property.
*
* @return
* possible object is
* {@link SeverityEnumeration }
*
*/
public SeverityEnumeration getSeverity() {
return severity;
}
/**
* Sets the value of the severity property.
*
* @param value
* allowed object is
* {@link SeverityEnumeration }
*
*/
public void setSeverity(SeverityEnumeration value) {
this.severity = value;
}
/**
* Gets the value of the priority property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getPriority() {
return priority;
}
/**
* Sets the value of the priority property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setPriority(BigInteger value) {
this.priority = value;
}
/**
* Gets the value of the sensitivity property.
*
* @return
* possible object is
* {@link SensitivityEnumeration }
*
*/
public SensitivityEnumeration getSensitivity() {
return sensitivity;
}
/**
* Sets the value of the sensitivity property.
*
* @param value
* allowed object is
* {@link SensitivityEnumeration }
*
*/
public void setSensitivity(SensitivityEnumeration value) {
this.sensitivity = value;
}
/**
* Gets the value of the audience property.
*
* @return
* possible object is
* {@link AudienceEnumeration }
*
*/
public AudienceEnumeration getAudience() {
return audience;
}
/**
* Sets the value of the audience property.
*
* @param value
* allowed object is
* {@link AudienceEnumeration }
*
*/
public void setAudience(AudienceEnumeration value) {
this.audience = value;
}
/**
* Gets the value of the scopeType property.
*
* @return
* possible object is
* {@link ScopeTypeEnumeration }
*
*/
public ScopeTypeEnumeration getScopeType() {
return scopeType;
}
/**
* Sets the value of the scopeType property.
*
* @param value
* allowed object is
* {@link ScopeTypeEnumeration }
*
*/
public void setScopeType(ScopeTypeEnumeration value) {
this.scopeType = value;
}
/**
* Gets the value of the reportType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReportType() {
return reportType;
}
/**
* Sets the value of the reportType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReportType(String value) {
this.reportType = value;
}
/**
* Gets the value of the planned property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isPlanned() {
return planned;
}
/**
* Sets the value of the planned property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setPlanned(Boolean value) {
this.planned = value;
}
/**
* Gets the value of the keywords property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the keywords property.
*
*
* For example, to add a new item, do as follows:
*
* getKeywords().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getKeywords() {
if (keywords == null) {
keywords = new ArrayList();
}
return this.keywords;
}
/**
* Gets the value of the secondaryReasons property.
*
* @return
* possible object is
* {@link PtSituationElement.SecondaryReasons }
*
*/
public PtSituationElement.SecondaryReasons getSecondaryReasons() {
return secondaryReasons;
}
/**
* Sets the value of the secondaryReasons property.
*
* @param value
* allowed object is
* {@link PtSituationElement.SecondaryReasons }
*
*/
public void setSecondaryReasons(PtSituationElement.SecondaryReasons value) {
this.secondaryReasons = value;
}
/**
* Gets the value of the language property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguage() {
return language;
}
/**
* Sets the value of the language property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguage(String value) {
this.language = value;
}
/**
* Gets the value of the summaries property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the summaries property.
*
*
* For example, to add a new item, do as follows:
*
* getSummaries().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DefaultedTextStructure }
*
*
*/
public List getSummaries() {
if (summaries == null) {
summaries = new ArrayList();
}
return this.summaries;
}
/**
* Gets the value of the descriptions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the descriptions property.
*
*
* For example, to add a new item, do as follows:
*
* getDescriptions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DefaultedTextStructure }
*
*
*/
public List getDescriptions() {
if (descriptions == null) {
descriptions = new ArrayList();
}
return this.descriptions;
}
/**
* Gets the value of the details property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the details property.
*
*
* For example, to add a new item, do as follows:
*
* getDetails().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DefaultedTextStructure }
*
*
*/
public List getDetails() {
if (details == null) {
details = new ArrayList();
}
return this.details;
}
/**
* Gets the value of the advices property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the advices property.
*
*
* For example, to add a new item, do as follows:
*
* getAdvices().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DefaultedTextStructure }
*
*
*/
public List getAdvices() {
if (advices == null) {
advices = new ArrayList();
}
return this.advices;
}
/**
* Gets the value of the internal property.
*
* @return
* possible object is
* {@link DefaultedTextStructure }
*
*/
public DefaultedTextStructure getInternal() {
return internal;
}
/**
* Sets the value of the internal property.
*
* @param value
* allowed object is
* {@link DefaultedTextStructure }
*
*/
public void setInternal(DefaultedTextStructure value) {
this.internal = value;
}
/**
* Gets the value of the images property.
*
* @return
* possible object is
* {@link PtSituationElement.Images }
*
*/
public PtSituationElement.Images getImages() {
return images;
}
/**
* Sets the value of the images property.
*
* @param value
* allowed object is
* {@link PtSituationElement.Images }
*
*/
public void setImages(PtSituationElement.Images value) {
this.images = value;
}
/**
* Gets the value of the infoLinks property.
*
* @return
* possible object is
* {@link PtSituationElement.InfoLinks }
*
*/
public PtSituationElement.InfoLinks getInfoLinks() {
return infoLinks;
}
/**
* Sets the value of the infoLinks property.
*
* @param value
* allowed object is
* {@link PtSituationElement.InfoLinks }
*
*/
public void setInfoLinks(PtSituationElement.InfoLinks value) {
this.infoLinks = value;
}
/**
* Gets the value of the affects property.
*
* @return
* possible object is
* {@link AffectsScopeStructure }
*
*/
public AffectsScopeStructure getAffects() {
return affects;
}
/**
* Sets the value of the affects property.
*
* @param value
* allowed object is
* {@link AffectsScopeStructure }
*
*/
public void setAffects(AffectsScopeStructure value) {
this.affects = value;
}
/**
* Gets the value of the consequences property.
*
* @return
* possible object is
* {@link PtConsequencesStructure }
*
*/
public PtConsequencesStructure getConsequences() {
return consequences;
}
/**
* Sets the value of the consequences property.
*
* @param value
* allowed object is
* {@link PtConsequencesStructure }
*
*/
public void setConsequences(PtConsequencesStructure value) {
this.consequences = value;
}
/**
* Gets the value of the publishingActions property.
*
* @return
* possible object is
* {@link ActionsStructure }
*
*/
public ActionsStructure getPublishingActions() {
return publishingActions;
}
/**
* Sets the value of the publishingActions property.
*
* @param value
* allowed object is
* {@link ActionsStructure }
*
*/
public void setPublishingActions(ActionsStructure value) {
this.publishingActions = value;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Image" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}ImageStructure">
* </extension>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"images"
})
public static class Images
implements Serializable
{
@XmlElement(name = "Image", required = true)
protected List images;
/**
* Gets the value of the images property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the images property.
*
*
* For example, to add a new item, do as follows:
*
* getImages().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PtSituationElement.Images.Image }
*
*
*/
public List getImages() {
if (images == null) {
images = new ArrayList();
}
return this.images;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}ImageStructure">
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Image
extends ImageStructure
implements Serializable
{
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="InfoLink" type="{http://www.siri.org.uk/siri}InfoLinkStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"infoLinks"
})
public static class InfoLinks
implements Serializable
{
@XmlElement(name = "InfoLink", required = true)
protected List infoLinks;
/**
* Gets the value of the infoLinks property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the infoLinks property.
*
*
* For example, to add a new item, do as follows:
*
* getInfoLinks().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InfoLinkStructure }
*
*
*/
public List getInfoLinks() {
if (infoLinks == null) {
infoLinks = new ArrayList();
}
return this.infoLinks;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}DayType" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"dayTypes"
})
public static class Repetitions
implements Serializable
{
@XmlElement(name = "DayType", required = true, defaultValue = "everyDay")
@XmlSchemaType(name = "NMTOKEN")
protected List dayTypes;
/**
* Gets the value of the dayTypes property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the dayTypes property.
*
*
* For example, to add a new item, do as follows:
*
* getDayTypes().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DayTypeEnumeration }
*
*
*/
public List getDayTypes() {
if (dayTypes == null) {
dayTypes = new ArrayList();
}
return this.dayTypes;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence maxOccurs="unbounded">
* <element name="Reason">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}ReasonGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"reasons"
})
public static class SecondaryReasons
implements Serializable
{
@XmlElement(name = "Reason", required = true)
protected List reasons;
/**
* Gets the value of the reasons property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the reasons property.
*
*
* For example, to add a new item, do as follows:
*
* getReasons().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PtSituationElement.SecondaryReasons.Reason }
*
*
*/
public List getReasons() {
if (reasons == null) {
reasons = new ArrayList();
}
return this.reasons;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}ReasonGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"undefinedReason",
"environmentReason",
"equipmentReason",
"personnelReason",
"miscellaneousReason",
"unknownReason",
"environmentSubReason",
"equipmentSubReason",
"personnelSubReason",
"miscellaneousSubReason",
"publicEventReason",
"reasonNames"
})
public static class Reason
implements Serializable
{
@XmlElement(name = "UndefinedReason")
protected String undefinedReason;
@XmlElement(name = "EnvironmentReason")
@XmlSchemaType(name = "NMTOKEN")
protected EnvironmentReasonEnumeration environmentReason;
@XmlElement(name = "EquipmentReason")
@XmlSchemaType(name = "NMTOKEN")
protected EquipmentReasonEnumeration equipmentReason;
@XmlElement(name = "PersonnelReason")
@XmlSchemaType(name = "NMTOKEN")
protected PersonnelReasonEnumeration personnelReason;
@XmlElement(name = "MiscellaneousReason")
@XmlSchemaType(name = "NMTOKEN")
protected MiscellaneousReasonEnumeration miscellaneousReason;
@XmlElement(name = "UnknownReason")
protected String unknownReason;
@XmlElement(name = "EnvironmentSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected EnvironmentSubReasonEnumeration environmentSubReason;
@XmlElement(name = "EquipmentSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected EquipmentSubReasonEnumeration equipmentSubReason;
@XmlElement(name = "PersonnelSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected PersonnelSubReasonEnumeration personnelSubReason;
@XmlElement(name = "MiscellaneousSubReason")
@XmlSchemaType(name = "NMTOKEN")
protected MiscellaneousSubReasonEnumeration miscellaneousSubReason;
@XmlElement(name = "PublicEventReason")
@XmlSchemaType(name = "string")
protected PublicEventTypeEnum publicEventReason;
@XmlElement(name = "ReasonName")
protected List reasonNames;
/**
* Gets the value of the undefinedReason property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUndefinedReason() {
return undefinedReason;
}
/**
* Sets the value of the undefinedReason property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUndefinedReason(String value) {
this.undefinedReason = value;
}
/**
* Environment reason.
*
* @return
* possible object is
* {@link EnvironmentReasonEnumeration }
*
*/
public EnvironmentReasonEnumeration getEnvironmentReason() {
return environmentReason;
}
/**
* Sets the value of the environmentReason property.
*
* @param value
* allowed object is
* {@link EnvironmentReasonEnumeration }
*
*/
public void setEnvironmentReason(EnvironmentReasonEnumeration value) {
this.environmentReason = value;
}
/**
* Gets the value of the equipmentReason property.
*
* @return
* possible object is
* {@link EquipmentReasonEnumeration }
*
*/
public EquipmentReasonEnumeration getEquipmentReason() {
return equipmentReason;
}
/**
* Sets the value of the equipmentReason property.
*
* @param value
* allowed object is
* {@link EquipmentReasonEnumeration }
*
*/
public void setEquipmentReason(EquipmentReasonEnumeration value) {
this.equipmentReason = value;
}
/**
* Personnel reason.
*
* @return
* possible object is
* {@link PersonnelReasonEnumeration }
*
*/
public PersonnelReasonEnumeration getPersonnelReason() {
return personnelReason;
}
/**
* Sets the value of the personnelReason property.
*
* @param value
* allowed object is
* {@link PersonnelReasonEnumeration }
*
*/
public void setPersonnelReason(PersonnelReasonEnumeration value) {
this.personnelReason = value;
}
/**
* Gets the value of the miscellaneousReason property.
*
* @return
* possible object is
* {@link MiscellaneousReasonEnumeration }
*
*/
public MiscellaneousReasonEnumeration getMiscellaneousReason() {
return miscellaneousReason;
}
/**
* Sets the value of the miscellaneousReason property.
*
* @param value
* allowed object is
* {@link MiscellaneousReasonEnumeration }
*
*/
public void setMiscellaneousReason(MiscellaneousReasonEnumeration value) {
this.miscellaneousReason = value;
}
/**
* Gets the value of the unknownReason property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUnknownReason() {
return unknownReason;
}
/**
* Sets the value of the unknownReason property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUnknownReason(String value) {
this.unknownReason = value;
}
/**
* Environment reason.
*
* @return
* possible object is
* {@link EnvironmentSubReasonEnumeration }
*
*/
public EnvironmentSubReasonEnumeration getEnvironmentSubReason() {
return environmentSubReason;
}
/**
* Sets the value of the environmentSubReason property.
*
* @param value
* allowed object is
* {@link EnvironmentSubReasonEnumeration }
*
*/
public void setEnvironmentSubReason(EnvironmentSubReasonEnumeration value) {
this.environmentSubReason = value;
}
/**
* Gets the value of the equipmentSubReason property.
*
* @return
* possible object is
* {@link EquipmentSubReasonEnumeration }
*
*/
public EquipmentSubReasonEnumeration getEquipmentSubReason() {
return equipmentSubReason;
}
/**
* Sets the value of the equipmentSubReason property.
*
* @param value
* allowed object is
* {@link EquipmentSubReasonEnumeration }
*
*/
public void setEquipmentSubReason(EquipmentSubReasonEnumeration value) {
this.equipmentSubReason = value;
}
/**
* Personnel reason.
*
* @return
* possible object is
* {@link PersonnelSubReasonEnumeration }
*
*/
public PersonnelSubReasonEnumeration getPersonnelSubReason() {
return personnelSubReason;
}
/**
* Sets the value of the personnelSubReason property.
*
* @param value
* allowed object is
* {@link PersonnelSubReasonEnumeration }
*
*/
public void setPersonnelSubReason(PersonnelSubReasonEnumeration value) {
this.personnelSubReason = value;
}
/**
* Gets the value of the miscellaneousSubReason property.
*
* @return
* possible object is
* {@link MiscellaneousSubReasonEnumeration }
*
*/
public MiscellaneousSubReasonEnumeration getMiscellaneousSubReason() {
return miscellaneousSubReason;
}
/**
* Sets the value of the miscellaneousSubReason property.
*
* @param value
* allowed object is
* {@link MiscellaneousSubReasonEnumeration }
*
*/
public void setMiscellaneousSubReason(MiscellaneousSubReasonEnumeration value) {
this.miscellaneousSubReason = value;
}
/**
* Gets the value of the publicEventReason property.
*
* @return
* possible object is
* {@link PublicEventTypeEnum }
*
*/
public PublicEventTypeEnum getPublicEventReason() {
return publicEventReason;
}
/**
* Sets the value of the publicEventReason property.
*
* @param value
* allowed object is
* {@link PublicEventTypeEnum }
*
*/
public void setPublicEventReason(PublicEventTypeEnum value) {
this.publicEventReason = value;
}
/**
* Gets the value of the reasonNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the reasonNames property.
*
*
* For example, to add a new item, do as follows:
*
* getReasonNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getReasonNames() {
if (reasonNames == null) {
reasonNames = new ArrayList();
}
return this.reasonNames;
}
}
}
}