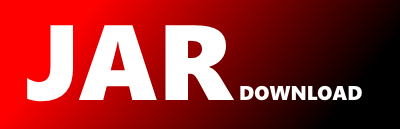
uk.org.siri.siri20.Siri Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of siri-java-model Show documentation
Show all versions of siri-java-model Show documentation
Java objects based on the public SIRI XSDs
The newest version!
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice>
* <group ref="{http://www.siri.org.uk/siri}RequestGroup"/>
* <group ref="{http://www.siri.org.uk/siri}ResponseGroup"/>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </choice>
* <attribute name="version" type="{http://www.siri.org.uk/siri}VersionString" default="2.0" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"extensions",
"connectionLinksDelivery",
"facilityDelivery",
"infoChannelDelivery",
"vehicleFeaturesDelivery",
"serviceFeaturesDelivery",
"productCategoriesDelivery",
"linesDelivery",
"stopPointsDelivery",
"capabilitiesResponse",
"checkStatusResponse",
"dataReceivedAcknowledgement",
"serviceDelivery",
"dataReadyAcknowledgement",
"subscriptionTerminatedNotification",
"terminateSubscriptionResponse",
"subscriptionResponse",
"connectionLinksRequest",
"facilityRequest",
"infoChannelRequest",
"vehicleFeaturesRequest",
"productCategoriesRequest",
"serviceFeaturesRequest",
"linesRequest",
"stopPointsRequest",
"capabilitiesRequest",
"heartbeatNotification",
"checkStatusRequest",
"dataSupplyRequest",
"dataReadyNotification",
"terminateSubscriptionRequest",
"subscriptionRequest",
"serviceRequest"
})
@XmlRootElement(name = "Siri")
public class Siri
implements Serializable
{
@XmlElement(name = "Extensions")
protected Extensions extensions;
@XmlElement(name = "ConnectionLinksDelivery")
protected ConnectionLinksDeliveryStructure connectionLinksDelivery;
@XmlElement(name = "FacilityDelivery")
protected FacilityDeliveryStructure facilityDelivery;
@XmlElement(name = "InfoChannelDelivery")
protected InfoChannelDeliveryStructure infoChannelDelivery;
@XmlElement(name = "VehicleFeaturesDelivery")
protected VehicleFeaturesDeliveryStructure vehicleFeaturesDelivery;
@XmlElement(name = "ServiceFeaturesDelivery")
protected ServiceFeaturesDeliveryStructure serviceFeaturesDelivery;
@XmlElement(name = "ProductCategoriesDelivery")
protected ProductCategoriesDeliveryStructure productCategoriesDelivery;
@XmlElement(name = "LinesDelivery")
protected LinesDeliveryStructure linesDelivery;
@XmlElement(name = "StopPointsDelivery")
protected StopPointsDeliveryStructure stopPointsDelivery;
@XmlElement(name = "CapabilitiesResponse")
protected CapabilitiesResponse capabilitiesResponse;
@XmlElement(name = "CheckStatusResponse")
protected CheckStatusResponseStructure checkStatusResponse;
@XmlElement(name = "DataReceivedAcknowledgement")
protected DataReceivedResponseStructure dataReceivedAcknowledgement;
@XmlElement(name = "ServiceDelivery")
protected ServiceDelivery serviceDelivery;
@XmlElement(name = "DataReadyAcknowledgement")
protected DataReadyResponseStructure dataReadyAcknowledgement;
@XmlElement(name = "SubscriptionTerminatedNotification")
protected SubscriptionTerminatedNotificationStructure subscriptionTerminatedNotification;
@XmlElement(name = "TerminateSubscriptionResponse")
protected TerminateSubscriptionResponseStructure terminateSubscriptionResponse;
@XmlElement(name = "SubscriptionResponse")
protected SubscriptionResponseStructure subscriptionResponse;
@XmlElement(name = "ConnectionLinksRequest")
protected ConnectionLinksDiscoveryRequestStructure connectionLinksRequest;
@XmlElement(name = "FacilityRequest")
protected FacilityRequestStructure facilityRequest;
@XmlElement(name = "InfoChannelRequest")
protected InfoChannelDiscoveryRequestStructure infoChannelRequest;
@XmlElement(name = "VehicleFeaturesRequest")
protected VehicleFeaturesRequestStructure vehicleFeaturesRequest;
@XmlElement(name = "ProductCategoriesRequest")
protected ProductCategoriesDiscoveryRequestStructure productCategoriesRequest;
@XmlElement(name = "ServiceFeaturesRequest")
protected ServiceFeaturesRequest serviceFeaturesRequest;
@XmlElement(name = "LinesRequest")
protected LinesDiscoveryRequestStructure linesRequest;
@XmlElement(name = "StopPointsRequest")
protected StopPointsRequest stopPointsRequest;
@XmlElement(name = "CapabilitiesRequest")
protected CapabilitiesRequest capabilitiesRequest;
@XmlElement(name = "HeartbeatNotification")
protected HeartbeatNotificationStructure heartbeatNotification;
@XmlElement(name = "CheckStatusRequest")
protected CheckStatusRequestStructure checkStatusRequest;
@XmlElement(name = "DataSupplyRequest")
protected DataSupplyRequestStructure dataSupplyRequest;
@XmlElement(name = "DataReadyNotification")
protected DataReadyRequestStructure dataReadyNotification;
@XmlElement(name = "TerminateSubscriptionRequest")
protected TerminateSubscriptionRequestStructure terminateSubscriptionRequest;
@XmlElement(name = "SubscriptionRequest")
protected SubscriptionRequest subscriptionRequest;
@XmlElement(name = "ServiceRequest")
protected ServiceRequest serviceRequest;
@XmlAttribute(name = "version")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String version;
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Gets the value of the connectionLinksDelivery property.
*
* @return
* possible object is
* {@link ConnectionLinksDeliveryStructure }
*
*/
public ConnectionLinksDeliveryStructure getConnectionLinksDelivery() {
return connectionLinksDelivery;
}
/**
* Sets the value of the connectionLinksDelivery property.
*
* @param value
* allowed object is
* {@link ConnectionLinksDeliveryStructure }
*
*/
public void setConnectionLinksDelivery(ConnectionLinksDeliveryStructure value) {
this.connectionLinksDelivery = value;
}
/**
* Gets the value of the facilityDelivery property.
*
* @return
* possible object is
* {@link FacilityDeliveryStructure }
*
*/
public FacilityDeliveryStructure getFacilityDelivery() {
return facilityDelivery;
}
/**
* Sets the value of the facilityDelivery property.
*
* @param value
* allowed object is
* {@link FacilityDeliveryStructure }
*
*/
public void setFacilityDelivery(FacilityDeliveryStructure value) {
this.facilityDelivery = value;
}
/**
* Gets the value of the infoChannelDelivery property.
*
* @return
* possible object is
* {@link InfoChannelDeliveryStructure }
*
*/
public InfoChannelDeliveryStructure getInfoChannelDelivery() {
return infoChannelDelivery;
}
/**
* Sets the value of the infoChannelDelivery property.
*
* @param value
* allowed object is
* {@link InfoChannelDeliveryStructure }
*
*/
public void setInfoChannelDelivery(InfoChannelDeliveryStructure value) {
this.infoChannelDelivery = value;
}
/**
* Gets the value of the vehicleFeaturesDelivery property.
*
* @return
* possible object is
* {@link VehicleFeaturesDeliveryStructure }
*
*/
public VehicleFeaturesDeliveryStructure getVehicleFeaturesDelivery() {
return vehicleFeaturesDelivery;
}
/**
* Sets the value of the vehicleFeaturesDelivery property.
*
* @param value
* allowed object is
* {@link VehicleFeaturesDeliveryStructure }
*
*/
public void setVehicleFeaturesDelivery(VehicleFeaturesDeliveryStructure value) {
this.vehicleFeaturesDelivery = value;
}
/**
* Gets the value of the serviceFeaturesDelivery property.
*
* @return
* possible object is
* {@link ServiceFeaturesDeliveryStructure }
*
*/
public ServiceFeaturesDeliveryStructure getServiceFeaturesDelivery() {
return serviceFeaturesDelivery;
}
/**
* Sets the value of the serviceFeaturesDelivery property.
*
* @param value
* allowed object is
* {@link ServiceFeaturesDeliveryStructure }
*
*/
public void setServiceFeaturesDelivery(ServiceFeaturesDeliveryStructure value) {
this.serviceFeaturesDelivery = value;
}
/**
* Gets the value of the productCategoriesDelivery property.
*
* @return
* possible object is
* {@link ProductCategoriesDeliveryStructure }
*
*/
public ProductCategoriesDeliveryStructure getProductCategoriesDelivery() {
return productCategoriesDelivery;
}
/**
* Sets the value of the productCategoriesDelivery property.
*
* @param value
* allowed object is
* {@link ProductCategoriesDeliveryStructure }
*
*/
public void setProductCategoriesDelivery(ProductCategoriesDeliveryStructure value) {
this.productCategoriesDelivery = value;
}
/**
* Gets the value of the linesDelivery property.
*
* @return
* possible object is
* {@link LinesDeliveryStructure }
*
*/
public LinesDeliveryStructure getLinesDelivery() {
return linesDelivery;
}
/**
* Sets the value of the linesDelivery property.
*
* @param value
* allowed object is
* {@link LinesDeliveryStructure }
*
*/
public void setLinesDelivery(LinesDeliveryStructure value) {
this.linesDelivery = value;
}
/**
* Gets the value of the stopPointsDelivery property.
*
* @return
* possible object is
* {@link StopPointsDeliveryStructure }
*
*/
public StopPointsDeliveryStructure getStopPointsDelivery() {
return stopPointsDelivery;
}
/**
* Sets the value of the stopPointsDelivery property.
*
* @param value
* allowed object is
* {@link StopPointsDeliveryStructure }
*
*/
public void setStopPointsDelivery(StopPointsDeliveryStructure value) {
this.stopPointsDelivery = value;
}
/**
* Responses with the capabilities of an implementation. Answers a CapabilityRequest.
*
* @return
* possible object is
* {@link CapabilitiesResponse }
*
*/
public CapabilitiesResponse getCapabilitiesResponse() {
return capabilitiesResponse;
}
/**
* Sets the value of the capabilitiesResponse property.
*
* @param value
* allowed object is
* {@link CapabilitiesResponse }
*
*/
public void setCapabilitiesResponse(CapabilitiesResponse value) {
this.capabilitiesResponse = value;
}
/**
* Gets the value of the checkStatusResponse property.
*
* @return
* possible object is
* {@link CheckStatusResponseStructure }
*
*/
public CheckStatusResponseStructure getCheckStatusResponse() {
return checkStatusResponse;
}
/**
* Sets the value of the checkStatusResponse property.
*
* @param value
* allowed object is
* {@link CheckStatusResponseStructure }
*
*/
public void setCheckStatusResponse(CheckStatusResponseStructure value) {
this.checkStatusResponse = value;
}
/**
* Gets the value of the dataReceivedAcknowledgement property.
*
* @return
* possible object is
* {@link DataReceivedResponseStructure }
*
*/
public DataReceivedResponseStructure getDataReceivedAcknowledgement() {
return dataReceivedAcknowledgement;
}
/**
* Sets the value of the dataReceivedAcknowledgement property.
*
* @param value
* allowed object is
* {@link DataReceivedResponseStructure }
*
*/
public void setDataReceivedAcknowledgement(DataReceivedResponseStructure value) {
this.dataReceivedAcknowledgement = value;
}
/**
* Gets the value of the serviceDelivery property.
*
* @return
* possible object is
* {@link ServiceDelivery }
*
*/
public ServiceDelivery getServiceDelivery() {
return serviceDelivery;
}
/**
* Sets the value of the serviceDelivery property.
*
* @param value
* allowed object is
* {@link ServiceDelivery }
*
*/
public void setServiceDelivery(ServiceDelivery value) {
this.serviceDelivery = value;
}
/**
* Gets the value of the dataReadyAcknowledgement property.
*
* @return
* possible object is
* {@link DataReadyResponseStructure }
*
*/
public DataReadyResponseStructure getDataReadyAcknowledgement() {
return dataReadyAcknowledgement;
}
/**
* Sets the value of the dataReadyAcknowledgement property.
*
* @param value
* allowed object is
* {@link DataReadyResponseStructure }
*
*/
public void setDataReadyAcknowledgement(DataReadyResponseStructure value) {
this.dataReadyAcknowledgement = value;
}
/**
* Gets the value of the subscriptionTerminatedNotification property.
*
* @return
* possible object is
* {@link SubscriptionTerminatedNotificationStructure }
*
*/
public SubscriptionTerminatedNotificationStructure getSubscriptionTerminatedNotification() {
return subscriptionTerminatedNotification;
}
/**
* Sets the value of the subscriptionTerminatedNotification property.
*
* @param value
* allowed object is
* {@link SubscriptionTerminatedNotificationStructure }
*
*/
public void setSubscriptionTerminatedNotification(SubscriptionTerminatedNotificationStructure value) {
this.subscriptionTerminatedNotification = value;
}
/**
* Gets the value of the terminateSubscriptionResponse property.
*
* @return
* possible object is
* {@link TerminateSubscriptionResponseStructure }
*
*/
public TerminateSubscriptionResponseStructure getTerminateSubscriptionResponse() {
return terminateSubscriptionResponse;
}
/**
* Sets the value of the terminateSubscriptionResponse property.
*
* @param value
* allowed object is
* {@link TerminateSubscriptionResponseStructure }
*
*/
public void setTerminateSubscriptionResponse(TerminateSubscriptionResponseStructure value) {
this.terminateSubscriptionResponse = value;
}
/**
* Gets the value of the subscriptionResponse property.
*
* @return
* possible object is
* {@link SubscriptionResponseStructure }
*
*/
public SubscriptionResponseStructure getSubscriptionResponse() {
return subscriptionResponse;
}
/**
* Sets the value of the subscriptionResponse property.
*
* @param value
* allowed object is
* {@link SubscriptionResponseStructure }
*
*/
public void setSubscriptionResponse(SubscriptionResponseStructure value) {
this.subscriptionResponse = value;
}
/**
* Gets the value of the connectionLinksRequest property.
*
* @return
* possible object is
* {@link ConnectionLinksDiscoveryRequestStructure }
*
*/
public ConnectionLinksDiscoveryRequestStructure getConnectionLinksRequest() {
return connectionLinksRequest;
}
/**
* Sets the value of the connectionLinksRequest property.
*
* @param value
* allowed object is
* {@link ConnectionLinksDiscoveryRequestStructure }
*
*/
public void setConnectionLinksRequest(ConnectionLinksDiscoveryRequestStructure value) {
this.connectionLinksRequest = value;
}
/**
* Gets the value of the facilityRequest property.
*
* @return
* possible object is
* {@link FacilityRequestStructure }
*
*/
public FacilityRequestStructure getFacilityRequest() {
return facilityRequest;
}
/**
* Sets the value of the facilityRequest property.
*
* @param value
* allowed object is
* {@link FacilityRequestStructure }
*
*/
public void setFacilityRequest(FacilityRequestStructure value) {
this.facilityRequest = value;
}
/**
* Gets the value of the infoChannelRequest property.
*
* @return
* possible object is
* {@link InfoChannelDiscoveryRequestStructure }
*
*/
public InfoChannelDiscoveryRequestStructure getInfoChannelRequest() {
return infoChannelRequest;
}
/**
* Sets the value of the infoChannelRequest property.
*
* @param value
* allowed object is
* {@link InfoChannelDiscoveryRequestStructure }
*
*/
public void setInfoChannelRequest(InfoChannelDiscoveryRequestStructure value) {
this.infoChannelRequest = value;
}
/**
* Gets the value of the vehicleFeaturesRequest property.
*
* @return
* possible object is
* {@link VehicleFeaturesRequestStructure }
*
*/
public VehicleFeaturesRequestStructure getVehicleFeaturesRequest() {
return vehicleFeaturesRequest;
}
/**
* Sets the value of the vehicleFeaturesRequest property.
*
* @param value
* allowed object is
* {@link VehicleFeaturesRequestStructure }
*
*/
public void setVehicleFeaturesRequest(VehicleFeaturesRequestStructure value) {
this.vehicleFeaturesRequest = value;
}
/**
* Gets the value of the productCategoriesRequest property.
*
* @return
* possible object is
* {@link ProductCategoriesDiscoveryRequestStructure }
*
*/
public ProductCategoriesDiscoveryRequestStructure getProductCategoriesRequest() {
return productCategoriesRequest;
}
/**
* Sets the value of the productCategoriesRequest property.
*
* @param value
* allowed object is
* {@link ProductCategoriesDiscoveryRequestStructure }
*
*/
public void setProductCategoriesRequest(ProductCategoriesDiscoveryRequestStructure value) {
this.productCategoriesRequest = value;
}
/**
* Gets the value of the serviceFeaturesRequest property.
*
* @return
* possible object is
* {@link ServiceFeaturesRequest }
*
*/
public ServiceFeaturesRequest getServiceFeaturesRequest() {
return serviceFeaturesRequest;
}
/**
* Sets the value of the serviceFeaturesRequest property.
*
* @param value
* allowed object is
* {@link ServiceFeaturesRequest }
*
*/
public void setServiceFeaturesRequest(ServiceFeaturesRequest value) {
this.serviceFeaturesRequest = value;
}
/**
* Gets the value of the linesRequest property.
*
* @return
* possible object is
* {@link LinesDiscoveryRequestStructure }
*
*/
public LinesDiscoveryRequestStructure getLinesRequest() {
return linesRequest;
}
/**
* Sets the value of the linesRequest property.
*
* @param value
* allowed object is
* {@link LinesDiscoveryRequestStructure }
*
*/
public void setLinesRequest(LinesDiscoveryRequestStructure value) {
this.linesRequest = value;
}
/**
* Gets the value of the stopPointsRequest property.
*
* @return
* possible object is
* {@link StopPointsRequest }
*
*/
public StopPointsRequest getStopPointsRequest() {
return stopPointsRequest;
}
/**
* Sets the value of the stopPointsRequest property.
*
* @param value
* allowed object is
* {@link StopPointsRequest }
*
*/
public void setStopPointsRequest(StopPointsRequest value) {
this.stopPointsRequest = value;
}
/**
* Gets the value of the capabilitiesRequest property.
*
* @return
* possible object is
* {@link CapabilitiesRequest }
*
*/
public CapabilitiesRequest getCapabilitiesRequest() {
return capabilitiesRequest;
}
/**
* Sets the value of the capabilitiesRequest property.
*
* @param value
* allowed object is
* {@link CapabilitiesRequest }
*
*/
public void setCapabilitiesRequest(CapabilitiesRequest value) {
this.capabilitiesRequest = value;
}
/**
* Gets the value of the heartbeatNotification property.
*
* @return
* possible object is
* {@link HeartbeatNotificationStructure }
*
*/
public HeartbeatNotificationStructure getHeartbeatNotification() {
return heartbeatNotification;
}
/**
* Sets the value of the heartbeatNotification property.
*
* @param value
* allowed object is
* {@link HeartbeatNotificationStructure }
*
*/
public void setHeartbeatNotification(HeartbeatNotificationStructure value) {
this.heartbeatNotification = value;
}
/**
* Gets the value of the checkStatusRequest property.
*
* @return
* possible object is
* {@link CheckStatusRequestStructure }
*
*/
public CheckStatusRequestStructure getCheckStatusRequest() {
return checkStatusRequest;
}
/**
* Sets the value of the checkStatusRequest property.
*
* @param value
* allowed object is
* {@link CheckStatusRequestStructure }
*
*/
public void setCheckStatusRequest(CheckStatusRequestStructure value) {
this.checkStatusRequest = value;
}
/**
* Gets the value of the dataSupplyRequest property.
*
* @return
* possible object is
* {@link DataSupplyRequestStructure }
*
*/
public DataSupplyRequestStructure getDataSupplyRequest() {
return dataSupplyRequest;
}
/**
* Sets the value of the dataSupplyRequest property.
*
* @param value
* allowed object is
* {@link DataSupplyRequestStructure }
*
*/
public void setDataSupplyRequest(DataSupplyRequestStructure value) {
this.dataSupplyRequest = value;
}
/**
* Gets the value of the dataReadyNotification property.
*
* @return
* possible object is
* {@link DataReadyRequestStructure }
*
*/
public DataReadyRequestStructure getDataReadyNotification() {
return dataReadyNotification;
}
/**
* Sets the value of the dataReadyNotification property.
*
* @param value
* allowed object is
* {@link DataReadyRequestStructure }
*
*/
public void setDataReadyNotification(DataReadyRequestStructure value) {
this.dataReadyNotification = value;
}
/**
* Gets the value of the terminateSubscriptionRequest property.
*
* @return
* possible object is
* {@link TerminateSubscriptionRequestStructure }
*
*/
public TerminateSubscriptionRequestStructure getTerminateSubscriptionRequest() {
return terminateSubscriptionRequest;
}
/**
* Sets the value of the terminateSubscriptionRequest property.
*
* @param value
* allowed object is
* {@link TerminateSubscriptionRequestStructure }
*
*/
public void setTerminateSubscriptionRequest(TerminateSubscriptionRequestStructure value) {
this.terminateSubscriptionRequest = value;
}
/**
* Gets the value of the subscriptionRequest property.
*
* @return
* possible object is
* {@link SubscriptionRequest }
*
*/
public SubscriptionRequest getSubscriptionRequest() {
return subscriptionRequest;
}
/**
* Sets the value of the subscriptionRequest property.
*
* @param value
* allowed object is
* {@link SubscriptionRequest }
*
*/
public void setSubscriptionRequest(SubscriptionRequest value) {
this.subscriptionRequest = value;
}
/**
* Gets the value of the serviceRequest property.
*
* @return
* possible object is
* {@link ServiceRequest }
*
*/
public ServiceRequest getServiceRequest() {
return serviceRequest;
}
/**
* Sets the value of the serviceRequest property.
*
* @param value
* allowed object is
* {@link ServiceRequest }
*
*/
public void setServiceRequest(ServiceRequest value) {
this.serviceRequest = value;
}
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
if (version == null) {
return "2.0";
} else {
return version;
}
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy