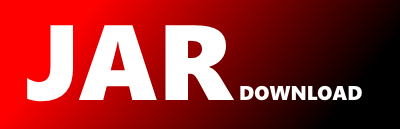
uk.org.siri.siri20.TimetabledFeederArrivalCancellation Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Type for Timetabled Deletion of a feeder connection.
*
* Java class for TimetabledFeederArrivalCancellationStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="TimetabledFeederArrivalCancellationStructure">
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}AbstractReferencingItemStructure">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}InterchangeFeederIdentityGroup"/>
* <element name="LineRef" type="{http://www.siri.org.uk/siri}LineRefStructure"/>
* <element name="DirectionRef" type="{http://www.siri.org.uk/siri}DirectionRefStructure"/>
* <element name="VehicleJourneyRef" type="{http://www.siri.org.uk/siri}FramedVehicleJourneyRefStructure"/>
* <group ref="{http://www.siri.org.uk/siri}JourneyPatternInfoGroup" minOccurs="0"/>
* <element name="Reason" type="{http://www.siri.org.uk/siri}NaturalLanguageStringStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TimetabledFeederArrivalCancellationStructure", propOrder = {
"interchangeRef",
"connectionLinkRef",
"stopPointRef",
"visitNumber",
"order",
"stopPointNames",
"lineRef",
"directionRef",
"vehicleJourneyRef",
"journeyPatternRef",
"journeyPatternName",
"vehicleModes",
"routeRef",
"publishedLineNames",
"groupOfLinesRef",
"directionNames",
"externalLineRef",
"reasons",
"extensions"
})
@XmlRootElement(name = "TimetabledFeederArrivalCancellation")
public class TimetabledFeederArrivalCancellation
extends AbstractReferencingItemStructure
implements Serializable
{
@XmlElement(name = "InterchangeRef")
protected InterchangeRef interchangeRef;
@XmlElement(name = "ConnectionLinkRef", required = true)
protected ConnectionLinkRef connectionLinkRef;
@XmlElement(name = "StopPointRef")
protected StopPointRef stopPointRef;
@XmlElement(name = "VisitNumber")
@XmlSchemaType(name = "positiveInteger")
protected BigInteger visitNumber;
@XmlElement(name = "Order")
@XmlSchemaType(name = "positiveInteger")
protected BigInteger order;
@XmlElement(name = "StopPointName")
protected List stopPointNames;
@XmlElement(name = "LineRef", required = true)
protected LineRef lineRef;
@XmlElement(name = "DirectionRef", required = true)
protected DirectionRefStructure directionRef;
@XmlElement(name = "VehicleJourneyRef", required = true)
protected FramedVehicleJourneyRefStructure vehicleJourneyRef;
@XmlElement(name = "JourneyPatternRef")
protected JourneyPatternRef journeyPatternRef;
@XmlElement(name = "JourneyPatternName")
protected NaturalLanguageStringStructure journeyPatternName;
@XmlElement(name = "VehicleMode")
@XmlSchemaType(name = "NMTOKEN")
protected List vehicleModes;
@XmlElement(name = "RouteRef")
protected RouteRefStructure routeRef;
@XmlElement(name = "PublishedLineName")
protected List publishedLineNames;
@XmlElement(name = "GroupOfLinesRef")
protected GroupOfLinesRefStructure groupOfLinesRef;
@XmlElement(name = "DirectionName")
protected List directionNames;
@XmlElement(name = "ExternalLineRef")
protected LineRef externalLineRef;
@XmlElement(name = "Reason")
protected List reasons;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the interchangeRef property.
*
* @return
* possible object is
* {@link InterchangeRef }
*
*/
public InterchangeRef getInterchangeRef() {
return interchangeRef;
}
/**
* Sets the value of the interchangeRef property.
*
* @param value
* allowed object is
* {@link InterchangeRef }
*
*/
public void setInterchangeRef(InterchangeRef value) {
this.interchangeRef = value;
}
/**
* Gets the value of the connectionLinkRef property.
*
* @return
* possible object is
* {@link ConnectionLinkRef }
*
*/
public ConnectionLinkRef getConnectionLinkRef() {
return connectionLinkRef;
}
/**
* Sets the value of the connectionLinkRef property.
*
* @param value
* allowed object is
* {@link ConnectionLinkRef }
*
*/
public void setConnectionLinkRef(ConnectionLinkRef value) {
this.connectionLinkRef = value;
}
/**
* Gets the value of the stopPointRef property.
*
* @return
* possible object is
* {@link StopPointRef }
*
*/
public StopPointRef getStopPointRef() {
return stopPointRef;
}
/**
* Sets the value of the stopPointRef property.
*
* @param value
* allowed object is
* {@link StopPointRef }
*
*/
public void setStopPointRef(StopPointRef value) {
this.stopPointRef = value;
}
/**
* Gets the value of the visitNumber property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getVisitNumber() {
return visitNumber;
}
/**
* Sets the value of the visitNumber property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setVisitNumber(BigInteger value) {
this.visitNumber = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setOrder(BigInteger value) {
this.order = value;
}
/**
* Name of SCHEDULED STOP POINT. (Unbounded since SIRI 2.0) Gets the value of the stopPointNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the stopPointNames property.
*
*
* For example, to add a new item, do as follows:
*
* getStopPointNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getStopPointNames() {
if (stopPointNames == null) {
stopPointNames = new ArrayList();
}
return this.stopPointNames;
}
/**
* Gets the value of the lineRef property.
*
* @return
* possible object is
* {@link LineRef }
*
*/
public LineRef getLineRef() {
return lineRef;
}
/**
* Sets the value of the lineRef property.
*
* @param value
* allowed object is
* {@link LineRef }
*
*/
public void setLineRef(LineRef value) {
this.lineRef = value;
}
/**
* Gets the value of the directionRef property.
*
* @return
* possible object is
* {@link DirectionRefStructure }
*
*/
public DirectionRefStructure getDirectionRef() {
return directionRef;
}
/**
* Sets the value of the directionRef property.
*
* @param value
* allowed object is
* {@link DirectionRefStructure }
*
*/
public void setDirectionRef(DirectionRefStructure value) {
this.directionRef = value;
}
/**
* Gets the value of the vehicleJourneyRef property.
*
* @return
* possible object is
* {@link FramedVehicleJourneyRefStructure }
*
*/
public FramedVehicleJourneyRefStructure getVehicleJourneyRef() {
return vehicleJourneyRef;
}
/**
* Sets the value of the vehicleJourneyRef property.
*
* @param value
* allowed object is
* {@link FramedVehicleJourneyRefStructure }
*
*/
public void setVehicleJourneyRef(FramedVehicleJourneyRefStructure value) {
this.vehicleJourneyRef = value;
}
/**
* Gets the value of the journeyPatternRef property.
*
* @return
* possible object is
* {@link JourneyPatternRef }
*
*/
public JourneyPatternRef getJourneyPatternRef() {
return journeyPatternRef;
}
/**
* Sets the value of the journeyPatternRef property.
*
* @param value
* allowed object is
* {@link JourneyPatternRef }
*
*/
public void setJourneyPatternRef(JourneyPatternRef value) {
this.journeyPatternRef = value;
}
/**
* Gets the value of the journeyPatternName property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getJourneyPatternName() {
return journeyPatternName;
}
/**
* Sets the value of the journeyPatternName property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setJourneyPatternName(NaturalLanguageStringStructure value) {
this.journeyPatternName = value;
}
/**
* Gets the value of the vehicleModes property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vehicleModes property.
*
*
* For example, to add a new item, do as follows:
*
* getVehicleModes().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VehicleModesEnumeration }
*
*
*/
public List getVehicleModes() {
if (vehicleModes == null) {
vehicleModes = new ArrayList();
}
return this.vehicleModes;
}
/**
* Gets the value of the routeRef property.
*
* @return
* possible object is
* {@link RouteRefStructure }
*
*/
public RouteRefStructure getRouteRef() {
return routeRef;
}
/**
* Sets the value of the routeRef property.
*
* @param value
* allowed object is
* {@link RouteRefStructure }
*
*/
public void setRouteRef(RouteRefStructure value) {
this.routeRef = value;
}
/**
* Name or Number by which the LINE is known to the public. (Unbounded since SIRI 2.0) Gets the value of the publishedLineNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishedLineNames property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishedLineNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getPublishedLineNames() {
if (publishedLineNames == null) {
publishedLineNames = new ArrayList();
}
return this.publishedLineNames;
}
/**
* Gets the value of the groupOfLinesRef property.
*
* @return
* possible object is
* {@link GroupOfLinesRefStructure }
*
*/
public GroupOfLinesRefStructure getGroupOfLinesRef() {
return groupOfLinesRef;
}
/**
* Sets the value of the groupOfLinesRef property.
*
* @param value
* allowed object is
* {@link GroupOfLinesRefStructure }
*
*/
public void setGroupOfLinesRef(GroupOfLinesRefStructure value) {
this.groupOfLinesRef = value;
}
/**
* Gets the value of the directionNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the directionNames property.
*
*
* For example, to add a new item, do as follows:
*
* getDirectionNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getDirectionNames() {
if (directionNames == null) {
directionNames = new ArrayList();
}
return this.directionNames;
}
/**
* Gets the value of the externalLineRef property.
*
* @return
* possible object is
* {@link LineRef }
*
*/
public LineRef getExternalLineRef() {
return externalLineRef;
}
/**
* Sets the value of the externalLineRef property.
*
* @param value
* allowed object is
* {@link LineRef }
*
*/
public void setExternalLineRef(LineRef value) {
this.externalLineRef = value;
}
/**
* Gets the value of the reasons property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the reasons property.
*
*
* For example, to add a new item, do as follows:
*
* getReasons().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getReasons() {
if (reasons == null) {
reasons = new ArrayList();
}
return this.reasons;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
}