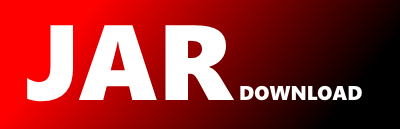
uk.org.siri.siri20.VehicleMonitoringServiceCapabilities Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:39 PM UTC
//
package uk.org.siri.siri20;
import java.io.Serializable;
import java.time.Duration;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.entur.siri.adapter.DurationXmlAdapter;
/**
* Type for Vehicle Monitoring Capabilities.
*
* Java class for VehicleMonitoringServiceCapabilitiesStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="VehicleMonitoringServiceCapabilitiesStructure">
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}AbstractCapabilitiesStructure">
* <sequence>
* <element name="TopicFiltering" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="DefaultPreviewInterval" type="{http://www.siri.org.uk/siri}PositiveDurationType"/>
* <element name="FilterByVehicleMonitoringRef" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element ref="{http://www.siri.org.uk/siri}FilterByVehicleRef" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}FilterByLineRef" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}FilterByDirectionRef" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="RequestPolicy" minOccurs="0">
* <complexType>
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}CapabilityRequestPolicyStructure">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}VehicleMonitoringVolumeGroup"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
* </element>
* <element name="SubscriptionPolicy" type="{http://www.siri.org.uk/siri}CapabilitySubscriptionPolicyStructure" minOccurs="0"/>
* <element name="AccessControl" minOccurs="0">
* <complexType>
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}CapabilityAccessControlStructure">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}CheckOperatorRef" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}CheckLineRef" minOccurs="0"/>
* <element name="CheckVehicleMonitoringRef" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
* </element>
* <element name="ResponseFeatures" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="HasLocation" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="HasSituations" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "VehicleMonitoringServiceCapabilitiesStructure", propOrder = {
"topicFiltering",
"requestPolicy",
"subscriptionPolicy",
"accessControl",
"responseFeatures",
"extensions"
})
@XmlRootElement(name = "VehicleMonitoringServiceCapabilities")
public class VehicleMonitoringServiceCapabilities
extends AbstractCapabilitiesStructure
implements Serializable
{
@XmlElement(name = "TopicFiltering")
protected VehicleMonitoringServiceCapabilities.TopicFiltering topicFiltering;
@XmlElement(name = "RequestPolicy")
protected VehicleMonitoringServiceCapabilities.RequestPolicy requestPolicy;
@XmlElement(name = "SubscriptionPolicy")
protected CapabilitySubscriptionPolicyStructure subscriptionPolicy;
@XmlElement(name = "AccessControl")
protected VehicleMonitoringServiceCapabilities.AccessControl accessControl;
@XmlElement(name = "ResponseFeatures")
protected VehicleMonitoringServiceCapabilities.ResponseFeatures responseFeatures;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the topicFiltering property.
*
* @return
* possible object is
* {@link VehicleMonitoringServiceCapabilities.TopicFiltering }
*
*/
public VehicleMonitoringServiceCapabilities.TopicFiltering getTopicFiltering() {
return topicFiltering;
}
/**
* Sets the value of the topicFiltering property.
*
* @param value
* allowed object is
* {@link VehicleMonitoringServiceCapabilities.TopicFiltering }
*
*/
public void setTopicFiltering(VehicleMonitoringServiceCapabilities.TopicFiltering value) {
this.topicFiltering = value;
}
/**
* Gets the value of the requestPolicy property.
*
* @return
* possible object is
* {@link VehicleMonitoringServiceCapabilities.RequestPolicy }
*
*/
public VehicleMonitoringServiceCapabilities.RequestPolicy getRequestPolicy() {
return requestPolicy;
}
/**
* Sets the value of the requestPolicy property.
*
* @param value
* allowed object is
* {@link VehicleMonitoringServiceCapabilities.RequestPolicy }
*
*/
public void setRequestPolicy(VehicleMonitoringServiceCapabilities.RequestPolicy value) {
this.requestPolicy = value;
}
/**
* Gets the value of the subscriptionPolicy property.
*
* @return
* possible object is
* {@link CapabilitySubscriptionPolicyStructure }
*
*/
public CapabilitySubscriptionPolicyStructure getSubscriptionPolicy() {
return subscriptionPolicy;
}
/**
* Sets the value of the subscriptionPolicy property.
*
* @param value
* allowed object is
* {@link CapabilitySubscriptionPolicyStructure }
*
*/
public void setSubscriptionPolicy(CapabilitySubscriptionPolicyStructure value) {
this.subscriptionPolicy = value;
}
/**
* Gets the value of the accessControl property.
*
* @return
* possible object is
* {@link VehicleMonitoringServiceCapabilities.AccessControl }
*
*/
public VehicleMonitoringServiceCapabilities.AccessControl getAccessControl() {
return accessControl;
}
/**
* Sets the value of the accessControl property.
*
* @param value
* allowed object is
* {@link VehicleMonitoringServiceCapabilities.AccessControl }
*
*/
public void setAccessControl(VehicleMonitoringServiceCapabilities.AccessControl value) {
this.accessControl = value;
}
/**
* Gets the value of the responseFeatures property.
*
* @return
* possible object is
* {@link VehicleMonitoringServiceCapabilities.ResponseFeatures }
*
*/
public VehicleMonitoringServiceCapabilities.ResponseFeatures getResponseFeatures() {
return responseFeatures;
}
/**
* Sets the value of the responseFeatures property.
*
* @param value
* allowed object is
* {@link VehicleMonitoringServiceCapabilities.ResponseFeatures }
*
*/
public void setResponseFeatures(VehicleMonitoringServiceCapabilities.ResponseFeatures value) {
this.responseFeatures = value;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}CapabilityAccessControlStructure">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}CheckOperatorRef" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}CheckLineRef" minOccurs="0"/>
* <element name="CheckVehicleMonitoringRef" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"checkOperatorRef",
"checkLineRef",
"checkVehicleMonitoringRef"
})
public static class AccessControl
extends CapabilityAccessControlStructure
implements Serializable
{
@XmlElement(name = "CheckOperatorRef", defaultValue = "true")
protected Boolean checkOperatorRef;
@XmlElement(name = "CheckLineRef", defaultValue = "true")
protected Boolean checkLineRef;
@XmlElement(name = "CheckVehicleMonitoringRef", defaultValue = "true")
protected Boolean checkVehicleMonitoringRef;
/**
* Gets the value of the checkOperatorRef property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCheckOperatorRef() {
return checkOperatorRef;
}
/**
* Sets the value of the checkOperatorRef property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCheckOperatorRef(Boolean value) {
this.checkOperatorRef = value;
}
/**
* Gets the value of the checkLineRef property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCheckLineRef() {
return checkLineRef;
}
/**
* Sets the value of the checkLineRef property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCheckLineRef(Boolean value) {
this.checkLineRef = value;
}
/**
* Gets the value of the checkVehicleMonitoringRef property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCheckVehicleMonitoringRef() {
return checkVehicleMonitoringRef;
}
/**
* Sets the value of the checkVehicleMonitoringRef property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCheckVehicleMonitoringRef(Boolean value) {
this.checkVehicleMonitoringRef = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}CapabilityRequestPolicyStructure">
* <sequence>
* <group ref="{http://www.siri.org.uk/siri}VehicleMonitoringVolumeGroup"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"hasDetailLevel",
"defaultDetailLevel",
"hasMaximumVehicles",
"hasMaximumNumberOfCalls",
"hasNumberOfOnwardsCalls",
"hasNumberOfPreviousCalls"
})
public static class RequestPolicy
extends CapabilityRequestPolicyStructure
implements Serializable
{
@XmlElement(name = "HasDetailLevel", defaultValue = "false")
protected Boolean hasDetailLevel;
@XmlElement(name = "DefaultDetailLevel", defaultValue = "normal")
@XmlSchemaType(name = "NMTOKEN")
protected VehicleMonitoringDetailEnumeration defaultDetailLevel;
@XmlElement(name = "HasMaximumVehicles", defaultValue = "true")
protected Boolean hasMaximumVehicles;
@XmlElement(name = "HasMaximumNumberOfCalls", defaultValue = "false")
protected Boolean hasMaximumNumberOfCalls;
@XmlElement(name = "HasNumberOfOnwardsCalls", defaultValue = "false")
protected Boolean hasNumberOfOnwardsCalls;
@XmlElement(name = "HasNumberOfPreviousCalls", defaultValue = "false")
protected Boolean hasNumberOfPreviousCalls;
/**
* Gets the value of the hasDetailLevel property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasDetailLevel() {
return hasDetailLevel;
}
/**
* Sets the value of the hasDetailLevel property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasDetailLevel(Boolean value) {
this.hasDetailLevel = value;
}
/**
* Gets the value of the defaultDetailLevel property.
*
* @return
* possible object is
* {@link VehicleMonitoringDetailEnumeration }
*
*/
public VehicleMonitoringDetailEnumeration getDefaultDetailLevel() {
return defaultDetailLevel;
}
/**
* Sets the value of the defaultDetailLevel property.
*
* @param value
* allowed object is
* {@link VehicleMonitoringDetailEnumeration }
*
*/
public void setDefaultDetailLevel(VehicleMonitoringDetailEnumeration value) {
this.defaultDetailLevel = value;
}
/**
* Gets the value of the hasMaximumVehicles property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasMaximumVehicles() {
return hasMaximumVehicles;
}
/**
* Sets the value of the hasMaximumVehicles property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasMaximumVehicles(Boolean value) {
this.hasMaximumVehicles = value;
}
/**
* Gets the value of the hasMaximumNumberOfCalls property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasMaximumNumberOfCalls() {
return hasMaximumNumberOfCalls;
}
/**
* Sets the value of the hasMaximumNumberOfCalls property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasMaximumNumberOfCalls(Boolean value) {
this.hasMaximumNumberOfCalls = value;
}
/**
* Gets the value of the hasNumberOfOnwardsCalls property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasNumberOfOnwardsCalls() {
return hasNumberOfOnwardsCalls;
}
/**
* Sets the value of the hasNumberOfOnwardsCalls property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasNumberOfOnwardsCalls(Boolean value) {
this.hasNumberOfOnwardsCalls = value;
}
/**
* Gets the value of the hasNumberOfPreviousCalls property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasNumberOfPreviousCalls() {
return hasNumberOfPreviousCalls;
}
/**
* Sets the value of the hasNumberOfPreviousCalls property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasNumberOfPreviousCalls(Boolean value) {
this.hasNumberOfPreviousCalls = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="HasLocation" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="HasSituations" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"hasLocation",
"hasSituations"
})
public static class ResponseFeatures
implements Serializable
{
@XmlElement(name = "HasLocation", defaultValue = "true")
protected Boolean hasLocation;
@XmlElement(name = "HasSituations", defaultValue = "false")
protected Boolean hasSituations;
/**
* Gets the value of the hasLocation property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasLocation() {
return hasLocation;
}
/**
* Sets the value of the hasLocation property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasLocation(Boolean value) {
this.hasLocation = value;
}
/**
* Gets the value of the hasSituations property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasSituations() {
return hasSituations;
}
/**
* Sets the value of the hasSituations property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasSituations(Boolean value) {
this.hasSituations = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="DefaultPreviewInterval" type="{http://www.siri.org.uk/siri}PositiveDurationType"/>
* <element name="FilterByVehicleMonitoringRef" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element ref="{http://www.siri.org.uk/siri}FilterByVehicleRef" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}FilterByLineRef" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}FilterByDirectionRef" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"defaultPreviewInterval",
"filterByVehicleMonitoringRef",
"filterByVehicleRef",
"filterByLineRef",
"filterByDirectionRef"
})
public static class TopicFiltering
implements Serializable
{
@XmlElement(name = "DefaultPreviewInterval", required = true, type = String.class, defaultValue = "PT60M")
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration defaultPreviewInterval;
@XmlElement(name = "FilterByVehicleMonitoringRef")
protected boolean filterByVehicleMonitoringRef;
@XmlElement(name = "FilterByVehicleRef", defaultValue = "false")
protected Boolean filterByVehicleRef;
@XmlElement(name = "FilterByLineRef", defaultValue = "true")
protected Boolean filterByLineRef;
@XmlElement(name = "FilterByDirectionRef", defaultValue = "true")
protected Boolean filterByDirectionRef;
/**
* Gets the value of the defaultPreviewInterval property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getDefaultPreviewInterval() {
return defaultPreviewInterval;
}
/**
* Sets the value of the defaultPreviewInterval property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDefaultPreviewInterval(Duration value) {
this.defaultPreviewInterval = value;
}
/**
* Gets the value of the filterByVehicleMonitoringRef property.
*
*/
public boolean isFilterByVehicleMonitoringRef() {
return filterByVehicleMonitoringRef;
}
/**
* Sets the value of the filterByVehicleMonitoringRef property.
*
*/
public void setFilterByVehicleMonitoringRef(boolean value) {
this.filterByVehicleMonitoringRef = value;
}
/**
* Gets the value of the filterByVehicleRef property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFilterByVehicleRef() {
return filterByVehicleRef;
}
/**
* Sets the value of the filterByVehicleRef property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setFilterByVehicleRef(Boolean value) {
this.filterByVehicleRef = value;
}
/**
* Gets the value of the filterByLineRef property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFilterByLineRef() {
return filterByLineRef;
}
/**
* Sets the value of the filterByLineRef property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setFilterByLineRef(Boolean value) {
this.filterByLineRef = value;
}
/**
* Gets the value of the filterByDirectionRef property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFilterByDirectionRef() {
return filterByDirectionRef;
}
/**
* Sets the value of the filterByDirectionRef property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setFilterByDirectionRef(Boolean value) {
this.filterByDirectionRef = value;
}
}
}