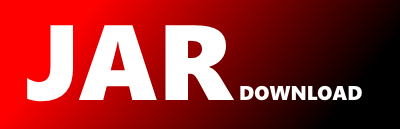
uk.org.siri.siri21.AffectedLineStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
* Information about the individual LINEs in the network that are affected by a SITUATION. If not explicitly overridden, modes and submodes will be defaulted to any values present (i) in the AffectedNetwork (ii) in the general context.
*
* Java class for AffectedLineStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AffectedLineStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedOperator" type="{http://www.siri.org.uk/siri}AffectedOperatorStructure" maxOccurs="unbounded" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}LineGroup"/>
* <element name="Origins" type="{http://www.siri.org.uk/siri}AffectedStopPointStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Destinations" type="{http://www.siri.org.uk/siri}AffectedStopPointStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Direction" type="{http://www.siri.org.uk/siri}DirectionStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Routes" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedRoute" type="{http://www.siri.org.uk/siri}AffectedRouteStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Sections" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedSection" type="{http://www.siri.org.uk/siri}AffectedSectionStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="StopPoints" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedStopPoint" type="{http://www.siri.org.uk/siri}AffectedStopPointStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="StopPlaces" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedStopPlace" type="{http://www.siri.org.uk/siri}AffectedStopPlaceStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AffectedLineStructure", propOrder = {
"affectedOperators",
"lineRef",
"publishedLineNames",
"origins",
"destinations",
"directions",
"routes",
"sections",
"stopPoints",
"stopPlaces",
"extensions"
})
public class AffectedLineStructure
implements Serializable
{
@XmlElement(name = "AffectedOperator")
protected List affectedOperators;
@XmlElement(name = "LineRef", required = true)
protected LineRef lineRef;
@XmlElement(name = "PublishedLineName")
protected List publishedLineNames;
@XmlElement(name = "Origins")
protected List origins;
@XmlElement(name = "Destinations")
protected List destinations;
@XmlElement(name = "Direction")
protected List directions;
@XmlElement(name = "Routes")
protected AffectedLineStructure.Routes routes;
@XmlElement(name = "Sections")
protected AffectedLineStructure.Sections sections;
@XmlElement(name = "StopPoints")
protected AffectedLineStructure.StopPoints stopPoints;
@XmlElement(name = "StopPlaces")
protected AffectedLineStructure.StopPlaces stopPlaces;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the affectedOperators property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the affectedOperators property.
*
*
* For example, to add a new item, do as follows:
*
* getAffectedOperators().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedOperatorStructure }
*
*
*/
public List getAffectedOperators() {
if (affectedOperators == null) {
affectedOperators = new ArrayList();
}
return this.affectedOperators;
}
/**
* Gets the value of the lineRef property.
*
* @return
* possible object is
* {@link LineRef }
*
*/
public LineRef getLineRef() {
return lineRef;
}
/**
* Sets the value of the lineRef property.
*
* @param value
* allowed object is
* {@link LineRef }
*
*/
public void setLineRef(LineRef value) {
this.lineRef = value;
}
/**
* Gets the value of the publishedLineNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishedLineNames property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishedLineNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getPublishedLineNames() {
if (publishedLineNames == null) {
publishedLineNames = new ArrayList();
}
return this.publishedLineNames;
}
/**
* Gets the value of the origins property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the origins property.
*
*
* For example, to add a new item, do as follows:
*
* getOrigins().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedStopPointStructure }
*
*
*/
public List getOrigins() {
if (origins == null) {
origins = new ArrayList();
}
return this.origins;
}
/**
* Gets the value of the destinations property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the destinations property.
*
*
* For example, to add a new item, do as follows:
*
* getDestinations().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedStopPointStructure }
*
*
*/
public List getDestinations() {
if (destinations == null) {
destinations = new ArrayList();
}
return this.destinations;
}
/**
* Gets the value of the directions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the directions property.
*
*
* For example, to add a new item, do as follows:
*
* getDirections().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DirectionStructure }
*
*
*/
public List getDirections() {
if (directions == null) {
directions = new ArrayList();
}
return this.directions;
}
/**
* Gets the value of the routes property.
*
* @return
* possible object is
* {@link AffectedLineStructure.Routes }
*
*/
public AffectedLineStructure.Routes getRoutes() {
return routes;
}
/**
* Sets the value of the routes property.
*
* @param value
* allowed object is
* {@link AffectedLineStructure.Routes }
*
*/
public void setRoutes(AffectedLineStructure.Routes value) {
this.routes = value;
}
/**
* Gets the value of the sections property.
*
* @return
* possible object is
* {@link AffectedLineStructure.Sections }
*
*/
public AffectedLineStructure.Sections getSections() {
return sections;
}
/**
* Sets the value of the sections property.
*
* @param value
* allowed object is
* {@link AffectedLineStructure.Sections }
*
*/
public void setSections(AffectedLineStructure.Sections value) {
this.sections = value;
}
/**
* Gets the value of the stopPoints property.
*
* @return
* possible object is
* {@link AffectedLineStructure.StopPoints }
*
*/
public AffectedLineStructure.StopPoints getStopPoints() {
return stopPoints;
}
/**
* Sets the value of the stopPoints property.
*
* @param value
* allowed object is
* {@link AffectedLineStructure.StopPoints }
*
*/
public void setStopPoints(AffectedLineStructure.StopPoints value) {
this.stopPoints = value;
}
/**
* Gets the value of the stopPlaces property.
*
* @return
* possible object is
* {@link AffectedLineStructure.StopPlaces }
*
*/
public AffectedLineStructure.StopPlaces getStopPlaces() {
return stopPlaces;
}
/**
* Sets the value of the stopPlaces property.
*
* @param value
* allowed object is
* {@link AffectedLineStructure.StopPlaces }
*
*/
public void setStopPlaces(AffectedLineStructure.StopPlaces value) {
this.stopPlaces = value;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedRoute" type="{http://www.siri.org.uk/siri}AffectedRouteStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"affectedRoutes"
})
public static class Routes
implements Serializable
{
@XmlElement(name = "AffectedRoute", required = true)
protected List affectedRoutes;
/**
* Gets the value of the affectedRoutes property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the affectedRoutes property.
*
*
* For example, to add a new item, do as follows:
*
* getAffectedRoutes().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedRouteStructure }
*
*
*/
public List getAffectedRoutes() {
if (affectedRoutes == null) {
affectedRoutes = new ArrayList();
}
return this.affectedRoutes;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedSection" type="{http://www.siri.org.uk/siri}AffectedSectionStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"affectedSections"
})
public static class Sections
implements Serializable
{
@XmlElement(name = "AffectedSection", required = true)
protected List affectedSections;
/**
* Gets the value of the affectedSections property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the affectedSections property.
*
*
* For example, to add a new item, do as follows:
*
* getAffectedSections().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedSectionStructure }
*
*
*/
public List getAffectedSections() {
if (affectedSections == null) {
affectedSections = new ArrayList();
}
return this.affectedSections;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedStopPlace" type="{http://www.siri.org.uk/siri}AffectedStopPlaceStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"affectedStopPlaces"
})
public static class StopPlaces
implements Serializable
{
@XmlElement(name = "AffectedStopPlace", required = true)
protected List affectedStopPlaces;
/**
* Gets the value of the affectedStopPlaces property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the affectedStopPlaces property.
*
*
* For example, to add a new item, do as follows:
*
* getAffectedStopPlaces().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedStopPlaceStructure }
*
*
*/
public List getAffectedStopPlaces() {
if (affectedStopPlaces == null) {
affectedStopPlaces = new ArrayList();
}
return this.affectedStopPlaces;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AffectedStopPoint" type="{http://www.siri.org.uk/siri}AffectedStopPointStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"affectedStopPoints"
})
public static class StopPoints
implements Serializable
{
@XmlElement(name = "AffectedStopPoint", required = true)
protected List affectedStopPoints;
/**
* Gets the value of the affectedStopPoints property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the affectedStopPoints property.
*
*
* For example, to add a new item, do as follows:
*
* getAffectedStopPoints().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AffectedStopPointStructure }
*
*
*/
public List getAffectedStopPoints() {
if (affectedStopPoints == null) {
affectedStopPoints = new ArrayList();
}
return this.affectedStopPoints;
}
}
}